MongoDB: The Ideal Non-SQL Database
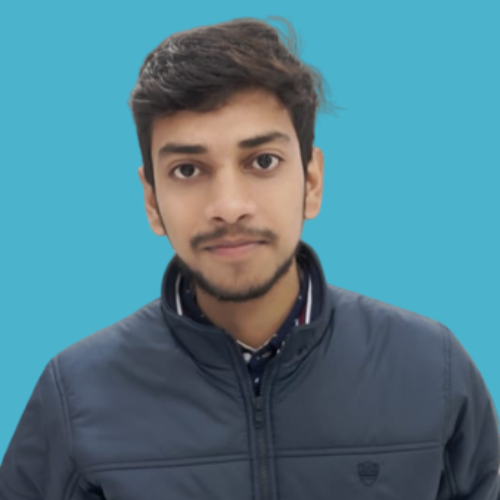
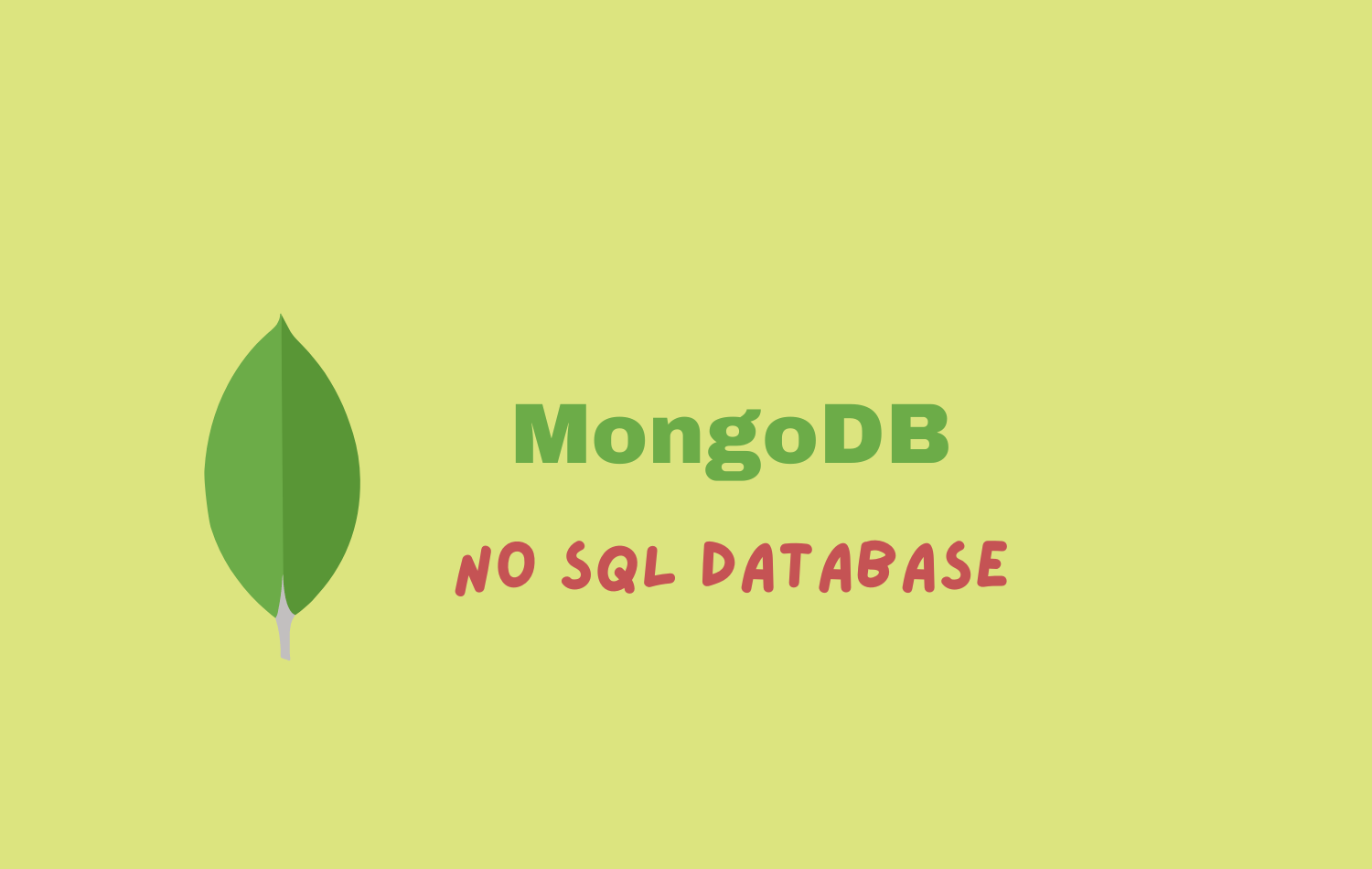
Introduction🚀
MongoDB, Inc. was founded by 10gen, the company that originally developed it. Eliot Horowitz and Dwight Merriman launched the company in 2007. In this blog, you will be able to download MongoDB components and study and use them in your projects. It covers all topics from the fundamentals to advanced & here I include only examples, so for syntax please go to Cheatsheet.
What is MongoDB
MongoDB is a document-oriented database that stores data in BSON (Binary JSON) format, enabling complex, hierarchical data structures. It is renowned for its scalability, flexibility, and excellent performance, making it appropriate for a wide range of applications.
JSON / BSON
JSON | BSON |
It stands for JavaScript Object Notation | It stands for Binary JSON |
a straightforward format for exchanging data that is readable and writeable by people and parsed and generated by machines. | Binary representation of JSON-like documents. BSON expands the JSON model by adding new data types and improving encoding and decoding efficiency across languages. |
Installation⬇️
To get started with MongoDB, you need to install three main components:
MongoDB Compass: A graphical user interface for MongoDB that provides an easy way to visualize and interact with your data.
MongoDB Atlas: A cloud-based database service provided by MongoDB, offering a fully managed database with built-in scalability and high availability.
MongoDB Shell: A command-line interface for MongoDB that allows you to interact with your database using commands.
Installation of Compass, Atlas & Shell
Go to the MongoDB to download all three components & check the video below for reference👇
Understanding MongoDB Atlas & Compass
MongoDB Atlas: Provides a managed environment to deploy, monitor, and maintain your MongoDB clusters. here management of our databases is superb and it also provides security to prevent malpractice.
MongoDB Compass: Allows you to visually explore your data, run queries, and manage indexes. It's a powerful tool for database administrators and developers to use MongoDB data.
Databases & Collections🧐
Databases: A database in MongoDB has a collection set. The file system sends a certain set of files to each database.
Collections: A collection is a set of documents in MongoDB. In relational databases, it is comparable to a table. Documents within a collection may have different fields since collections do not impose a schema.
Note - Please first grab my cheatsheet because I have simply included examples in this blog, which would confuse you.
Connecting to the MongoDB Shell🛰️
Start the MongoDB server:
Depending on your installation, the MongoDB server might start automatically.
If not, refer to your specific OS documentation for instructions on starting the MongoDB service.
Connect to the shell:
Open your terminal and navigate to a directory of your choice.
Type the following command and press Enter:
mongosh
Now Performs operations, starting with command -
show dbs
to check databases in your MongoDB
CRUD Operation🤔
- Insert: Adding new documents to a collection.
db.collection.insertOne({ name: "Alice", age: 25 });
- Find: Retrieving documents from a collection.
db.collection.find({ age: { $gt: 20 } });
- Update: Modifying existing documents in a collection.
db.collection.updateOne({ name: "Nehal" }, { $set: { fees: 2060 } });
- Delete: Removing documents from a collection.
db.collection.deleteOne({ name: "Alice" });
Basic Query Operations
Logical Operator
Logical operators enable you to combine numerous query conditions to filter your MongoDB documents using complicated criteria.
db.collection.find({ $or: [{ age: { $lt: 25 } }, { name: "Alice" }] });
1. $and: Matches documents that satisfy all specified conditions.
db.products.find({
$and: [
{ price: { $gt: 10 } }, // Price greater than 10
{ category: "Electronics" } // Category is "Electronics"
]
});
This query will only return products that are priced above $10 and belong to the "Electronics" category.
2. $or: matches documents that meet one or more of the requirements.
db.users.find({
$or: [
{ age: { $gt: 25 } }, // Age greater than 25
{ country: "USA" } // Country is USA
]
});
This query will return users who are either older than 25 or reside in the USA.
3. $not: Inverts the effect of a sub-query. Matches documents that do not satisfy the inner condition.
db.orders.find({
$not: { status: "cancelled" } // Exclude cancelled orders
});
This query will return all orders except those with a "cancelled" status.
Advanced Query Operations🤯
$all vs $elemMatch
Both $all
and $elemMatch
are used for querying arrays in MongoDB, but they serve different purposes:
$all: Checks if all elements in an array field match a specific condition.
$all
ensures all elements meet the criteria, while$elemMatch
requires only one element to match.db.products.find({ tags: { $all: ["electronics", "sale"] } }); // Find products with both "electronics" and "sale" tags
$elemMatch: Checks if at least one element in an array field matches a specific condition, including embedded documents within the array.
$elemMatch
can be used for complex queries on embedded documents within the array.db.orders.find({ items: { $elemMatch: { name: "Product A", quantity: { $gt: 1 } } } }); // Find orders with "Product A" and a quantity greater than 1
Complex Expressions
You can combine comparison operators and logical operators ($and
, $or
, $not
) to create complex queries that match documents based on multiple conditions.
db.movies.find({
year: { $gt: 2000 },
genre: { $in: ["Action", "Adventure"] }
}); // Find movies after 2000 in Action or Adventure genres
Operators*️⃣
- Comparison Operator: Compare values within documents
db.collection.find({ age: { $ne: 30 } });
- Array Operator: Perform operations on arrays
db.collection.find({ tags: { $all: ["tech", "science"] } });
- Update Operator: Modify documents
db.collection.updateMany({}, { $inc: { age: 1 } });
Comparison Operators
$eq (Equality): finds documents with fields whose values match the given values.
db.products.find({ price: { $eq: 10.99 } });
$ne (Not Equal): Matches documents where a field's value is not equal to the specified value.
db.users.find({ age: { $ne: 25 } });
$gt (Greater Than): Matches documents where a field's value is greater than the specified value.
db.scores.find({ score: { $gt: 80 } });
$gte (Greater Than or Equal): Matches documents where a field's value is greater than or equal to the specified value.
db.inventory.find({ quantity: { $gte: 5 } });
$lt (Less Than): Matches documents where a field's value is less than the specified value.
db.posts.find({ comments: { $lt: 10 } });
$lte (Less Than or Equal): Matches documents where a field's value is less than or equal to the specified value.
db.orders.find({ total: { $lte: 200 } });
$in (In): matches documents whose values in a given field are in the designated list of values.
db.customers.find({ country: { $in: ["USA", "Canada", "UK"] } });
$nin (Not In): Matches documents where a field's value is not included in the specified list of values.
db.products.find({ category: { $nin: ["Electronics", "Clothing"] } });
Updating Arrays & Embedded Documents in MongoDB
1. Update Operators:
$set: This command changes the value of an existing field.
db.users.updateOne({ _id: 1 }, { $set: { name: "John Doe Jr." } }); // Update name
$unset: Updates a single document by removing fieldName.
db.products.updateOne({ _id: 2 }, { $unset: { description: 1 } }); // Remove description
2. Array Update Operators:
$push: Updates a single document by adding a new element to the array array field.
db.orders.updateOne({ _id: 3 }, { $push: { items: { name: "Headphones", quantity: 1 } } }); // Add item to "items" array
$pop: removes the array field's first (value: -1) or last (value: 1) element to update a single document.
db.cart.updateOne({ _id: 4 }, { $pop: { items: -1 } }); // Remove last item from "items" array
$pull: Removes elements from an array that meet a specified condition.
db.inventory.updateOne({ _id: 5 }, { $pull: { outOfStock: { name: "Shirt" } } }); // Remove "Shirt" from outOfStock array
$addToSet: Adds an element to an array only if it does not exist (preventing duplication).
db.tags.updateOne({ name: "Sports" }, { $addToSet: { subcategories: "Basketball" } }); // Add subcategory if not present
3. Positional Operators (for updating specific array elements):
$: Updates the array's first matched element.
db.comments.updateOne({ _id: 6 }, { $set: { "replies.$[elem].author": "Admin" } }, { arrayFilters: [{ "elem.isApproved": true }] }); // Update author of the first approved reply
$[index]: Updates the element at a given index (zero-based).
db.scores.updateOne({ _id: 7 }, { $inc: { "tests.1.score": 5 } }); // Increment score of the second test (index 1)
4. Update with $elemMatch (for updating embedded documents within an array):
db.orders.updateOne(
{ <field>: { $elemMatch: {
prize : 10,
index : 19,
... } } }
);
Cursor Methods
count(): It returns the all numbers of documents that come under the search parameters.
db.users.find().count(); // Count all users
limit(n): Limits the number of documents returned in the cursor to
n
.db.articles.find().limit(10); // Get the first 10 articles
skip(n): Skips the first
n
documents that match the query criteria. Often used withlimit
for pagination.db.orders.find().skip(20).limit(10); // Get orders 21-30
sort({ field1: 1, field2: -1 }): Sorts the results based on the specified fields. 1 for ascending, -1 for descending.
db.products.find().sort({ price: 1, name: -1 }); // Sort by price (ascending) and name (descending)
Element Operators
$exists: Checks if a field exists in a document, regardless of its value.
db.users.find({ email: { $exists: true } }); // Find users with an email field
$type: Returns the data type of a field's value.
db.inventory.find({ quantity: { $type: "number" } }); // Find documents with a numeric "quantity" field
$size: Returns the number of elements in an array field.
db.comments.find({ replies: { $size: { $gt: 2 } } }); // Find comments with more than 2 replies
Projection🔍
Projection allows you to specify which fields to include or exclude when returning documents from a query.
Indexes in MongoDB🎯
Indexes accelerate query operations by allowing efficient access to documents. However, they come with an added cost for storage and maintenance overhead.
When Indexes Should Not Be Used
We should avoid the excessive usage of indexing as it can cause maximize storage and slower processing operations.
Aggregation Framework💯
Aggregation operations combine data records to provide computed results. MongoDB offers a sophisticated aggregation framework for data transformations and computations.
- $group Operator: Groups documents by a specified field and performs aggregations.
db.collection.aggregate([{ $group: { _id: "$age", total: { $sum: 1 } } }]);
- $sort Operator: Sorts documents in a specified order.
db.collection.aggregate([{ $sort: { age: 1 } }]);
- $project Operator: Reshapes documents by including, excluding, or adding fields
db.collection.aggregate([{ $project: { name: 1, age: 1, _id: 0 } }]);
- $unwind Operator: Here single documents break down from an array document that contains large data. Every output document is an input document with the element substituted for the array field's value.
db.people.aggregate([
{
$unwind: "$students"
}
]);
- $match Operator: Filters documents to pass only those that match the specified conditions.
db.collection.aggregate([{ $match: { age: { $gt: 25 } } }]);
- $filter Operator: It filters elements of an array based on specific conditions
db.collection.aggregate([{ $filter: { age: { $gt: 25 } } }]);
- $addToSet Operator: Adds elements to an array field while preventing duplicates
db.collection.updateOne({ name: "Sports" }, { $addToSet: { subcategories: "Basketball" } }); // Add subcategory if not present
- $project Operator: used to specify which fields to include or exclude from the documents passed through the aggregation pipeline. It allows you to reshape the output documents by including existing fields, Excluding fields, Renaming fields, Creating new fields, and Resetting field values.
db.products.aggregate([
{
$project: {
_id: 0, // Exclude _id for clarity
name: 1, // Include name field
price: 1, // Include price field
category: { $toUpper: "$category" } // Rename and uppercase category
}
}
]);
Importing JSON In MongoDB📥
To import the JSON file of your document
Command -
mongoimport jsonfile.json -d database_name -c collection_name
To import the Array JSON file of your document
Command -
mongoimport jsonfile.json -d database_name -c collection_name --jsonArray
Note - There is a limit to imports of 16 MB or less
Conclusion
This article provides an in-depth guide to MongoDB, a document-oriented database, covering installation, key features, CRUD operations, and essential concepts like databases, collections, indexes, and aggregation framework, making it a valuable resource for both beginners and advanced users.
Follow Me On Socials :
Like👍| Share📲| Comment💭
Subscribe to my newsletter
Read articles from MOHD NEHAL KHAN directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
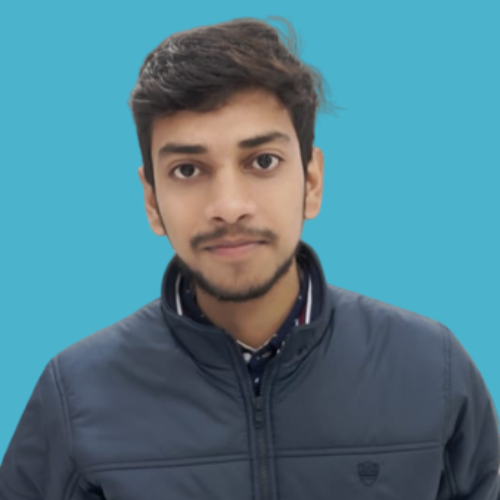
MOHD NEHAL KHAN
MOHD NEHAL KHAN
I am a student at Manipal University Jaipur and a web developer, learning and exploring tech stack in web development and implementing in my projects