Understanding Rendering In Next js
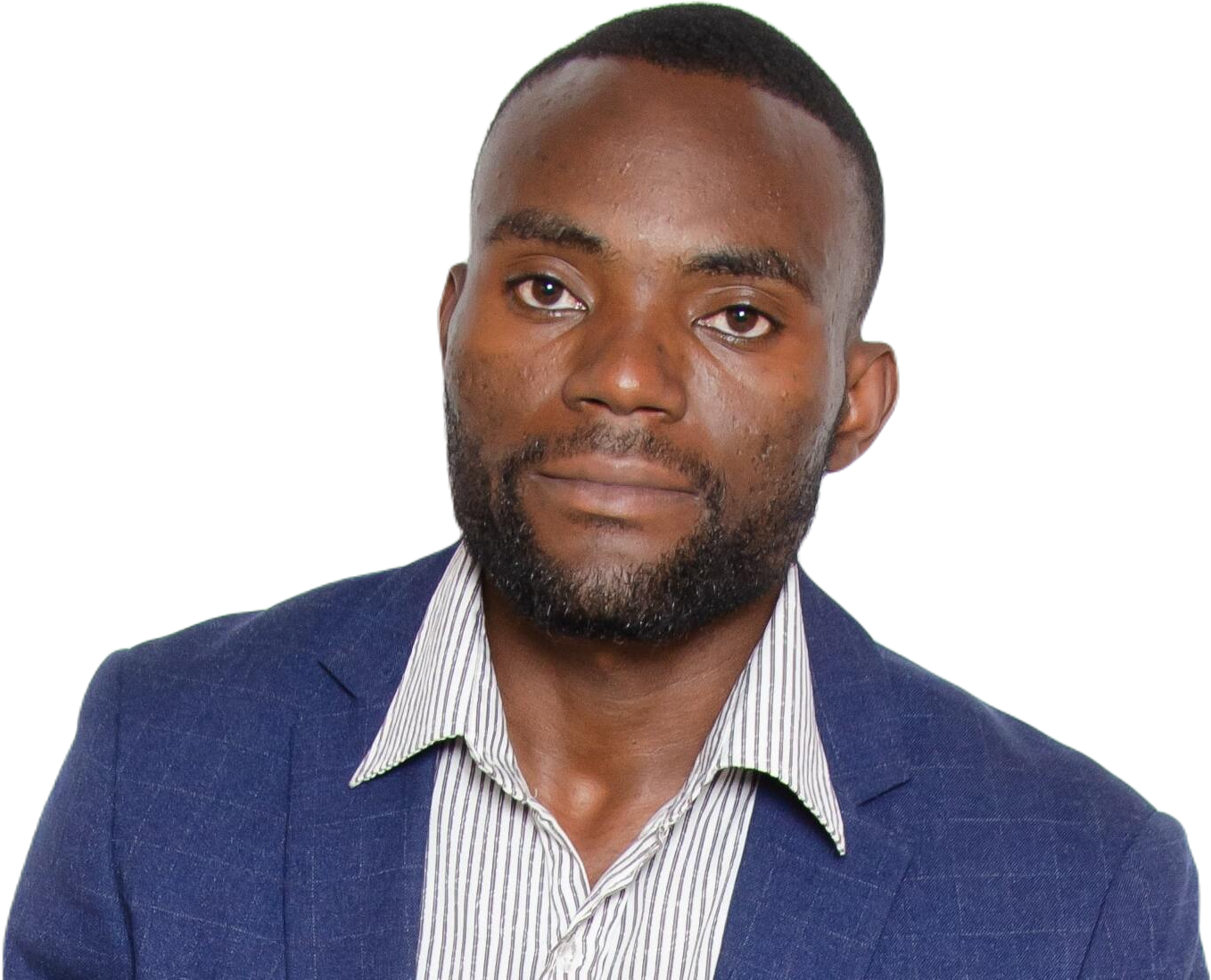
Rendering in the NextJS framework is one of the powerful feature that can be used to optimize application performance, improve search engine optimization (SEO), and improve the user experience. NextJS offers basically four types of rendering techniques, including client-side rendering (CSR), server-side rendering (SSR), and incremental regeneration rendering (ISR).
Before we discuss each of these rendering strategies, let's understand what rendering is.
What is Rendering?
Rendering is the process of converting code and components into viewable or interactive web content. NextJS allows the developer to create hybrid applications that can be rendered on a client or server.
Client Side Rendering (CSR)
Client-side rendering (CRS) uses javascript to render the website or application. The process of rendering content happens in the browser. The CRS is mostly used in highly interactive applications. In context to NextJS, client-side rendering can be used to create react components that require dynamic interactions and browers Apis. These components are referred to as client components.
"use client";
import { useState } from "react";
export default function Counter() {
const [count, setCount] = useState<number>(0);
const handleIncrement = () => {
setCount((prevState) => prevState + 1);
};
return (
<div>
<p>Counter {count}</p>
<button onClick={handleIncrement}>Click me</button>
</div>
);
}
Server Side Rendering (SSR)
Basically, server-side rendering is a technique used to render the web page on the server and send a fully rendered page to the users. This rendering technique offers a number of benefits.
Faster load time. A server-side rendered application speeds up page loading. Thus, it greatly improves the whole user experience.
Indexation by search engines. As the content can be rendered before the page is loaded when rendered server-side, search engines can easily index and crawl it.
Here is an example of fetching posts using SSR:
import React from "react";
const getPosts = async () => {
const res = await fetch("https://jsonplaceholder.typicode.com/posts", {
method: "GET",
headers: {
"Content-type": "application/json",
},
});
if (!res.ok) {
throw new Error("Failed to get posts");
}
return await res.json();
};
const PostList = async () => {
const posts = await getPosts();
return (
<div className="flex flex-col gap-4">
{posts.map(({ id, title }) => (
<div key={id}> {title}</div>
))}
</div>
);
};
export default PostList;
Incremental Static Regeneration (ISR)
This approach allows the developer to create and update the web content without redeploying the entire application.
using ISR offer several benefits
Better Performance: Static pages are consistently fast because ISR allows Vercel to cache generated pages on the global edge network and persist files into durable storage.
Reduced Backend Load: ISR reduces backend load by using cached content to make fewer requests to your data sources.
Faster Builds: Pages can be generated when requested by a visitor or through an API instead of during the build, speeding up build times as your application grows.
Here is an example of fetching posts using ISR:
import React from "react";
const getPosts = async () => {
const res = await fetch("https://jsonplaceholder.typicode.com/posts", {
method: "GET",
headers: {
"Content-type": "application/json",
},
// Revalidate at most every hour
next: { revalidate: 3600 },
});
if (!res.ok) {
throw new Error("Failed to get posts");
}
return await res.json();
};
const PostList = async () => {
const posts = await getPosts();
return (
<div className="flex flex-col gap-4">
{posts.map(({ id, title }) => (
<div key={id}> {title}</div>
))}
</div>
);
};
export default PostList;
Conclusion
Rendering in NextJS, whether it’s CSR, SSR, or ISR, plays a crucial role in optimizing application performance, improving SEO, and enhancing the user experience. By understanding and leveraging these rendering techniques, developers can create highly efficient and scalable applications.
Benefits and Trade-offs
Knowing the benefits and trade-offs of each rendering technique is important to make informed decisions about when to use one over the other.
Client-Side Rendering (CSR)
Benefits: Highly interactive applications, faster initial load for subsequent navigation, dynamic content updates.
Trade-offs: Slower initial load time, potential SEO challenges.
Server-Side Rendering (SSR)
Benefits: Faster initial load time, better SEO as content is pre-rendered, improved performance for static content.
Trade-offs: Higher server load, longer time to interactive as the server must render the page for each request.
Incremental Static Regeneration (ISR)
Benefits: Fast performance with static pages, reduced backend load due to caching, faster build times for large applications.
Trade-offs: Complexity in managing revalidation, initial content might be outdated until the next regeneration.
Understanding these benefits and trade-offs will help you choose the most appropriate rendering strategy for your NextJS applications, ensuring the best balance between performance, scalability, and user experience.
Subscribe to my newsletter
Read articles from Kennedy Ngosa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
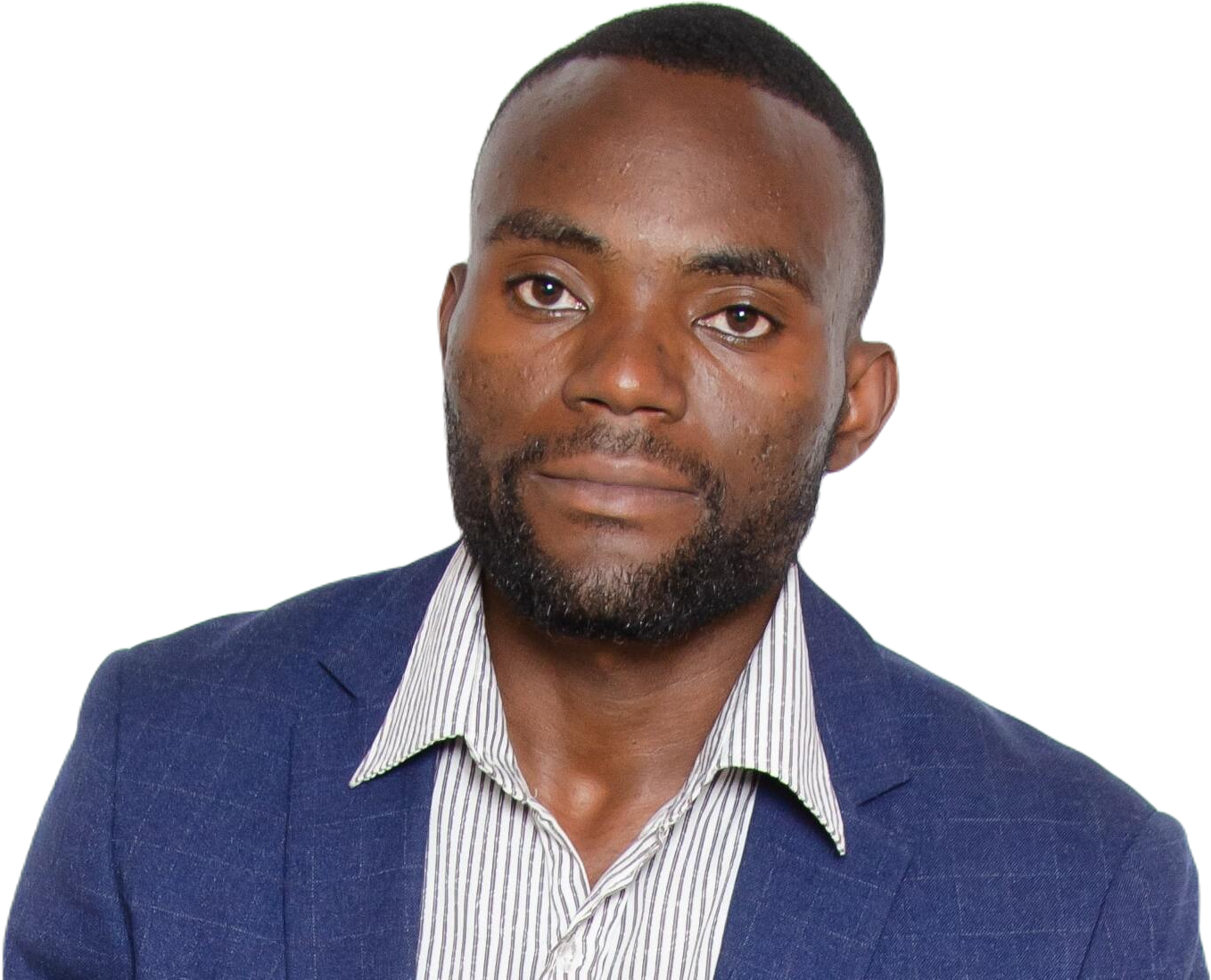