👮 What The Hell Is Csp?
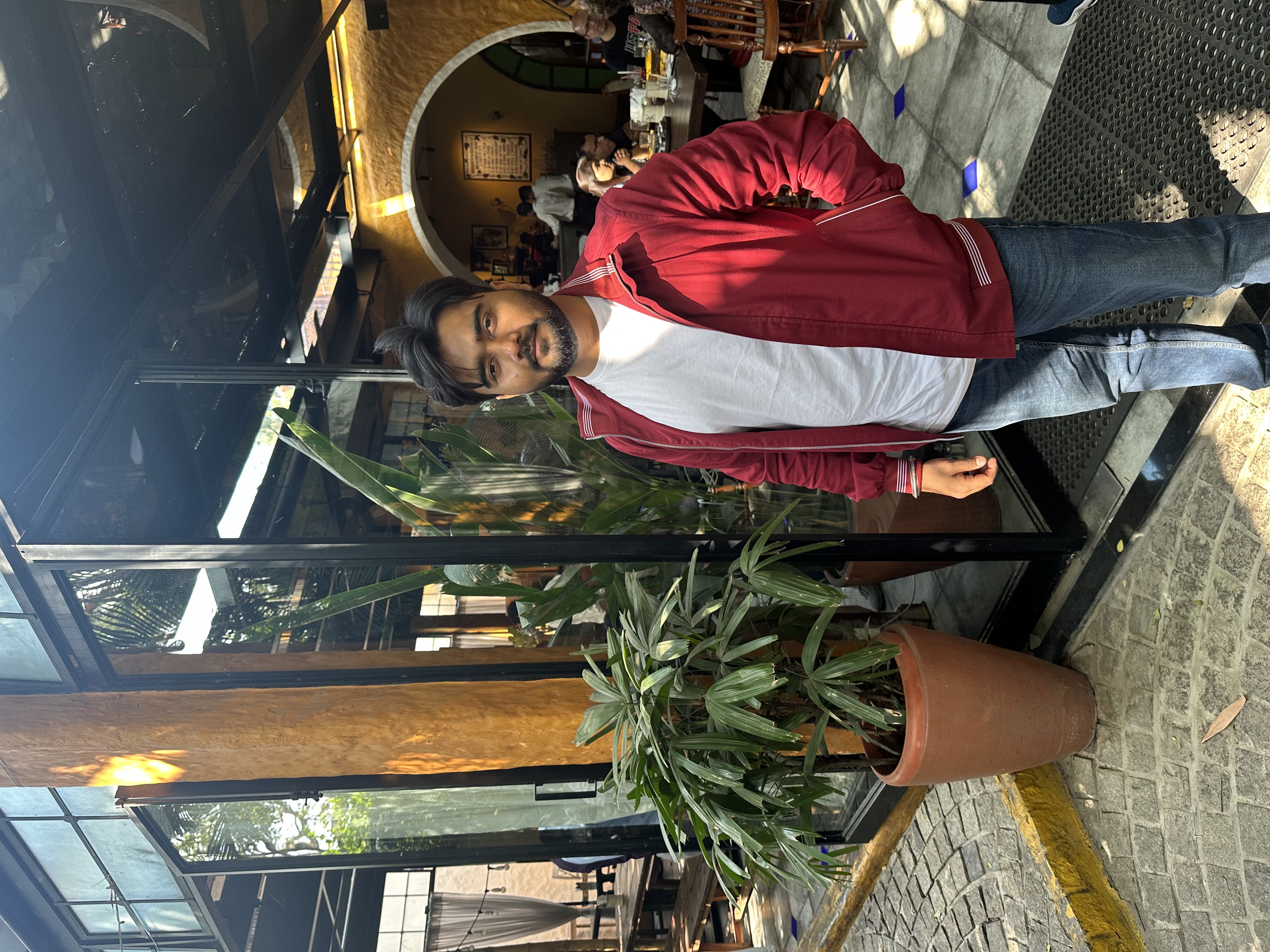
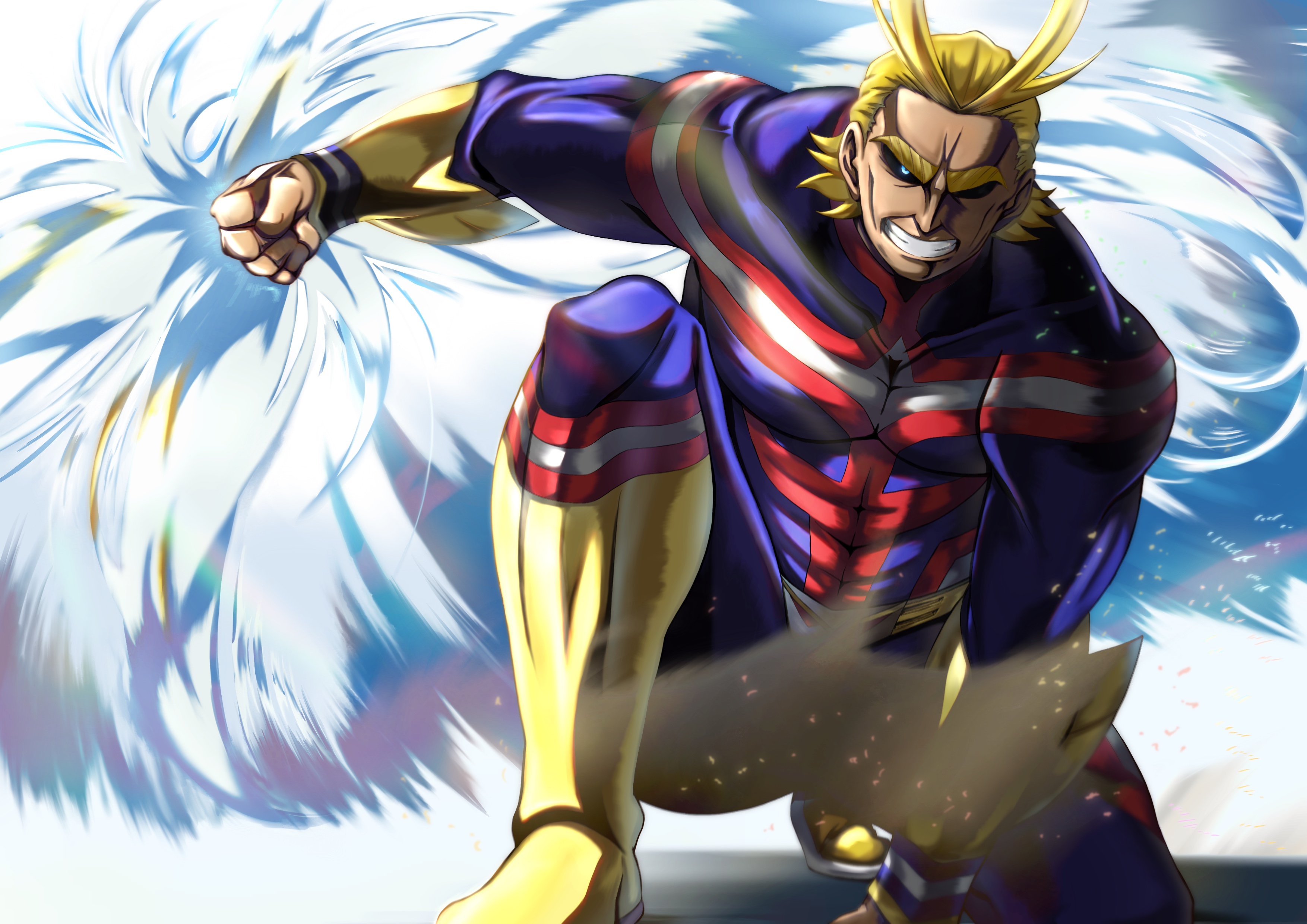
So, you're planning to switch to a Senior Frontend Developer role. Aiming for that 2000% salary hike at a startup or FAANG, huh?
If you don’t know this, I think you should re-consider and pull the brakes.
Today, I'll show you how to prevent your application from getting nuked by XSS.
SO what is XSS?
For all the brilliant people out there, it's cross-site scripting—or,
in simple terms,the injection of scripts into a browser.
Yes, you heard it right; hackers can inject scripts into the input box that took you 2 hours to create, meticulously designed with every UI element from your designer's thoughtful Figma file.
That script typically gets injected and saved in a database until you learn how to implement CSP.
👾 CSP SYNTAX
CSP helps to prevent XSS (CONTENT SECURTIY POLICY)
How do you protect your phone from getting access by your parents from accessing it , simple answer by providing a password.
Similarly CSP let's you set a list(password) of the addresses from where the resources can be imported & used.
For all the resources whether it be CSS,SCRIPTS,FONTS,iframe etc..
Content-Security-Policy: default-src 'self';Content-Security-Policy: default-src 'self'; img-src 'self' cdn.examplecsp.com;
Let's breakdown the RHS of above content-security-header
default-src
is the name of the directive.(you don't need to learn each directive name, All Directives List)The second item is the value to the mentioned directive
'self'
: lt means content coming should be from the site's own origin.You can add more sources to a directive like adding any custom URL from which you want to fetch content, separated by a space, after
self
.
You can use multiple directives separated with a semi-colon , each directive again can have multiple sources.
COMMONLY USED DIRECTIVES
Here's the link to all the possible directives and the key values they can accept, still let me mention some of the commonly used directives (All Directives List)
Fetch Directives: It controls from which links resources can be fetched & used.
img-src
script-src
frame-src
font-src
Navigation Directives: It generally handles where user can submit a certain a form or navigate to a URL provided.
form-action
: Restricts the URLs which can be used as the target of a form submissions from a given context.frame-ancestors
: Specifies valid parents that may embed a page using <frame><ifram>, <object>, or <embed>.
KEY VALUES
none
: Won't allow loading of any resources.self
: Only allow resources from the current origin.strict-dynamic
: The trust granted to a script in the page due to an accompanying nonce or hash is extended to the scripts it loads.unsafe-inline
: Allow use of inline resources.unsafe-hashes
: Allows enabling specific inline event handlers.
HOW TO SETUP CSP
It is generally setup in the HTTP header of the response received from the server.But you can also implement this in the FE side either using meta tag or Javascript.
Using HTTP HEADER
Here's a sample code of a server written in node.js
app.use((req, res, next) => {
let policyValue;
// Example conditions
if (req.path.startsWith('/admin')) {
// More restrictive policy for admin routes
policyValue = "default-src 'self'; script-src 'self' https://admin-tech-blog.com;";
} else if (req.query.allowExternalScripts === 'true') {
// Less restrictive policy allowing external scripts based on query parameter
policyValue = "default-src 'self'; script-src 'self' https://user-tech-blog.com https://external-style-scripts.com;";
} else {
// Default policy for all other routes
policyValue = "default-src 'self'; script-src 'self';";
}
// Set the CSP header dynamically
res.setHeader('Content-Security-Policy', policyValue);
next();
});
Using META TAG
<meta http-equiv="Content-Security-Policy" content="default-src https://www.user-tech.com" />
💰 BONUS (Google Analytics GA4 EXAMPLE)
GA4 is extensively used to measure traffic and engagement across web apps and websites. If you are using GA4, following this step is crucial. I've also implemented GA4 in a tool I developed at the startup where I work.
google-analytics config file : Let's assume the name of this file isga-config-file.js
window.dataLayer = window.dataLayer || [];
function gtag(){dataLayer.push(arguments);}
gtag('js', new Date());
gtag('config', 'YOUR_GTAG_ID'); // paste your own gtag id
// this is generally provided
// when you setup GA4;no need to worry
HTML CODE
<script async src="https://www.googletagmanager.com/gtag/js?id=YOUR_GTAG_ID"></script>
<script src="/ga-config-file.js"></script>
CSP Policy related to this will be
<meta http-equiv="Content-Security-Policy" content="script-src 'self' www.googletagmanager.com/gtag/js; connect-src www.google-analytics.com;"/>
BREAKING DOWN THE CSP POLICY
script-src
: It has 2 values ,
self
keyword allows the/ga-config-file.js
script.Policy also allows any script to load on the www.googletagmanager.com domain.
connect-src
: It allows to restrict the data to be loaded from the mentioned scripts only.
This policy allows google analytics to make a XHR request under the domain www.google-analytics.com in order to send the analytics data.Just in case google plans to change this endpoint , we need to update here as well.
There's another Crucial Topic NONCE ,which I'll try to explain in the second part of it.
Untill than let's push our limits and try to become a better dev.
SAYONARA !!!
Subscribe to my newsletter
Read articles from Chetanya Tomar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
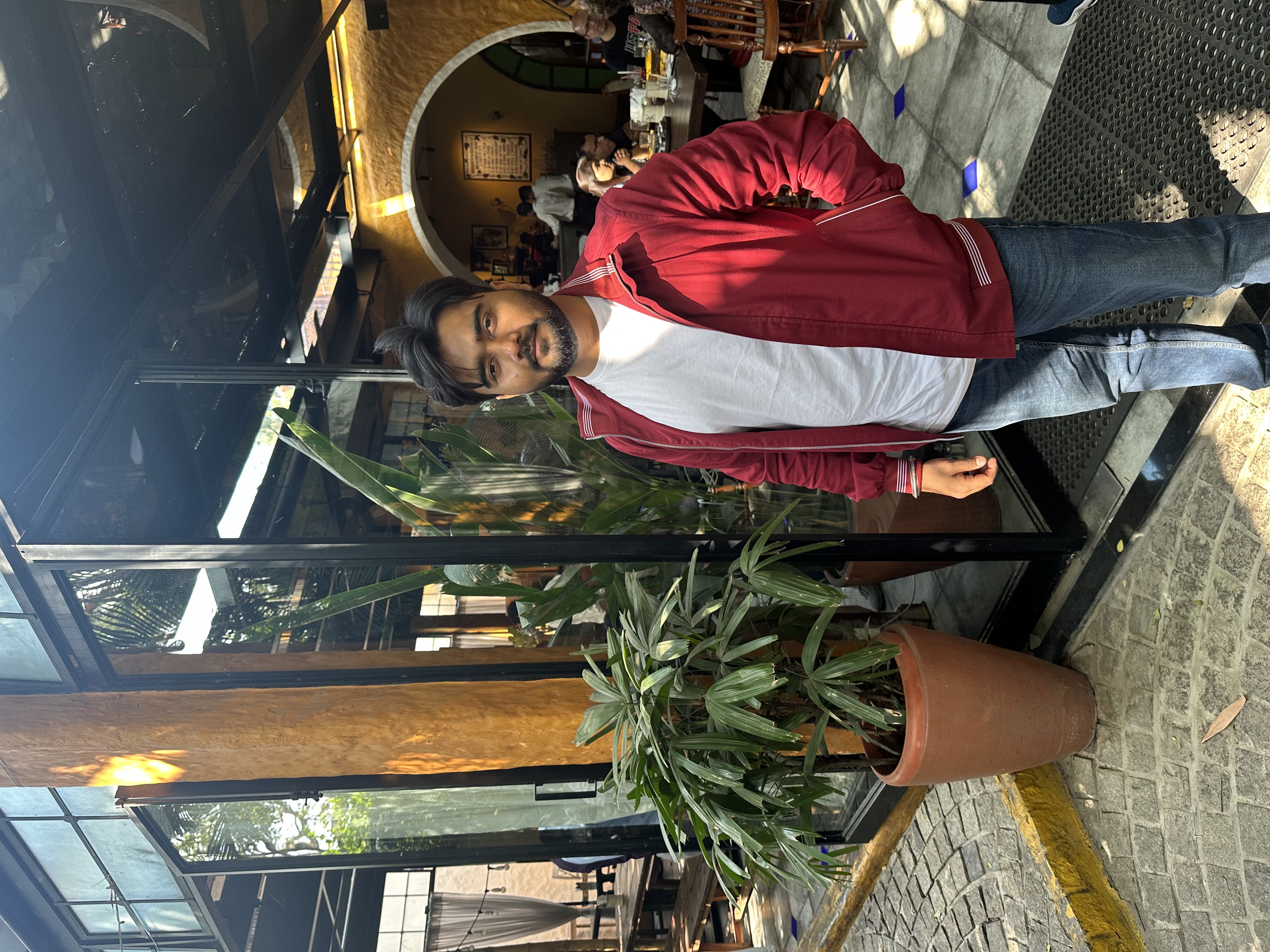
Chetanya Tomar
Chetanya Tomar
A Frontend Dev by profession & an artist by heart. Have a knack for learning & building cool things from scratch.