Understanding flutter widgets: Stateless and Stateful Widgets
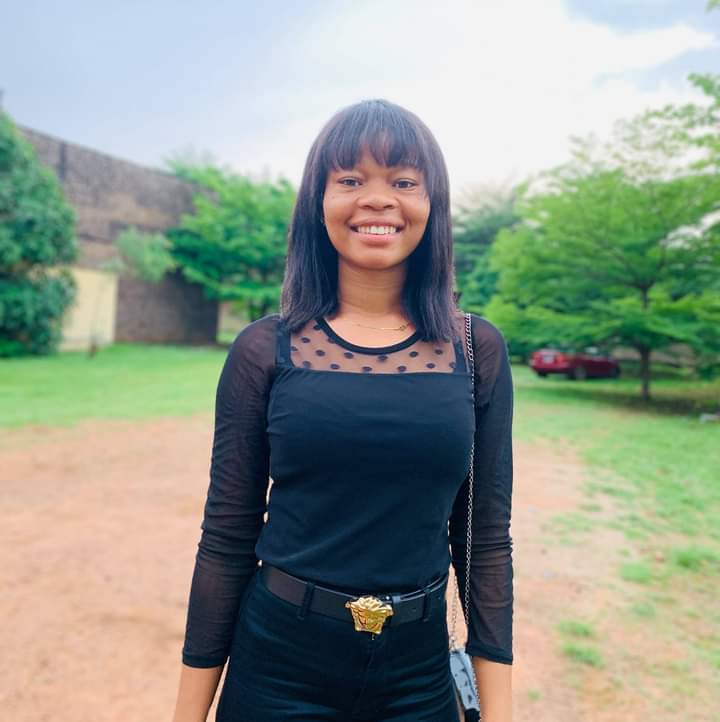
Flutter is an open-source UI software development kit created by Google. It is a cross-platform framework that uses a single codebase to build applications for different platforms such as Windows, macOS, Android, and iOS. The flutter framework is described as a blueprint of widgets, it has two main widgets which are stateful and stateless widgets.
The Stateless and stateful widgets are concepts commonly used in user interface development, particularly in frameworks like Flutter and React. They refer to how widgets manage and update their internal state, which affects how they respond to user interactions and changes in data.
In this article, we will be outlining the core difference between the stateless and stateful Widgets.
Stateless Widgets:
Immutable: Stateless widgets are immutable, meaning their properties (parameters) are set when they are created and cannot change during their lifetime.
No Internal State: They do not have any mutable (changeable) internal state variables. They rely solely on the data passed to them by their properties.
Rebuild Completely: When a change occurs, a new instance of a stateless widget is created with updated properties. This makes them highly efficient since there's no need to track changes or manage state.
Example: A static button that doesn't change its appearance or behavior based on user interactions or data changes.
Stateful Widgets:
Mutable State: Stateful widgets can hold mutable (changeable) state within themselves. This state can change over time in response to user interactions, timers, or other factors.
Internal state Management: They have a mechanism for managing their internal state, typically using a state object. This state object can be updated and rebuilt independently of the parent widget.
Rebuild Partially: When the internal state of a stateful widget changes, only the part of the widget that needs to be updated is rebuilt. This can be more efficient than rebuilding the entire widget.
Example: A button that changes name when pressed.
Here's a simple code example in Flutter to illustrate the difference:
Stateless widget:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
//USING STATELESSWIDGET
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('STATELESS WIDGET EXAMPLE'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Queen'),
Text('Anthony'),
],
),
),
),
);
}
}
Stateful Widget:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
//USING THE STATEFULWIDGET
class MyApp extends StatefulWidget {
@override
MyAppState createState() => MyAppState();
}
class _MyAppState extends State<MyApp> {
String nameOne = 'Queen';
String nameTwo = 'Anthony';
//the setState rebuilds the UI
void _changeName() {
setState(() {
nameTwo = 'TheQueen';
});
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Two Names'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(nameOne),
Text(nameTwo),
ElevatedButton(
onPressed: _changeName,
child: Text('Change Name'),
),
],
),
),
),
);
}
}
Hello, my name is Queen Anthony I’m a mobile app developer who loves writing and a tech enthusiast who believes in using codes to make life easier. I’m currently building my tech career as a mobile app developer, I use the flutter framework because of its ability create beautiful UIs and its specialty in using one code base to build apps for multiple platforms. I have not yet reached the peak of my career, so I continually look for ways to improve.
In my quest for growth, I discovered HNG an internship program that helps techies grow their tech careers while working on real life projects and collaborating with others. I applied and I’m glad to say I would be starting this journey of growth with HNG, I’m looking forward to learning new approaches while completing my tasks, to collaborate with others in a team and most importantly to master working under pressure.
You can get more information about HNG;
Subscribe to my newsletter
Read articles from Queen Anthony directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
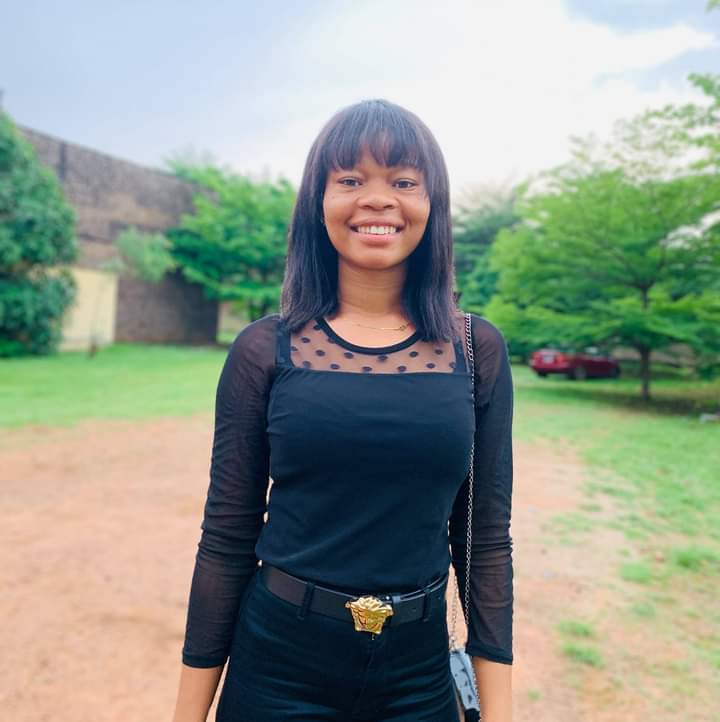