Mastering GitHub: Essential Commands and Tips

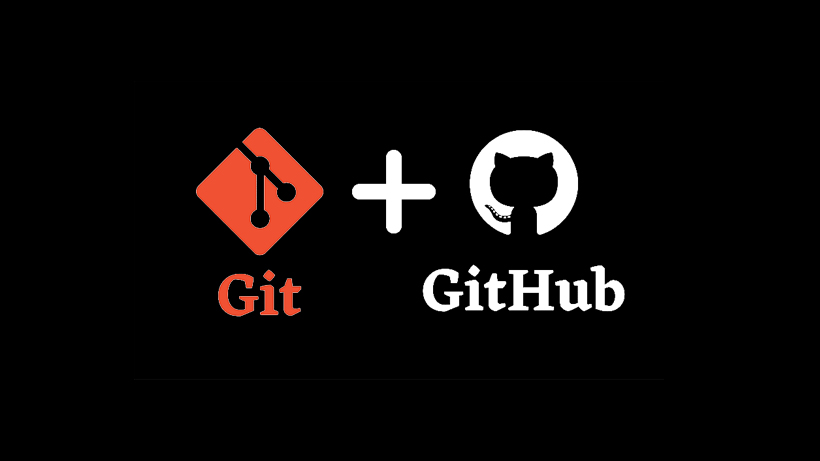
Git & GitHub is a powerful tool for version control and collaboration. Here's a guide to essential Git commands and configurations to get you started.
Git Workflow
Working Directory/Workspace: This is where you make changes to your files.
Staging Area/Index: This is where you prepare your changes before committing.
Local Repository: This is your local version of the project.
Remote Repository (GitHub): This is where you share your project with others.
Git Configuration
Before you start, configure Git with your details:
git config --list
git config --local --list
git config --global --list
git config --system --list
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
git config --global --unset user.name
Adding New Files
To add new files to your project:
ls # List files in the directory
ls -a # List all files, including hidden ones
touch day1.txt # Create a new file
open day1.txt # Open the file to edit (use start on Windows)
git status # Check the status of your files
pwd # Show current directory
cd .. # Move up one directory
Staging and Un-staging Files
To stage your changes:
git add filename # Add a specific file
git add -A # Stage all changes in the directory and subdirectories
git add . # Stage all changes in the directory
git add *.js # Stage all JavaScript files in the directory
git add **/*.js # Stage all JavaScript files in the directory and subdirectories
To check differences:
git diff # Show changes
Committing Changes
To commit your changes:
git commit -m "Your commit message" # Commit with a message
git commit -am "Your commit message" # Stage and commit all changes
git log # View commit history
To undo commits:
git reset --soft HEAD^ # Undo last commit, keep changes staged
git reset HEAD^ # Undo last commit, unstage changes
git reset --hard HEAD^ # Undo last commit, discard changes
git reset --soft HEAD~2 # Undo last 2 commits, keep changes staged
To exit 'git log':
: + q or ctrl + q
Branching and Merging
To manage branches:
git branch # List branches
git branch feature1 # Create a new branch
git checkout feature1 # Switch to a branch
git branch -d feature1 # Delete a branch
git checkout -b feature1 # Create and switch to a new branch
To merge branches:
git checkout main # Switch to the main branch
git merge feature1 # Merge feature1 into main
git pull # Pull changes from remote
.gitignore File
To ignore files and directories:
touch .gitignore # Create .gitignore file
start .gitignore # Open .gitignore to edit
# Examples of what to include:
.env
*.txt # Ignore all .txt files
!story.txt # Exception: don't ignore story.txt
node_modules/ # Ignore the node_modules folder
temp/
Remote Repositories
To manage remote connections:
git remote # Check remote connections
git remote -v # Show remote URLs
git remote add origin <REMOTE_URL> # Add a remote repository
If remote origin already exists
then your local repository is already connected to a GitHub repository. If you see that the remote URL needs to be updated, you can modify it with:
git remote set-url https://github.com/user-name/folder.git
After setting or confirming the correct URL, you can push your commits:
git push -u origin main
To remove a directory:
rm -rf directory_name # Remove a directory
Pushing and Pulling Changes
To push changes:
git add .
git commit -m "Your message"
git push -u origin main # Push changes to the main branch
To pull changes:
git pull
Forking and Cloning
To contribute to other projects:
Fork > Clone > Make changes > Push > Pull request
Setting Up SSH Keys
To set up SSH keys:
mkdir ~/.ssh # Create .ssh directory
ssh-keygen -t ed25519 -C "your_email@example.com" # Generate SSH key
cat ~/.ssh/id_ed25519.pub # Show the public key
# Copy the key and add it to GitHub under Settings > SSH and GPG keys
Useful Keyboard Shortcuts
ctrl + L # Clear the terminal
cd /g # Change partition path in terminal
With these commands and tips, you'll be well on your way to mastering Git and GitHub. Happy coding!
Subscribe to my newsletter
Read articles from Mahmudul Hasan Nisat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mahmudul Hasan Nisat
Mahmudul Hasan Nisat
I am deeply committed to continuous learning and keeping up with the latest developments in DevOps, ML, and cloud technologies. Beyond my technical skills, I actively engage in open-source contributions and participate in the broader tech community. I am also passionate about mentoring and helping others harness technology to drive positive impact.