Simplifying State Management in React with "useContext"
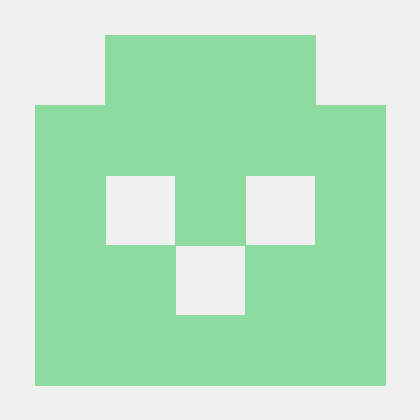
In a React application, passing data from parent components to child components using props is a common practice. However, as your application grows, managing state and passing props through multiple layers of components can become cumbersome and error-prone. This is where the React Context API, specifically the useContext
hook, can simplify state management and make your code more maintainable.
Passing Props
Consider a scenario where you have a deeply nested component tree, and you need to pass a piece of state from a top-level component down to a component several levels deep. Here’s an example:
// App.js
import React, { useState } from 'react';
import ParentComponent from './ParentComponent';
const App = () => {
const [data, setData] = useState("Hello from App");
return (
<div>
<ParentComponent data={data} />
</div>
);
};
export default App;
// ParentComponent.js
import React from 'react';
import ChildComponent from './ChildComponent';
const ParentComponent = ({ data }) => {
return (
<div>
<ChildComponent data={data} />
</div>
);
};
export default ParentComponent;
// ChildComponent.js
import React from 'react';
const ChildComponent = ({ data }) => {
return (
<div>
<p>{data}</p>
</div>
);
};
export default ChildComponent;
In this example, the data
prop is passed from the App
component through ParentComponent
to ChildComponent
. As the number of components grows, passing props through each layer can become increasingly tedious and challenging to maintain.
Simplifying with useContext
The React Context API, combined with the useContext
hook, offers a cleaner solution. By creating a context, you can avoid prop drilling and provide data directly to the components that need it.
First, let's create a context and a provider component:
// context.js
import React, { createContext, useState } from 'react';
const DataContext = createContext();
const DataProvider = ({ children }) => {
const [data, setData] = useState("Hello from App");
return (
<DataContext.Provider value={{ data, setData }}>
{children}
</DataContext.Provider>
);
};
export { DataContext, DataProvider };
Now, we can wrap our application in the DataProvider
and use the useContext
hook to access the data in any component:
jsxCopy code// App.js
import React from 'react';
import ParentComponent from './ParentComponent';
import { DataProvider } from './context';
const App = () => {
return (
<DataProvider>
<div>
<ParentComponent />
</div>
</DataProvider>
);
};
export default App;
// ParentComponent.js
import React from 'react';
import ChildComponent from './ChildComponent';
const ParentComponent = () => {
return (
<div>
<ChildComponent />
</div>
);
};
export default ParentComponent;
// ChildComponent.js
import React, { useContext } from 'react';
import { DataContext } from './context';
const ChildComponent = () => {
const { data } = useContext(DataContext);
return (
<div>
<p>{data}</p>
</div>
);
};
export default ChildComponent;
With this approach, the data
state is provided by DataProvider
and accessed directly in ChildComponent
using useContext
. This eliminates the need to pass props through each intermediate component.
Passing Functions with useContext
In addition to passing variables, you can also pass functions through context to manage state updates. For example:
// ChildComponent.js
import React, { useContext } from 'react';
import { DataContext } from './context';
const ChildComponent = () => {
const { data, setData } = useContext(DataContext);
const updateData = () => {
setData("Updated data from ChildComponent");
};
return (
<div>
<p>{data}</p>
<button onClick={updateData}>Update Data</button>
</div>
);
};
export default ChildComponent;
Here, the ChildComponent
can update the data
state directly by using the setData
function provided by the context.
Conclusion
Using the React Context API and the useContext
hook can significantly simplify state management in your application. By avoiding prop drilling, you make your code cleaner, more readable, and easier to maintain. Whether you're passing variables or functions, useContext
provides a powerful tool for managing state across your React components.
By adopting this approach, you'll find that your codebase becomes more scalable and manageable, especially as your application grows in complexity. Get to it!
Subscribe to my newsletter
Read articles from Jacob Gonzales directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
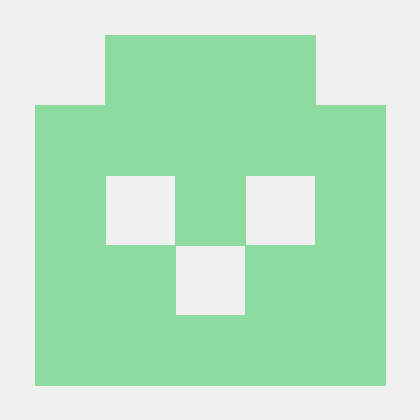