How to Generate Barcodes in Python
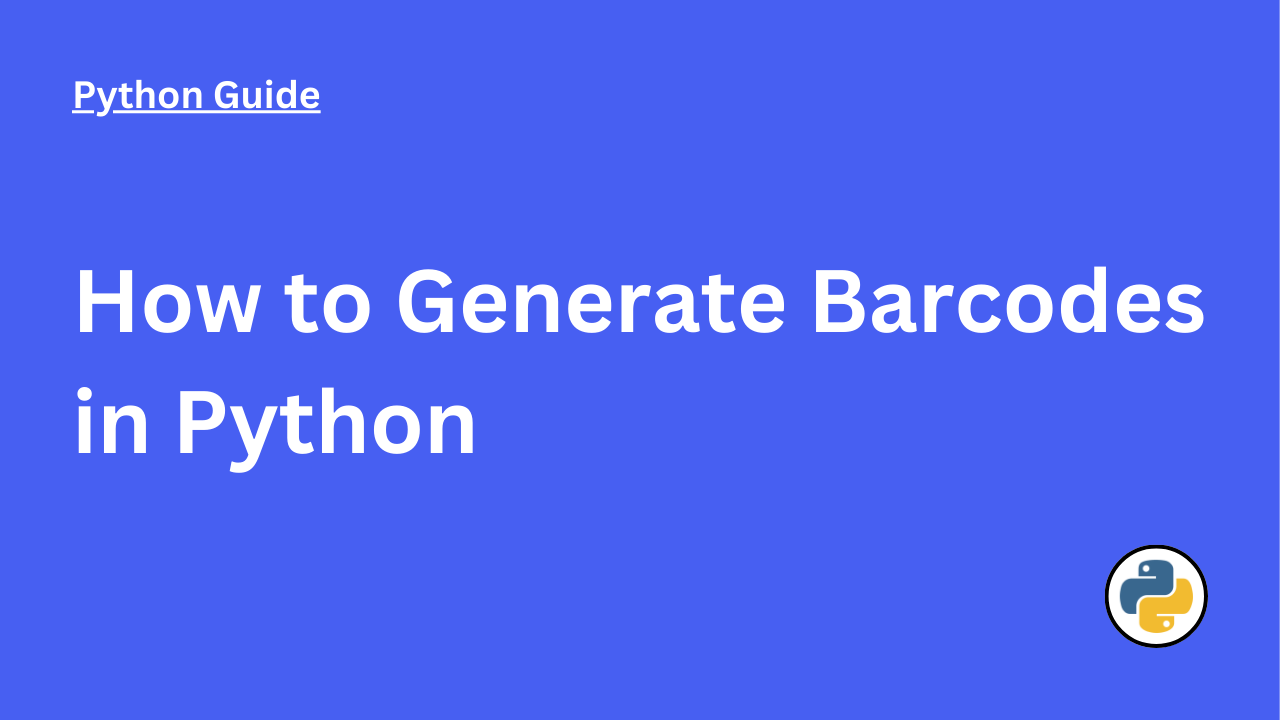
Barcodes are widely used in various industries for tracking products, managing inventory, and more. Creating barcodes programmatically can be extremely useful for automation and integration into software applications. In this tutorial, we will explore how to make a barcode generator in Python using the python-barcode
library.
Introduction to Barcodes
Barcodes are machine-readable representations of data. They are commonly used to encode information such as product numbers, serial numbers, and batch numbers. Barcodes come in various formats, including the widely recognized EAN-13, Code 128, and QR codes.
Python, being a versatile language, offers libraries that simplify the creation and customization of barcodes. One such library is python-barcode, which supports a variety of barcode formats and provides easy-to-use functions for generating and customizing barcodes.
Setting Up the Environment
Before we start generating barcodes, ensure that you have Python installed on your system. You can download the latest version of Python from the official Python website.
We also recommend using a virtual environment to manage dependencies. You can create and activate a virtual environment using the following commands:
# Create a virtual environment
python -m venv barcode_env
# Activate the virtual environment
# On Windows
barcode_env\Scripts\activate
# On macOS/Linux
source barcode_env/bin/activate
Installing the python-barcode
Library
The python-barcode
library can be installed using pip
. Open your terminal and run the following command:
pip install python-barcode
Generating Simple Barcodes
Once the library is installed, generating barcodes is straightforward. The following example demonstrates how to generate a simple EAN-13 barcode:
Example:
import barcode
from barcode.writer import ImageWriter
# Specify the type of barcode
EAN = barcode.get_barcode_class('ean13')
# Generate the barcode
ean = EAN('123456789102', writer=ImageWriter())
# Save the barcode as an image file
ean.save('ean13_barcode')
In this example, we use the ImageWriter
class to generate the barcode as an image file. The barcode is saved as ean13_barcode.png
in the current working directory.
Customizing Barcodes
The python-barcode
library allows customization of various barcode properties, such as text alignment, font size, and background color. Here’s an example of how to customize a barcode:
Example:
import barcode
from barcode.writer import ImageWriter
# Specify the type of barcode
EAN = barcode.get_barcode_class('ean13')
# Create the barcode with custom settings
options = {
'module_width': 0.2,
'module_height': 15.0,
'font_size': 10,
'text_distance': 5.0,
'background': 'white',
'foreground': 'black',
'write_text': True
}
# Generate the barcode with custom options
ean = EAN('123456789102', writer=ImageWriter(), writer_options=options)
# Save the barcode as an image file
ean.save('custom_ean13_barcode')
In this example, we customize the barcode by specifying options for module width, module height, font size, text distance, background color, and foreground color.
Saving Barcodes to Files
The python-barcode
library supports saving barcodes in various file formats, including PNG, SVG, and PDF. By default, the library saves barcodes as PNG images when using ImageWriter
. You can also save barcodes in other formats as shown below:
Saving as SVG:
import barcode
# Specify the type of barcode
EAN = barcode.get_barcode_class('ean13')
# Generate the barcode
ean = EAN('123456789102')
# Save the barcode as an SVG file
ean.save('ean13_barcode_svg')
Saving as PDF:
import barcode
from barcode.writer import PDFWriter
# Specify the type of barcode
EAN = barcode.get_barcode_class('ean13')
# Generate the barcode
ean = EAN('123456789102', writer=PDFWriter())
# Save the barcode as a PDF file
ean.save('ean13_barcode_pdf')
Advanced Customizations
For more advanced customizations, you can create your own writer class by extending the ImageWriter
class. This allows you to modify the way barcodes are generated and saved.
Example of Custom Writer:
import barcode
from barcode.writer import ImageWriter
class CustomImageWriter(ImageWriter):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.text_distance = 3.0
self.font_size = 12
# Specify the type of barcode
EAN = barcode.get_barcode_class('ean13')
# Generate the barcode using the custom writer
ean = EAN('123456789102', writer=CustomImageWriter())
# Save the barcode as an image file
ean.save('custom_writer_ean13_barcode')
Practical Use Cases
Inventory Management System
In an inventory management system, barcodes can be used to label products and track their movement. Here’s an example of generating barcodes for a list of product codes:
import barcode
from barcode.writer import ImageWriter
def generate_barcodes(product_codes):
EAN = barcode.get_barcode_class('ean13')
for code in product_codes:
ean = EAN(code, writer=ImageWriter())
ean.save(f'barcode_{code}')
# List of product codes
product_codes = ['123456789102', '987654321098', '112233445566']
# Generate barcodes for each product code
generate_barcodes(product_codes)
Generating Barcodes for Event Tickets
Event tickets often include barcodes for entry validation. Here’s an example of generating barcodes for event tickets:
import barcode
from barcode.writer import ImageWriter
def generate_ticket_barcodes(ticket_ids):
Code128 = barcode.get_barcode_class('code128')
for ticket_id in ticket_ids:
ticket_barcode = Code128(ticket_id, writer=ImageWriter())
ticket_barcode.save(f'ticket_{ticket_id}')
# List of ticket IDs
ticket_ids = ['TICKET001', 'TICKET002', 'TICKET003']
# Generate barcodes for each ticket ID
generate_ticket_barcodes(ticket_ids)
Embedding Barcodes in Reports
Barcodes can be embedded in reports generated using libraries like ReportLab. Here’s an example of embedding a barcode in a PDF report:
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
import barcode
from barcode.writer import ImageWriter
def create_report_with_barcode(report_file, barcode_data):
# Create a canvas
c = canvas.Canvas(report_file, pagesize=letter)
# Draw text
c.drawString(100, 750, "Product Report")
# Generate barcode
EAN = barcode.get_barcode_class('ean13')
ean = EAN(barcode_data, writer=ImageWriter())
barcode_filename = 'report_barcode'
ean.save(barcode_filename)
# Draw barcode on canvas
c.drawImage(f'{barcode_filename}.png', 100, 600, width=200, height=100)
# Save the PDF
c.save()
# Create a report with a barcode
create_report_with_barcode('product_report.pdf', '123456789102')
Conclusion
In this tutorial, we explored how to make a barcode generator in Python using the python-barcode
library. We covered the installation of the library, generating simple barcodes, customizing barcodes, saving barcodes to various file formats, and advanced customizations. We also discussed practical use cases, including inventory management, event ticket generation, and embedding barcodes in reports.
So next time you need some barcodes, instead of creating them with an online barcode generator, you will be able to create your own with just a few lines of python. Experiment with different barcode formats and customizations to fit your specific needs, and integrate barcode generation into your projects for improved efficiency and functionality.
Subscribe to my newsletter
Read articles from Hichem MG directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Hichem MG
Hichem MG
Python dev, coding enthusiast, problem solver. Passionate about clean code and tech innovation.