Backtracking: The Hidden Gem of Problem-Solving
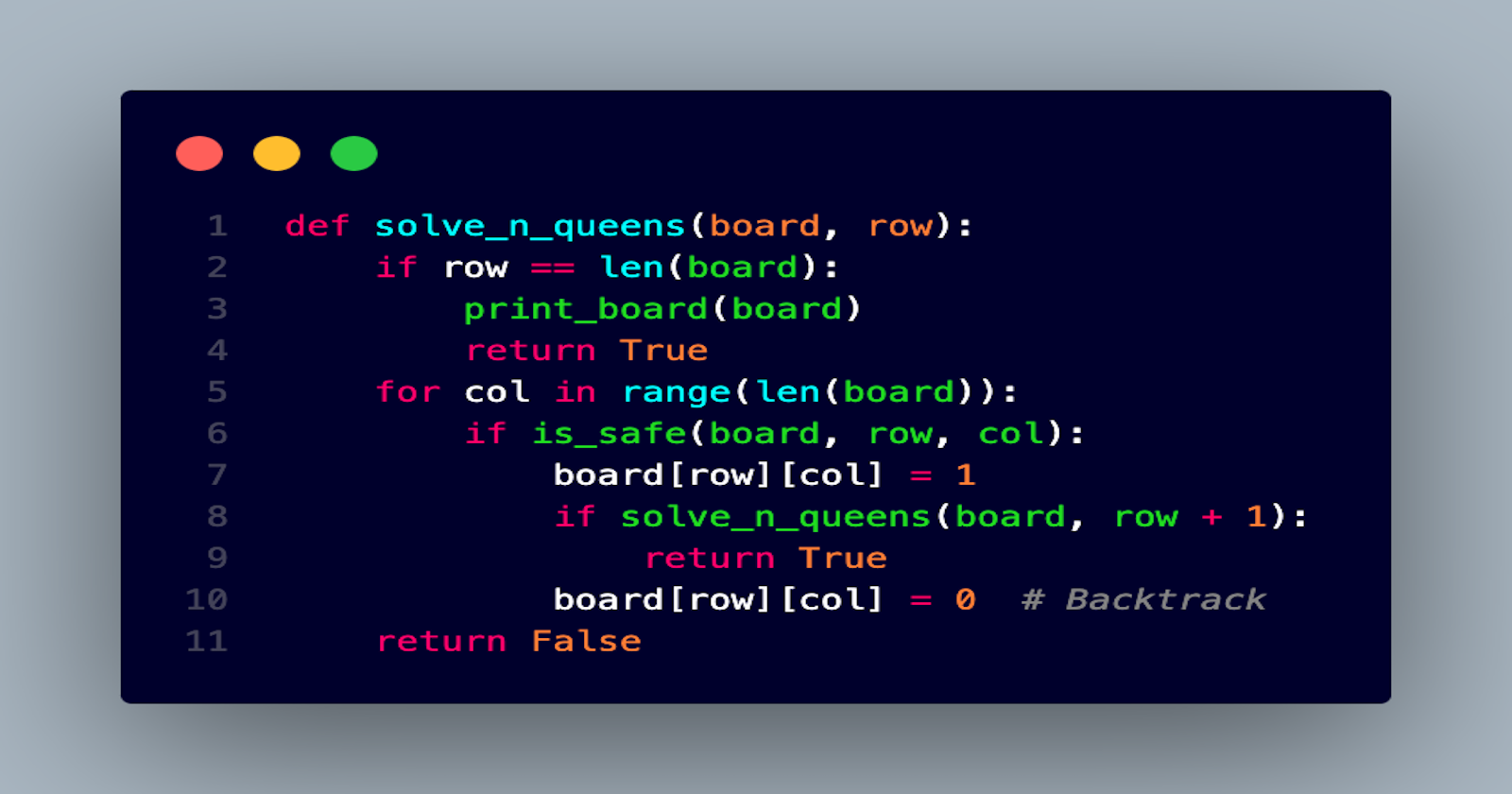
Backtracking is a powerful and often underrated technique in computer science. It's like a problem-solving Swiss Army knife that can help you find solutions to complex problems efficiently. In this blog, we'll explore what backtracking is, how it works, and why it's so valuable. We'll also use real-life examples and show how it integrates seamlessly with recursion.
What is Backtracking?
Backtracking is a method for solving problems by trying to build a solution step by step. It involves exploring all possible options to solve a problem and "backtracking" when a particular option fails. This means undoing the last step and trying another path. Think of it as a smart trial-and-error method.
Real-Life Example: Solving a Maze
Imagine you're in a maze, and you need to find the exit. Here's how backtracking works in this context:
Start at the Entrance: You begin at the entrance of the maze.
Move Forward: You take a step forward and choose a path.
Check for Success: If you reach a dead end, you backtrack to the previous step.
Try Another Path: You try a different path and repeat the process until you find the exit.
By systematically exploring and backtracking, you can navigate through the maze efficiently.
Why is Backtracking Underrated?
Backtracking is often overlooked because it seems simple or is perceived as just a brute-force method. However, it's incredibly powerful for several reasons:
Versatility: It can be applied to a wide range of problems, from puzzles to optimization problems.
Efficiency: When combined with clever pruning techniques, it can significantly reduce the number of options to explore.
Simplicity: The basic idea is easy to understand and implement.
Use in Recursion
Backtracking naturally fits with recursion. In fact, many backtracking algorithms are implemented using recursive functions. Here's how it works:
Recursive Function: Define a function that tries to solve a part of the problem.
Base Case: Identify when the problem is solved and return the solution.
Recursive Step: For each possible option, call the function recursively.
Backtrack: If an option fails, undo the last step and try another option.
Example: Solving the N-Queens Problem
The N-Queens problem is a classic example of backtracking. The goal is to place N queens on an N×N chessboard so that no two queens attack each other.
Here's a simplified version of the algorithm:
Place Queen: Start in the first row and try to place a queen in each column.
Check Safety: If a queen can be placed safely (no attacks), move to the next row and repeat.
Backtrack: If no safe column is found, backtrack to the previous row and move the queen to the next column.
def solve_n_queens(board, row):
if row == len(board):
print_board(board)
return True
for col in range(len(board)):
if is_safe(board, row, col):
board[row][col] = 1
if solve_n_queens(board, row + 1):
return True
board[row][col] = 0 # Backtrack
return False
Real-Life Application: Scheduling
Backtracking is used in scheduling problems where you need to assign tasks without conflicts. For example, consider scheduling classes in a school where no two classes should overlap for the same teacher or classroom.
Conclusion
Backtracking is a highly valuable technique that deserves more attention. It's versatile, efficient, and straightforward, making it a go-to method for solving various complex problems. By understanding and using backtracking, you can tackle challenges more effectively and find solutions that might otherwise seem elusive.
If you found this blog helpful, please like, follow, comment, and subscribe to my newsletter for more insights into powerful problem-solving techniques and other computer science topics!
Subscribe to my newsletter
Read articles from Animesh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Animesh Kumar
Animesh Kumar
I’m a software developer who loves problem-solving, data structures, algorithms, and competitive coding. I’ve a keen interest in product development. I’m passionate about AI, ML, and Python. I love exploring new ideas and enjoy innovating with advanced tech. I am eager to learn and contribute effectively to teams.