Non-Printing Characters: A Guide for Programmers
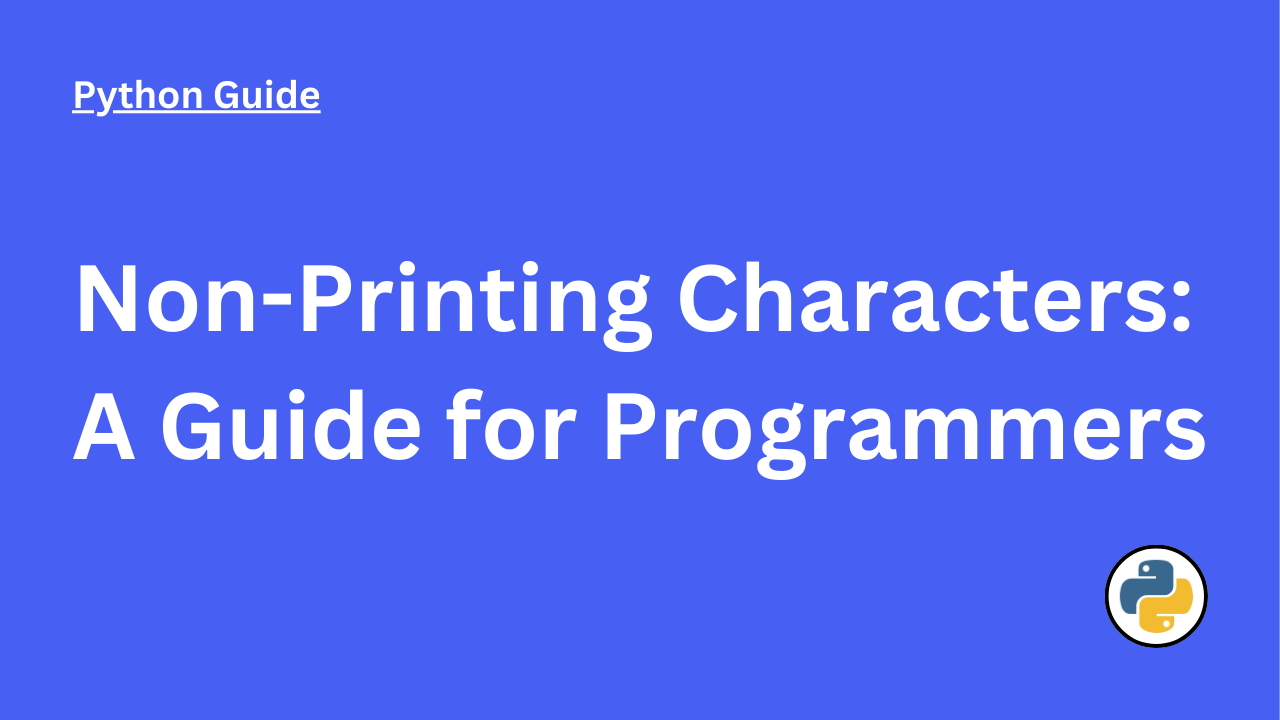
Non-printing characters, often overlooked in the world of programming, play a crucial role in various computing tasks. Unlike regular characters, which display visual symbols, non-printing characters are invisible and serve special purposes within text and data processing.
Understanding these characters and their functions can significantly enhance a programmer's ability to manipulate and control text data effectively.
Types of Non-Printing Characters
Control Characters
Control characters are a subset of non-printing characters used to manage the flow of text and the behavior of devices. Examples include:
Null Character (
\0
): Often used to signify the end of a string in languages like C.Newline (
\n
): Moves the cursor to the next line.Carriage Return (
\r
): Returns the cursor to the beginning of the line.Tab (
\t
): Moves the cursor to the next tab stop.
Formatting Characters
Formatting characters help in the layout and presentation of text. They include:
Space ( ): The regular space character.
Non-Breaking Space (
\u00A0
): Prevents automatic line breaks at its position.Zero Width Space (
\u200B
): An invisible character that doesn't occupy any space but can be used to control text flow.
Special Unicode Characters
Unicode includes a range of special characters that do not produce visible marks but serve specific purposes:
Zero Width Joiner (
\u200D
): Used in complex scripts to join characters without adding space.Zero Width Non-Joiner (
\u200C
): Prevents characters from joining in scripts where this behavior is default.
Programming Use Cases
Parsing Text Data
Non-printing characters are invaluable in parsing tasks. For example, newline characters (\n
) help in reading text line-by-line, which is essential in log analysis, file reading, and data processing scripts.
with open('data.txt', 'r') as file:
for line in file:
process(line)
Data Manipulation
In data manipulation, non-printing characters can be used to structure and format data for better readability and processing. For instance, tabs (\t
) and spaces are often used in generating formatted text files, like CSVs and TSVs.
data = [
['Name', 'Age', 'City'],
['Alice', 30, 'New York'],
['Bob', 25, 'Los Angeles']
]
with open('output.tsv', 'w') as file:
for row in data:
file.write('\t'.join(map(str, row)) + '\n')
Handling White Space
Whitespace characters such as spaces and tabs can affect string comparison and processing. Proper handling of these characters ensures that text processing functions work correctly and efficiently.
text = "Hello\tWorld"
print(text.split()) # Output: ['Hello', 'World']
Handling and Escaping Non-Printing Characters
When dealing with non-printing characters, it's essential to handle them correctly to avoid issues in text processing and data storage.
Escaping Characters
Escaping non-printing characters allows them to be included in strings without causing unexpected behavior. For example, in many programming languages, the backslash (\
) is used as an escape character.
escaped_string = "This is a newline character: \\n"
print(escaped_string) # Output: This is a newline character: \n
Using Libraries and Tools
Several libraries and tools can help manage non-printing characters. For instance, Python's re
module allows for regular expression-based handling of such characters.
import re
text = "Hello\nWorld"
cleaned_text = re.sub(r'\n', ' ', text)
print(text)
# Output:
# Hello
# World
print(cleaned_text)
# Output:
# Hello World
For those looking for a quick way to copy an empty character to use in their projects, sites like empty-character.com offer a convenient solution.
Conclusion
Non-printing characters, though invisible, have a substantial impact on programming and text processing. From controlling text flow to formatting data and parsing complex strings, these characters are indispensable in software development. By understanding and effectively handling non-printing characters, programmers can improve their coding practices and create more robust applications.
Subscribe to my newsletter
Read articles from Hichem MG directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Hichem MG
Hichem MG
Python dev, coding enthusiast, problem solver. Passionate about clean code and tech innovation.