Automating User and Group Management with Shell Scripts
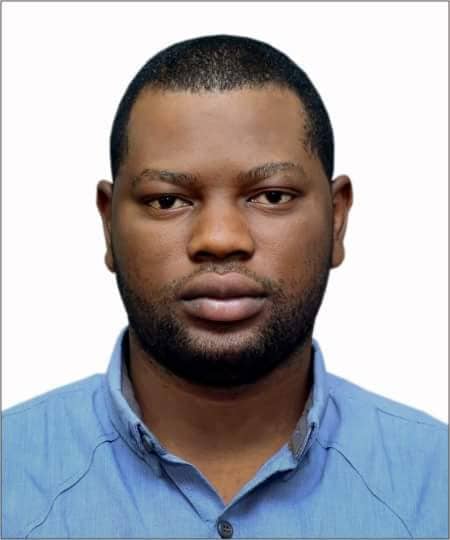
Managing users and groups in a Linux environment can be a tedious task, especially in larger systems. Automation of these tasks not only saves time but also minimizes errors. In this article, we will explore two shell scripts that automate the creation and deletion of users and groups based on a predefined list. This article is structured to provide a clear understanding of the steps involved and the reasoning behind each step.
Table of Contents
Introduction
User and group management is a critical aspect of system administration. It involves creating, modifying, and deleting user accounts and groups, as well as managing permissions and access controls. Automating these tasks can significantly improve efficiency and reduce the likelihood of human errors.
In this article, we will discuss two scripts:
create_
users.sh
- A script to create users and groups.delete_
users.sh
- A script to delete users and groups.
Both scripts take a text file (users.txt
) as input, which contains the usernames and groups in a specific format.
Script Overview
create_
users.sh
This script reads the users.txt
file, creates users, assigns them to the specified groups, and generates a random password for each user. It logs all actions to a log file and saves the generated passwords to a secure file.
Here is the create_
users.sh
script:
#!/bin/bash
# Script to create users and groups from a text file
# Usage: ./create_users.sh users.txt
USER_FILE=$1
# Check if file is provided and exists
if [[ -z "$USER_FILE" || ! -f "$USER_FILE" ]]; then
echo "Usage: $0 <user_file>"
exit 1
fi
LOG_FILE="/var/log/user_management.log"
PASSWORD_FILE="/var/secure/user_passwords.csv"
# Ensure the log and password directories and files exist
mkdir -p /var/log
touch "$LOG_FILE"
mkdir -p /var/secure
touch "$PASSWORD_FILE"
chmod 600 "$PASSWORD_FILE"
# Function to generate a random password
generate_password() {
openssl rand -base64 8
}
# Log action
log_action() {
echo "$(date '+%Y-%m-%d %H:%M:%S') - $1" | tee -a "$LOG_FILE"
}
# Loop through each line in the user file
while IFS=';' read -r username groups; do
username=$(echo "$username" | xargs) # Trim whitespace
groups=$(echo "$groups" | xargs) # Trim whitespace
# Check if the user already exists
if id "$username" &>/dev/null; then
log_action "User $username already exists. Skipping."
continue
fi
# Create personal group for the user
if ! getent group "$username" &>/dev/null; then
groupadd "$username"
if [[ $? -eq 0 ]]; then
log_action "Group $username created."
else
log_action "Failed to create group $username. Command output: $(groupadd "$username" 2>&1)"
continue
fi
fi
# Create additional groups if they do not exist
IFS=',' read -ra group_array <<< "$groups"
for group in "${group_array[@]}"; do
group=$(echo "$group" | xargs) # Trim whitespace
if ! getent group "$group" &>/dev/null; then
groupadd "$group"
if [[ $? -eq 0 ]]; then
log_action "Group $group created."
else
log_action "Failed to create group $group. Command output: $(groupadd "$group" 2>&1)"
continue 2
fi
fi
done
# Create user and add to groups
password=$(generate_password)
useradd -m -g "$username" -G "$groups" -s /bin/bash -p "$(openssl passwd -1 "$password")" "$username"
if [[ $? -eq 0 ]]; then
log_action "User $username created and added to groups: $groups"
echo "$username,$password" >> "$PASSWORD_FILE"
chmod 600 "$PASSWORD_FILE"
chmod 700 "/home/$username"
chown "$username:$username" "/home/$username"
else
log_action "Failed to create user $username. Command output: $(useradd -m -g "$username" -G "$groups" -s /bin/bash -p "$(openssl passwd -1 "$password")" "$username" 2>&1)"
fi
done < "$USER_FILE"
log_action "User creation process completed."
delete_
users.sh
This script reads the same users.txt
file and deletes the users and their associated groups if they are no longer in use. It also logs all actions to the same log file.
Here is the delete_
users.sh
script:
#!/bin/bash
# Script to delete users and groups from a text file
# Usage: ./delete_users.sh users.txt
USER_FILE=$1
# Check if file is provided and exists
if [[ -z "$USER_FILE" || ! -f "$USER_FILE" ]]; then
echo "Usage: $0 <user_file>"
exit 1
fi
LOG_FILE="/var/log/user_management.log"
# Log action
log_action() {
echo "$(date '+%Y-%m-%d %H:%M:%S') - $1" | tee -a "$LOG_FILE"
}
# Loop through each line in the user file
while IFS=';' read -r username groups; do
username=$(echo "$username" | xargs) # Trim whitespace
groups=$(echo "$groups" | xargs) # Trim whitespace
# Delete user
if id "$username" &>/dev/null; then
userdel -r "$username"
if [[ $? -eq 0 ]]; then
log_action "User $username and their home directory deleted."
else
log_action "Failed to delete user $username. Command output: $(userdel -r "$username" 2>&1)"
fi
else
log_action "User $username does not exist. Skipping."
fi
# Delete groups if empty
IFS=',' read -ra group_array <<< "$groups"
for group in "${group_array[@]}"; do
group=$(echo "$group" | xargs) # Trim whitespace
if getent group "$group" &>/dev/null; then
if [[ -z "$(getent group "$group" | cut -d: -f4)" ]]; then
groupdel "$group"
if [[ $? -eq 0 ]]; then
log_action "Group $group deleted."
else
log_action "Failed to delete group $group. Command output: $(groupdel "$group" 2>&1)"
fi
else
log_action "Group $group not empty, skipping deletion."
fi
else
log_action "Group $group does not exist. Skipping."
fi
done
done < "$USER_FILE"
log_action "User deletion process completed."
Detailed Explanation
Creating the users.txt
File
The users.txt
file should contain the list of users and their respective groups in the following format:
username;group1,group2,group3
For example:
adebola;developers
tobiloba;backend
dhebbie;developers
ayodeji;sudo,developers
balogun;admin
niyi;sudo,developers,admin
rasheed;frontend
bayo;frontend
shola;account
tope;account
rasak;backend
adedeji;developers
musty;technical
Each line specifies a username followed by the groups they should be added to, separated by commas.
Explanation ofniyi;sudo,developers,admin
:
niyi: The username.
sudo: Adding
niyi
to thesudo
group grants administrative privileges.developers: A custom group, usually for development-related tasks.
admin: Another custom group for administrative purposes.
create_
users.sh
Script
The create_
users.sh
script performs the following tasks:
Checks if the input file is provided and exists.
Ensures the log and password directories and files exist.
Reads each line from the
users.txt
file.Creates personal and additional groups for each user if they do not already exist.
Generates a random password for each user.
Creates the user and adds them to the specified groups.
Logs all actions and saves the generated passwords.
delete_
users.sh
Script
The delete_
users.sh
script performs the following tasks:
Checks if the input file is provided and exists.
Reads each line from the
users.txt
file.Deletes each user and their home directory.
Deletes each group if it is empty.
Logs all actions.
Example Usage
Running create_
users.sh
chmod +x create_users.sh
sudo ./create_users.sh users.txt
Running delete_
users.sh
chmod +x delete_users.sh
sudo ./delete_users.sh users.txt
Conclusion
By automating the user and group management process with these scripts, you can streamline administrative tasks, improve efficiency, and ensure consistency across your systems. Feel free to modify the scripts to suit your specific requirements.
For more insights into DevOps practices and automation, check out the HNG Internship and HNG Premium programs. These programs offer excellent opportunities to learn, grow, and connect with other professionals in the industry.
You can access the scripts and related files on my GitHub repository for practice and further customization.
By following the guidelines in this article, you can efficiently manage user and group creation and deletion, ensuring a streamlined and error-free system administration process. For more information and resources, explore the HNG Internship website and take advantage of the learning opportunities offered.
Subscribe to my newsletter
Read articles from Rufai Adeniyi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
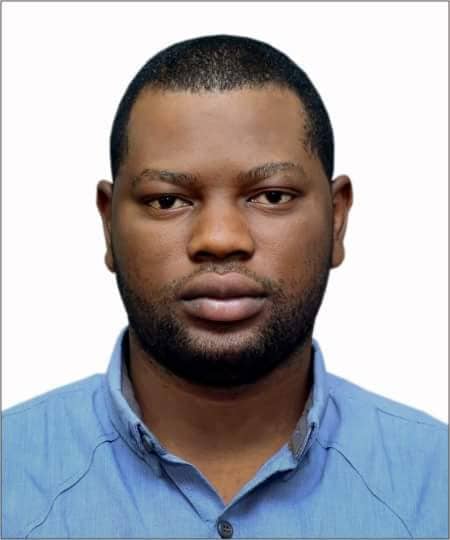
Rufai Adeniyi
Rufai Adeniyi
I am a Junior DevOps Engineer who completed DevOps training less than a year ago. Throughout my training, I have gained hands-on experience with a variety of DevOps tools and practices. I am passionate about automating processes, enhancing system reliability, and collaborating with development teams to streamline workflows. Currently, I am actively seeking internship opportunities to further develop my skills and contribute to real-world projects.