Building a Todo App with React

Table of contents
- Introduction
- Setting Up Your Development Environment
- Planning Your Todo App
- Creating the Basic Layout
- Managing State with React Hooks
- Adding Todos
- Displaying and Styling Todos
- Implementing Todo Completion
- Deleting Todos
- Editing Existing Todos
- Using Local Storage for Persistence
- Optimizing the App
- Adding Advanced Features
- Deploying Your React Todo App
- Conclusion
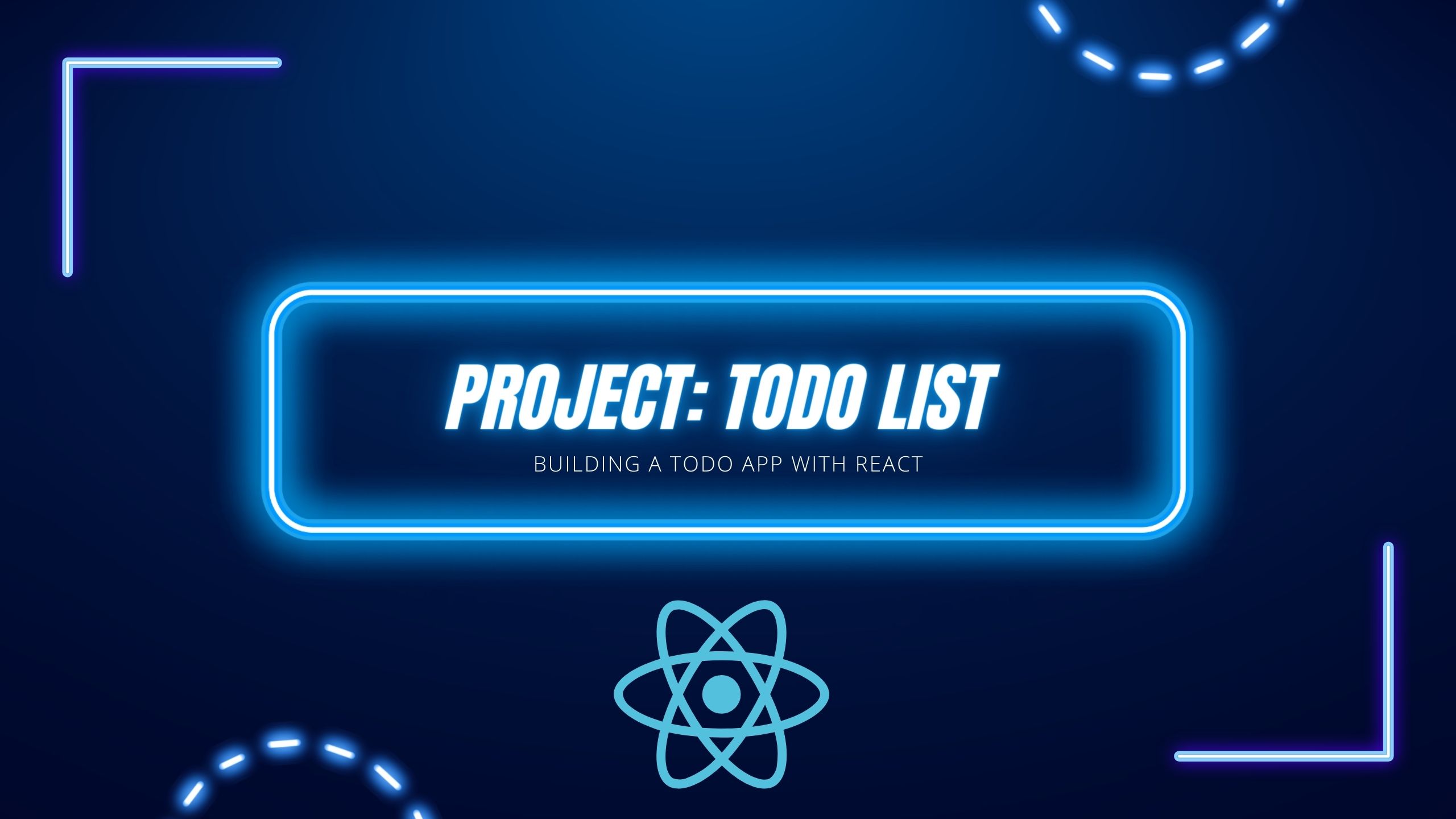
Introduction
The Importance of Todo Apps in Daily Productivity
In our fast-paced, modern world, staying organized and productive is paramount. Todo apps have become indispensable tools for managing tasks and boosting efficiency. They allow users to capture and prioritize their tasks, ensuring that nothing falls through the cracks. With a well-designed todo app, individuals can break down their goals into manageable steps, leading to a structured and productive day.
Why Choose React for Building a Todo App?
React has emerged as one of the leading frameworks for building dynamic user interfaces, especially single-page applications. Its component-based architecture allows developers to build encapsulated components that manage their own state, making the code more modular and easier to maintain. Moreover, React's virtual DOM ensures optimal performance, and its extensive ecosystem of tools and libraries accelerates the development process. For building a todo app, React offers a perfect balance of simplicity and power.
Setting Up Your Development Environment
Installing Node.js and npm
To begin building a React application, you need Node.js and npm (Node Package Manager) installed on your machine. Node.js provides the runtime environment, while npm manages the packages you need for your project. Installing Node.js is straightforward—simply download the installer from the official Node.js website and follow the instructions. npm comes bundled with Node.js, so once Node.js is installed, you are ready to manage your project's dependencies.
Setting Up a New React Project with Create React App
Create React App is a tool developed by Facebook to help developers set up a new React project quickly. It abstracts away the complex configuration and setup process. To create a new React project, open your terminal and run npx create-react-app my-todo-app
. This command sets up a new React project with all the necessary configurations, allowing you to focus on coding rather than setup.
Overview of the Project Structure
Once the project is created, you'll notice a specific structure in the project directory. The src
folder is where your application code resides. The public
folder contains the static assets. Key files like index.js
and App.js
serve as the entry points of your application. Understanding this structure is crucial as it helps you navigate through the project efficiently and organize your code effectively.
Planning Your Todo App
Defining the Core Features
Before diving into coding, it's essential to outline the core features of your todo app. Typical features include adding new todos, marking them as complete, editing existing tasks, and deleting tasks. Advanced features might include setting due dates, categorizing tasks, and persistent storage. Having a clear set of features helps in structuring your code and ensuring that all functionalities are covered.
Creating a Wireframe or Mockup
A wireframe or mockup is a visual guide that represents the skeletal framework of your app. Tools like Figma or Sketch can be used to create a simple layout. This step helps in visualizing the user interface and planning the placement of different components. A well-designed wireframe ensures a user-friendly interface and smooth user experience.
Creating the Basic Layout
Structuring the Main Components
In React, the user interface is built using components. For a todo app, you might start by creating main components such as App
, Header
, TodoList
, TodoItem
, and TodoForm
. Breaking down the UI into smaller components makes the code more readable and reusable. Each component should have a clear responsibility, adhering to the single responsibility principle.
Designing a Clean and Simple User Interface
A clean and simple UI enhances user experience. Use CSS to style your components, ensuring that the design is intuitive and accessible. Consistent margins, padding, and font sizes contribute to a polished look. Incorporate elements like buttons, input fields, and checkboxes in a way that guides the user seamlessly through the app's functionality.
Managing State with React Hooks
Introduction to useState Hook
React hooks, introduced in React 16.8, revolutionized state management in functional components. The useState
hook allows you to add state to your functional components. For instance, managing the state of the todo list or the current input value becomes straightforward with useState
. It simplifies state handling, making your components more concise and easier to understand.
Handling Input and Form State
To manage form state, use the useState
hook to track input values. This involves creating state variables for each input field and updating them as the user types. Handling form submissions involves preventing the default form action and updating the todo list state with the new task. This ensures that your app remains interactive and responsive.
Displaying the Todo List
Displaying the todo list involves mapping over the state array and rendering each todo item as a component. This dynamic rendering ensures that any changes in the state are immediately reflected in the UI. Using keys for each item helps React identify which items have changed, optimizing the rendering process.
Adding Todos
Creating a Form Component for New Todos
The form component is where users can input their new tasks. This component should include an input field and a button to submit the form. The input field captures the task details, while the button triggers the form submission. Designing a user-friendly form encourages users to add tasks efficiently.
Implementing Form Submission Logic
Upon form submission, capture the input value and update the state to include the new todo. This involves using the useState
hook to manage the form's state and the onSubmit
event to handle the form submission. Ensure that the input field is cleared after submission, providing a seamless user experience.
Updating the Todo List State
Updating the state involves appending the new task to the existing todo list. This requires creating a new state array with the updated list of todos. By managing the state efficiently, your app remains responsive and up-to-date with the user's inputs.
Displaying and Styling Todos
Mapping Over the Todo List
Mapping over the todo list involves rendering each todo item dynamically. This is achieved using the map
function, which iterates over the array and returns a component for each item. This approach ensures that the UI reflects the current state of the todo list accurately.
Using CSS for Basic Styling
CSS plays a crucial role in making your app visually appealing. Use CSS to style the todo items, adding margins, padding, and background colors to differentiate between tasks. Consistent styling creates a cohesive look and enhances the overall user experience.
Adding Conditional Styling for Completed Todos
Conditional styling allows you to visually differentiate between completed and incomplete tasks. This can be achieved using CSS classes that change the appearance of completed tasks, such as striking through the text or changing the background color. This visual cue helps users easily identify their progress.
Implementing Todo Completion
Adding Checkboxes to Mark Todos as Complete
Checkboxes provide a simple way for users to mark tasks as complete. Each todo item should include a checkbox that, when clicked, updates the task's state. This interaction should be intuitive and immediate, providing instant feedback to the user.
Updating the State for Completed Todos
Updating the state involves toggling the completed status of the task. This requires modifying the state array to reflect the change in the task's status. Efficient state management ensures that the UI remains synchronized with the underlying data.
Visual Indicators for Completed Tasks
Visual indicators, such as strikethrough text or faded colors, provide clear feedback to users about the status of their tasks. These indicators enhance the user experience by making it easy to distinguish between completed and pending tasks.
Deleting Todos
Adding a Delete Button to Each Todo
A delete button allows users to remove tasks from their list. Each todo item should include a button that, when clicked, triggers the deletion process. This functionality ensures that users can manage their tasks effectively.
Handling Delete Events
Handling delete events involves updating the state to remove the selected task. This requires creating a new state array that excludes the deleted task. Proper event handling ensures that the app remains responsive and the UI reflects the current state accurately.
Updating the State to Remove Todos
Updating the state involves filtering the todo list to remove the specified task. This operation should be efficient and ensure that the state is updated correctly. Proper state management ensures that the app functions smoothly and remains user-friendly.
Editing Existing Todos
Creating an Editable Input Field
An editable input field allows users to modify existing tasks. This involves rendering an input field when the user clicks on a task, allowing them to edit the text. This feature enhances the app's flexibility and usability.
Handling Edit Submissions
Handling edit submissions involves updating the state with the new task details. This requires capturing the edited text and updating the corresponding task in the state array. Efficient handling ensures that the app remains responsive and the changes are reflected immediately.
Updating the Todo State with Edited Values
Updating the state involves modifying the task's details in the state array. This operation should be efficient and ensure that the state is updated correctly. Proper state management ensures that the app functions smoothly and remains user-friendly.
Using Local Storage for Persistence
Introduction to Local Storage
Local Storage is a web storage API that allows you to store data locally on the user's browser. It provides a simple way to persist data across page reloads, ensuring that users do not lose their tasks when they close the app.
Saving Todos to Local Storage
Saving todos to Local Storage involves converting the state array to a JSON string and storing it using the localStorage.setItem
method. This ensures that the data is saved persistently and can be retrieved later.
Loading Todos from Local Storage on App Start
Loading todos from Local Storage involves retrieving the data using the localStorage.getItem
method and parsing the JSON string back into an array. This ensures that the app loads the saved tasks when it starts, providing a seamless user experience.
Optimizing the App
Avoiding Re-Renders with React.memo
React.memo is a higher-order component that prevents unnecessary re-renders. By wrapping components with React
.memo, you ensure that they only re-render when their props change. This optimization improves the app's performance and responsiveness.
Improving Performance with useCallback and useMemo
useCallback and useMemo are hooks that help optimize performance by memoizing functions and values. useCallback memoizes callback functions, preventing them from being recreated on every render. useMemo memoizes values, ensuring that expensive calculations are only performed when necessary. These hooks improve the efficiency of your app.
Best Practices for State Management
Effective state management involves organizing your state logically and minimizing unnecessary updates. This includes using local state for component-specific data and global state for shared data. Following best practices ensures that your app remains maintainable and performs well.
Adding Advanced Features
Implementing Due Dates and Reminders
Adding due dates and reminders involves extending the todo model to include date fields. This feature allows users to set deadlines and receive notifications, enhancing the app's utility. Proper implementation ensures that users can manage their time effectively.
Adding Categories or Tags to Todos
Categories or tags help users organize their tasks more efficiently. This involves extending the todo model to include category or tag fields and providing UI elements for assigning them. This feature enhances the app's flexibility and usability.
Creating a Search Functionality
Search functionality allows users to quickly find specific tasks. This involves implementing a search input field and filtering the todo list based on the search query. Efficient implementation ensures that users can manage their tasks effectively.
Deploying Your React Todo App
Preparing Your App for Deployment
Preparing your app for deployment involves building the production version of your React app using the npm run build
command. This creates an optimized bundle that can be served by a web server. Proper preparation ensures that your app is ready for deployment.
Deploying to GitHub Pages
GitHub Pages provides a simple way to host your static websites. To deploy your React app, push the built files to a GitHub repository and configure GitHub Pages to serve them. This provides a quick and easy way to make your app available to users.
Alternative Deployment Options (Netlify, Vercel, etc.)
Alternative deployment options include services like Netlify and Vercel, which offer more features and flexibility. These platforms provide continuous deployment, custom domains, and other advanced features. Choosing the right deployment option ensures that your app is accessible and reliable.
Conclusion
Recap of Key Points
Building a todo app with React involves setting up your development environment, planning the app's features, creating the UI, managing state, and implementing advanced functionalities. Following best practices ensures that your app is efficient and maintainable.
Encouragement to Experiment with Additional Features
Experimenting with additional features like due dates, tags, and search functionality can enhance the app's utility. Customizing the app to meet your specific needs makes it more useful and engaging.
Further Learning Resources and Next Steps
For further learning, explore React's official documentation, online tutorials, and community forums. Continuous learning and experimentation will help you master React and build more complex applications.
Subscribe to my newsletter
Read articles from Manvendra Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Manvendra Singh
Manvendra Singh
R3F | Laravel | Babylon | Three.js | WordPress Performance Enthusiast