Predicting IPL Match Outcomes with Machine Learning
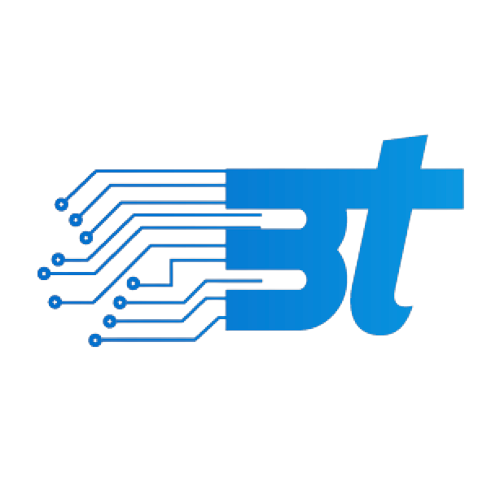
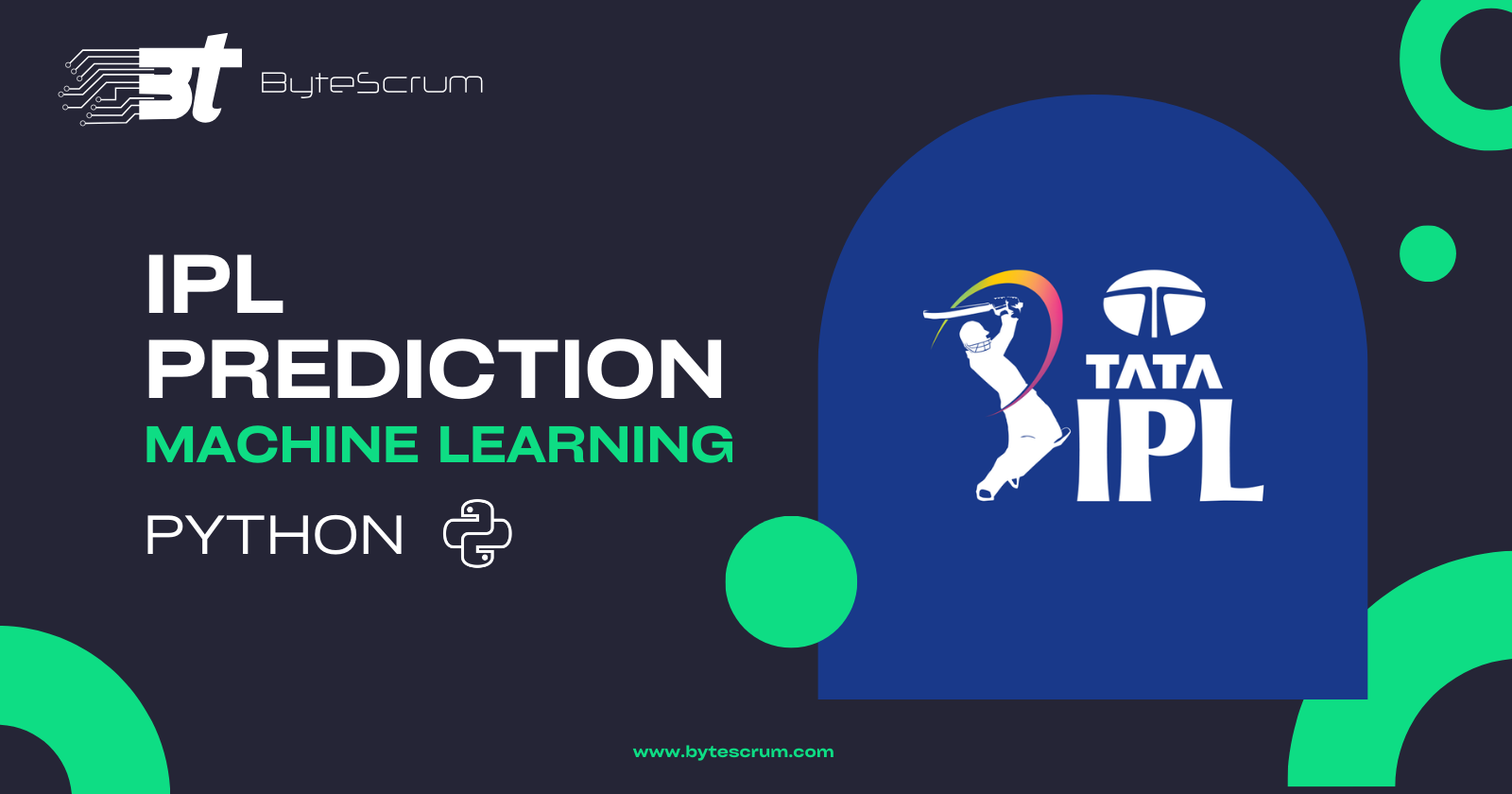
Welcome to our guide on predicting IPL (Indian Premier League) match outcomes using machine learning! In this blog, we'll explore how to utilize data science techniques and Python to forecast the results of IPL matches. Whether you're a cricket enthusiast, a data scientist, or someone keen on applying machine learning to real-world scenarios, this guide is tailored for you. Let's dive into the fascinating world of sports analytics and see how we can predict IPL match outcomes with the power of machine learning.
Why Predict IPL Match Outcomes?
Predicting the outcomes of IPL matches is not only an exciting challenge but also a valuable application of machine learning. Accurate predictions can enhance fan engagement, aid in strategic decision-making for teams, and offer insights into the dynamics of the game. By analyzing historical match data, player statistics, and various other factors, we can build models that provide meaningful forecasts for upcoming matches.
Prerequisites
Before we start, make sure you have the following Python libraries installed:
pandas
numpy
matplotlib
scikit-learn
You can install these libraries using pip:
pip install pandas numpy matplotlib scikit-learn
Step 1: Data Collection
To predict match outcomes, we'll need historical IPL match data. This dataset typically includes information such as team names, player performances, venue details, and match results. You can find IPL datasets on platforms like Kaggle or other sports data websites.
For this example, we'll assume you have a CSV file named ipl_data.csv
with the necessary match information.
import pandas as pd
# Load the dataset
ipl_data = pd.read_csv('ipl_data.csv')
print(ipl_data.head())
Here is a sample ipl_data.csv
file that you can use for predicting IPL match outcomes with machine learning. This file includes basic information about IPL matches such as team names, venue, toss winner, toss decision, and match winner.
Sample Data (ipl_data.csv)
team1 | team2 | venue | toss_winner | toss_decision | winner |
Mumbai Indians | Chennai Super Kings | Wankhede Stadium | Mumbai Indians | bat | Mumbai Indians |
Chennai Super Kings | Mumbai Indians | M. A. Chidambaram Stadium | Chennai Super Kings | field | Mumbai Indians |
Royal Challengers Bangalore | Sunrisers Hyderabad | M. Chinnaswamy Stadium | Royal Challengers Bangalore | bat | Sunrisers Hyderabad |
Sunrisers Hyderabad | Royal Challengers Bangalore | Rajiv Gandhi Intl. Cricket Stadium | Sunrisers Hyderabad | field | Sunrisers Hyderabad |
Kolkata Knight Riders | Mumbai Indians | Eden Gardens | Mumbai Indians | bat | Mumbai Indians |
Step 2: Data Preprocessing
Data preprocessing is crucial to prepare the dataset for machine learning. This involves handling missing values, encoding categorical variables, and selecting relevant features.
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import LabelEncoder
# Handle missing values
ipl_data = ipl_data.dropna()
# Encode categorical variables
label_encoder = LabelEncoder()
ipl_data['team1'] = label_encoder.fit_transform(ipl_data['team1'])
ipl_data['team2'] = label_encoder.fit_transform(ipl_data['team2'])
ipl_data['winner'] = label_encoder.fit_transform(ipl_data['winner'])
# Feature selection
features = ipl_data[['team1', 'team2', 'venue', 'toss_winner', 'toss_decision']]
target = ipl_data['winner']
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(features, target, test_size=0.2, random_state=42)
Step 3: Building the Prediction Model
We'll use a Random Forest classifier from the scikit-learn
library to predict the match outcomes.
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score, classification_report
# Initialize and train the model
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, predictions)
print(f'Accuracy: {accuracy * 100:.2f}%')
print(classification_report(y_test, predictions))
Step 4: Visualizing the Results
Visualization helps in understanding the performance of the prediction model. We’ll plot a confusion matrix to see how well the model predicts the outcomes.
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn.metrics import confusion_matrix
# Plot confusion matrix
conf_matrix = confusion_matrix(y_test, predictions)
plt.figure(figsize=(10, 7))
sns.heatmap(conf_matrix, annot=True, fmt='d', cmap='Blues', xticklabels=label_encoder.classes_, yticklabels=label_encoder.classes_)
plt.title('Confusion Matrix')
plt.xlabel('Predicted')
plt.ylabel('Actual')
plt.show()
Step 5: Enhancing the Model
While a Random Forest classifier provides a good starting point, we can explore more sophisticated models and additional features to improve prediction accuracy. Consider incorporating the following enhancements:
Adding Player Statistics
Player performance metrics such as batting and bowling averages, strike rates, and economy rates can provide deeper insights into match outcomes.
# Example: Adding a feature for team average batting score
ipl_data['avg_batting_score'] = ipl_data.apply(lambda row: calculate_avg_batting_score(row['team1'], row['team2']), axis=1)
features = ipl_data[['team1', 'team2', 'venue', 'toss_winner', 'toss_decision', 'avg_batting_score']]
Using Advanced Machine Learning Models
Consider using more advanced models such as Gradient Boosting, XGBoost, or Neural Networks for better accuracy.
from xgboost import XGBClassifier
# Initialize and train the XGBoost model
xgb_model = XGBClassifier(n_estimators=100, learning_rate=0.05, random_state=42)
xgb_model.fit(X_train, y_train)
# Make predictions
xgb_predictions = xgb_model.predict(X_test)
# Evaluate the model
xgb_accuracy = accuracy_score(y_test, xgb_predictions)
print(f'XGBoost Accuracy: {xgb_accuracy * 100:.2f}%')
print(classification_report(y_test, xgb_predictions))
Conclusion
Feel free to customize and expand upon this template to suit your specific needs and preferences. The world of sports analytics is vast, and continual learning and experimentation will yield the best results.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
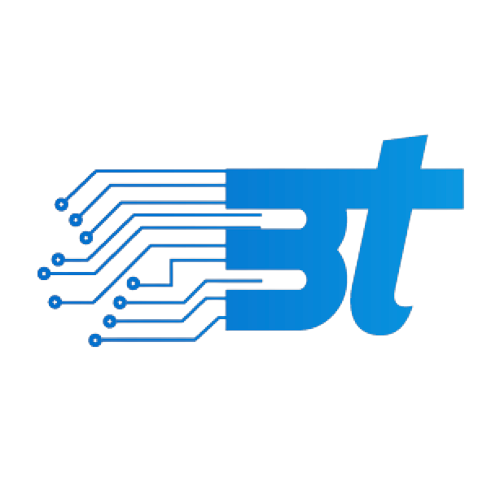
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.