The Importance of Code Reviews in Software Development
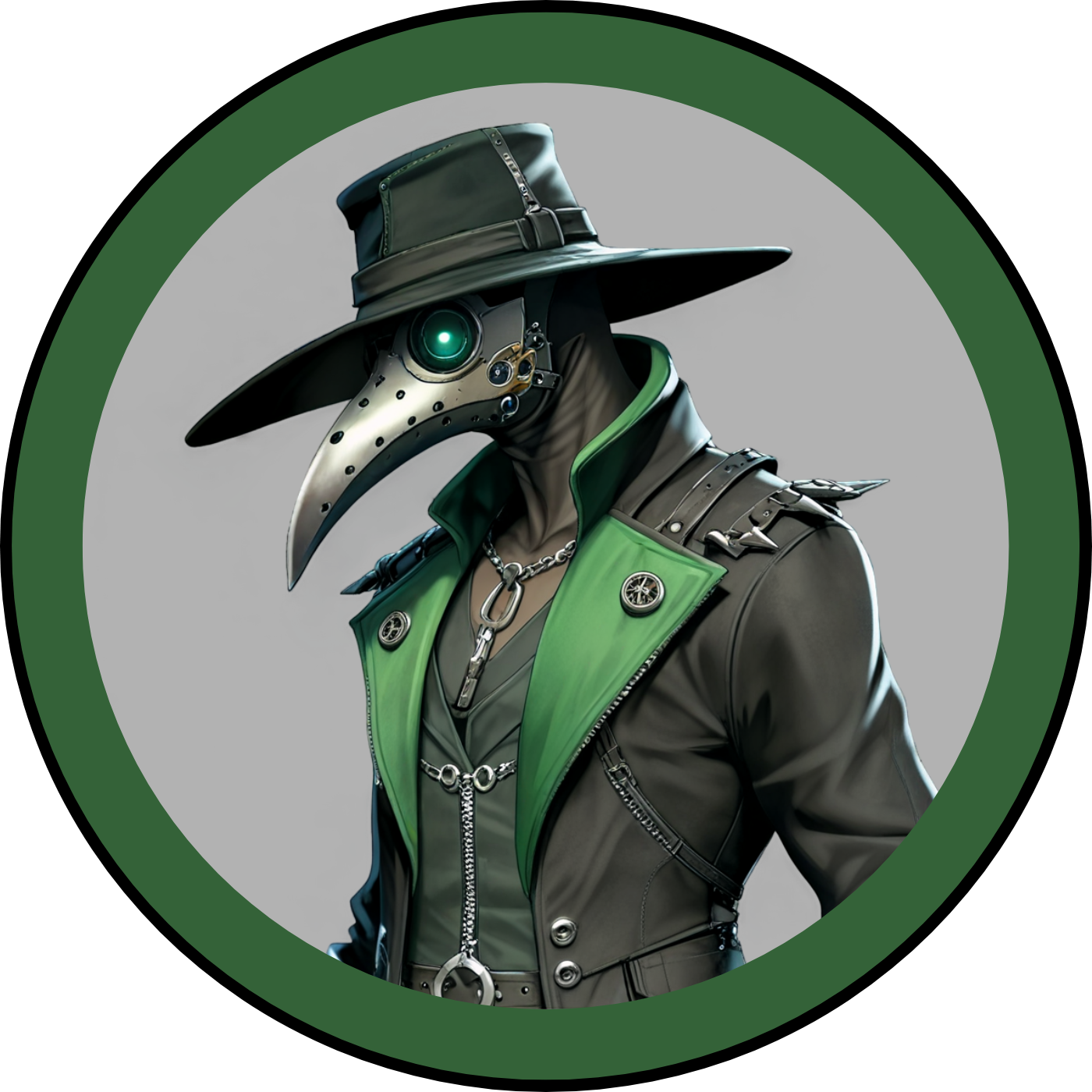

Code reviews are a pivotal component of the software development lifecycle, serving as crucial checkpoints to ensure code quality, foster team collaboration, and mitigate bugs and technical debt. This practice involves team members scrutinizing each other's code, and providing constructive feedback and suggestions for improvement before the code is integrated into the main branch. Through this collaborative process, not only is the code enhanced, but a culture of continuous improvement and accountability is cultivated within development teams.
Catching Bugs and Security Vulnerabilities Early
One of the most significant benefits of code reviews is their ability to catch bugs and security vulnerabilities early in the development process. According to a study by SmartBear Software, code reviews boast a defect removal rate of 60-90%. This early detection is critical; a study by IBM found that fixing defects during the code review process is 15 times cheaper than fixing them after release. By identifying and addressing issues early, teams can avoid costly and time-consuming fixes down the line, improve their security posture, and enhance customer satisfaction.
Example: Early Bug Detection
Imagine a scenario where a developer writes a function that processes user input without proper validation. During the code review, a colleague identifies this oversight and suggests implementing input validation. This not only prevents potential security vulnerabilities like SQL injection but also ensures data integrity. By catching this issue early, the team avoids future security breaches and maintains customer trust.
# Example of code before and after a code review suggestion
def process_user_input(input_data):
# Before: No input validation
return database.query(f"SELECT * FROM users WHERE name = '{input_data}'")
# After: Implementing input validation to prevent SQL injection
def process_user_input(input_data):
if not is_valid_input(input_data):
raise ValueError("Invalid input")
return database.query("SELECT * FROM users WHERE name = %s", (input_data,))
Promoting Knowledge Sharing and Best Practices
Code reviews are an excellent platform for knowledge sharing and promoting best practices among team members. When developers review each other's code, they gain insights into different coding styles and techniques. This exchange of knowledge helps elevate the overall skill level of the team. As John Doe, a Senior Software Engineer, aptly puts it:
"Code reviews are essential for maintaining code quality and ensuring that best practices are followed consistently."
Example: Sharing Best Practices
For instance, a junior developer might learn about more efficient algorithms or coding patterns by observing feedback on their code. This not only enhances individual skills but also strengthens the entire team’s capabilities.
pythonCopy code# Example of using a more efficient algorithm suggested in a code review
# Before: Using a nested loop for searching
def find_duplicates(data):
duplicates = []
for i in range(len(data)):
for j in range(i + 1, len(data)):
if data[i] == data[j]:
duplicates.append(data[i])
return duplicates
# After: Using a set for better performance
def find_duplicates(data):
seen = set()
duplicates = set()
for item in data:
if item in seen:
duplicates.add(item)
else:
seen.add(item)
return list(duplicates)
Improving Code Readability and Maintainability
Another key advantage of code reviews is the improvement in code readability and maintainability. Code that is easy to read and understand is easier to maintain and extend in the future. By having multiple eyes on the code, reviewers can suggest changes that make the code more logical and easier to follow. This not only benefits the current project but also makes it easier for new team members to get up to speed with the codebase.
Example: Enhancing Readability
Consider a piece of code that uses unclear variable names and lacks comments. A reviewer might suggest renaming variables and adding comments, transforming the code into something more understandable:
pythonCopy code# Before: Unclear variable names
def calc(x, y):
return x * y / 2
# After: Improved readability with clear names and comments
def calculate_area_of_triangle(base, height):
"""
Calculate the area of a triangle given its base and height.
"""
return (base * height) / 2
Jane Smith, a Software Developer, shares her experience:
"I have seen firsthand how code reviews have helped our team deliver more reliable and maintainable software products."
Facilitating Developer Growth
Code reviews are not just about finding faults; they are also an educational tool. They provide an opportunity for developers to learn from their peers and grow their skills. Whether it's learning a new coding technique or understanding a different approach to solving a problem, code reviews are a valuable learning experience for all involved.
Example: Developer Growth Through Feedback
Developers, especially those new to the team or industry, can benefit significantly from constructive feedback. A senior developer’s suggestion to refactor a complex function into smaller, more manageable pieces can be a valuable lesson in modular programming:
pythonCopy code# Before: Complex function with multiple responsibilities
def manage_user_account(user):
update_user_profile(user)
send_confirmation_email(user)
log_user_activity(user)
# After: Refactoring into smaller functions
def manage_user_account(user):
update_user_profile(user)
notify_user(user)
log_user_activity(user)
def notify_user(user):
send_confirmation_email(user)
Fostering a Culture of Continuous Improvement and Accountability
Finally, code reviews contribute to a culture of continuous improvement and accountability within the team. When everyone is involved in reviewing each other's work, there is a sense of collective responsibility for the quality of the code. This collaborative spirit drives teams to constantly strive for better, more efficient code.
Example: Building a Collaborative Culture
In teams where code reviews are standard practice, developers feel more responsible for the code they write. Knowing that peers will review their work, they tend to adhere to higher standards, resulting in cleaner, more efficient codebases.
Feedback is not just about pointing out errors but also about suggesting improvements and celebrating well-written code.
Conclusion
In conclusion, code reviews are an indispensable practice in software development. They help catch bugs and security vulnerabilities early, promote knowledge sharing and best practices, improve code readability and maintainability, facilitate developer growth, and foster a culture of continuous improvement and accountability. As statistics and quotes from industry professionals demonstrate, the benefits of code reviews are substantial, making them a cornerstone of effective software development processes.
Embracing code reviews can transform your development workflow, making it more efficient and collaborative. So, whether you are a junior developer or a seasoned engineer, engaging in regular code reviews is a step toward not just better code, but a better development team as a whole.
def engage_with_blog(reaction):
if reaction == 'happy':
leave_comment()
elif reaction == 'loved':
share_with_friends()
elif reaction == 'amazed':
react_with_emoji('😲')
else:
print('Thanks for reading!')
Subscribe to my newsletter
Read articles from Surgical directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
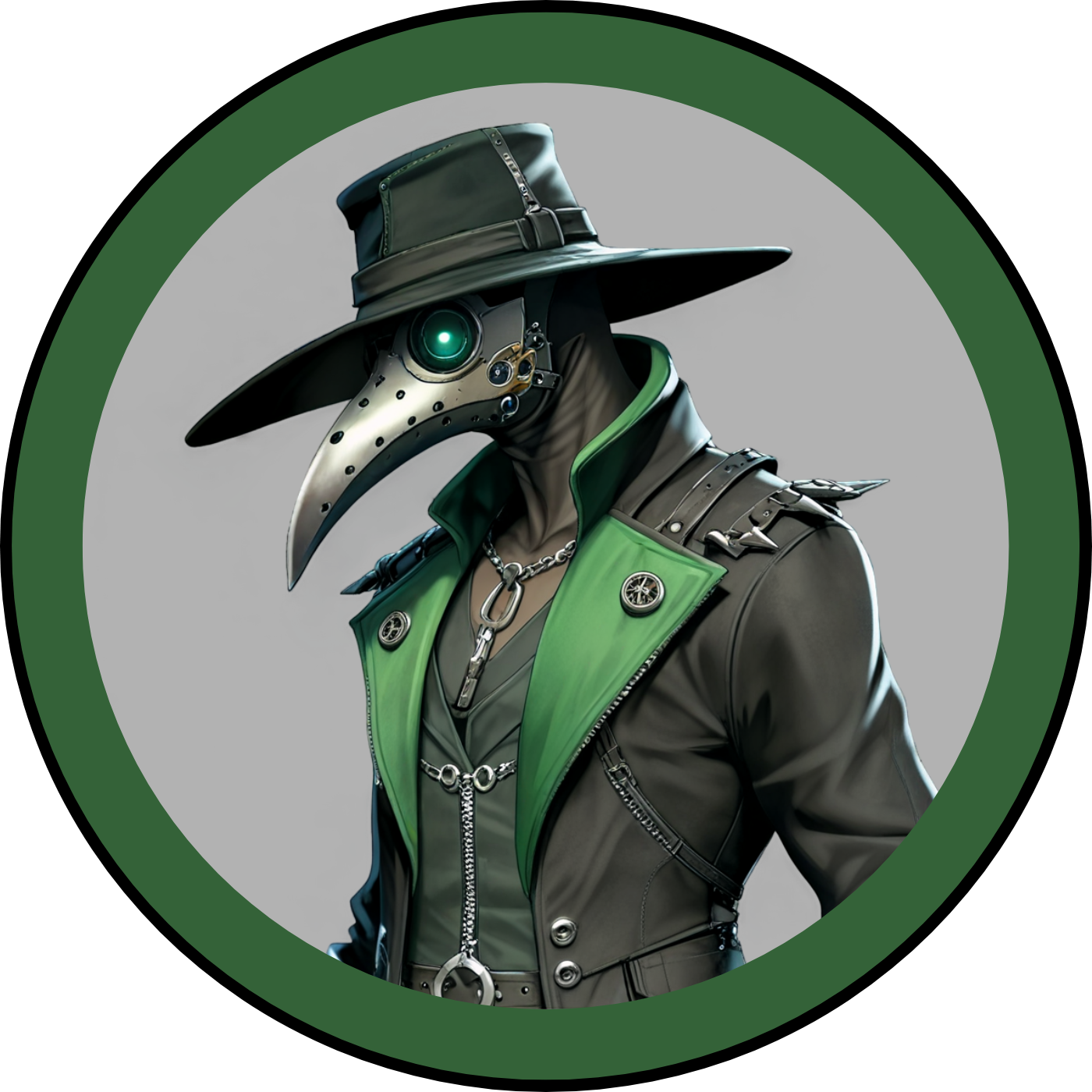
Surgical
Surgical
a Multifaceted professional specializing in IT Support, Web Design, System Management, Software Development, and Hosting Solutions. With a deep-rooted passion for technology and its transformative potential, I have dedicated myself to crafting beautiful, functional, and user-centric digital experiences.