Learn Python Fundamentals Fast
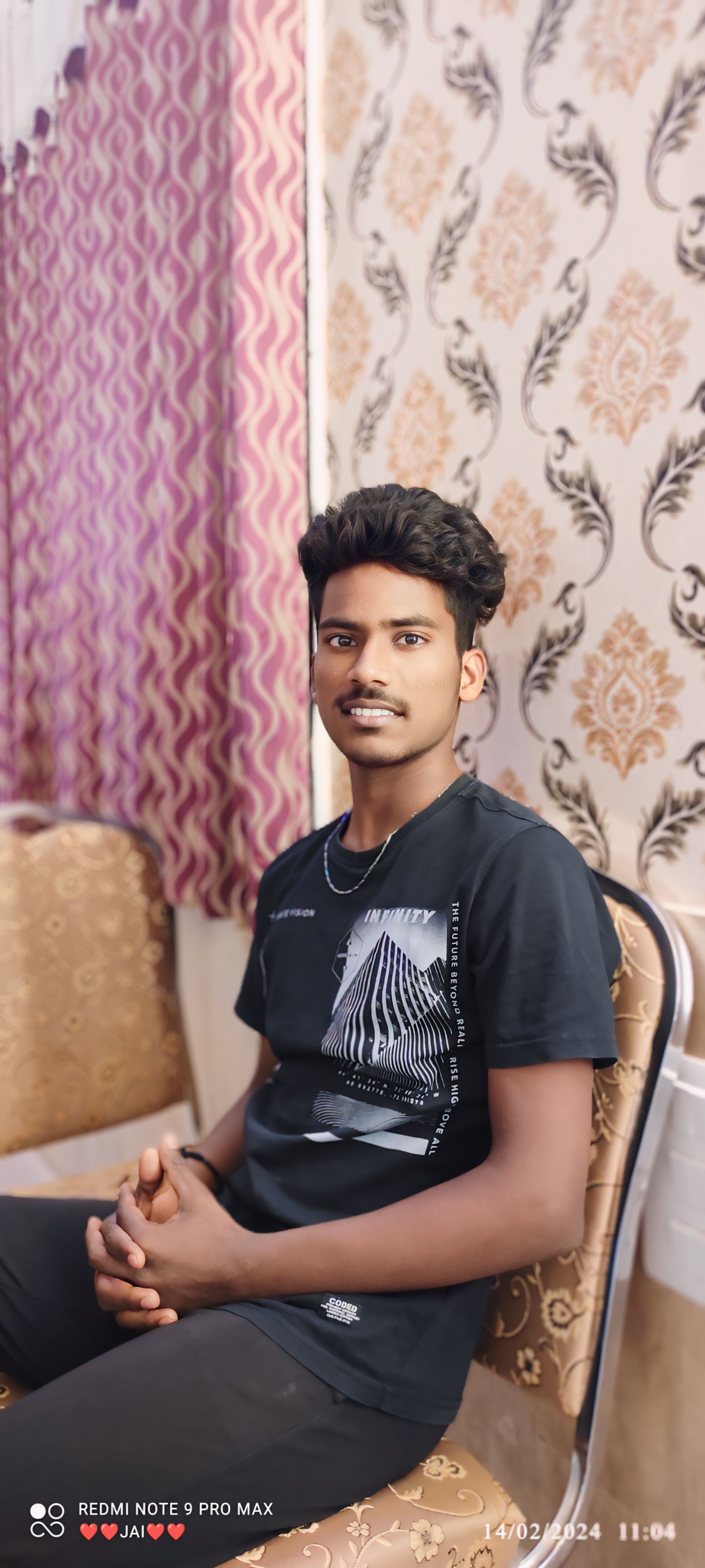
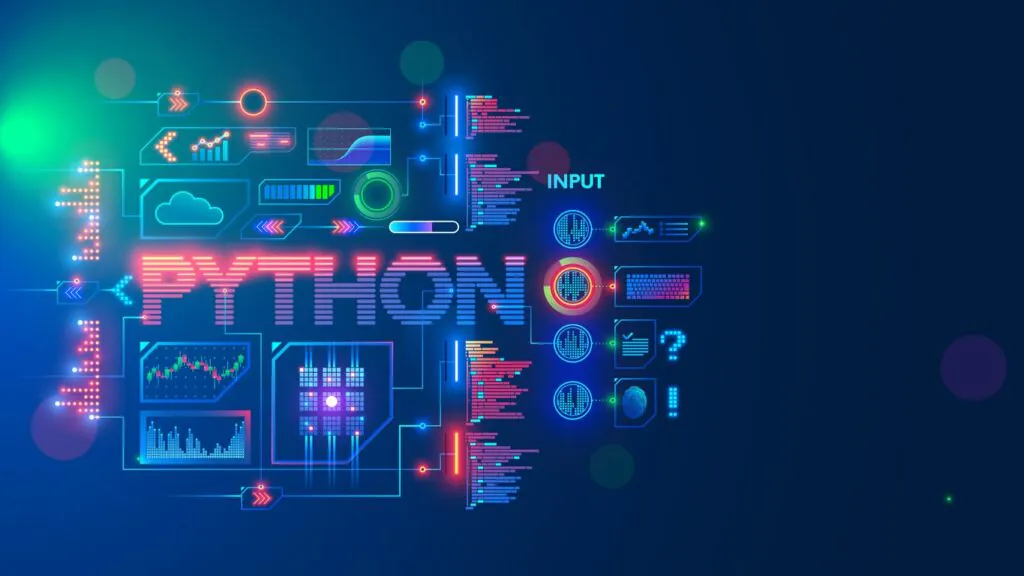
Intro Python
python is a high level language and general purpose programming language known for its simplicity and readability and "GUIDO VAN RASSUM" was created python in 1991 .python supports multiple inheritence .it is mainly used to web development ,data analysis,mechaine learning ,automation and sceintific computing in web developmet ,python is commonly used with frame works like django and flask
Hello world program in python
To show you how fun and easy python is, we have provided a classic "Hello World" program in python hello world program
To create a "Hello World" program in python, you can use the following simple code
print("Hello, World!")
print()
is a Python function that outputs a message to the console or terminal."Hello, World!"
is a string literal, enclosed in double quotes, which is the message you want to display.
When you run this Python code, it will output Hello, World!
to the console or terminal.
python comments
Comments can be used to make the code more readable.
Comments can be used to prevent execution when testing code.
comments can be used to expalain python code.
*single line comment (#)
*multi line comment (" ")
python variables
variables are like container for storing values .values in the variables can be changed
*which will store a value
*address of a value
python data types
in programming, data type is an important concept.
variables can store data of different types, and different types can do different things
python has the following types builti-in by default ,in these categories.
Text Type: | str |
Numeric Types: | int , float , complex |
Sequence Types: | list , tuple , range |
Mapping Type: | dict |
Set Types: | set , frozenset |
Boolean Type: | bool |
Binary Types: | bytes , bytearray , memoryview |
None Type: | NoneType |
python numbers
In programming languages every value or data has a associated type to it known as data data type saome commonly used data type
data types
*float
*integer
*complex numbers
Integers (
int
): These are whole numbers, such as -2, -1, 0, 1, 2, etc. In Python, integers can be of arbitrary size, meaning they can be as large as the available memory allows.Floating-point numbers (
float
): These are numbers with a decimal point or an exponent (e.g., 3.14, -0.001, 2.7e5). Floating-point numbers in Python are implemented using the IEEE 754 double-precision standard.Complex numbers (
complex
): These are numbers of the forma + bj
, wherea
andb
are floats, andj
(orJ
) represents the imaginary unit. For example,3 + 4j
is a complex number with real part 3 and imaginary part
python operators
operators are used to perform on variables and values
in the example below, we use the +operator to add together two value
python arithmetic operators
arithmetic operators are used with numeric values to perform commom mathmatical operations
Operator | Name | Example | |||
+ | Addition | x + y | |||
- | Subtraction | x - y | |||
* | Multiplication | x * y | |||
/ | Division | x / y | |||
% | Modulus | x % y | T | ||
** | Exponentiation | x ** y | |||
// | Floor division | x // y |
python lists
lists are used to store multiple items in a single variable.
lists are one of 4built-in data types in python used to store collections of data,the other 3 are tuple ,set, and dictionary all with different qualities and usage.
lists are created using square brackets .
giri = ["apple", "banana", "cherry"]
print(giri)
it alows duplicates values
lists are mutable [changing]
lists items are indexed .index starts from [0],[1] are ect.......
python collection [arrys]
there are four collection data types in the pythin programming language
*list is a collection which is orderd and changeable .allow duplicates members
*tuple is a collection which is orderd and unchangeable .allow duplicates members
\set is a collection which is a unordered ,unchangeable \ and unindexed it no duplictes
dictionary is a collection which is orderd **and changeable .allow no duplicates
python if -else
the if- else statement is used to make decisions based on certain conditions.
example : If
a=33
b = 200
if b > a:
print("b is greater than a")
In this example we use two variables, a and b, which are used as part of the if statement to test whether b is greater than a. As a is 33, and b is 200, we know that 200 is greater than 33, and so we print to screen that "b is greater than a".
example: Elif
the elif is way of saying "if the if condition were not true ,then try elif condition"
a = 33
b = 33
if b > a:
print("b is greater than a")
elif a == b:
print("a and b are equal")
n this example a is equal to b, so the first condition is not true, but the elif condition is true, so we print to screen that "a and b are equal".
example :else
the else condition catches anything which is'nt caught by the preceding condition
a = 200
b = 33
if b > a:
print("b is greater than a")
elif a == b:
print("a and b are equal")
else:
print("a is greater than b")
In this example a is greater than b, so the first condition is not true, also the elif condition is not true, so we go to the else condition and print to screen that "a is greater than b".
python loops
python has two primitive loop commonds
while loops
for loops
the while loop
with the whie loop we can execute a set of statement as a long as condition is true
Print i as long as i is less than 6:
i = 1
while i < 6:
print(i)
i += 1the for loop
A for loop is used for iterating over a sequence (that is either a list, a tuple, a dictionary, a set, or a string).
fruits = ["apple", "banana", "cherry"]
for x in fruits:
print(x)
The for loop does not require an indexing variable to set beforehand.
###
Subscribe to my newsletter
Read articles from Utukuru Gireesh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
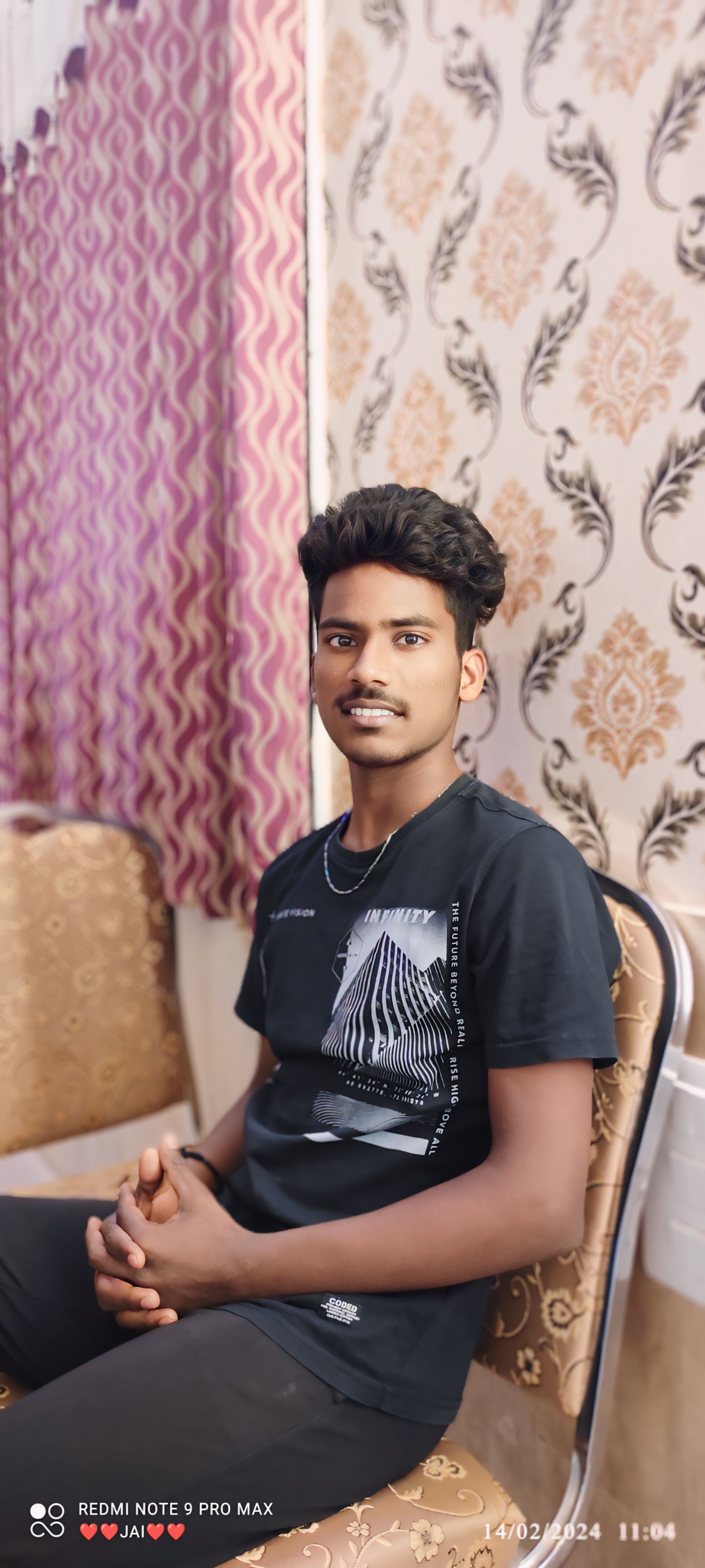
Utukuru Gireesh
Utukuru Gireesh
Iam a python full stack developer