How to Automate User Creation on a Linux Server Using Bash Scripts
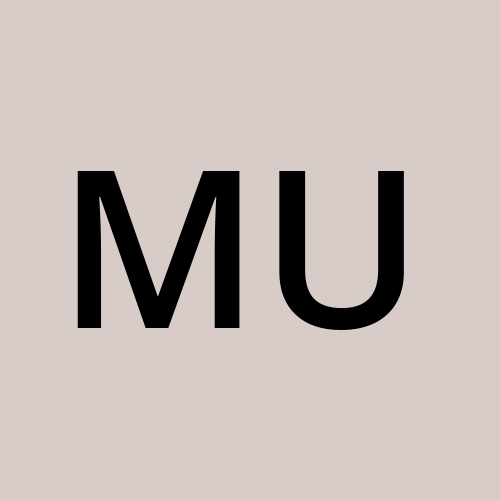
The role of a systems operation (SysOps) engineer can sometimes become intimidating and dificult to deal with. One of the common tasks you'll encounter is managing user accounts, groups, and passwords on Linux servers. This includes creating users, assigning them to groups, and ensuring they have secure passwords. This process can become tiring, especially when onboarding multiple new employees. In this article, I'll guide you through writing a Bash script that automates these tasks, making user management efficient and error-free.
Managing user accounts on Linux can be challenging, especially as your organization grows. Automating this process helps keep things consistent, secure, and efficient. In this article, we'll guide you through creating a bash script to automate the creation of users and groups, set the right permissions, and log all activities.
Task Overview
As a systems operation engineer, your company has hired several new developers, and you need to create user accounts for them. Each user should have a home directory, be assigned to specific groups, and have a secure password. You are also required to log all actions performed by the script and store the generated passwords securely. Sounds too much right? Never worry. Automation will make things easy for you.
Requirements:
It is required that;
A user can belong to multiple groups, separated by commas.
Each user must have a personal group with the same name as their username.
Usernames and groups are separated by a semicolon in the input file.
- For example:
light; sudo,dev,www-data
idimma; sudo
mayowa; dev,www-data
Bash Script to Automate User Creation
Bash Script to Automate User Creation
Are you ready to simplify your user management tasks on Linux server? Let me walk through an easy-to-follow Bash script that will automate user and group creation, set permissions, and log everything. Dive in, follow along, and see how this script can ease your task.
#!/bin/bash
# Paths for logging and password storage
LOG_FILE="/var/log/user_management.log"
PASSWORD_FILE="/var/secure/user_passwords.csv"
# Ensure log and password directories exist
sudo mkdir -p /var/log
sudo mkdir -p /var/secure
sudo touch "$LOG_FILE"
sudo touch "$PASSWORD_FILE"
sudo chmod 600 "$PASSWORD_FILE"
# Function to generate random password
generate_password() {
< /dev/urandom tr -dc 'A-Za-z0-9!@#$%&*' | head -c 16
}
# Read user list from file provided as argument
while IFS=";" read -r username groups; do
# Remove whitespace
username=$(echo "$username" | xargs)
groups=$(echo "$groups" | xargs)
# Create user group with the same name as the username
if ! getent group "$username" > /dev/null; then
sudo groupadd "$username"
echo "$(date): Group $username created" | sudo tee -a "$LOG_FILE"
else
echo "$(date): Group $username already exists" | sudo tee -a "$LOG_FILE"
fi
# Create user if it doesn't exist
if ! id -u "$username" > /dev/null 2>&1; then
sudo useradd -m -g "$username" -G "$groups" "$username"
password=$(generate_password)
echo "$username:$password" | sudo chpasswd
echo "$(date): User $username created with groups $groups" | sudo tee -a "$LOG_FILE"
echo "$username,$password" | sudo tee -a "$PASSWORD_FILE"
sudo chown root:root "$PASSWORD_FILE"
else
echo "$(date): User $username already exists" | sudo tee -a "$LOG_FILE"
fi
# Assign user to additional groups
IFS=',' read -ra ADDR <<< "$groups"
for group in "${ADDR[@]}"; do
if ! getent group "$group" > /dev/null; then
sudo groupadd "$group"
echo "$(date): Group $group created" | sudo tee -a "$LOG_FILE"
fi
sudo usermod -aG "$group" "$username"
echo "$(date): User $username added to group $group" | sudo tee -a "$LOG_FILE"
done
# Set appropriate permissions for home directory
sudo chmod 700 "/home/$username"
sudo chown "$username:$username" "/home/$username"
done < "$1"
echo "User creation process completed."
Let's further Explain the script above
Logging and Password Storage Setup
We define paths for the log file and the password file. The log file will store a record of all actions taken by the script, while the password file will securely store generated passwords.
We ensure the directories and files exist and set appropriate permissions.
Password Generation Function
- The
generate_password
function creates a random 16-character password using/dev/urandom
.
- The
Reading User List from File
- The script reads from the input file provided as an argument. Each line in the file is expected to have the format
username;groups
.
- The script reads from the input file provided as an argument. Each line in the file is expected to have the format
Creating User Groups
- For each user, we create a group with the same name as the username if it doesn't already exist.
Creating Users
If the user does not already exist, we create the user with their personal group and any additional groups specified.
We generate a random password for the user and set it.
The username and password are logged.
Assigning Users to Groups
- We ensure the user is assigned to all specified groups. If any group does not exist, it is created.
Setting Permissions
We set the home directory permissions to ensure only the user can access their home directory.
Let us test your script to ensure it is performing as expected.
To test the script:
Create an Input File
- Create a text file named
user_list.txt
with the following content:
- Create a text file named
light; sudo,dev,www-data
idimma; sudo
mayowa; dev,www-data
Run the Script
- Execute the script with the input file as an argument:
bash create_users.sh user_list.txt
Verify Users and Groups using the 'get enteries' command
- Use the
getent
command to check if users and groups were created correctly:
getent passwd light
getent group dev
You can also visit the HNG Internship and Premium program to learn more.
Let automation take care of your repetitive task.
Subscribe to my newsletter
Read articles from Michael Udofia directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
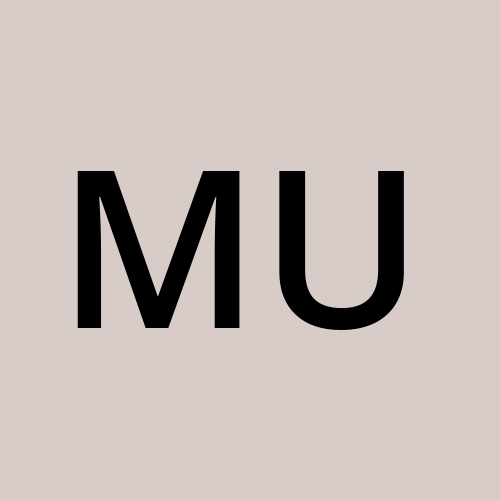