CSS selectors
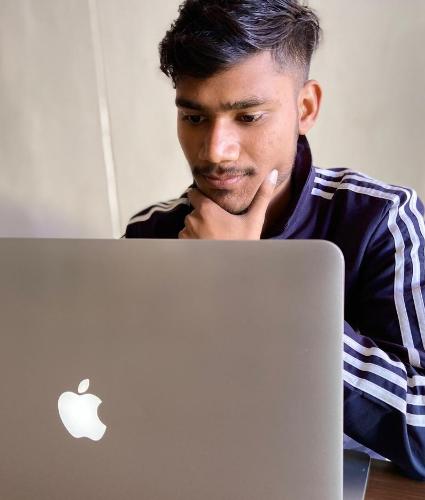
Layman Terms
Let's take an example of painting a house. If you want to paint the house you select different colors and styles for different rooms, cielings and furniture.For e.g. all walls of drawing room could be same but in bedroom the colour of walls might change.
In the similar way you choose different styles for different elements.But you need different selectors to select some common elements to optimise code readability.
For e.g. you want to change colour of all the p tags except one selector can help you out over here. Let's see it in detail.
Technically
CSS selectors are patterns used to select the elements you want to style. When you write CSS, you apply styles to HTML elements using these selectors. Think of them as instructions that tell the browser, "Hey, apply these styles to these specific parts of the webpage."
Types of CSS Selectors
Below HTML code demonstrates various CSS selectors and their effects on the elements. Each selector type is defined and explained above to provide a clear understanding of their usage.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
* {
background-color: #4d4d4d;
}
p {
background-color: #d1ea76;
}
.warning {
background-color: #ef9323;
color: #FFFFFF;
}
#danger {
background-color: #e93916;
color: #FFFFFF;
}
li.bg-black.text-white {
background-color: #000;
color: #ef9323;
}
span, li {
background-color: burlywood;
}
div ul li {
background-color: #4b35f1;
}
div > li > p {
background-color: #7667e4;
}
.sibling + p {
background-color: pink;
}
</style>
</head>
<body>
<div>Welcome to live class</div>
<span>Span is just a span, nothing more</span>
<p>We all know paragraph</p>
<ul>
<li class="bg-black text-white">item1</li>
<li>item2</li>
<li>item3</li>
<li>item4</li>
<li>item5</li>
</ul>
<div>
<p>lorem</p>
<li>awesome</li>
<ul>
<li>highlight me <a href="#">test</a></li>
<li>highlight me</li>
</ul>
</div>
<section>
<p class="sibling">Test 1</p>
<p>Test 2</p>
<p>Test 3</p>
<p>Test 4</p>
<p>Test 5</p>
</section>
</body>
</html>
Let us debunk all of them one by one :-
Universal Selector
Definition: The universal selector *
applies styles to all elements on the page.
* {
background-color: #4d4d4d;
}
Effect: This selector sets the background color of every element on the page to #4d4d4d
.
Example:
<p>We all know paragraph</p>
<ul>
<li>item1</li>
</ul>
Result: Both the <p>
and <ul>
elements, along with all other elements on the page, will have a background color of #4d4d4d
.
Individual Selector
Definition: The individual selector applies styles to all instances of the specified element type.
Code:
p {
background-color: #d1ea76;
}
Effect: This selector sets the background color of all <p>
(paragraph) elements to #d1ea76
.
Example:
<p>We all know paragraph</p>
Result: The <p>
element will have a background color of #d1ea76
.
Class and ID Selector
Class Selector Definition: Applies styles to all elements with the specified class. ID Selector Definition: Applies styles to the element with the specified ID.
Code:
.warning {
background-color: #ef9323;
color: #FFFFFF;
}
#danger {
background-color: #e93916;
color: #FFFFFF;
}
Effect:
Any element with the class
warning
will have a background color of#ef9323
and text color#FFFFFF
.Any element with the ID
danger
will have a background color of#e93916
and text color#FFFFFF
.
Example:
<p class="warning">Warning text</p>
<p id="danger">Danger text</p>
Result:
The
<p>
element with the classwarning
will have a background color of#ef9323
and text color#FFFFFF
.The
<p>
element with the IDdanger
will have a background color of#e93916
and text color#FFFFFF
.
Chained Selector (And Selector)
Definition: Applies styles to elements that match multiple classes.
Code:
li.bg-black.text-white {
background-color: #000;
color: #ef9323;
}
Effect: This selector sets the background color to #000
and text color to #ef9323
for <li>
elements that have both the bg-black
and text-white
classes.
Example:
<li class="bg-black text-white">item1</li>
Result: The <li>
element with both bg-black
and text-white
classes will have a background color of #000
and text color #ef9323
.
Combined Selector
Definition: Applies styles to multiple element types.
Code:
span, li {
background-color: burlywood;
}
Effect: This selector sets the background color of all <span>
and <li>
elements to burlywood
.
Example:
<span>Span text</span>
<li>List item</li>
Result: Both the <span>
and <li>
elements will have a background color of burlywood
.
Descendant Selector (Inside an Element)
Definition: Applies styles to elements that are nested within specific parent elements.
Code:
div ul li {
background-color: #4b35f1;
}
Effect: This selector sets the background color of <li>
elements that are inside a <ul>
, which is inside a <div>
, to #4b35f1
.
Example:
<div>
<ul>
<li>highlight me</li>
</ul>
</div>
Result: The <li>
element within the <ul>
and <div>
will have a background color of #4b35f1
.
Direct Child Selector
Definition: Applies styles to elements that are direct children of the specified parent. Code:
div > li > p {
background-color: #7667e4;
}
Effect: This selector would apply styles to <p>
elements that are direct children of <li>
elements, which are direct children of <div>
elements.
Example:
<div>
<li>
<p>Direct child paragraph</p>
</li>
</div>
Result: The <p>
element will have a background color of #7667e4
.
Sibling Selector
Definition: Applies styles to elements that are adjacent siblings of the specified element.
Code:
.sibling + p {
background-color: pink;
}
Effect: This selector sets the background color of the <p>
element that directly follows an element with the class sibling
to pink
.
Example:
<p class="sibling">Test 1</p>
<p>Test 2</p>
Result: The first <p>
element following the element with the class sibling
gets a pink background.
Difference between direct child and descendant selector
Direct Child Selector
Definition: The direct child selector (>
) applies styles to elements that are direct children of the specified parent.
Syntax: parent > child
Explanation: This selector targets only the immediate children of the parent element, not any deeper nested elements.
Example:
<div>
<p>Direct child paragraph</p> <!-- This will be styled -->
<span>
<p>Nested paragraph</p> <!-- This will NOT be styled -->
</span>
</div>
div > p {
color: blue;
}
Result: Only the <p>
element that is a direct child of the <div>
will have blue text. The nested <p>
within the <span>
will not be affected.
Descendant Selector
Definition: The descendant selector (a space between selectors) applies styles to all elements that are nested within the specified parent element, at any depth.
Syntax: ancestor descendant
Explanation: This selector targets all elements that are descendants of the specified parent element, regardless of how deeply they are nested.
Example:
<div>
<p>Direct child paragraph</p> <!-- This will be styled -->
<span>
<p>Nested paragraph</p> <!-- This will also be styled -->
</span>
</div>
div p {
color: red;
}
Result: Both the direct child <p>
and the nested <p>
within the <span>
will have red text.
Subscribe to my newsletter
Read articles from Rupesh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
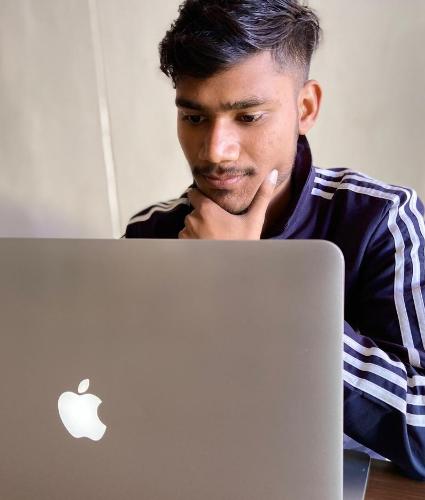