Building Scalable Applications with Spring Boot and MongoDB
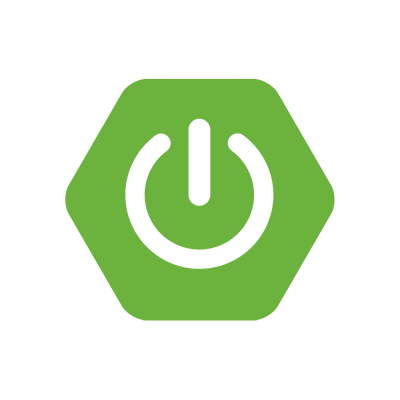
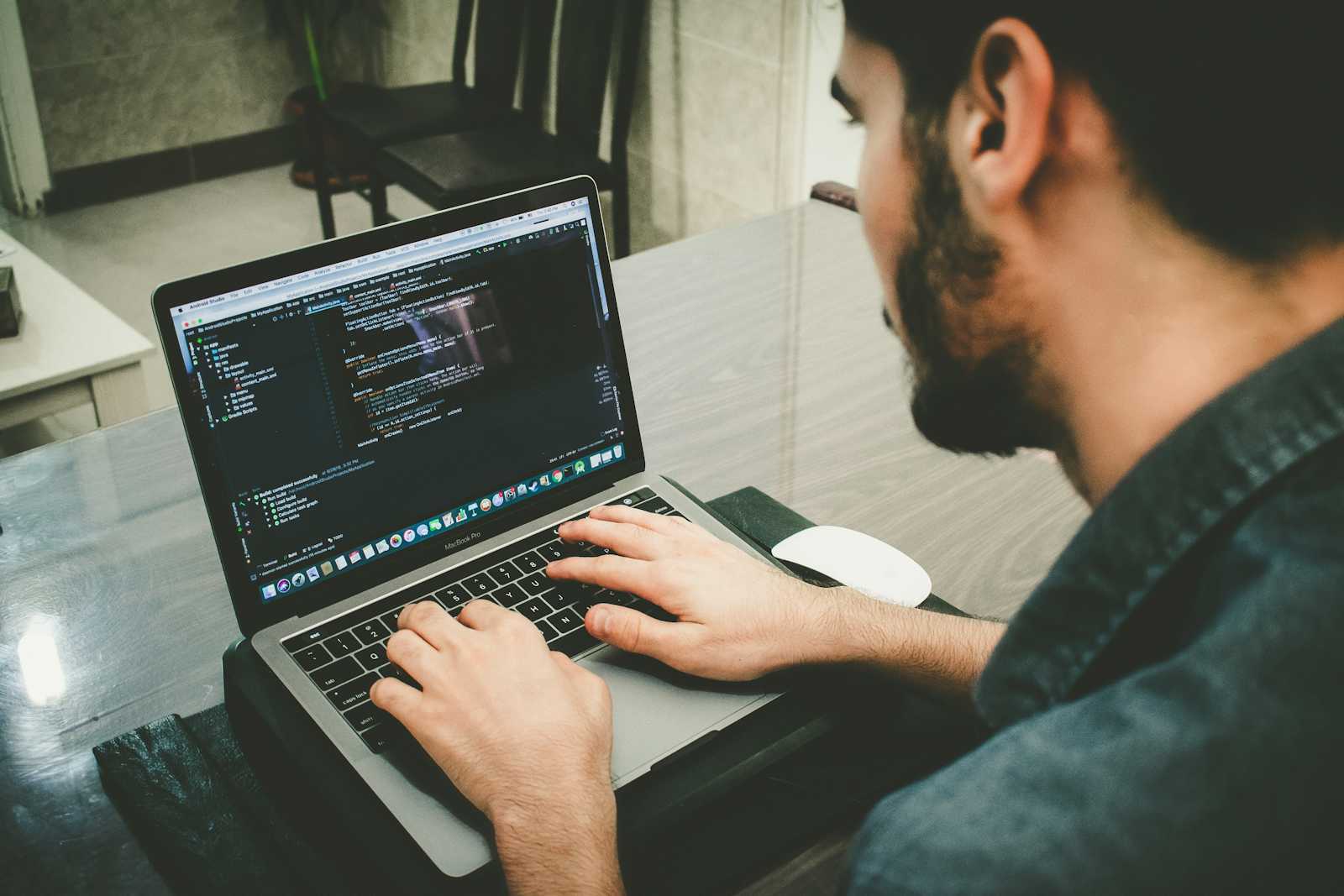
Introduction
Spring Boot is a powerful, versatile framework for building Java applications with ease. Its convention-over-configuration approach simplifies the development process, making it a favorite among developers. MongoDB, on the other hand, is a NoSQL database that offers flexibility and scalability, making it ideal for handling large volumes of unstructured data. Combining Spring Boot with MongoDB allows developers to create robust, scalable applications efficiently.
This article will guide you through the process of integrating Spring Boot with MongoDB, covering essential steps and best practices.
Prerequisites
Before we begin, ensure you have the following installed on your system:
Java Development Kit (JDK) 8 or later
Maven or Gradle
MongoDB Server
Step 1: Setting Up the Spring Boot Application
Start by creating a new Spring Boot project. You can use Spring Initializr (https://start.spring.io/) to generate the project structure or create it manually.
If using Spring Initializr, select the following dependencies:
Spring Web
Spring Data MongoDB
If creating manually, set up your pom.xml
(for Maven) or build.gradle
(for Gradle) file to include the necessary dependencies.
Maven pom.xml
:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
Gradle build.gradle
:
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-data-mongodb'
implementation 'org.springframework.boot:spring-boot-starter-web'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
Step 2: Configuring MongoDB Connection
Spring Boot simplifies database connection setup with its auto-configuration capabilities. To configure MongoDB, add the connection details to your application.properties
or application.yml
file.
application.properties
:
spring.data.mongodb.uri=mongodb://localhost:27017/yourdbname
application.yml
:
spring:
data:
mongodb:
uri: mongodb://localhost:27017/yourdbname
Step 3: Creating the Data Model
Define the data model class that maps to the MongoDB collection. Annotate the class with @Document
to specify the MongoDB collection.
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.mapping.Document;
@Document(collection = "users")
public class User {
@Id
private String id;
private String name;
private String email;
// Getters and setters
}
Step 4: Creating the Repository
Spring Data MongoDB provides a repository abstraction that simplifies data access. Create a repository interface for your data model.
import org.springframework.data.mongodb.repository.MongoRepository;
public interface UserRepository extends MongoRepository<User, String> {
}
Step 5: Creating the Service Layer
The service layer contains the business logic of your application. Create a service class to manage User
entities.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
import java.util.Optional;
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public List<User> getAllUsers() {
return userRepository.findAll();
}
public Optional<User> getUserById(String id) {
return userRepository.findById(id);
}
public User saveUser(User user) {
return userRepository.save(user);
}
public void deleteUser(String id) {
userRepository.deleteById(id);
}
}
Step 6: Creating the Controller
The controller handles HTTP requests and delegates them to the service layer. Create a controller class to handle user-related requests.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import java.util.List;
import java.util.Optional;
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserService userService;
@GetMapping
public List<User> getAllUsers() {
return userService.getAllUsers();
}
@GetMapping("/{id}")
public ResponseEntity<User> getUserById(@PathVariable String id) {
Optional<User> user = userService.getUserById(id);
return user.map(ResponseEntity::ok).orElseGet(() -> ResponseEntity.notFound().build());
}
@PostMapping
public User createUser(@RequestBody User user) {
return userService.saveUser(user);
}
@DeleteMapping("/{id}")
public ResponseEntity<Void> deleteUser(@PathVariable String id) {
userService.deleteUser(id);
return ResponseEntity.noContent().build();
}
}
Step 7: Running the Application
With everything set up, you can now run your Spring Boot application. Use the following command if you’re using Maven:
mvn spring-boot:run
Or, if you’re using Gradle:
gradle bootRun
Navigate to http://localhost:8080/users
in your browser or use a tool like Postman to interact with your API.
Conclusion
Integrating Spring Boot with MongoDB is straightforward, thanks to Spring Data MongoDB’s repository abstraction and Spring Boot’s auto-configuration capabilities. This setup allows you to focus on your application’s business logic without worrying about boilerplate code for database interactions. With this guide, you should be well on your way to building robust and scalable applications using Spring Boot and MongoDB.
Further Reading
By following these steps and utilizing the resources provided, you can efficiently integrate Spring Boot with MongoDB in your projects. Happy coding!
Subscribe to my newsletter
Read articles from Nikhil Soman Sahu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
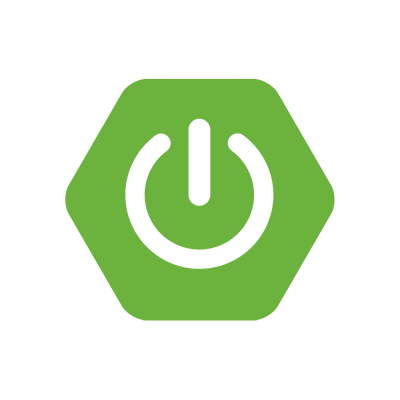
Nikhil Soman Sahu
Nikhil Soman Sahu
Software Developer