Efficient User Management with Bash: A Script for User Creation, Group Assignment, and Secure Passwords
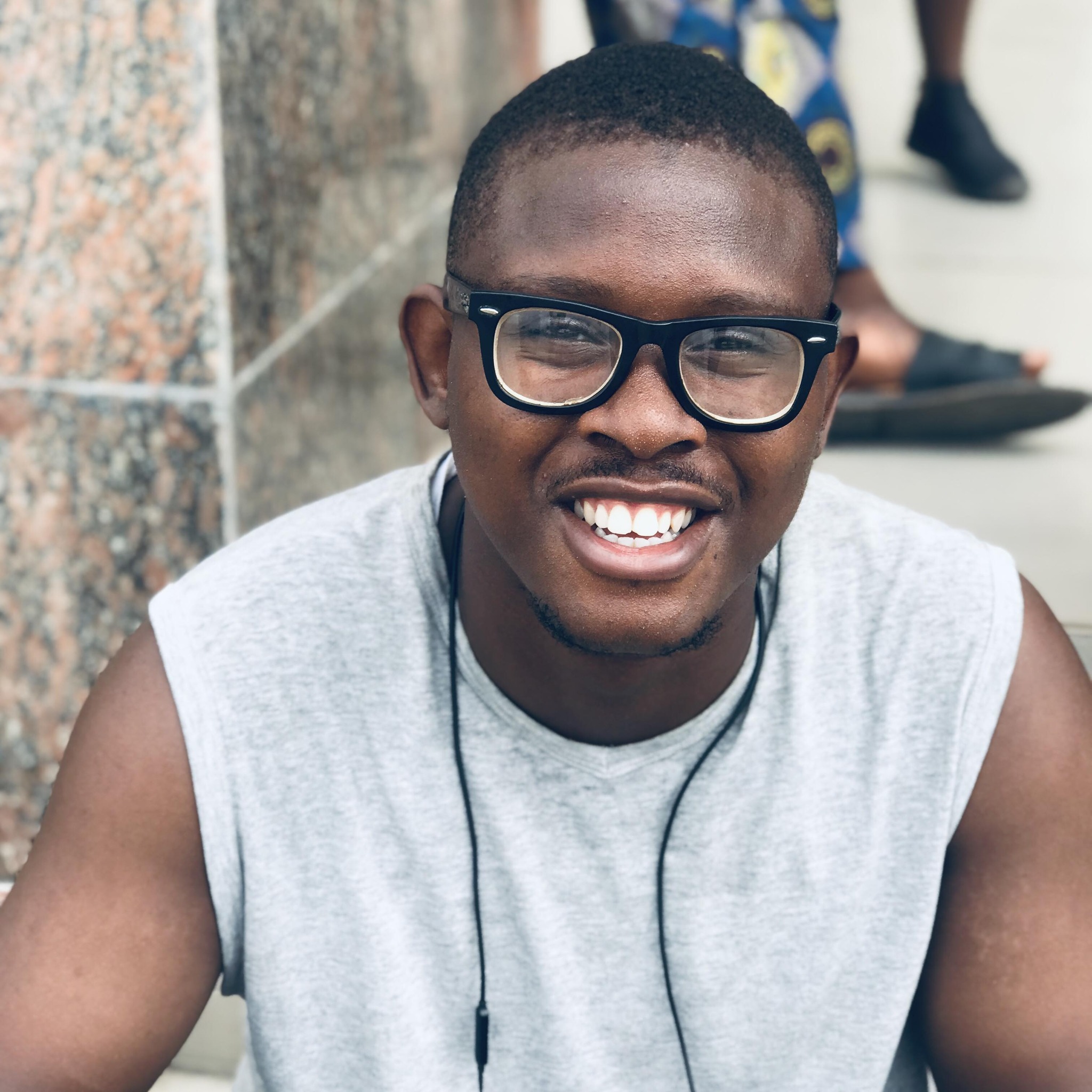
As organizations expand and grow, managing user permissions and passwords may become more complex, and human error is more likely to occur. The best option is to automate the process. Why? Well, automation ensures consistency, saves time, and reduces human error.
Throughout this article, I'll walk you through a bash script that reads a text file containing usernames and groups, creates users, sets up home directories, generates random passwords, and logs all actions. The script is designed for a SysOps engineer responsible for onboarding new developers and is part of my task for the week in the HNG11 internship. If you'd like to check out the various benefits of their premium tier, you can do so here.
Prerequisites
You must have a basic knowledge of bash scripting as well as access to a Linux system with administrative privileges to practice the steps in this article.
The Overview
We have a basic knowledge of bash scripting and the Linux system with the necessary privileges; what exactly does the script that we will call create_users.sh
do? Let's get an overview before we break it down block by block.
Reads a text file containing usernames and group names.
Creates users and their primary groups.
Adds users to specified groups.
Sets up home directories with appropriate permissions and ownership.
Generates random passwords for the users.
Logs all actions to
/var/log/user_management.log
.Stores the generated passwords securely in
/var/secure/user_passwords.txt
.
The Breakdown
Setting Up The Logs
We start by creating the log and password files while making sure they have the correct permissions to protect sensitive information.
LOG_FILE="/var/log/user_management.log" PASSWORD_FILE="/var/secure/user_passwords.txt" mkdir -p /var/log mkdir -p /var/secure touch $LOG_FILE touch $PASSWORD_FILE chmod 600 $PASSWORD_FILE
The log_action Function
This function logs actions to the log file and also prints them to the console.
log_action() { echo "$(date): $1" | tee -a $LOG_FILE }
Input Validation
We check if the script received exactly one argument, which would be the input file, and if the file exists.
if [ $# -ne 1 ]; then
echo "Usage: $0 <name-of-text-file>"
exit 1
fi
INPUT_FILE=$1
if [ ! -f "$INPUT_FILE" ]; then
echo "File $INPUT_FILE does not exist."
exit 1
fi
The Main Processing Loop
We read each line of the input file, extract the usernames and groups, and process them.
while IFS=';' read -r username groups; do
username=$(echo "$username" | xargs)
groups=$(echo "$groups" | xargs)
if id "$username" &>/dev/null; then
log_action "User $username already exists."
else
useradd -m -s /bin/bash "$username"
log_action "User $username created."
if [ $? -eq 0 ]; then
user_group="$username"
groupadd "$user_group"
usermod -aG "$user_group" "$username"
log_action "Group $user_group created and user $username added to group $user_group."
IFS=',' read -ra ADDR <<< "$groups"
for group in "${ADDR[@]}"; do
group=$(echo "$group" | xargs)
if ! getent group "$group" > /dev/null; then
groupadd "$group"
log_action "Group $group created."
fi
usermod -aG "$group" "$username"
log_action "User $username added to group $group."
done
PASSWORD=$(openssl rand -base64 12)
echo "$username:$PASSWORD" | chpasswd
echo "$username,$PASSWORD" >> $PASSWORD_FILE
log_action "Password set for user $username."
chmod 700 /home/$username
chown $username:$user_group /home/$username
log_action "Home directory for user $username set with appropriate permissions and ownership."
else
log_action "Failed to create user $username."
fi
fi
done < "$INPUT_FILE"
log_action "User creation process completed."
And there you have it. A script that ensures that each user has a personal group, handles multiple groups, sets up secure home directories, generates random passwords, and logs all activities.
I hope this script helps budding as well as full-time SysOps engineers improve security, efficiency, and accuracy through the automation of user management.
To find out how I tested the script on my own and generated a sample file for its use, you should check out the README.md file in the repository where the script resides.
Until the next article, May The Force Be With You.
Subscribe to my newsletter
Read articles from Eze Francis directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
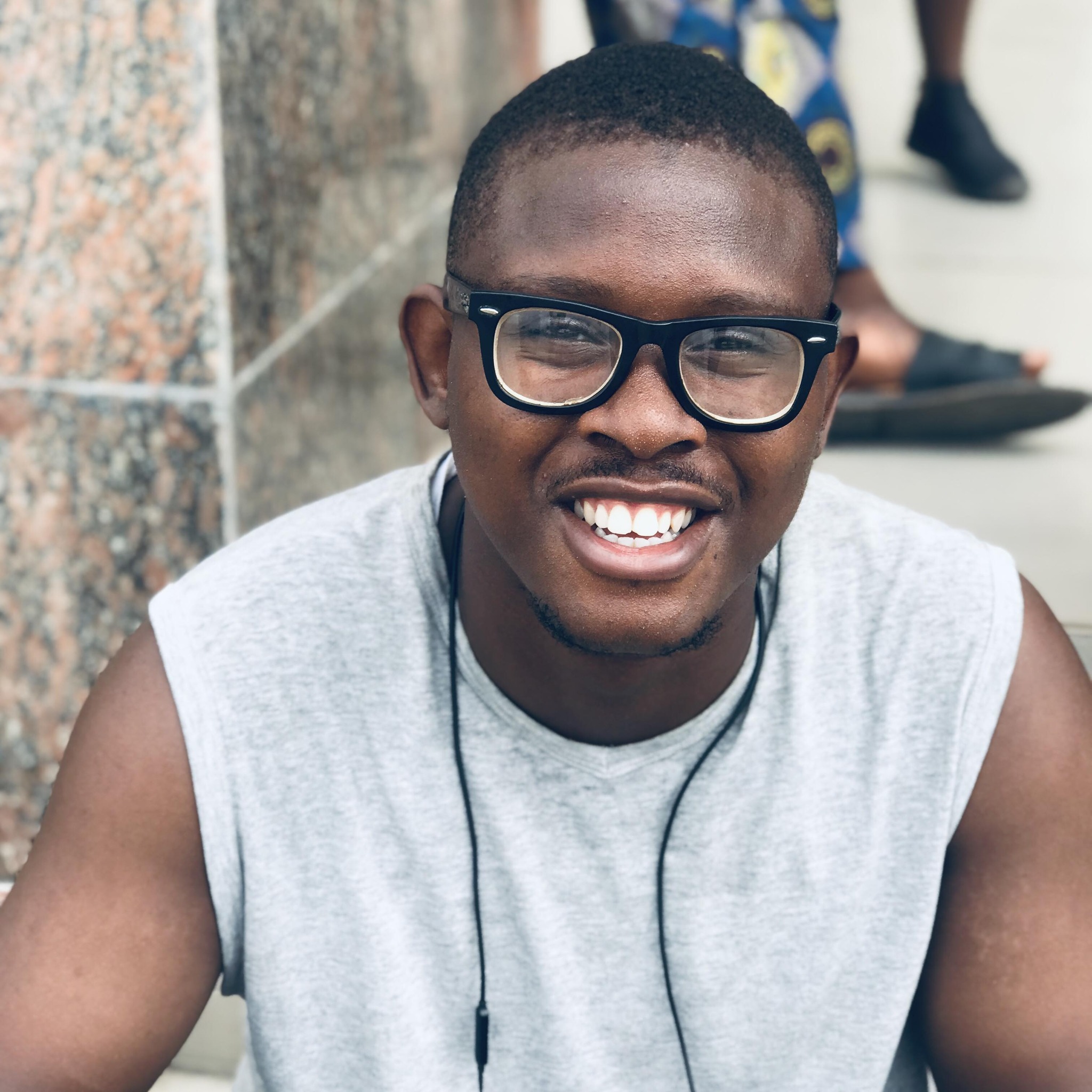