Understanding Polling, WebRTC, and Webhooks: Key Concepts and Examples in JavaScript
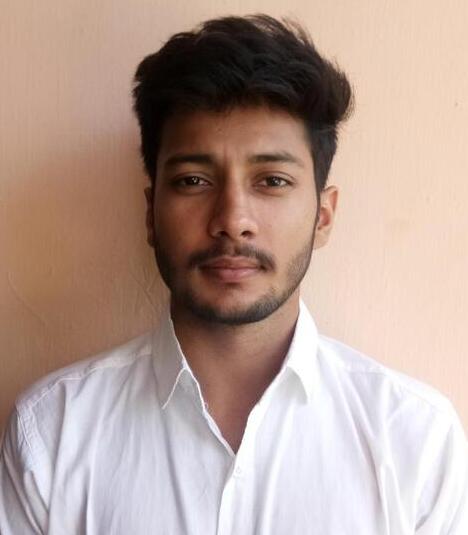
In the ever-evolving world of web development, keeping up with real-time communication and event-driven architectures is crucial. Polling, WebRTC, and Webhooks are three powerful technologies that play significant roles in this domain. In this blog, we’ll delve into these concepts, explain how they work, and provide JavaScript examples to help you learn and implement them effectively.
Polling
What is Polling?
Polling is a technique where a client repeatedly requests data from a server at regular intervals. It’s commonly used to check for updates or new data without maintaining a constant connection.
Use Cases
Live data dashboards: Continuously fetch the latest data to display in real-time.
Chat applications: Check for new messages periodically.
Monitoring systems: Regularly check the status of servers or services.
Advantages and Disadvantages
Advantages:
Simple to implement.
No need for a persistent connection.
Disadvantages:
Can be inefficient and resource-intensive.
Latency between updates.
Code Example
Here’s a simple JavaScript example demonstrating polling:
setInterval(async () => {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
}, 5000);
WebRTC
What is WebRTC?
Web Real-Time Communication (WebRTC) is a technology that enables peer-to-peer communication directly between browsers, allowing for audio, video, and data sharing without the need for intermediary servers.
Use Cases
Video conferencing: Facilitate face-to-face meetings over the web.
Live streaming: Stream video content in real-time.
Peer-to-peer file sharing: Transfer files directly between users.
How It Works
WebRTC uses a combination of STUN/TURN servers and the ICE framework to establish and maintain connections. It handles the complexities of NAT traversal and peer discovery.
Code Example
A basic example of setting up a WebRTC connection in JavaScript:
const peerConnection = new RTCPeerConnection();
navigator.mediaDevices.getUserMedia({ video: true, audio: true })
.then(stream => {
document.getElementById('localVideo').srcObject = stream;
stream.getTracks().forEach(track => peerConnection.addTrack(track, stream));
});
peerConnection.ontrack = event => {
document.getElementById('remoteVideo').srcObject = event.streams[0];
};
Webhooks
What are Webhooks?
Webhooks are user-defined HTTP callbacks triggered by specific events. They allow one system to send real-time data to another whenever an event occurs, without requiring continuous polling.
Use Cases
Notifications: Receive alerts for specific events (e.g., new commits on GitHub).
Integrations: Connect different services and automate workflows.
Data synchronization: Keep data consistent across multiple systems.
Setting Up a Webhook
Here’s a step-by-step guide on setting up a webhook using Node.js and Express:
- Create an Express application:
const express = require('express');
const app = express();
app.use(express.json());
app.post('/webhook', (req, res) => {
const data = req.body;
console.log(data); // Log the webhook payload
res.status(200).send({ status: 'success' });
});
app.listen(5000, () => {
console.log('Server is running on port 5000');
});
Set up a webhook in GitHub:
Go to your repository settings.
Select “Webhooks” and click “Add webhook.”
Enter the URL of your Express application (e.g.,
http://yourdomain.com/webhook
) and choose the events you want to trigger the webhook.
Advantages and Disadvantages
Advantages:
Efficient and real-time.
Reduces server load compared to polling.
Disadvantages:
Requires endpoint security.
Dependent on external service availability.
Conclusion
Polling, WebRTC, and Webhooks are essential tools in the modern web developer’s toolkit. Each has its own strengths and use cases, making them valuable for different scenarios. By understanding these technologies and exploring the provided JavaScript examples, you can enhance your web development skills and create more dynamic, real-time applications.
Additional Resources
Polling:
WebRTC:
Webhooks:
By experimenting with these technologies and the associated examples, you’ll gain practical experience and a deeper understanding of how to implement real-time communication and event-driven architectures in your own applications.
Happy coding!
Subscribe to my newsletter
Read articles from Achutendra Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
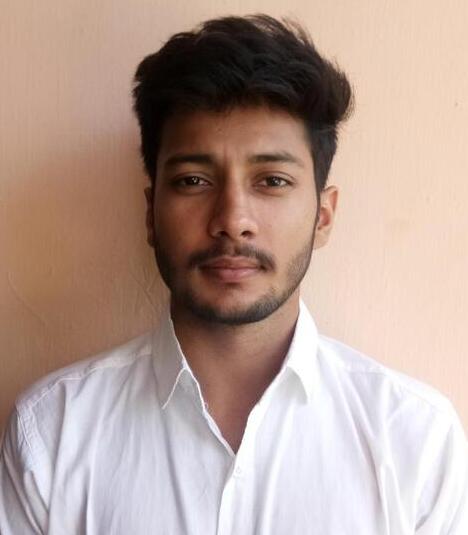
Achutendra Singh
Achutendra Singh
Full Stack Developer with a passion for continuous learning. Connect with me to explore the fascinating world of technology and beyond!