TypeScript | Java Iterable Implementations

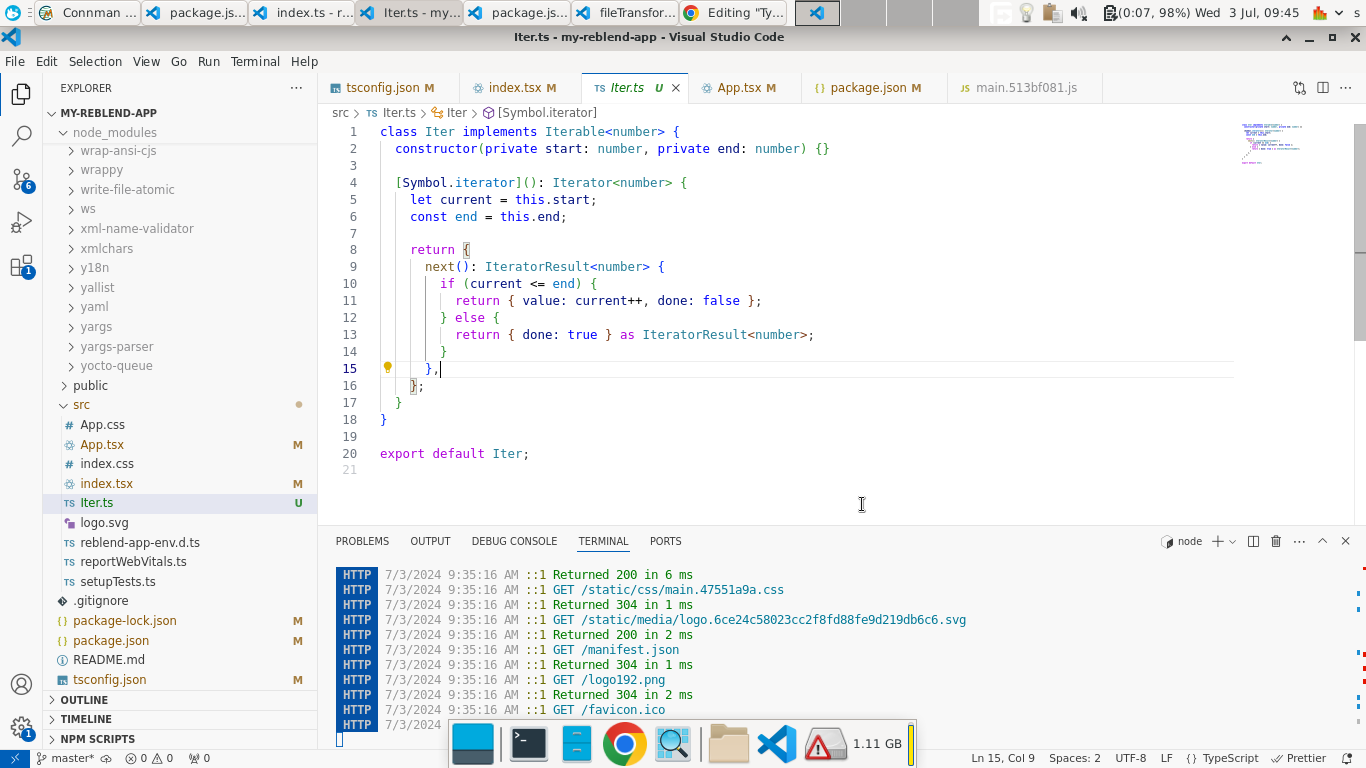
Introduction
TypeScript and Java are two popular programming languages that are often used in different contexts: TypeScript in the world of web development and Java in enterprise and Android development. Despite their differences, both languages provide mechanisms for creating iterable data structures. Understanding how to implement iterables in TypeScript and Java can be incredibly useful for developers working in either language. In this blog post, we will explore how to create and use iterable implementations in TypeScript and Java.
Understanding Iterables
An iterable is an object that can be iterated over, typically using a for...of
loop in TypeScript or an enhanced for
loop in Java. Iterables provide a standard way to access elements sequentially without exposing the underlying data structure.
TypeScript Iterables
In TypeScript, the concept of iterables is built on top of JavaScript's iterators and iterables introduced in ECMAScript 2015 (ES6). An object is considered iterable if it implements the @@iterator
method, which returns an iterator. An iterator is an object with a next
method that returns the next item in the sequence.
Creating an Iterable in TypeScript
To create an iterable in TypeScript, you need to define an object that implements the Iterable
interface. Here is an example of a simple iterable implementation:
class Iter implements Iterable<number> {
constructor(private start: number, private end: number) {}
[Symbol.iterator](): Iterator<number> {
let current = this.start;
const end = this.end;
return {
next(): IteratorResult<number> {
if (current <= end) {
return { value: current++, done: false };
} else {
return { done: true } as IteratorResult<number>;
}
}
};
}
}
const iter = new Iter(1, 5);
for (const num of iter) {
console.log(num); // 1, 2, 3, 4, 5
}
In this example, the Iter
class implements the Iterable
interface. The [Symbol.iterator]()
method returns an iterator object that has a next
method, which yields the numbers from the start to the end.
Using Generators for Iterables
TypeScript also supports generators, which provide a simpler way to create iterables. Here is an example using a generator function:
function* iterGenerator(start: number, end: number): IterableIterator<number> {
for (let i = start; i <= end; i++) {
yield i;
}
}
const genIter = iterGenerator(1, 5);
for (const num of genIter) {
console.log(num); // 1, 2, 3, 4, 5
}
The iterGenerator
function is a generator that yields values from the start to the end. Using a generator makes the code more concise and easier to understand.
Java Iterables
In Java, iterables are part of the core language, with the Iterable
interface being a key component of the Java Collections Framework. An object that implements the Iterable
interface must provide an iterator
method that returns an Iterator
.
Creating an Iterable in Java
To create an iterable in Java, you need to define a class that implements the Iterable
interface and provides an iterator. Here is an example:
import java.util.Iterator;
import java.util.NoSuchElementException;
public class Iter implements Iterable<Integer> {
private final int start;
private final int end;
public Iter(int start, int end) {
this.start = start;
this.end = end;
}
@Override
public Iterator<Integer> iterator() {
return new Iterator<Integer>() {
private int current = start;
@Override
public boolean hasNext() {
return current <= end;
}
@Override
public Integer next() {
if (!hasNext()) {
throw new NoSuchElementException();
}
return current++;
}
};
}
public static void main(String[] args) {
Iter iter = new Iter(1, 5);
for (int num : iter) {
System.out.println(num); // 1, 2, 3, 4, 5
}
}
}
In this example, the Iter
class implements the Iterable
interface. The iterator
method returns an Iterator
that provides the numbers from the start to the end. The hasNext
method checks if there are more elements, and the next
method returns the next element in the sequence.
Comparing TypeScript and Java Iterables
While both TypeScript and Java provide mechanisms for creating iterables, there are some differences in their implementations:
Syntax: TypeScript uses the
Symbol.iterator
method and can leverage generator functions for a more concise syntax. Java uses theIterable
interface and requires implementing theiterator
method.Error Handling: In Java, calling
next
on an iterator that has no more elements throws aNoSuchElementException
. In TypeScript, thenext
method returns an object with adone
property to indicate the end of the sequence.Flexibility: TypeScript's generator functions offer a more flexible and less boilerplate-heavy way to create iterables compared to Java's explicit iterator implementation.
Conclusion
Understanding how to implement and use iterables in TypeScript and Java can enhance your ability to work with sequences of data in both languages. While TypeScript provides a more modern and concise approach with generators, Java offers a robust and well-established framework for iterables within its collections framework. By mastering iterables in both languages, you can write more efficient, readable, and maintainable code.
Happy coding!
Subscribe to my newsletter
Read articles from Emmanuel Paul Elom directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Emmanuel Paul Elom
Emmanuel Paul Elom
Experienced Full Stack Developer with expertise in JAVA, JavaScript, TypeScript, NodeJS, Rust, ExpressJS, and PHP. Passionate about open-source contributions and creating scalable solutions. Seeking a challenging role to leverage my skills in both front-end and back-end development to drive innovation and efficiency.