Introduction to Functional Programming in Elixir: Key Concepts You Need to Know
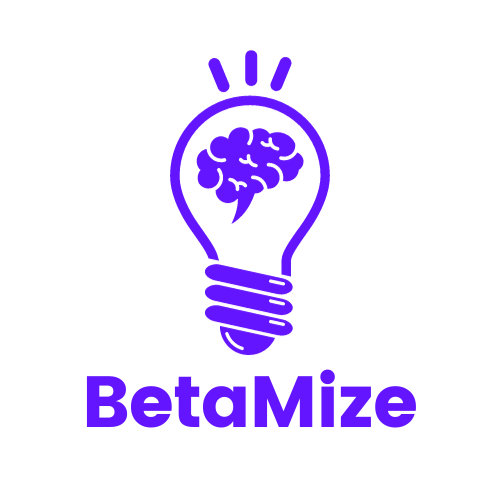
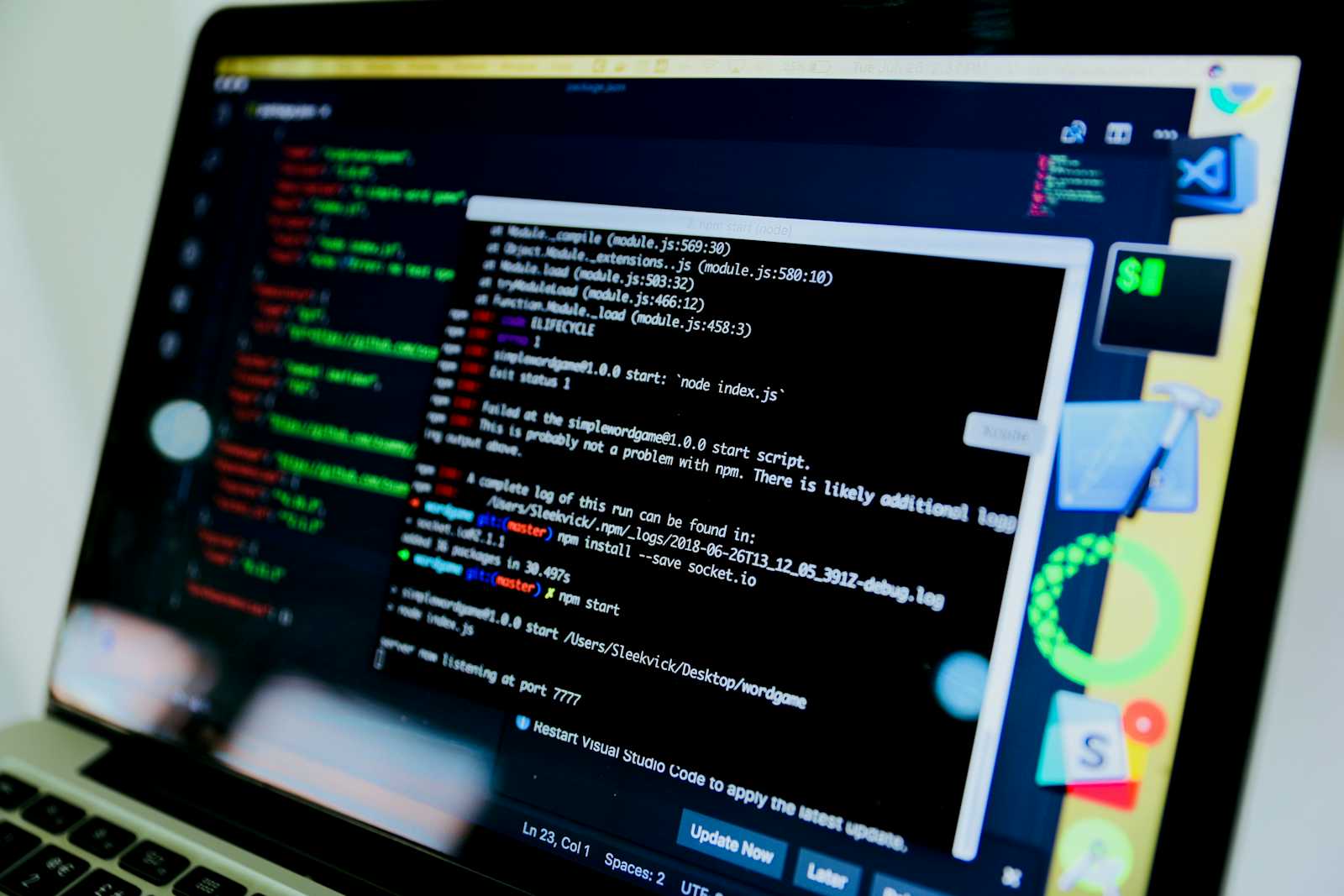
In the realm of modern programming, functional programming stands out for its clarity, conciseness, and robustness. Elixir, a dynamic and functional language built on the Erlang VM, is an excellent choice for developers looking to harness the power of functional programming. In this blog, we'll dive into the core principles of functional programming in Elixir: immutability, first-class functions, higher-order functions, and recursion. Plus, we'll explore how Elixir's built-in functions facilitate functional programming. Ready to elevate your coding skills? Let's get started!
Immutability: The Cornerstone of Functional Programming
One of the foundational principles of functional programming is immutability. In Elixir, once a variable is assigned a value, that value cannot be changed. This leads to more predictable and less error-prone code. Consider the following example:
x = 10
x = x + 5
In many programming languages, the value of x
would be updated to 15. However, in Elixir, the second assignment creates a new variable, preserving the immutability of the original x
.
First-Class Functions: Treating Functions as Values
Elixir treats functions as first-class citizens, meaning functions can be assigned to variables, passed as arguments, and returned from other functions. This flexibility allows for more modular and reusable code. Here's a simple example:
add = fn a, b -> a + b end
result = add.(5, 3) # result is 8
In this example, add
is a function assigned to a variable, and it can be invoked using the .(...)
syntax.
Higher-Order Functions: Functions That Operate on Other Functions
Higher-order functions take one or more functions as arguments and/or return a function. This enables powerful abstractions and code reuse. Elixir's Enum
module is packed with higher-order functions:
list = [1, 2, 3, 4]
Enum.map(list, fn x -> x * 2 end) # [2, 4, 6, 8]
In this example, Enum.map
applies the anonymous function fn x -> x * 2 end
to each element in the list.
Recursion: A Natural Way to Iterate
Recursion, the process of a function calling itself, is a natural fit for functional programming and is often used instead of loops. Elixir's tail call optimization ensures that recursive calls are efficient. Here's a simple recursive function to calculate the factorial of a number:
defmodule Math do
def factorial(0), do: 1
def factorial(n) when n > 0 do
n * factorial(n - 1)
end
end
Math.factorial(5) # 120
Elixir's Built-in Functions for Functional Programming
Elixir provides a rich set of built-in functions that facilitate functional programming. The Enum
and Stream
modules are particularly noteworthy. For instance, Enum.reduce
can be used to accumulate values in a list:
list = [1, 2, 3, 4]
sum = Enum.reduce(list, 0, fn x, acc -> x + acc end) # 10
Conclusion
Embracing functional programming in Elixir allows you to write more robust, maintainable, and concise code. The principles of immutability, first-class functions, higher-order functions, and recursion, along with Elixir's powerful built-in functions, make it an ideal language for modern software development.
If you're eager to master Elixir and take your functional programming skills to the next level, check out ElixirMasters. ElixirMasters offers top-notch Elixir and Phoenix development services, Elixir developer hiring solutions, and consultancy to help you build and scale your projects effectively. Start your journey today and unlock the full potential of functional programming with Elixir!
Subscribe to my newsletter
Read articles from BetaMize directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
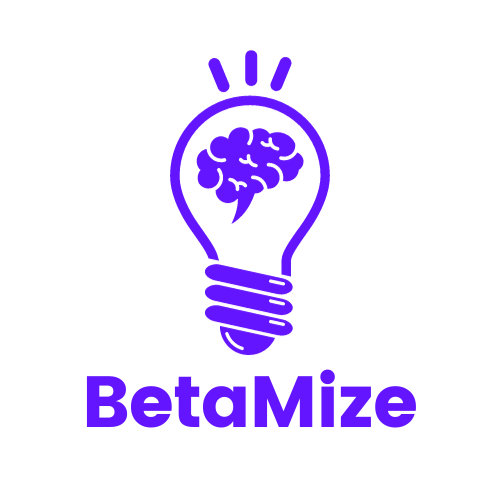