Boosting Laravel Performance for High Traffic Sites

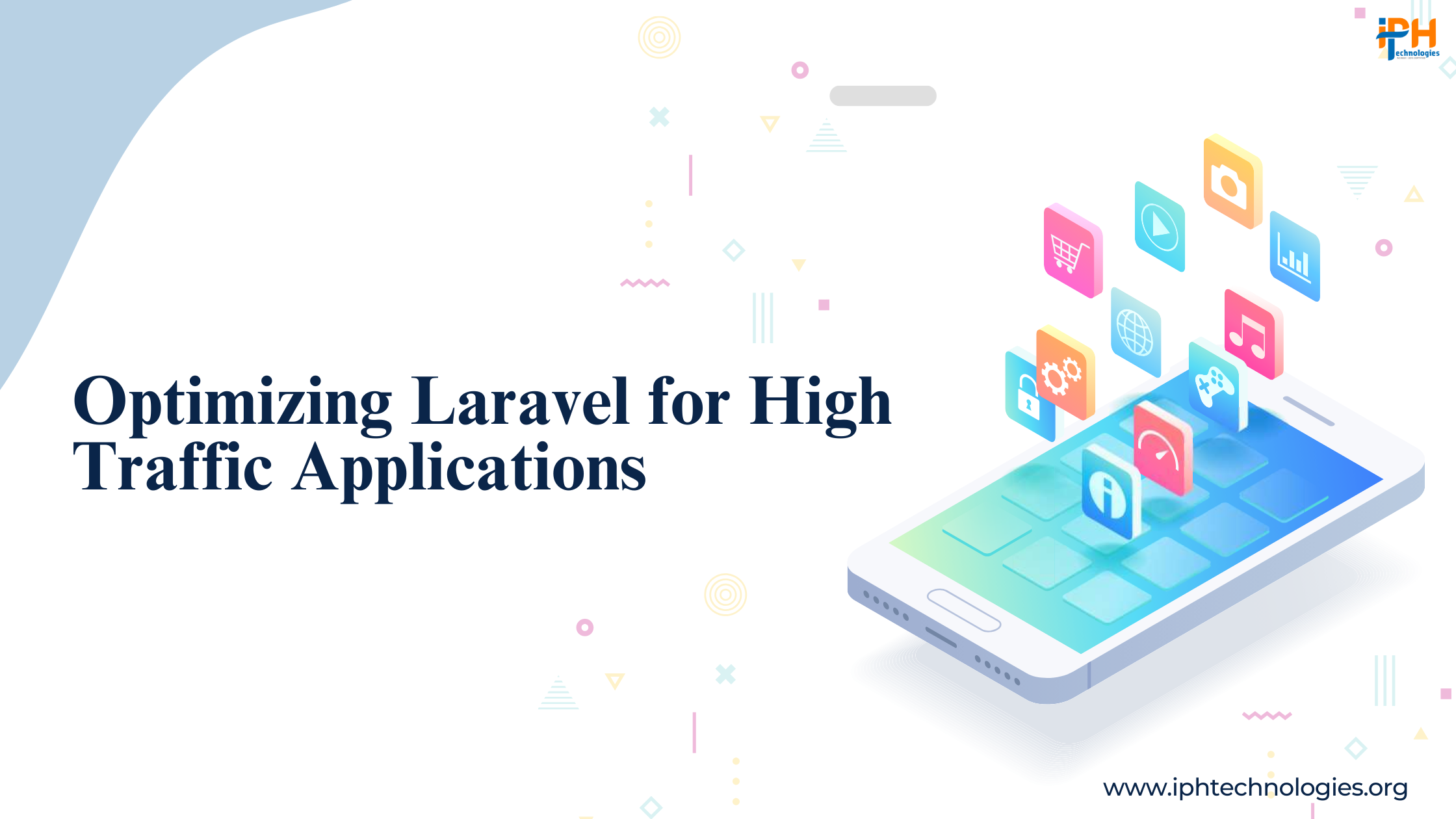
In today’s fast-paced digital landscape, where the performance and scalability of web applications can make or break a business, Laravel stands out as a powerful PHP framework. Optimizing Laravel for high traffic applications ensures seamless user experiences, quick load times, and robust performance under heavy loads.
Understanding Laravel's Scalability
Laravel’s architecture is inherently designed to be scalable. Its modular structure and built-in features like caching, queues, and database optimization make it a go-to choice for developing high-performance web applications. However, as traffic increases, it’s essential to fine-tune these elements to handle the load efficiently.
1. Efficient Caching Strategies
Caching is a fundamental technique for improving the performance of any web application. Laravel provides several caching drivers like Memcached, Redis, and database caching. For high traffic applications, Redis or Memcached are preferred due to their in-memory data storage capabilities, which significantly reduce the time to fetch frequently accessed data.
Implementation Tip:
// Configure Redis in .env file CACHE_DRIVER=redis
// Use caching in your controller use Illuminate\Support\Facades\Cache;
$data = Cache::remember('cache_key', $seconds, function () { return DB::table('your_table')->get(); });
2. Optimizing Database Queries
Efficient database interaction is critical for high traffic applications. Laravel’s Eloquent ORM simplifies database operations, but it's crucial to write optimized queries to avoid performance bottlenecks.
Best Practices:
- Use Eager Loading: Avoid N+1 query issues by using eager loading.
// Eager load relationships $users = User::with('posts')->get();
Optimize Indexes: Ensure your database tables have proper indexing.
Use Query Caching: Cache complex queries to reduce load on the database.
3. Implementing Queues
Queues help in offloading time-consuming tasks, thereby improving the response time of your application. Laravel’s built-in queue system supports various queue drivers, including Redis, Beanstalkd, and Amazon SQS.
Example:
// Dispatch a job to the queue use App\Jobs\SendEmailJob;
SendEmailJob::dispatch($user);
4. Load Balancing and Horizontal Scaling
For applications expecting substantial traffic, load balancing is essential. Distribute incoming requests across multiple servers to prevent any single server from becoming a bottleneck.
Steps to Implement:
Use a load balancer like NGINX or AWS Elastic Load Balancer.
Scale horizontally by adding more servers as traffic grows.
5. Utilizing Content Delivery Networks (CDNs)
CDNs distribute your content across multiple servers globally, reducing latency and improving load times for users worldwide. Integrate CDNs like Cloudflare or AWS CloudFront with your Laravel application for optimal performance.
6. Monitoring and Profiling
Continuous monitoring and profiling help in identifying performance issues before they impact your users. Tools like Laravel Telescope, New Relic, and Blackfire.io provide insights into your application’s performance.
Example:
// Installing Laravel Telescope composer require laravel/telescope
// Publishing the Telescope service provider php artisan telescope:install php artisan migrate
7. Optimizing Frontend Performance
While backend optimization is crucial, frontend performance also plays a significant role in overall user experience. Minify CSS and JavaScript files, leverage browser caching, and optimize images to ensure quick load times.
Conclusion
Optimizing Laravel for high traffic applications involves a multi-faceted approach, from efficient caching and database optimization to implementing queues and load balancing. By leveraging Laravel’s built-in features and following best practices, you can build robust, high-performing web applications capable of handling substantial traffic. If you’re looking for expert assistance, our Laravel Development Services offer tailored solutions to help your business thrive in the digital landscape.
By following these strategies, you’ll ensure your Laravel application not only meets but exceeds the expectations of your high traffic demands. For more insights and personalized solutions, feel free to reach out to us.
Subscribe to my newsletter
Read articles from Sam Miller directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sam Miller
Sam Miller
Best App Development Company In USA To provide the greatest custom business process solutions, we employ technology, agile processes, and expertise. Our team of experts is committed to providing you with the most effective technological solutions to help you remain ahead of the competition. We recognize the importance of remaining one step ahead against peers in an ever-changing market, which is why we offer some of the best-in-class technological solutions and great client experience.