Rotate the array by K places
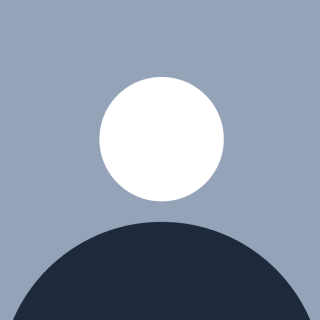
Question link - https://leetcode.com/problems/rotate-array/
Approach 1
Brute Force approach
Create a temporary vector that will store the number of arrays present to be rotated.
Now we need to normalize the rotation because for example if the size of the array is 6 and we need to rotate 7 digits then effectively we need to rate only 1 because after 6 rotations we will get the original array.
Initialize a variable here we have taken the count to 1, this will help us shift the numbers without disturbing the sequence.
Store the numbers to be rotated in the separate array, now shift the position of the numbers using the reverse for loop on the array.
Finally, add the rotated numbers to the front.
code
class Solution {
public:
void rotate(vector<int>& nums, int k) {
vector<int> arr;
int count = 1;
int n = nums.size();
int r = k % nums.size(); // Normalize k
// Store the last r elements in a temporary array
for(int i = nums.size() - r; i < nums.size(); i++) {
arr.push_back(nums[i]);
}
// Shift the elements to the right
for(int i = nums.size() - r - 1; i >= 0; i--) {
nums[n - count] = nums[i];
count++;
}
// Put the rotated elements
for(int i = 0; i < r; i++) {
nums[i] = arr[i];
}
}
};
Approach 2
Optimal Approach
First of all we will normalize the rotation like we did in the brute force approach.
We will reverse the entire array.
Now since the array is in the reversed manner we will again rotate the numbers to be rotated as given in the question.
Now we will reverse the rest of the numbers again to convert the array into the initial state.
Dry run
let the array be [1,2,3,4,5,6,7] and we need to rotate it by 3 digits
So our r is 3 in this case.
Reverse the array -> [7,6,5,4,3,2,1].
reverse the digits to be rotated -> [6,7,4,5,3,2,1].
Now reverse the rest of the array again -> [6,7,1,2,3,4,5].
class Solution {
public:
void rotate(vector<int>& nums, int k) {
//handle the edge case
int r = k%nums.size();
// optimal approach
reverse(nums.begin(), nums.end());
// reverse the rotated numbers
reverse(nums.begin(), nums.begin()+r);
//reverse rest of the numbers
reverse(nums.begin()+r, nums.end());
}
};
Just leetcode things
Video for your reference
All the best for your DSA journey! Let me know your feedback so that we can improve this article together.
Ujjwal Sharma
Subscribe to my newsletter
Read articles from Ujjwal Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by