Understanding CSRF Tokens: Protecting Your Web Applications
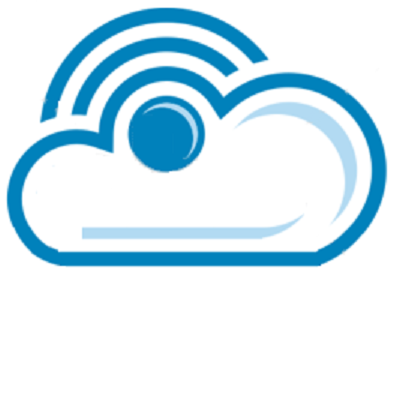
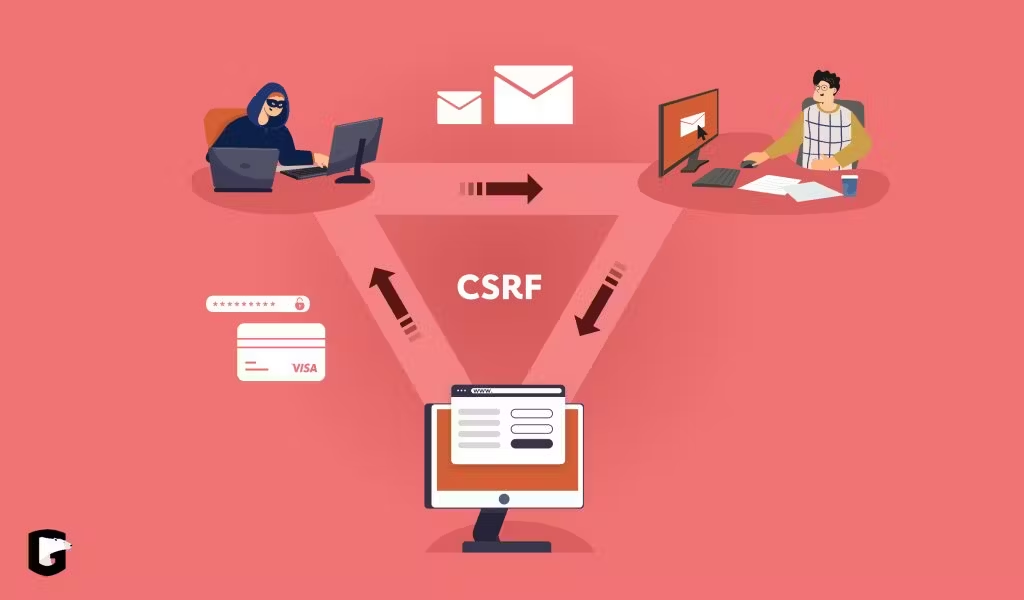
Protecting Your Web Applications
Introduction
Cross-Site Request Forgery (CSRF) is a common web security vulnerability that allows an attacker to trick a user's browser into making unwanted requests to a different site without the user's consent. CSRF attacks can result in unauthorized actions such as changing account details, making unauthorized transactions, or altering user settings. One of the most effective ways to prevent CSRF attacks is through the use of CSRF tokens. This article explores what CSRF tokens are, how they work, and why they are essential for securing web applications.
What is CSRF?
Cross-Site Request Forgery (CSRF) is a type of attack that occurs when a malicious website, email, or program causes a user's browser to perform an unwanted action on a trusted site where the user is authenticated. Because the browser includes the user's session information with the request (such as cookies), the server cannot distinguish between a legitimate request from the user and a malicious request from an attacker.
How CSRF Tokens Work
CSRF tokens work by adding a secret, unique, and unpredictable token to each request that changes state on the server. Here's how it works:
Token Generation: When a user visits a site, the server generates a CSRF token and embeds it in the HTML form or HTTP headers.
Token Submission: When the user submits a form or makes a state-changing request, the token is included in the request.
Token Validation: The server checks the submitted token against the token stored in the user's session. If the tokens match, the request is considered legitimate; otherwise, it is rejected.
Example of CSRF Token Implementation
Here is a simple example of how CSRF tokens can be implemented in a web application:
Server-Side (e.g., using Express in Node.js)
const express = require('express');
const csrf = require('csurf');
const cookieParser = require('cookie-parser');
const app = express();
app.use(cookieParser());
const csrfProtection = csrf({ cookie: true });
app.get('/form', csrfProtection, (req, res) => {
res.send(`
<form action="/process" method="POST">
<input type="hidden" name="_csrf" value="${req.csrfToken()}">
<button type="submit">Submit</button>
</form>
`);
});
app.post('/process', csrfProtection, (req, res) => {
res.send('Form submission was successful');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Client-Side
In the form rendered by the server, the CSRF token is included as a hidden input field:
<form action="/process" method="POST">
<input type="hidden" name="_csrf" value="the-generated-token">
<button type="submit">Submit</button>
</form>
When the form is submitted, the CSRF token is included in the request and validated by the server.
Benefits of Using CSRF Tokens
Protection Against CSRF Attacks
CSRF tokens provide a robust defense against CSRF attacks by ensuring that state-changing requests can only be made by the legitimate user and not by an attacker.
Easy to Implement
CSRF tokens are relatively easy to implement in web applications, especially with the availability of libraries and frameworks that provide built-in support for CSRF protection.
Compatible with Various Technologies
CSRF tokens can be used with different web technologies and frameworks, making them a versatile solution for protecting web applications.
Conclusion
CSRF tokens are an essential security measure for protecting web applications from cross-site request forgery attacks. By ensuring that state-changing requests include a valid token, CSRF tokens help prevent unauthorized actions and protect user data. Implementing CSRF tokens is a straightforward and effective way to enhance the security of your web applications.
If you found this article helpful and want to stay updated with more content like this, please leave a comment below and subscribe to our blog newsletter. Stay informed about the latest in web application security and development practices!
We value your feedback! Please share your thoughts in the comments section and don't forget to subscribe to our newsletter for more informative articles and updates.
Subscribe to my newsletter
Read articles from Cloud Tuned directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
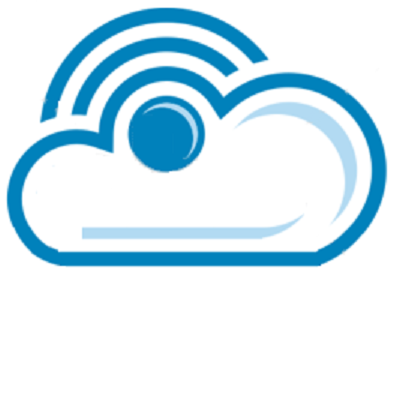