Promises in JavaScript: A Real-Life Example
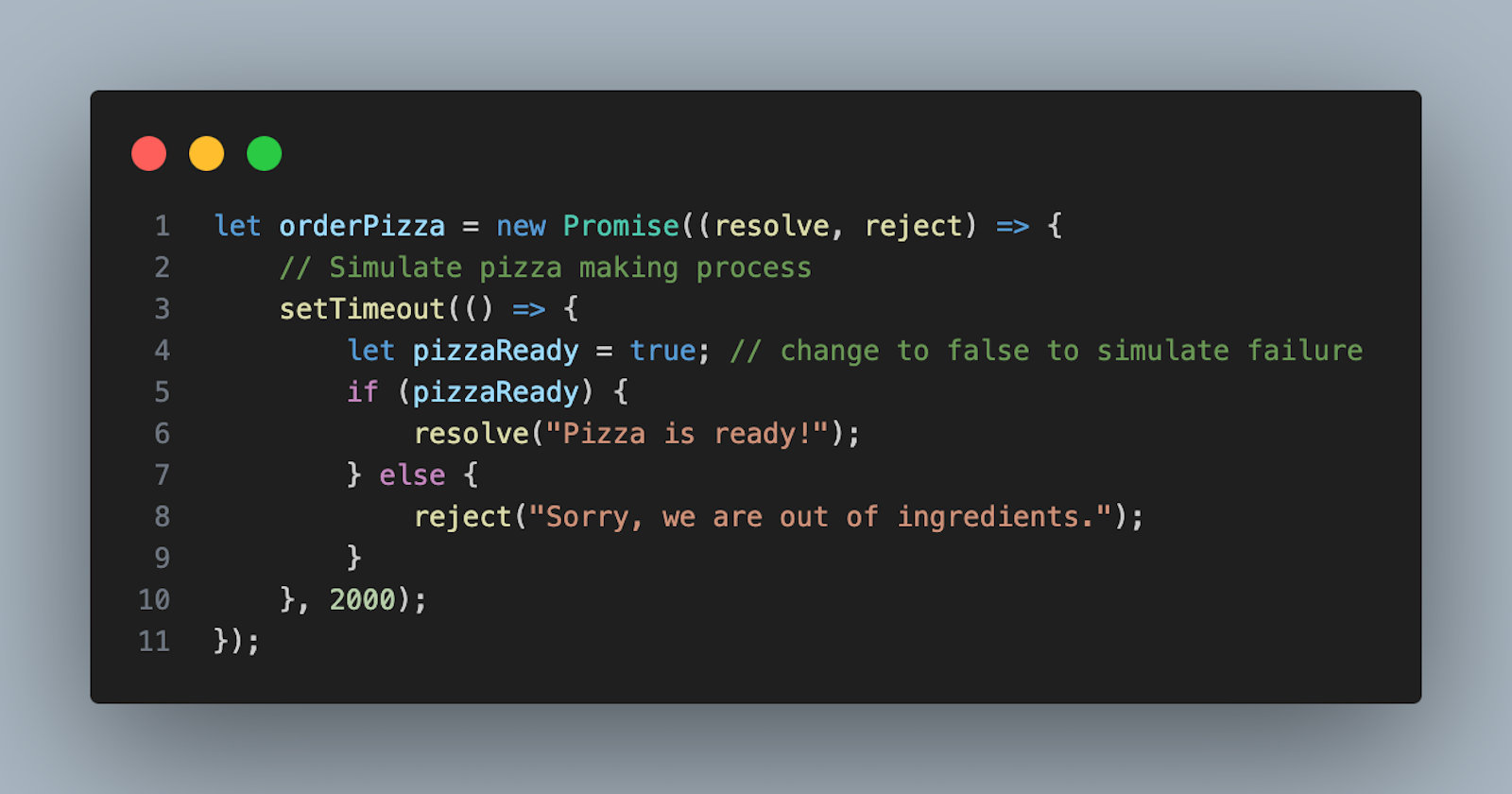
Introduction
JavaScript Promises can be a bit tricky to understand at first, but they’re a game-changer for handling asynchronous operations. Let’s dive into what Promises are, why they’re essential, and how they can be related to real-life situations.
What are Promises?
A Promise in JavaScript is an object that represents the eventual completion (or failure) of an asynchronous operation and its resulting value. Think of it as a placeholder for a value that isn’t available yet but will be at some point in the future.
Promise States
A Promise can be in one of three states:
Pending: The initial state, neither fulfilled nor rejected.
Fulfilled: The operation completed successfully.
Rejected: The operation failed.
Real-Life Example: Ordering Pizza
Imagine you’re ordering a pizza. Here’s how the process goes:
You call the pizza place and place your order.
The pizza place confirms your order and gives you an estimated delivery time.
You wait for the pizza to arrive.
The pizza arrives, and you enjoy your meal.
Now, let’s map this to how Promises work in JavaScript.
Placing the Order: Creating a Promise
When you place the order, you’re essentially creating a promise. You’re saying, “I want a pizza, and I expect it to be delivered.” In JavaScript, you create a promise like this:
let orderPizza = new Promise((resolve, reject) => {
// Simulate pizza making process
setTimeout(() => {
let pizzaReady = true; // change to false to simulate failure
if (pizzaReady) {
resolve("Pizza is ready!");
} else {
reject("Sorry, we are out of ingredients.");
}
}, 2000);
});
Waiting for the Pizza: Using then
and catch
While you wait for the pizza, you don’t just sit there doing nothing. You might watch TV or do something else. This is where the .then()
method comes in. It lets you define what happens when the promise is fulfilled.
orderPizza.then((message) => {
console.log(message); // "Pizza is ready!"
}).catch((error) => {
console.log(error); // "Sorry, we are out of ingredients."
});
Real-Life Scenario Breakdown
Placing the Order (Creating a Promise): You initiate the asynchronous task.
Pizza Making Process (Promise Execution): The task is in progress, and you wait.
Pizza Delivered (Promise Resolved): The task is successfully completed, and you get the desired result.
Pizza Not Delivered (Promise Rejected): Something goes wrong, and you handle the failure.
Why Use Promises?
Promises are essential because they allow you to handle asynchronous tasks in a more manageable way. Without Promises, you’d end up with “callback hell,” where nested callbacks make the code hard to read and maintain.
Another Example: Fetching Data
Let’s look at another example: fetching data from an API.
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Here, fetch
returns a promise. The .then()
methods handle the successful data retrieval, and the .catch()
method handles any errors that occur during the fetch operation.
Conclusion
Promises simplify the process of working with asynchronous code in JavaScript. By understanding them through real-life examples, you can see how they help manage tasks that don’t complete immediately. So next time you’re dealing with asynchronous operations, think of them as ordering a pizza – place your order, wait patiently, and handle the outcome, whether it’s good or bad.
Liked reading?
Let me know in the comments section, and do like & subscribe to my newsletter.
Subscribe to my newsletter
Read articles from Animesh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Animesh Kumar
Animesh Kumar
I’m a software developer who loves problem-solving, data structures, algorithms, and competitive coding. I’ve a keen interest in product development. I’m passionate about AI, ML, and Python. I love exploring new ideas and enjoy innovating with advanced tech. I am eager to learn and contribute effectively to teams.