A Comprehensive Overview of Node.js
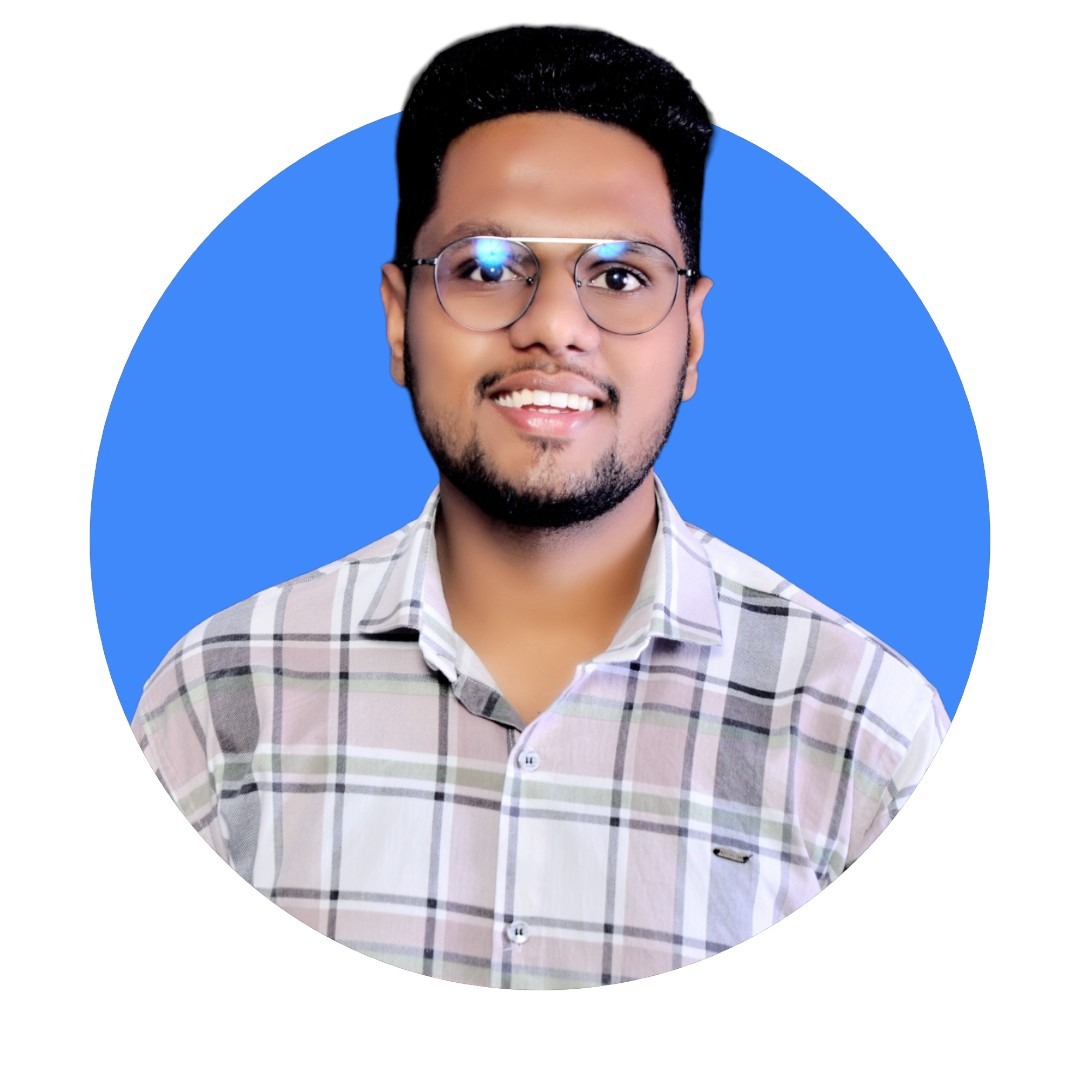
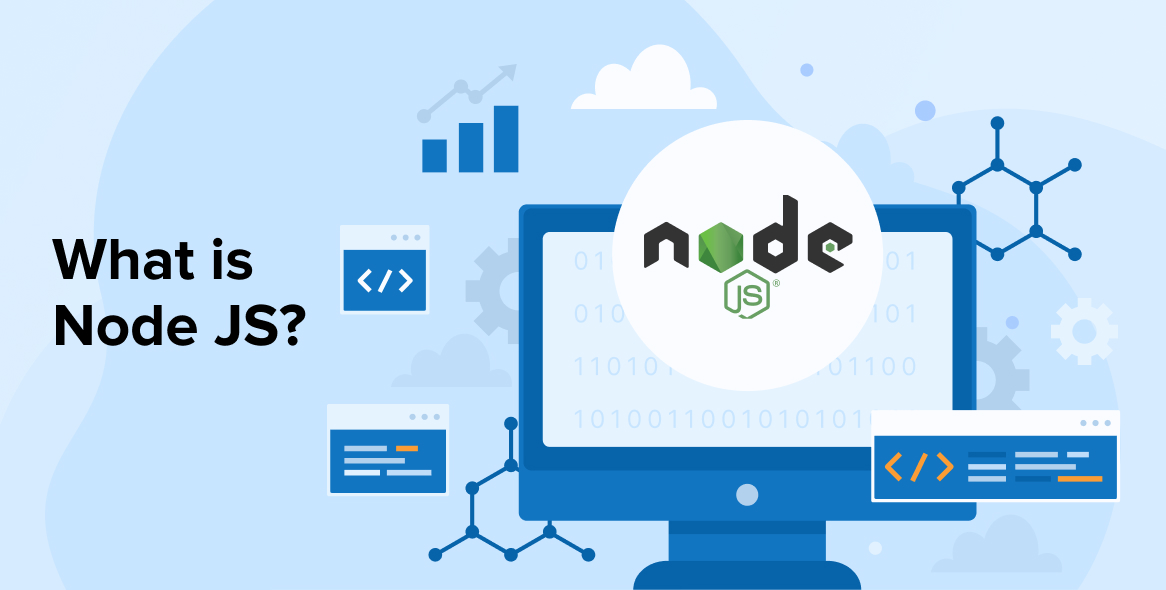
What is Node.js :
Node.js is a cross-platform runtime environment and library for running JavaScript applications outside the browser.
It is used for creating server-side and networking web applications.
It is open source and free to use.
Many of the basic modules of Node.js are written in JavaScript.
Node.js is mostly used to run real-time server applications.
Node.js uses an event-driven, non-blocking I/O model that makes it lightweight and efficient, perfect for data-intensive real-time applications that run across distributed devices.?
Node.js also provides a rich library of various JavaScript modules to simplify the development of web applications.
- Node.js = Runtime Environment + JavaScript Library
Different parts of Node.js :
The following diagram specifies some important parts of Node.js:
Features of Node.js :
Following is a list of some important features of Node.js that makes it the first choice of software architects.
Extremely fast: Node.js is built on Google Chrome's V8 JavaScript Engine, so its library is very fast in code execution.
I/O is Asynchronous and Event Driven: All APIs of Node.js library are asynchronous i.e. non-blocking. So a Node.js based server never waits for an API to return data. The server moves to the next API after calling it and a notification mechanism of Events of Node.js helps the server to get a response from the previous API call. It is also a reason that it is very fast.
Single threaded: Node.js follows a single threaded model with event looping.
Highly Scalable: Node.js is highly scalable because event mechanism helps the server to respond in a non-blocking way.
No buffering: Node.js cuts down the overall processing time while uploading audio and video files. Node.js applications never buffer any data. These applications simply output the data in chunks.
Open source: Node.js has an open source community which has produced many excellent modules to add additional capabilities to Node.js applications.
License: Node.js is released under the MIT license.
Node.js Console :
The Node.js console module provides a simple debugging console similar to JavaScript console mechanism provided by web browsers.
There are three console methods that are used to write any node.js stream:
console.log()
console.error()
console.warn()
Node.js console.log() :
The console.log() function is used to display simple message on console.
- console.log('Hello Student');
Open Node.js command prompt and run the following code:
- node console_example1.js
Ourput: Hello Student
Node.js console.error() :
The console.error() function is used to render error message on console.
- console.error(new Error('Hell! This is a wrong method.'));
Open Node.js command prompt and run the following code:
- node console_example3.js
Node.js console.warn()
The console.warn() function is used to display warning message on console.
File: console_example4.js
const name = 'John';
console.warn(`Don't mess with me ${name}! Don't mess with me!`);
Open Node.js command prompt and run the following code:
- node console_example4.js
Node.js REPL
The term REPL stands for Read Eval Print and Loop.
It specifies a computer environment like a window console or a Unix/Linux shell where you can enter the commands and the system responds with an output in an interactive mode.
REPL Environment
The Node.js or node come bundled with REPL environm/ent.
Each part of the REPL environment has a specific work.
Read: It reads user's input; parse the input into JavaScript data-structure and stores in memory.
Eval: It takes and evaluates the data structure.
Print: It prints the result.
Loop: It loops the above command until user press ctrl-c twice.
How to start REPL
You can start REPL by simply running "node" on the command prompt. See this:
You can execute various mathematical operations on REPL Node.js command prompt:
Node.js Simple expressions :
After starting REPL node command prompt put any mathematical expression:
Example: \>10+20-5
25
- Example2: \>10+12 + (5*4)/7
Using variable :
Variables are used to store values and print later.
If you don't use var keyword then value is stored in the variable and printed whereas if var keyword is used then value is stored but not printed.
You can print variables using console.log().
Example:
Node.js Multiline expressions
Node REPL supports multiline expressions like JavaScript. See the following do-while loop example:
var x = 0
undefined
> do {
... x++;
... console.log("x: " + x);
... } while ( x < 10 );
Node.js Underscore Variable :
You can also use underscore _ to get the last result.
Example:
Node.js REPL Commands :
Commands | Description |
ctrl + c | It is used to terminate the current command. |
ctrl + c twice | It terminates the node repl. |
ctrl + d | It terminates the node repl. |
up/down keys | It is used to see command history and modify previous commands. |
tab keys | It specifies the list of current command. |
.help | It specifies the list of all commands. |
.break | It is used to exit from multi-line expressions. |
.clear | It is used to exit from multi-line expressions. |
.save filename | It saves current node repl session to a file. |
.load filename | It is used to load file content in current node repl session. |
Node.js Exit REPL:
Use ctrl + c command twice to come out of Node.js REPL.
What Next?
Node.js Package Manager
Subscribe to my newsletter
Read articles from Aditya Gadhave directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
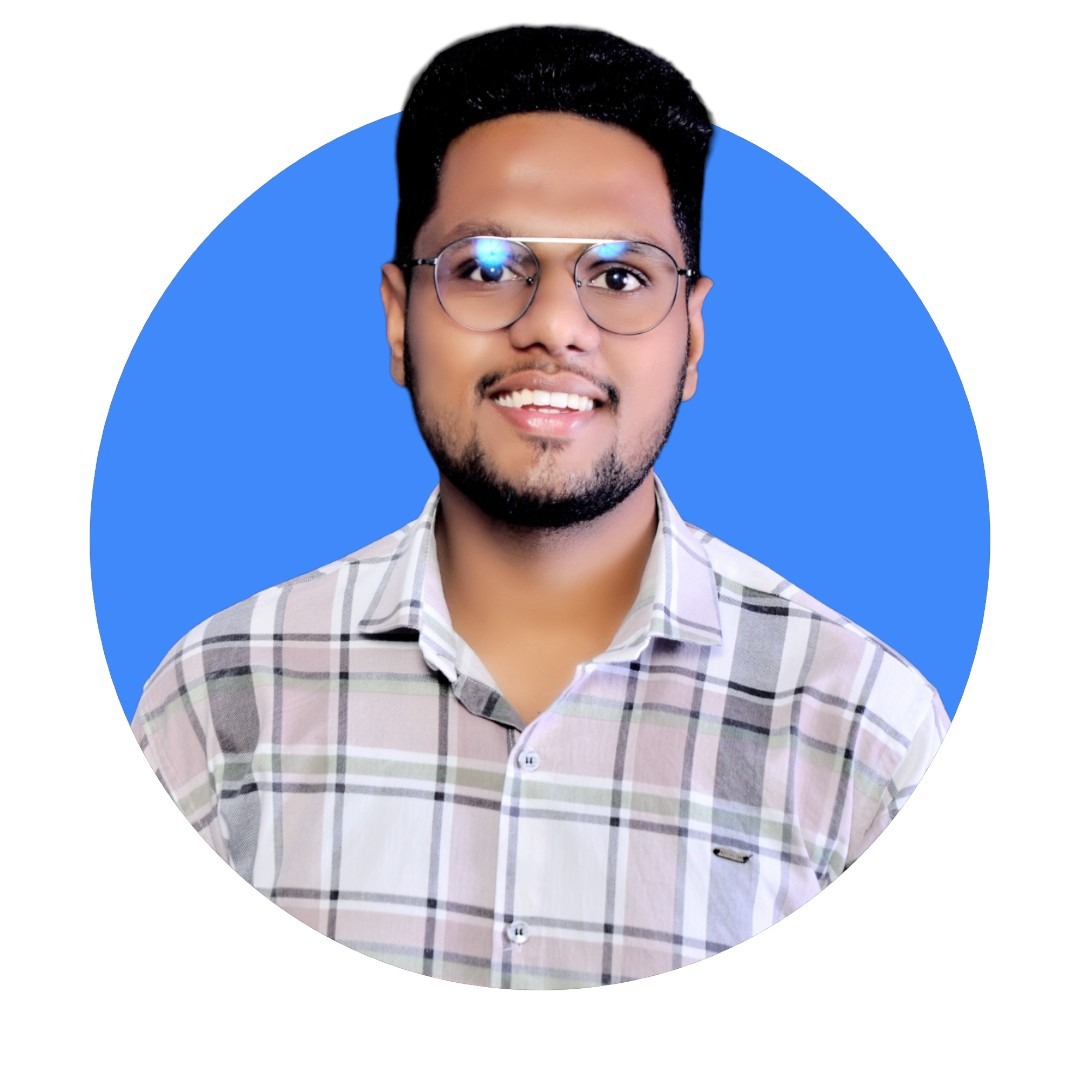
Aditya Gadhave
Aditya Gadhave
๐ Hello! I'm Aditya Gadhave, an enthusiastic Computer Engineering Undergraduate Student. My passion for technology has led me on an exciting journey where I'm honing my skills and making meaningful contributions.