How "Add" and "Offer" Work Differently in Queue Structures
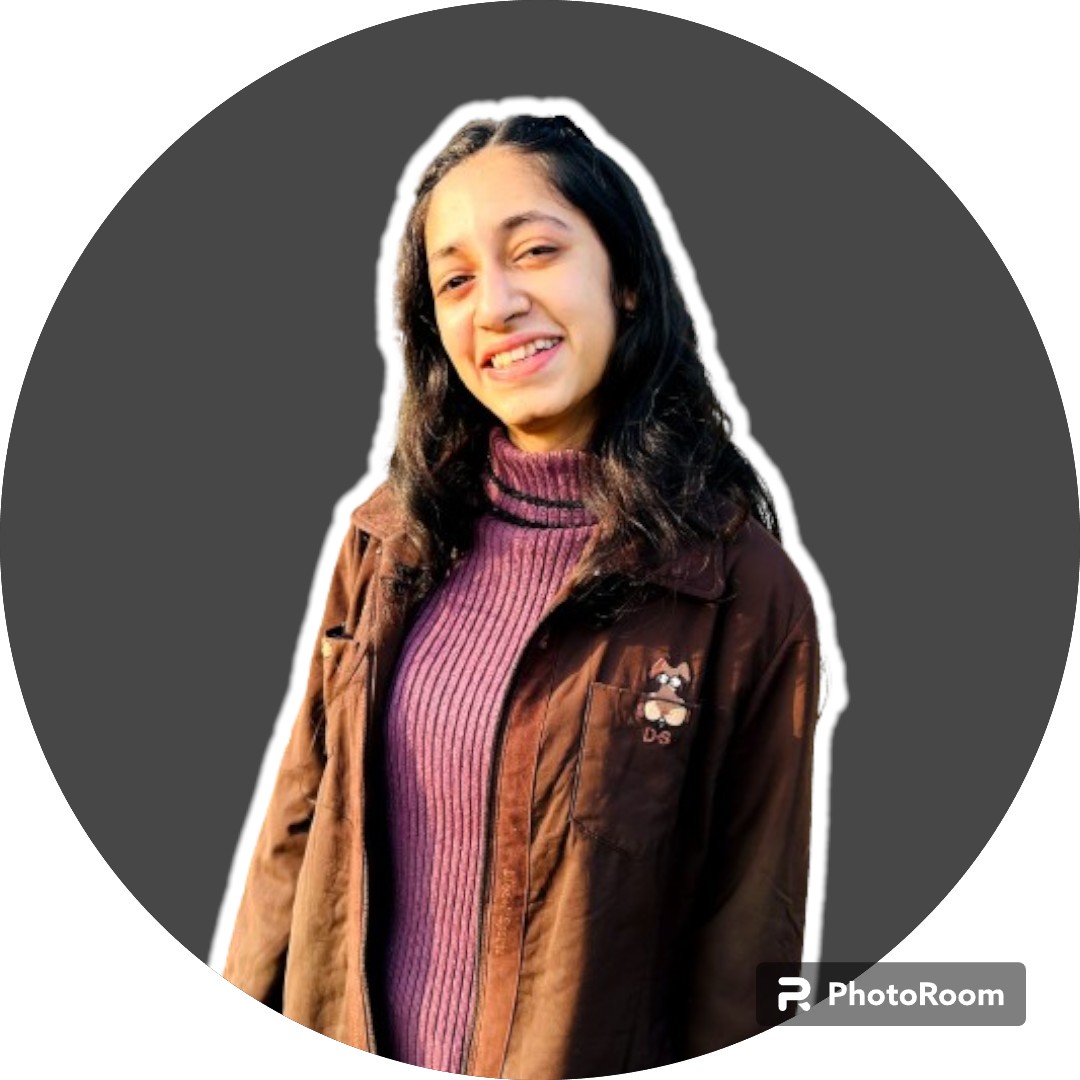
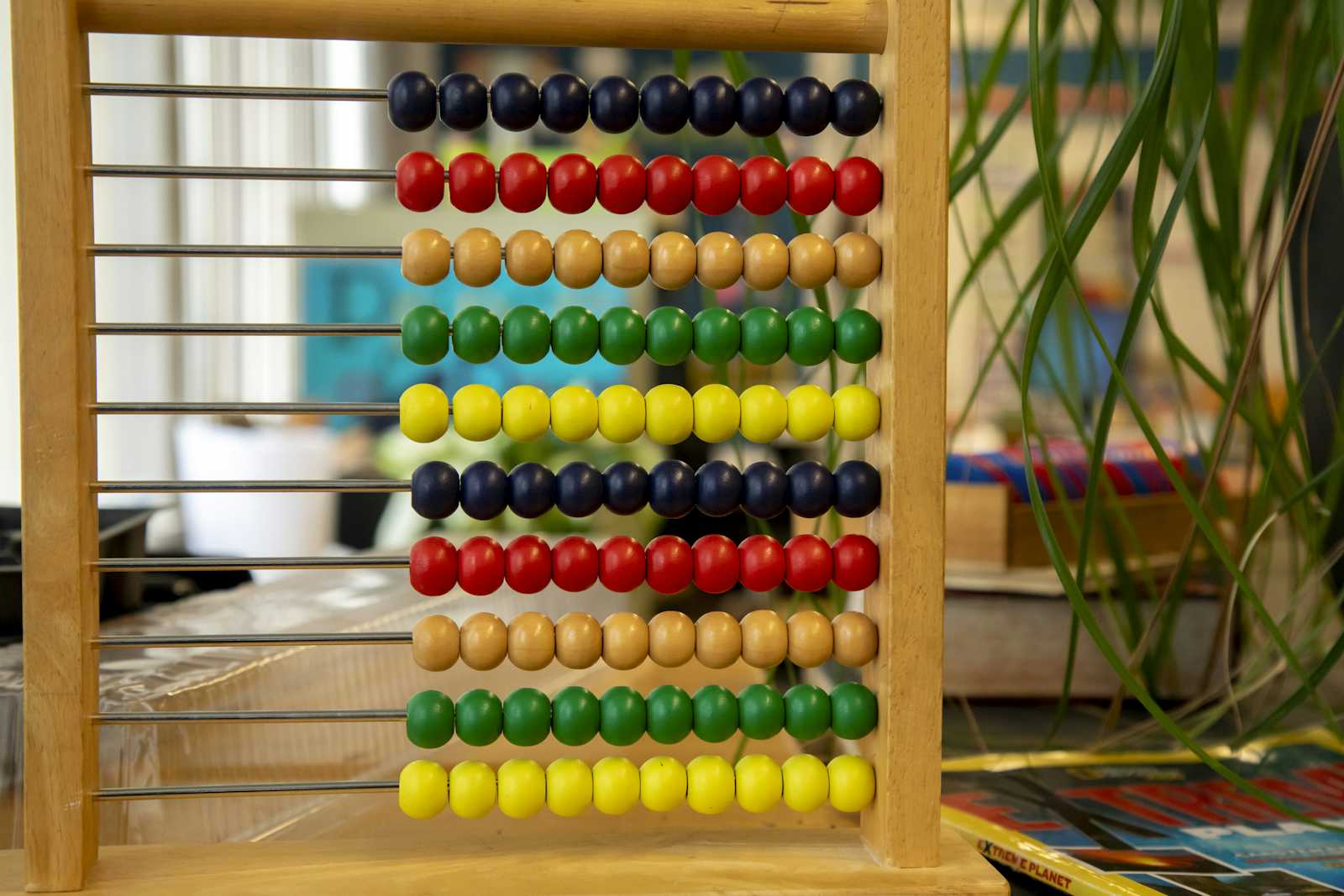
In the realm of data structures, the queue stands out for its simplicity and utility. A queue follows the First-In-First-Out (FIFO) principle, making it essential for managing tasks where order matters. Think of it as a line at a grocery store – the first person in line is the first to be served.
When it comes to implementing queues in Java, the Java Collections Framework (JCF) offers robust and flexible solutions. Two fundamental methods for inserting elements into a queue are add
and offer
. Although they might seem similar at first glance, their subtle differences can significantly impact how your application handles certain scenarios.
In this blog post, we’ll dive deep into these methods, exploring their usage, differences, and practical applications.
Understanding the Queue Interface in Java
Before we delve into add
and offer
, let’s get a quick overview of the queue interface in Java.
The Queue Interface
The Queue
interface in Java is part of the java.util
package and extends the Collection
interface. It represents a collection designed for holding elements prior to processing. Various implementations of the Queue
interface, like LinkedList
, PriorityQueue
, and ArrayDeque
, provide different characteristics suited to specific use cases.
Common Queue Operations
Enqueue (Insertion): Adding elements to the rear of the queue.
Dequeue (Removal): Removing elements from the front of the queue.
Peek: Viewing the front element without removing it.
IsEmpty: Checking if the queue is empty.
Size: Determining the number of elements in the queue.
The add
Method
The add
method inserts the specified element into the queue. Here's a quick look at its behavior:
Syntax:
boolean add(E e)
Return Value: Always returns
true
if the element is added successfully.Exception Handling:
Throws
IllegalStateException
if the queue has a fixed capacity and is full.Throws
NullPointerException
if the element being added isnull
and the queue implementation does not permitnull
elements.
Example Usage
Queue<Integer> queue = new LinkedList<>();
queue.add(1); // Adds the element successfully
queue.add(2); // Adds another element successfully
Using add
is straightforward and works well with queues like LinkedList
where there’s typically no strict capacity constraint.
When to Use add
Unbounded Queues: Ideal for queues without capacity limits, where adding elements is unlikely to fail due to size constraints.
Exception Handling: Suitable when you want to be explicitly informed through exceptions if an element cannot be added.
The offer
Method
The offer
method also inserts the specified element into the queue, but with a key difference in how it handles full capacity scenarios:
Syntax:
boolean offer(E e)
Return Value: Returns
true
if the element was added successfully; returnsfalse
if the queue is full.Exception Handling:
Does not throw exceptions if the queue is full.
Throws
NullPointerException
if the element being added isnull
and the queue implementation does not permitnull
elements.
Example Usage
Queue<Integer> queue = new LinkedList<>();
queue.offer(1); // Adds the element successfully
queue.offer(2); // Adds another element successfully
Using offer
is particularly beneficial when working with bounded queues, where you may encounter capacity constraints.
When to Use offer
Bounded Queues: Perfect for fixed-capacity queues where gracefully handling full capacity without exceptions is preferred.
Non-Disruptive Failure: Useful when you want the program to continue smoothly even if an element cannot be added due to capacity limits.
Practical Comparison
Capacity Handling
add
: Throws anIllegalStateException
if the queue is full. It’s strict and ensures you are immediately aware of the capacity issue.offer
: Returnsfalse
if the queue is full. It provides a non-exceptional way to handle full capacity, making it less disruptive.
Exception Behavior
add
: Enforces stricter exception handling. It’s useful when you want a clear and explicit signal that adding an element failed.offer
: Offers a lenient approach by returningfalse
on failure, making it more suitable for situations where you expect and can handle such scenarios gracefully.
Example of Bounded Queue with add
and offer
To illustrate these differences, let’s consider an ArrayBlockingQueue
with a fixed capacity of 2.
import java.util.concurrent.ArrayBlockingQueue;
ArrayBlockingQueue<Integer> boundedQueue = new ArrayBlockingQueue<>(2);
boundedQueue.add(1); // Adds successfully
boundedQueue.add(2); // Adds successfully
boundedQueue.add(3); // Throws IllegalStateException because the queue is full
ArrayBlockingQueue<Integer> anotherQueue = new ArrayBlockingQueue<>(2);
anotherQueue.offer(1); // Adds successfully
anotherQueue.offer(2); // Adds successfully
boolean wasAdded = anotherQueue.offer(3); // Returns false because the queue is full
System.out.println("Was the third element added? " + wasAdded); // Output: false
In the above example, add
throws an exception when trying to add the third element to a full queue, while offer
simply returns false
, allowing the program to handle the situation without interruption.
Conclusion
Understanding the subtle differences between add
and offer
is crucial for effectively managing queue operations in Java.
add
is suited for scenarios where exceptions are the preferred way to handle capacity constraints.offer
is ideal for bounded queues and scenarios where non-disruptive handling of capacity limits is required.
By choosing the appropriate method based on your needs, you can ensure that your application handles queue operations smoothly and efficiently. Whether you’re working with unbounded queues in a simple application or managing complex, high-performance bounded queues, knowing when to use add
or offer
will help you write more robust and resilient code.
Happy coding, and may your queues always flow smoothly!
Feel free to leave your thoughts or questions in the comments section below. Have you encountered any specific challenges with add
or offer
in your projects? How did you handle them? Let’s discuss!
Subscribe to my newsletter
Read articles from Mahak Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
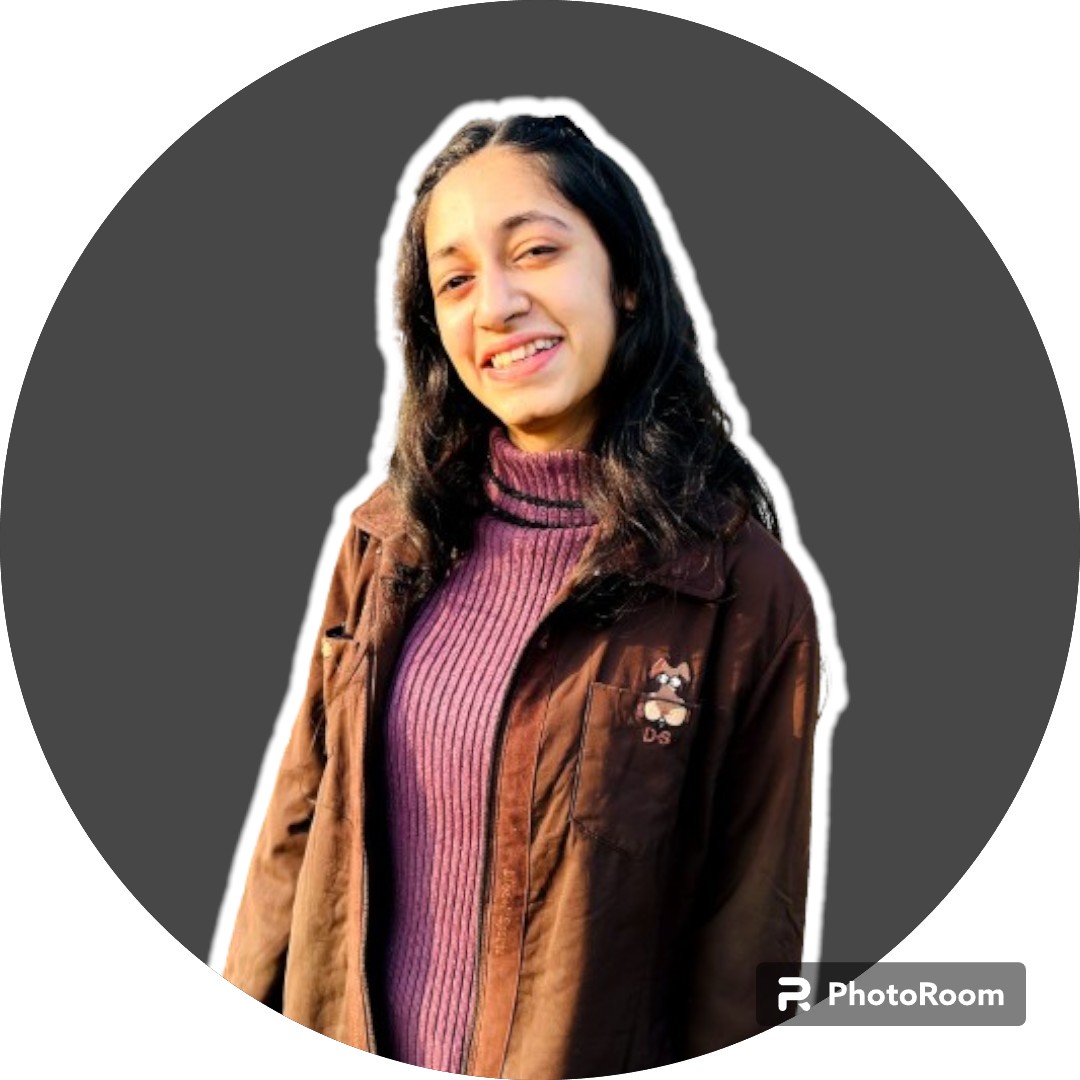
Mahak Pandey
Mahak Pandey
Hey, I am currently a 4th year student majoring in Computer science & Information Technology. I have a strong academic background with coursework in software development, database management, Operating System, OOPS and cybersecurity, and I’ve maintained a CGPA of 9.28. I am proficient in Java, HTML, CSS, Figma, JavaScript, Learning React and core Knowledge Of MERN STack. Additionally, I have hands-on experience with version control systems like Git, and I am familiar with Visual studio code IDE, working experience with Blender for 3D modeling and rendering, Figma for UI design and Prototyping, Canva for Designing assets. Here to share knowledge i've learned and learning. I recently publish the "Cyber Security" Book as a Co-Author. I like to learn and build in public. If you want to connect with me do follow my socials.