Step-by-Step Guide to Building Datasets for Fine-Tuning Language Models
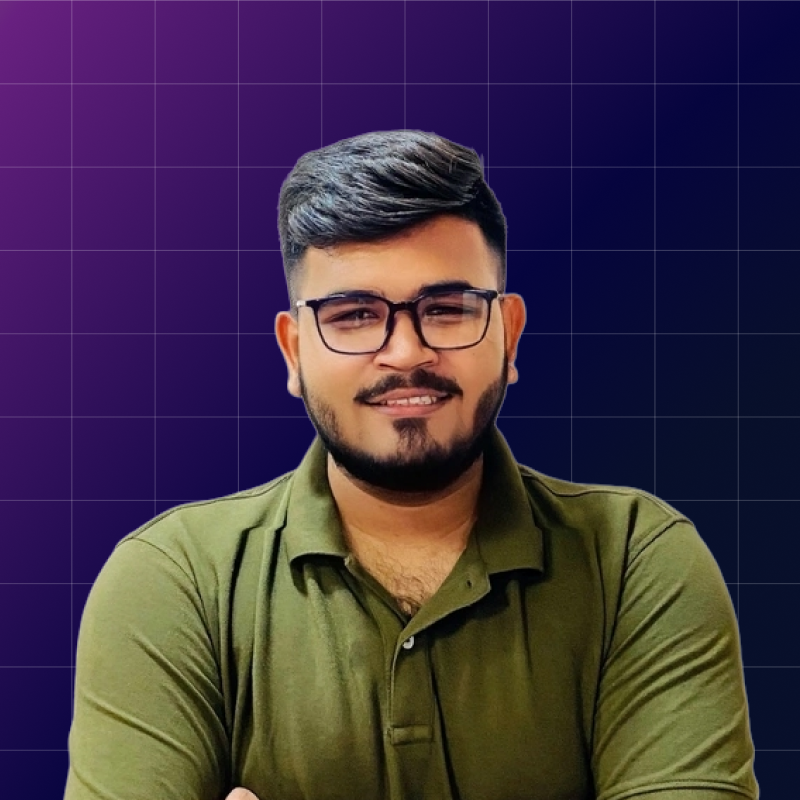

Best Practices for Building and Formatting Datasets for Language Model Fine-Tuning
Introduction
Fine-tuning language models (LLMs) like Llama 3, Google Gemma, and Mistral involves adapting pre-trained models to specific tasks using carefully curated datasets. This process is crucial for improving the models' performance on particular tasks by providing them with task-specific data during the training phase.
In this comprehensive guide, I will walk you through the detailed steps required to build high-quality datasets for fine-tuning LLMs. I will begin by discussing the importance of selecting relevant and diverse data to ensure the model can generalize well to various inputs. Next, I will cover techniques for cleaning and preprocessing your data to remove noise and inconsistencies, which can significantly impact the model's performance.
Additionally, I will provide examples of chat templates tailored for different LLMs, illustrating how to structure your data to align with the specific requirements of each model. These templates will serve as a foundation for creating effective training datasets that can improve the model's ability to understand and generate human-like text.
Furthermore, I will include code snippets to help you format your data correctly and push it to a hub, such as Hugging Face, for easy access and collaboration. By the end of this guide, you will have a thorough understanding of the fine-tuning process and the tools needed to optimize LLMs for your specific applications.
Step 1: Define Your Task
The first step in building high-quality datasets for fine-tuning LLMs is to clearly define the task you want the model to perform. This involves specifying the type of input the model will receive and the expected output. By having a well-defined task, you can ensure that the data you collect and preprocess is relevant and useful for training the model.
Here are some common tasks for which you might want to fine-tune your LLM:
Text Classification: This task involves categorizing text into predefined labels. For instance, you might classify customer reviews as positive, negative, or neutral. This is useful for sentiment analysis, spam detection, and topic categorization.
Text Generation: In this task, the model generates coherent and contextually relevant text based on a given prompt. Applications include writing assistance, story generation, and content creation. The objective is to produce text that is indistinguishable from human-written text.
Question Answering: This task involves providing accurate answers to user queries. The model is trained to understand the context of the question and retrieve or generate the most relevant answer. This is beneficial for customer support, educational tools, and search engines.
Dialogue Systems: This involves building conversational agents that can interact with users in a natural and engaging manner. These systems are used in chatbots, virtual assistants, and customer service automation. The focus is on maintaining a coherent and contextually appropriate conversation flow.
Step 2: Collect Data
Once the task is defined, the next crucial step is to collect relevant data. The quality and quantity of the data you gather will significantly impact the performance of your fine-tuned model. Here are some detailed methods to collect the necessary data:
Public Datasets: Utilize datasets that are freely available on various platforms. Websites like Kaggle, Google Dataset Search, or the Hugging Face Hub offer a wide range of datasets tailored to different tasks. These datasets are often well-structured and come with predefined labels, making them an excellent starting point for training your model.
Web Scraping: If public datasets do not meet your requirements, you can collect data directly from the web. This involves extracting information from websites, forums, or social media platforms. It is essential to ensure that your web scraping activities comply with legal and ethical guidelines, including respecting the terms of service of the websites and protecting user privacy.
Manual Annotation: In some cases, you might need highly specific data that is not readily available. Here, manual annotation comes into play. This process involves creating or refining datasets by manually labeling text according to your task's requirements. Although time-consuming, manual annotation ensures high-quality, task-specific data. You can use tools like Labelbox, Prodigy, or even simple spreadsheets to facilitate this process.
By combining these methods, you can build a comprehensive and high-quality dataset that will serve as a solid foundation for fine-tuning your model.
Step 3: Clean and Preprocess Data
Ensuring the quality of your data is crucial for effective fine-tuning of your model. High-quality data leads to better performance and more accurate results. The preprocessing steps you undertake can significantly impact the overall effectiveness of your model. Here are some detailed steps you can follow to clean and preprocess your data:
Removing Duplicates: Ensure that each data point in your dataset is unique. Duplicate entries can skew your model's learning process, leading to biased or inaccurate results. Use tools and scripts to identify and eliminate any repeated data points.
Filtering: Not all data you collect will be relevant or useful for your specific task. Filtering involves removing any irrelevant or noisy data that could negatively impact your model's performance. This step might include eliminating outliers, irrelevant text, or data points that do not meet your criteria.
Handling Missing Values: Missing data can pose a significant problem. You need to decide how to handle these gaps. Options include removing data points with missing values, filling in missing values with a placeholder, or using algorithms to estimate and replace missing data.
By meticulously cleaning and preprocessing your data, you set a solid foundation for the fine-tuning process. Each of these steps contributes to improving the quality and relevance of your dataset, ultimately leading to a more robust and accurate model.
Step 4: Create a Prompt Template
LLMs require data in specific formats to function effectively. To help you get started, we provide detailed chat template examples for three popular language models: Llama 3 and Google Gemma.
Llama 3 Prompt Template Example
<|begin_of_text|><|start_header_id|>system<|end_header_id|>
{{ system_prompt }}<|eot_id|><|start_header_id|>user<|end_header_id|>
{{ user_message }}<|eot_id|><|start_header_id|>assistant<|end_header_id|>
Google Gemma Prompt Template Example
<start_of_turn>user> What is the capital of France? <end_of_turn>
<start_of_turn>model> The capital of France is Paris. <end_of_turn>
Step 5: Format Data into Chat Template
Below is an example of Python code that demonstrates how to format your data into the Llama 3 chat template. This code snippet not only formats the data but also shows how to push it to a hub for further use. By following this example, you can ensure that your data is in the correct format required by the Llama 3 model, which will help in achieving better performance and accuracy.
import json
from huggingface_hub import HfApi, HfFolder
# Define the data to be formatted
data = {
"system_prompt": "You are a helpful assistant.",
"user_message": "What is the weather like today?"
}
# Format the data into the Llama 3 chat template
formatted_data = f"system\n{data['system_prompt']}user\n{data['user_message']}assistant"
# Print the formatted data
print("Formatted Data:\n", formatted_data)
# Convert the formatted data to JSON
json_data = json.dumps({"formatted_data": formatted_data})
# Save the formatted data to a local file
file_path = "formatted_data.json"
with open(file_path, "w") as f:
f.write(json_data)
# Function to push data to the Hugging Face Hub
def push_to_hub(repo_id, file_path, commit_message="Add formatted data"):
api = HfApi()
user = HfFolder.get_token()
api.upload_file(
path_or_fileobj=file_path,
path_in_repo="formatted_data.json",
repo_id=repo_id,
commit_message=commit_message
)
# Example repository ID on Hugging Face Hub
repo_id = "username/repository_name"
# Push the formatted data to the Hugging Face Hub
push_to_hub(repo_id, file_path)
Step 6: Fine-Tune Your LLM
With your dataset prepared and formatted, you can now fine-tune your chosen LLM using libraries such as Hugging Face's transformers
. Here is a basic example:
pythonCopy codefrom transformers import AutoModelForCausalLM, AutoTokenizer, Trainer, TrainingArguments
# Load model and tokenizer
model_name = "llama-3"
model = AutoModelForCausalLM.from_pretrained(model_name)
tokenizer = AutoTokenizer.from_pretrained(model_name)
# Load dataset
from datasets import load_dataset
dataset = load_dataset('json', data_files='llama_chat_data.json')
# Training arguments
training_args = TrainingArguments(
output_dir='./results',
num_train_epochs=3,
per_device_train_batch_size=4,
per_device_eval_batch_size=4,
warmup_steps=500,
weight_decay=0.01,
logging_dir='./logs',
)
# Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=dataset['train'],
eval_dataset=dataset['validation']
)
# Train the model
trainer.train()
Conclusion
In conclusion, fine-tuning a language model can improve its performance for specific tasks. Follow the steps to prepare your dataset, format it, and push it to the Hugging Face Hub. Then, use libraries like Hugging Face's transformers to load your model and tokenizer, set up training arguments, and start training.
Fine-tuning involves loading your dataset, configuring training parameters, and using the Trainer class to manage training. The example shows how to fine-tune a model with a JSON dataset, specifying key parameters like epochs, batch size, and learning rate.
By setting up your training environment and monitoring the process, you can adapt pre-trained models to your needs, making them more effective for your applications. The quality of your dataset and the precision of your training setup are crucial. With practice, you can leverage language models to create powerful, customized solutions.
FAQs
Q1: Why is it important to clean and preprocess data before fine-tuning? Cleaning and preprocessing data ensures that the dataset is free from noise and inconsistencies, which can negatively impact the model's performance.
Q2: Can I use multiple datasets for fine-tuning? Yes, combining multiple datasets can be beneficial, especially if they are relevant to the task. However, ensure that the data is consistently formatted and preprocessed.
Q3: How much data do I need for fine-tuning? The amount of data required depends on the complexity of the task and the model size. Generally, more data leads to better performance, but even smaller datasets can be effective if they are of high quality.
Q4: What are prompt templates, and why are they necessary? Prompt templates structure the input and output data in a format that the model can understand. They are necessary to ensure that the model receives data in a consistent and expected format.
Q5: How can I validate the quality of my fine-tuned model? Validation can be done using a separate validation set or cross-validation. Metrics such as accuracy, F1 score, and perplexity can be used to evaluate performance.
Building a high-quality dataset and properly fine-tuning your LLM can significantly improve its performance on specific tasks. By following this guide, you should be well-equipped to create, format, and utilize datasets for fine-tuning various language models.
Subscribe to my newsletter
Read articles from Sunil Ghanchi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
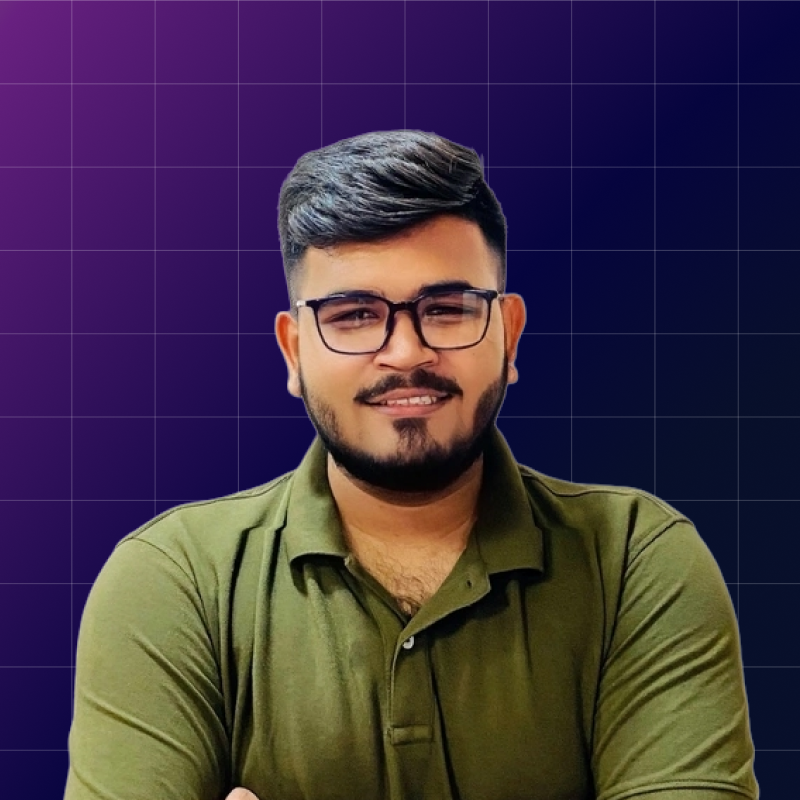
Sunil Ghanchi
Sunil Ghanchi
๐ AI/ML Engineer | Passionate about NLP and RAG ๐ Currently specializing in Natural Language Processing (NLP) and Retrieval-Augmented Generation (RAG) techniques, utilizing vector databases to enhance information retrieval and generation capabilities. ๐ก Committed to staying at the forefront of AI technology, adept at quickly adapting to emerging tools and techniques. ๐ Let's connect to explore opportunities in the exciting world of AI and machine learning!