How to Master Linux for DevOps: A Beginner's Handbook
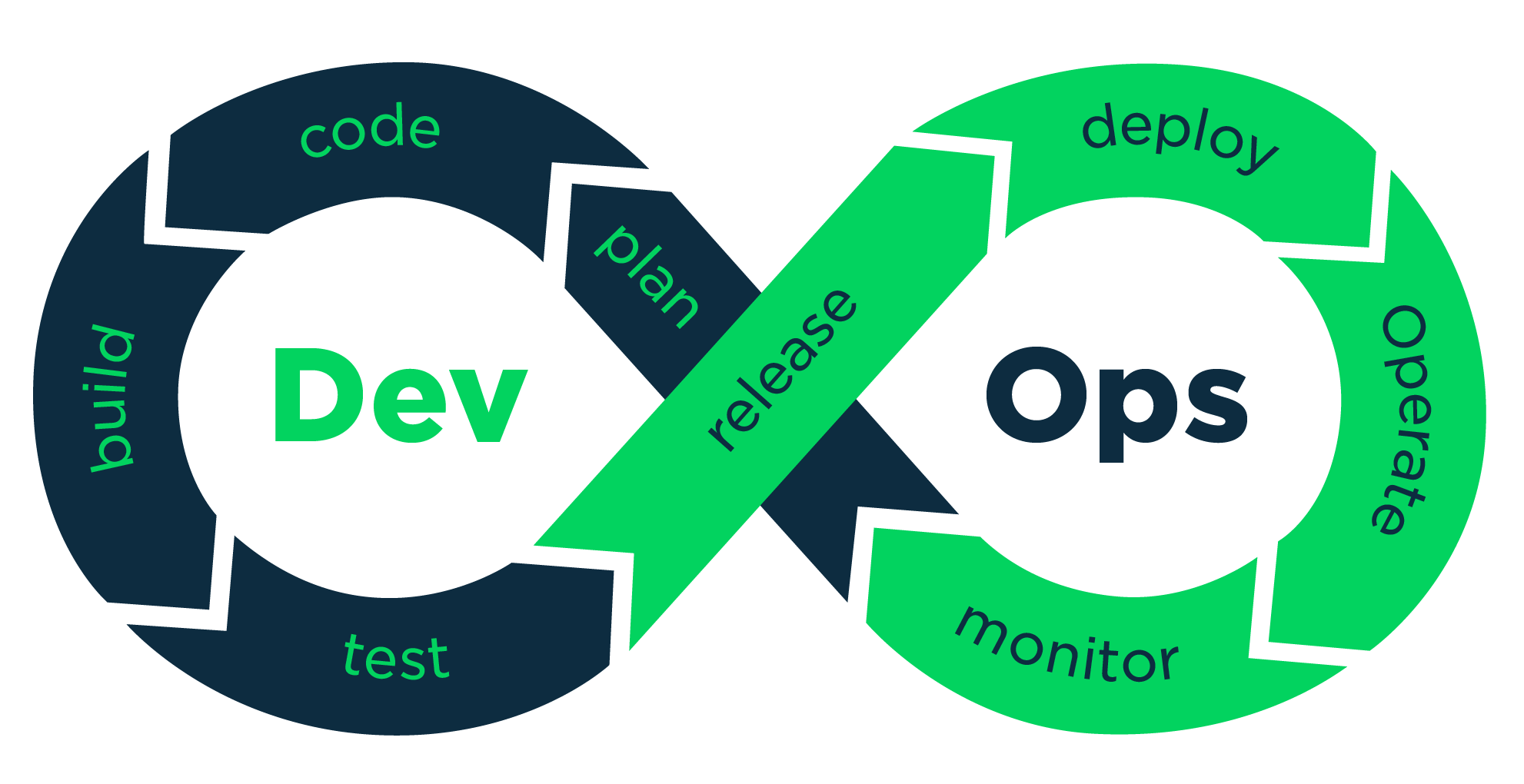
Table of contents
- Introduction
- Understanding the Linux File System
- Basic Commands: Your DevOps Toolkit
- Text Processing: Manipulating Data
- Networking: Connecting the Dots
- Package Management: Installing and Updating Software
- System Monitoring and Performance
- Shell Scripting: Automating Tasks
- Handling User Permissions and Security
- Wrapping Up
Introduction
Welcome to the world of DevOps! If you're just starting out, you've probably realized that Linux is a crucial skill in your toolkit. But why is Linux so important in DevOps? Well, it's the backbone of most cloud infrastructure, offers powerful automation capabilities, and provides the flexibility and control that DevOps practices demand.
In this guide, we'll walk you through the essential Linux skills you need to thrive in DevOps. By the end of this article, you'll have a solid foundation in:
Navigating the Linux file system
Using basic and advanced command-line tools
Managing packages and updates
Monitoring system performance
Writing shell scripts for automation
Handling user permissions and security
So, let's dive in and start your journey to becoming a Linux-savvy DevOps engineer!
Understanding the Linux File System
Before we jump into commands, let's talk about how Linux organizes files. Unlike Windows with its drive letters, Linux has a single root directory (/
) with everything branching off from there. Here's a quick rundown of some important directories:
/home
: Where user files live/etc
: System configuration files/var
: Variable data like logs/tmp
: Temporary files/usr
: User binaries and program files
Think of it like a big tree with branches - once you get the hang of it, it's actually pretty intuitive.
Absolute vs. Relative Paths
In Linux, you can specify file locations using either absolute or relative paths:
Absolute paths start from the root directory and begin with a
/
. For example:/home/username/Documents/file.txt
Relative paths start from your current location. For example, if you're in
/home/username
, you can refer to the same file asDocuments/file.txt
Let's see this in action:
$ pwd
/home/username
$ cd /etc # Using an absolute path
$ pwd
/etc
$ cd ../home/username/Documents # Using a relative path
$ pwd
/home/username/Documents
Basic Commands: Your DevOps Toolkit
Navigating the File System
These are the commands you'll use constantly:
pwd
: Print Working Directory. It's like asking "Where am I?"ls
: List files and directories. Add-l
for more details,-h
for human-readable sizes, or-a
to see hidden files.cd
: Change Directory. Usecd ..
to go up one level.
For example:
$ pwd
/home/username
$ ls -lh
total 4.0K
drwxr-xr-x 2 username username 4.0K Jul 4 10:00 Documents
drwxr-xr-x 2 username username 4.0K Jul 4 10:00 Downloads
$ cd Documents
$ pwd
/home/username/Documents
$ ls -a
. .. .hidden_file visible_file.txt
File Operations
These commands let you manipulate files and directories:
cp
: Copy files or directoriesmv
: Move or rename filesrm
: Remove files (be careful with this one!)touch
: Create an empty filemkdir
: Make a new directoryrmdir
: Remove an empty directory
Let's try them out:
$ touch myfile.txt
$ mkdir mydir
$ mv myfile.txt mydir/
$ cp mydir/myfile.txt mydir/myfile_backup.txt
$ ls mydir
myfile.txt myfile_backup.txt
$ rm mydir/myfile.txt
$ ls mydir
myfile_backup.txt
Warning: Be extremely cautious with rm -rf
. This command recursively removes files and directories without prompting, and it can be destructive if used incorrectly. Always double-check your command before using it, especially with sudo privileges.
Using Wildcards
Wildcards are powerful tools for working with multiple files. The most common wildcards are:
*
: Matches any number of characters?
: Matches any single character
For example:
$ ls *.txt # List all .txt files
$ rm test_*.log # Remove all files starting with "test_" and ending with ".log"
Viewing File Contents
When you need to peek inside files:
cat
: Display the entire fileless
ormore
: View files page by page (press 'q' to quit)head
: Show the first few lines of a filetail
: Show the last few lines (great for log files!)
For instance:
$ cat /etc/hostname
myserver
$ head -n 3 /etc/passwd
root:x:0:0:root:/root:/bin/bash
daemon:x:1:1:daemon:/usr/sbin:/usr/sbin/nologin
bin:x:2:2:bin:/bin:/usr/sbin/nologin
$ tail -n 5 /var/log/syslog
Jul 4 15:30:01 myserver CRON[12345]: (root) CMD (command_to_run)
Jul 4 15:35:01 myserver CRON[12346]: (root) CMD (command_to_run)
...
$ less /var/log/syslog # Interactive viewing of large files
The -f
option in tail
lets you watch the file in real-time, which is super useful for monitoring log files:
$ tail -f /var/log/syslog
Text Processing: Manipulating Data
In DevOps, you'll often need to search through and manipulate text files. These tools are lifesavers:
grep: Searching for Patterns
grep
is used to search for specific patterns in files or output:
# Find all ERROR messages in a log file
$ grep ERROR /var/log/myapp.log
2023-07-04 15:45:23 ERROR Failed to connect to database
# Search for a pattern case-insensitively
$ grep -i error /var/log/myapp.log
# Show line numbers in the output
$ grep -n ERROR /var/log/myapp.log
sed: Stream Editor for Text Manipulation
sed
is used for finding, replacing, or deleting patterns in text:
# Replace 'foo' with 'bar' in a file
$ sed 's/foo/bar/g' myfile.txt > myfile_updated.txt
# Delete lines containing a specific pattern
$ sed '/pattern_to_remove/d' input.txt > output.txt
awk: Pattern Scanning and Processing
awk
is powerful for processing structured text data:
# Sum up the total bytes sent by each IP in an Apache access log
$ awk '{print $1,$10}' access.log | sort | uniq -c | sort -rn | head -10
1500 192.168.1.100 15000000
1200 192.168.1.101 12000000
...
# Print specific fields from a CSV file
$ awk -F',' '{print $1, $3}' data.csv
Additional Text Processing Tools
cut
: Extract specific columns from filessort
: Sort lines of textuniq
: Report or filter out repeated lines in a file
# Extract the third column from a CSV file
$ cut -d',' -f3 data.csv
# Sort a file numerically
$ sort -n numbers.txt
# Count unique occurrences of lines
$ sort myfile.txt | uniq -c
Networking: Connecting the Dots
Networking is crucial in DevOps. Here are some commands you'll use often:
ping
: Check if a host is reachableifconfig
orip
: View or configure network interfacesnetstat
: Network statisticstraceroute
: Trace the route to a hostnslookup
ordig
: DNS lookup
For example:
$ ping -c 4 google.com
PING google.com (172.217.16.142) 56(84) bytes of data.
64 bytes from lhr25s10-in-f14.1e100.net (172.217.16.142): icmp_seq=1 ttl=57 time=7.84 ms
...
$ ip addr show
1: lo: <LOOPBACK,UP,LOWER_UP> mtu 65536 qdisc noqueue state UNKNOWN group default qlen 1000
link/loopback 00:00:00:00:00:00 brd 00:00:00:00:00:00
inet 127.0.0.1/8 scope host lo
valid_lft forever preferred_lft forever
2: eth0: <BROADCAST,MULTICAST,UP,LOWER_UP> mtu 1500 qdisc fq_codel state UP group default qlen 1000
link/ether 00:15:5d:01:ca:05 brd ff:ff:ff:ff:ff:ff
inet 172.17.210.228/20 brd 172.17.223.255 scope global eth0
valid_lft forever preferred_lft forever
$ netstat -tuln # Show active connections
Active Internet connections (only servers)
Proto Recv-Q Send-Q Local Address Foreign Address State
tcp 0 0 0.0.0.0:22 0.0.0.0:* LISTEN
tcp6 0 0 :::80 :::* LISTEN
Using curl and wget
curl
and wget
are useful for making network requests and downloading files:
# Make a GET request to a URL
$ curl https://api.example.com/data
# Download a file
$ wget https://example.com/file.zip
Basic Network Troubleshooting with netcat (nc)
netcat
is a versatile networking utility:
# Test if a port is open
$ nc -zv example.com 80
# Create a simple chat server
$ nc -l 1234 # On the server
$ nc server_ip 1234 # On the client
Package Management: Installing and Updating Software
Different Linux distributions use different package managers. Here's a quick overview:
Debian-based Systems (Ubuntu, Debian)
On Ubuntu and Debian-based systems, you'll use apt
:
$ sudo apt update # Update package list
$ sudo apt install nginx # Install Nginx web server
$ sudo apt upgrade # Upgrade all packages
Red Hat-based Systems (CentOS, Fedora)
On Red Hat-based systems, you'll use yum
or dnf
:
$ sudo yum update
$ sudo yum install httpd # Install Apache web server
Other Package Managers
Arch Linux:
pacman
openSUSE:
zypper
Keeping Your System Updated
Regularly updating your system is crucial for security and stability:
# On Ubuntu/Debian
$ sudo apt update && sudo apt upgrade
# On CentOS/Fedora
$ sudo yum update
System Monitoring and Performance
Keeping an eye on your system's health is a big part of DevOps. Here are some tools:
top and htop
top
provides a real-time view of system processes:
$ top
top - 15:50:36 up 5:11, 1 user, load average: 0.00, 0.01, 0.05
Tasks: 102 total, 1 running, 101 sleeping, 0 stopped, 0 zombie
%Cpu(s): 0.0 us, 0.3 sy, 0.0 ni, 99.7 id, 0.0 wa, 0.0 hi, 0.0 si, 0.0 st
MiB Mem : 3919.1 total, 2339.5 free, 801.5 used, 778.1 buff/cache
MiB Swap: 0.0 total, 0.0 free, 0.0 used. 2861.5 avail Mem
PID USER PR NI VIRT RES SHR S %CPU %MEM TIME+ COMMAND
1 root 20 0 169880 11972 8480 S 0.0 0.3 0:02.41 systemd
2 root 20 0 0 0 0 S 0.0 0.0 0:00.00 kthreadd
...
htop
is an interactive process viewer with a more user-friendly interface:
$ htop
Disk and Memory Usage
Check disk space usage with df
:
$ df -h # The -h option makes the output human-readable
Filesystem Size Used Avail Use% Mounted on
/dev/sda1 20G 5.2G 14G 28% /
/dev/sda2 100G 24G 77G 24% /home
See how much space directories are taking up with du
:
$ du -sh /var/log/* # See how much space log files are taking up
4.0K /var/log/alternatives.log
4.0K /var/log/apt
4.0K /var/log/auth.log
...
Check memory usage with free
:
$ free -m # Show memory usage in megabytes
total used free shared buff/cache available
Mem: 3919 801 2339 16 778 2861
Swap: 0 0 0
Advanced Monitoring Tools
For more detailed system monitoring, you can use:
iostat
: Report CPU statistics and I/O statistics for devices and partitionsvmstat
: Report virtual memory statistics
$ iostat
Linux 5.4.0-42-generic (username) 07/04/2023 _x86_64_ (4 CPU)
avg-cpu: %user %nice %system %iowait %steal %idle
0.50 0.00 0.23 0.02 0.00 99.25
Device tps kB_read/s kB_wrtn/s kB_dscd/s kB_read kB_wrtn kB_dscd
sda 0.47 7.31 2.29 0.00 283621 88896 0
sdb 0.01 0.11 0.00 0.00 4136 0 0
$ vmstat
procs -----------memory---------- ---swap-- -----io---- -system-- ------cpu-----
r b swpd free buff cache si so bi bo in cs us sy id wa st
1 0 0 2339500 77816 700304 0 0 5 1 52 92 0 0 99 0 0
Shell Scripting: Automating Tasks
Shell scripting is where Linux really shines for DevOps. Here's a more advanced script that demonstrates loops, conditionals, and error handling:
#!/bin/bash
# Script to backup multiple directories and clean up old backups
# Define variables
BACKUP_DIRS=("/path/to/dir1" "/path/to/dir2" "/path/to/dir3")
BACKUP_DEST="/path/to/backups"
MAX_BACKUPS=5
DATETIME=$(date +"%Y%m%d_%H%M%S")
# Function to log messages
log_message() {
echo "[$(date +'%Y-%m-%d %H:%M:%S')] $1"
}
# Create backup destination if it doesn't exist
mkdir -p "$BACKUP_DEST"
# Loop through each directory and create a backup
for dir in "${BACKUP_DIRS[@]}"; do
if [ ! -d "$dir" ]; then
log_message "Warning: Directory $dir does not exist. Skipping."
continue
fi
backup_file="$BACKUP_DEST/$(basename "$dir")_$DATETIME.tar.gz"
log_message "Creating backup of $dir at $backup_file"
tar -czf "$backup_file" "$dir"
if [ $? -eq 0 ]; then
log_message "Backup of $dir completed successfully."
else
log_message "Error: Backup of $dir failed."
fi
done
# Remove old backups
log_message "Cleaning up old backups, keeping only the latest $MAX_BACKUPS backups."
find "$BACKUP_DEST" -type f -name "*.tar.gz" | sort -r | sed -e "1,${MAX_BACKUPS}d" | xargs -d '\n' rm -f
log_message "Backup and cleanup process completed."
Save this script as backup.sh
, make it executable (chmod +x
backup.sh
), and run it periodically using cron to automate backups and cleanup.
Handling User Permissions and Security
Managing Users and Groups
In Linux, managing users and groups is essential for maintaining security and access control:
adduser
: Add a new userpasswd
: Change a user's passwordusermod
: Modify a user accountdeluser
: Delete a usergroupadd
: Add a new groupusermod -aG
: Add a user to a group
For example
# Add a new user
$ sudo adduser newuser
# Change a user's password
$ sudo passwd newuser
# Add a user to the sudo group
$ sudo usermod -aG sudo newuser
# Delete a user
$ sudo deluser newuser
# Create a new group
$ sudo groupadd devops
File Permissions and Ownership
Linux file permissions determine who can read, write, and execute files. Use chmod
to change permissions and chown
to change ownership:
r
(read): 4w
(write): 2x
(execute): 1
For example:
# Change file permissions
$ chmod 755 myfile.sh # Owner can read/write/execute, others can read/execute
# Change file ownership
$ sudo chown newuser:newgroup myfile.sh
Using sudo
sudo
allows permitted users to execute commands as the superuser. Manage sudo
privileges by editing the /etc/sudoers
file using visudo
:
$ sudo visudo
Add a line to grant a user sudo
privileges:
newuser ALL=(ALL) NOPASSWD:ALL
SSH for Secure Remote Access
SSH (Secure Shell) is used for secure remote access to servers. Basic SSH commands include:
ssh
: Connect to a remote serverscp
: Securely copy files between serversssh-keygen
: Generate SSH key pairsssh-copy-id
: Install SSH keys on a remote server
For example:
# Connect to a remote server
$ ssh user@remote_host
# Copy a file to a remote server
$ scp myfile.txt user@remote_host:/path/to/destination
# Generate an SSH key pair
$ ssh-keygen -t rsa -b 4096
# Copy the public key to a remote server
$ ssh-copy-id user@remote_host
Firewall Configuration with ufw
ufw
(Uncomplicated Firewall) is a frontend for managing firewall rules:
# Enable the firewall
$ sudo ufw enable
# Allow SSH connections
$ sudo ufw allow ssh
# Deny all incoming traffic and allow all outgoing traffic
$ sudo ufw default deny incoming
$ sudo ufw default allow outgoing
# Check the firewall status
$ sudo ufw status
Wrapping Up
Whew! We've covered a lot of ground here. Remember, becoming proficient with Linux takes time and practice. Don't get discouraged if it feels overwhelming at first - we've all been there.
My advice? Set up a virtual machine or use a cloud provider's free tier to create a Linux sandbox. Play around, break things (intentionally!), and learn from your mistakes. That's how I learned, and it's still how I pick up new skills today.
Keep exploring, stay curious, and before you know it, you'll be navigating Linux like a pro. Happy DevOps-ing!
Subscribe to my newsletter
Read articles from vikash kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
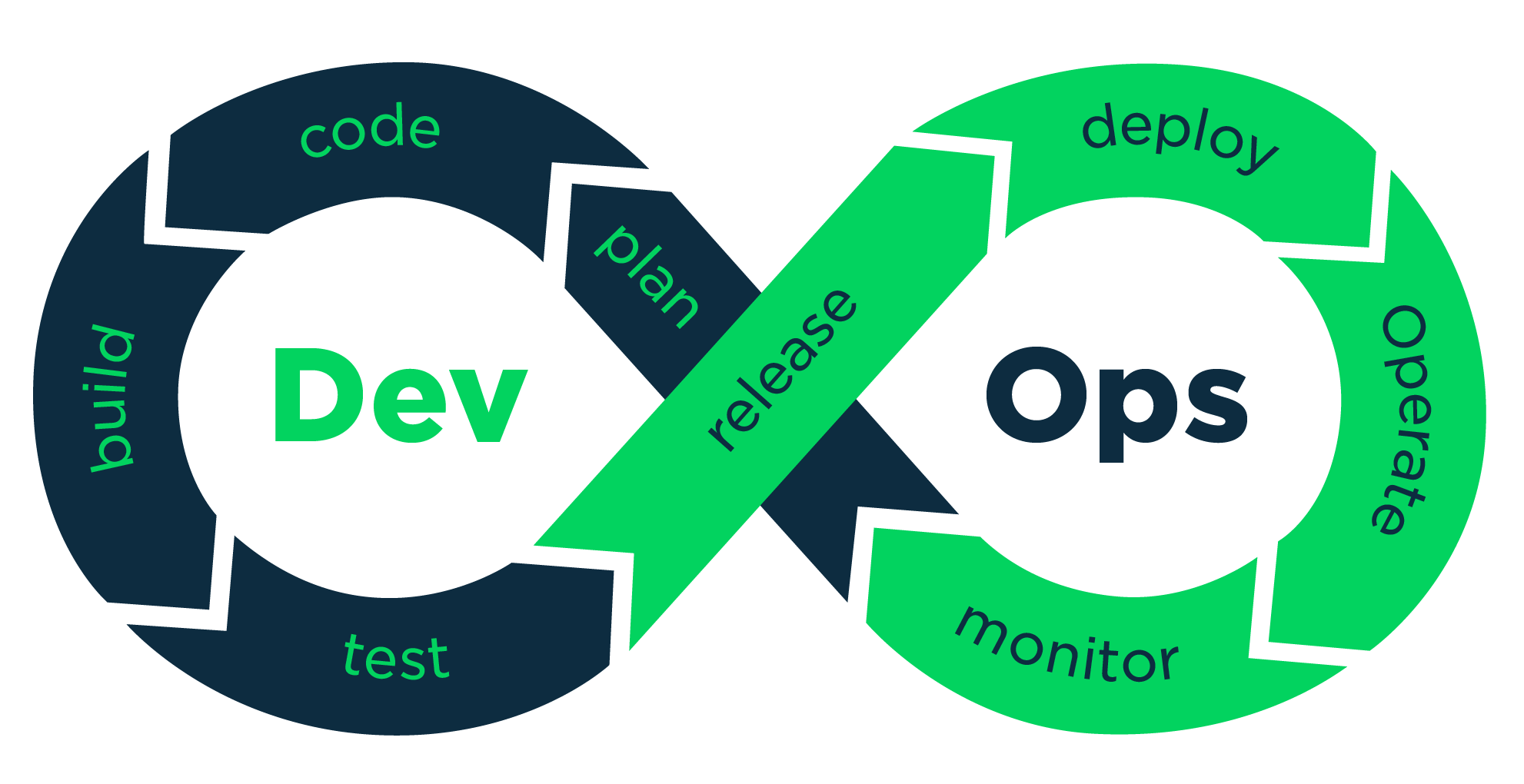
vikash kumar
vikash kumar
Hey folks! ๐ I'm Vikash Kumar, a seasoned DevOps Engineer navigating the thrilling landscapes of DevOps and Cloud โ๏ธ. My passion? Simplifying and automating processes to enhance our tech experiences. By day, I'm a Terraform wizard; by night, a Kubernetes aficionado crafting ingenious solutions with the latest DevOps methodologies ๐. From troubleshooting deployment snags to orchestrating seamless CI/CD pipelines, I've got your back. Fluent in scripts and infrastructure as code. With AWS โ๏ธ expertise, I'm your go-to guide in the cloud. And when it comes to monitoring and observability ๐, Prometheus and Grafana are my trusty allies. In the realm of source code management, I'm at ease with GitLab, Bitbucket, and Git. Eager to stay ahead of the curve ๐, I'm committed to exploring the ever-evolving domains of DevOps and Cloud. Let's connect and embark on this journey together! Drop me a line at thenameisvikash@gmail.com.