How to Use File Permissions and Access Control Lists. #Day-6

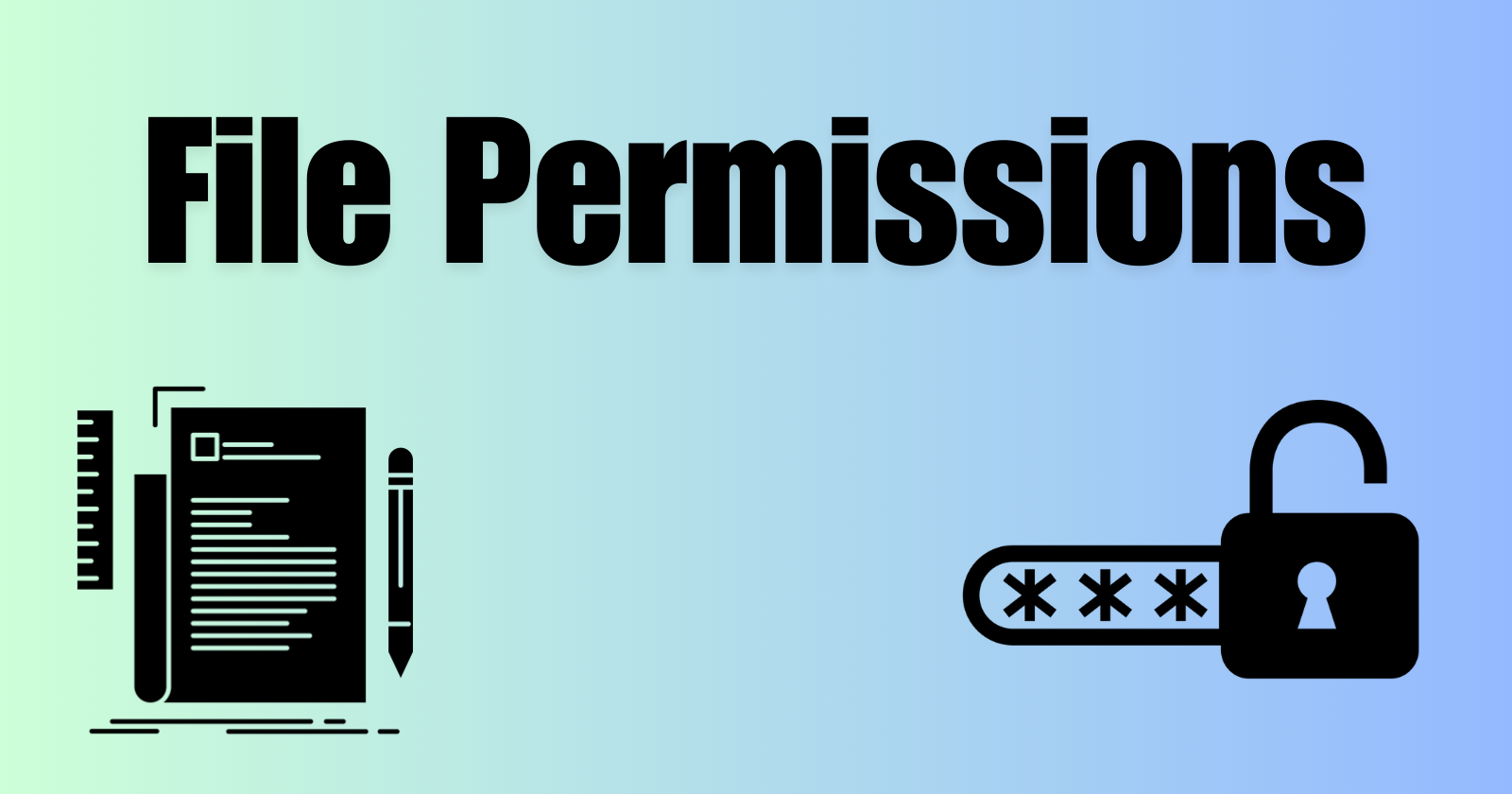
Introduction
File permissions are crucial in Unix/Linux systems for securing files and directories. They define who can read, write, and execute a file. Let's dive into the world of file permissions!
Types of File Permissions
In Unix/Linux systems, there are three types of permissions:
Read (r): Allows viewing the contents of the file.
Write (w): Allows modifying the contents of the file.
Execute (x): Allows running the file as a program.
Read (R) | Write (W) | Execute (X) |
4 | 2 | 1 |
Permission Categories
Permissions are divided into three categories:
Owner/User (u): The person who owns the file.
Group (g): A set of users who share the same permissions.
Others (o): Everyone else.
Viewing File Permissions
To view file permissions, use the ls -l
command. Here's an example output:
-rwxr-xr--
This output shows:
-
File type (regular file in this case)rwx
Owner permissions (read, write, execute)r-x
Group permissions (read, execute)r--
Other permissions (read only)
Changing File Permissions
To change file permissions, use the chmod
command.
Grant execute permission to the owner
chmod u+x filename
Remove write permission from others
chmod o-w filename
Set read, write, and execute permissions for everyone
chmod 777 filename
Numerical Representation
File permissions can also be represented numerically:
4
Read (r)2
Write (w)1
Execute (x)
Combine these numbers to set permissions.
755 (rwxr-xr-x):
chmod 755 filename
Permission for Folder and files both:
chmod -R 777 folder/
Permission for Folder only:
chmod 777 folder
Null Permission:
chmod 000 folder/
Special Permissions
Sticky bit: Ensures that only the file owner can delete or rename files in a directory.
To set the sticky bit on a directory:
chmod +t /path/to/directory
To remove the sticky bit:
chmod -t /path/to/directory
chown
to change the ownership permission of a file or directory.
Change owner of file:
chown user file.txt
Change Owner of Folder:
chown -R user folder/
Change Owner and Group of file:
chown user:group file.txt
Alternatively, you can use the numerical representation. The sticky bit is represented by the number 1
in the thousands place:
chmod 1777 /path/to/directory
In this example, 1777
sets the sticky bit and gives read, write, and execute permissions to everyone.
chgrp
to change the group permission of a file or directory.
Change only group of file:
chgrp group file.txt
Change group for Folder and files:
chgrp -R group folder/
Access Control Lists (ACL)
The getfacl
command is used to display the ACLs of a file or directory.
getfacl example.txt
Result:
# file: example.txt
# owner: user
# group: group
user::rw-
user:anotheruser:r--
group::r--
mask::r--
other::r--
The setfacl
command is used to set ACLs for a file or directory.
setfacl -m u:username:permissions /path/to/file_or_directory
-m
: Modify the ACL.u:username:permissions
: Set permissions for a user.
setfacl -m u:anotheruser:rw example.txt
To remove specific ACL entries, use the -x
option:
setfacl -x u:anotheruser example.txt
Remove All ACLs:
setfacl -b example.txt
SUID (Set User ID)
Significance: When an executable file has the SUID bit set, it runs with the privileges of the file owner, rather than the user who runs the file. This is useful for programs that need to perform tasks that require higher privileges.
Example:
- Create a script that prints the current user and set the SUID bit:
echo -e '#!/bin/bash\necho "Current user: $(whoami)"' > /tmp/suid_test.sh
chmod 4755 /tmp/suid_test.sh
chown root /tmp/suid_test.sh
4
in4755
sets the SUID bit.7
gives read, write, and execute permissions to the owner (root).5
gives read and execute permissions to group and others.
Run the script as a non-root user:
/tmp/suid_test.sh
Output
Current user: root
The script runs with root privileges because of the SUID bit.
SGID (Set Group ID)
Significance: When the SGID bit is set on a directory, files created within the directory inherit the group ownership of the directory, not the creating user’s primary group. When set on an executable, the process runs with the group ID of the file's group.
Example:
- Create a directory and apply the SGID bit:
mkdir /tmp/sgid_test
chmod 2775 /tmp/sgid_test
chgrp somegroup /tmp/sgid_test
2
in2775
sets the SGID bit.7
gives read, write, and execute permissions to the owner.7
gives read, write, and execute permissions to the group.5
gives read and execute permissions to others.
Create a file within the directory as a non-group member:
touch /tmp/sgid_test/testfile
ls -l /tmp/sgid_test
Output
-rw-r--r-- 1 user somegroup 0 Jul 4 12:05 testfile
The file testfile
has the group somegroup
instead of the user's primary group.
These examples illustrate how the sticky bit, SUID, and SGID can control access and permissions to enhance security and functionality in a multi-user environment.
Create a script that backs up the current permissions of files in a directory to a file.
#!/bin/bash
# Check if a directory is provided
if [ -z "$1" ]; then
echo "Usage: $0 <directory>"
exit 1
fi
DIRECTORY=$1
BACKUP_FILE="permissions_backup_$(date +%Y%m%d_%H%M%S).txt"
# Check if the directory exists
if [ ! -d "$DIRECTORY" ]; then
echo "Directory $DIRECTORY does not exist."
exit 1
fi
# Create or overwrite the backup file
echo "Backing up permissions for directory: $DIRECTORY" > $BACKUP_FILE
# List the files and their permissions
find "$DIRECTORY" -type f -exec stat --format '%A %U %G %n' {} \; >> $BACKUP_FILE
echo "Backup completed. Permissions saved to $BACKUP_FILE"
Explanation
if [ -z "$1" ]; then
: Checks if a directory argument is provided.
DIRECTORY=$1
: Assigns the first argument to theDIRECTORY
variable.
BACKUP_FILE="permissions_backup_$(date +%Y%m%d_%H%M%S).txt"
: Creates a unique backup file name based on the current date and time.
if [ ! -d "$DIRECTORY" ]; then
: Checks if the provided directory exists.
echo "Backing up permissions for directory: $DIRECTORY" > $BACKUP_FILE
: Writes a header to the backup file.
find "$DIRECTORY" -type f -exec stat --format '%A %U %G %n' {} \; >> $BACKUP_FILE
: Usesfind
to locate all files in the directory andstat
to get the permissions, owner, group, and file name. This information is appended to the backup file.
echo "Backup completed. Permissions saved to $BACKUP_FILE"
: Prints a completion message.This script ensures that the current permissions of all files in the specified directory are backed up to a file, which can be useful for restoring permissions later if needed.
Create another script that restores the permissions from the backup file.
#!/bin/bash
# Check if a backup file is provided
if [ -z "$1" ]; then
echo "Usage: $0 <backup_file>"
exit 1
fi
BACKUP_FILE=$1
# Check if the backup file exists
if [ ! -f "$BACKUP_FILE" ]; then
echo "Backup file $BACKUP_FILE does not exist."
exit 1
fi
# Read the backup file and restore permissions
while IFS= read -r line
do
# Skip the header line
if [[ "$line" == "Backing up permissions for directory:"* ]]; then
continue
fi
# Extract the permissions, owner, group, and file name
perms=$(echo "$line" | awk '{print $1}')
owner=$(echo "$line" | awk '{print $2}')
group=$(echo "$line" | awk '{print $3}')
file=$(echo "$line" | awk '{print $4}')
# Restore the owner and group
chown "$owner:$group" "$file"
# Restore the permissions
chmod "$perms" "$file"
done < "$BACKUP_FILE"
echo "Restoration completed from $BACKUP_FILE"
Explanation
if [ -z "$1" ]; then
: Checks if a backup file argument is provided.
BACKUP_FILE=$1
: Assigns the first argument to theBACKUP_FILE
variable.
if [ ! -f "$BACKUP_FILE" ]; then
: Checks if the provided backup file exists.
while IFS= read -r line
: Reads each line of the backup file.
if [[ "$line" == "Backing up permissions for directory:"* ]]; then
: Skips the header line.
perms=$(echo "$line" | awk '{print $1}')
: Extracts the permissions from the line.
owner=$(echo "$line" | awk '{print $2}')
: Extracts the owner from the line.
group=$(echo "$line" | awk '{print $3}')
: Extracts the group from the line.
file=$(echo "$line" | awk '{print $4}')
: Extracts the file name from the line.
chown "$owner:$group" "$file"
: Restores the owner and group.
chmod "$perms" "$file"
: Restores the permissions.
done < "$BACKUP_FILE"
: Ends the loop after reading the entire file.
echo "Restoration completed from $BACKUP_FILE"
: Prints a completion message.This script reads the backup file line by line, extracts the permissions, owner, group, and file name, and then restores them to the corresponding files.
Conclusion
Understanding file permissions is essential for managing the security of your files and directories in Unix/Linux systems. With these basics, you're well on your way to mastering file permissions!
Connect and Follow Me On Socials :
Like👍 | Share📲 | Comment💭
Subscribe to my newsletter
Read articles from Nikunj Vaishnav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Nikunj Vaishnav
Nikunj Vaishnav
👋 Hi there! I'm Nikunj Vaishnav, a passionate QA engineer Cloud, and DevOps. I thrive on exploring new technologies and sharing my journey through code. From designing cloud infrastructures to ensuring software quality, I'm deeply involved in CI/CD pipelines, automated testing, and containerization with Docker. I'm always eager to grow in the ever-evolving fields of Software Testing, Cloud and DevOps. My goal is to simplify complex concepts, offer practical tips on automation and testing, and inspire others in the tech community. Let's connect, learn, and build high-quality software together! 📝 Check out my blog for tutorials and insights on cloud infrastructure, QA best practices, and DevOps. Feel free to reach out – I’m always open to discussions, collaborations, and feedback!