Coding a Morning Weather Text

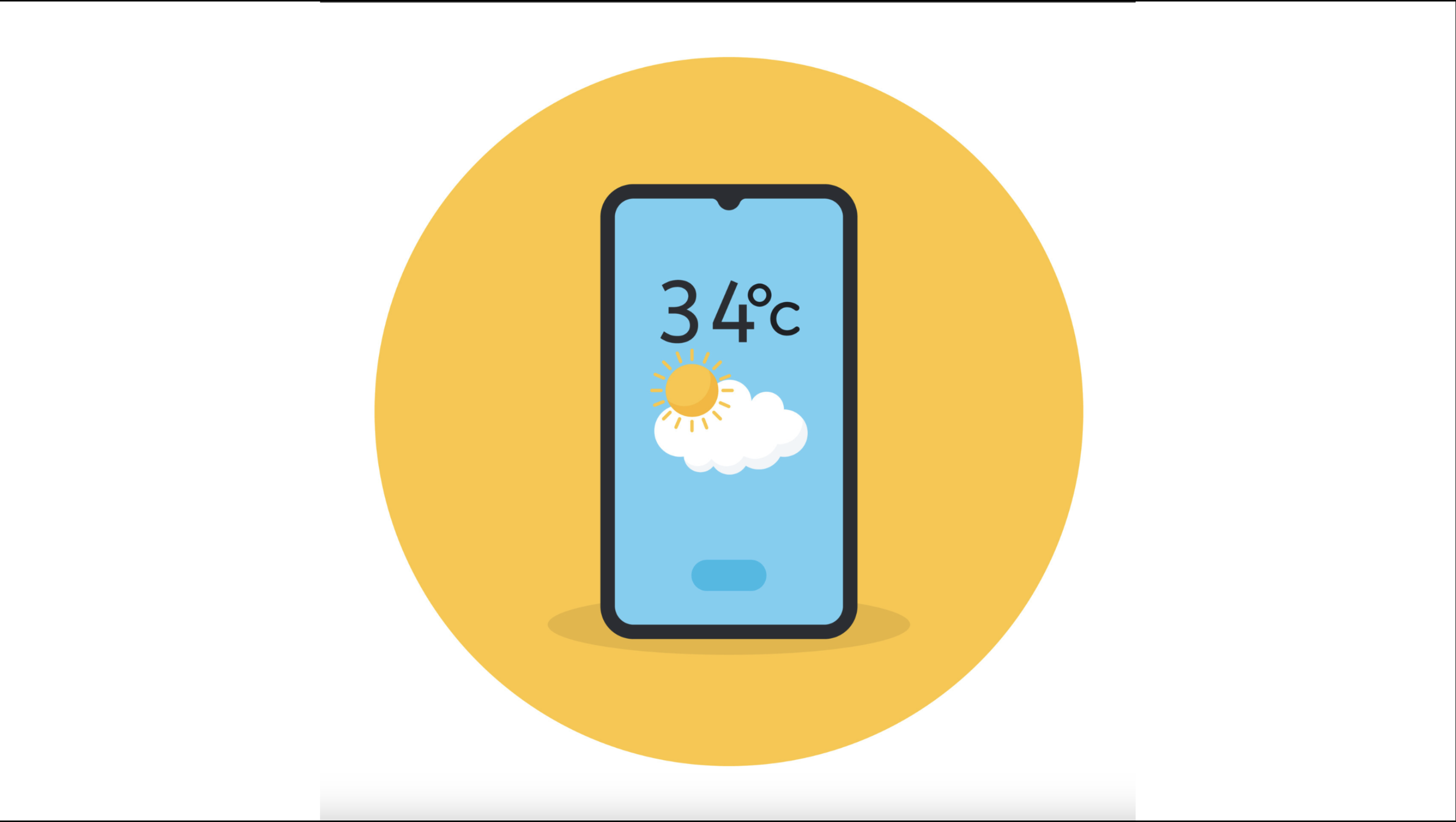
Introduction
I wanted to get started on using python to automate everyday tasks, so for my first automation project I decided to write a program that texts me the weather everyday. I split up this project into 3 big tasks:
Use web scrapers to get the data from the weather website
Send a text with that data using python
Run this code at 6am every day without running the manually
Web Scraping
This project I wanted to look for a different web scraper than what I usually use to see if there were more efficient ways to get data from a website. That's when I came across playwright. Playwright has a unique feature that when you type the this command line in the terminal it opens up a browser and any action you do on the browser would be written in the code in real time.
playwright codegen
Unfortunately, when I clicked on the element that I wanted to scrape playwright auto-coded to find the element by the number. For example if I wanted to scrape the current temperature it would find the element as "100" instead of the actual class or xpath that it was on. Although this would work for that day, the next day when the temperature changes it wouldn't be able to find the element. Eventually, I decided to ditch playwright and stick with selenium and beautiful soup which worked perfectly. However, playwright is an interesting library that I plan on using in the future if I ever need to quickly do monotonous web scraping.
Sending the Text
My initial plan was to use google voice because it was free and easy to use. However, every time I tried to login into google using selenium google blocked the browser. This is because of google's protection software preventing people who are trying to use automated browsers to steal user's information.
My next plan was to use Twilio. There were a lot of youTube tutorials of Twilio saying you get one free trial number. So I made my Twilio account and tried to use the free number. However, in order to get the number verified I needed a business name, website, and location, so that didn't work.
Finally, I stumbled across this reddit post saying that you can send a text using email. If you emailed a number in a specific format it would come through as a text. Here is the reddit post with all the different types of cell phone providers and their format.
So, I used the library smtplib to work out sending a text message. When I used my home email to send the message it would be inconsistent and take a while to send. I assumed this was because I use my home email a lot, so I just created another google account and used that one to send the emails and it worked seamlessly.
Running Everyday
Every time I tried looking for a way to run this code autonomously this solution always came up.
def sendTxt():
print("The weather is...")
Schedule.every().day.at("6:00").do(sendTxt())
While True:
schedule.run_pending()
time.sleep(1)
The main problem with this is I have to keep this code running 24/7 which means I have to constantly have to have a VS code tab open and I can't run new code without stopping this one.
After further research, I found crontab. Crontab is a command you use in the terminal to run scripts at certain time. After playing around with it, here is what I found was the easiest way to use crontab. There are many editors for crontab the easiest one to use is nano here is how you open the nano editor.
export EDITOR=nano
export VISUAL=nano
crontab -e
Then once you open up the crontab viewer you'll need to type in a command to signify when you want the code to run. This is the website that helped me learn the syntax of crontab. https://crontab.guru/
Here is what the final command looks like in the terminal:
When I originally wrote this I kept having the same problem. Every time the crontab would run, it would says "operation not permitted". Solutions online would say to give the terminal full disk access from system preferences, but that didn't work. This part took my hours to figure out, but how to get around this is in System Setting when you give an application permission to full disk access, you have to hold cmd+shift+g to reveal the hidden files. Under those, go to user and give cron full disk access and then it should solve the issue.
So now at 5:55am crontab will run my code which will send me a text of the weather. However, my computer needs to be on during this time, and the whole point of this is to wake up to a weather text. Luckily, there is a simple command to wake your computer at a certain time called pmset, here is the command I used.
sudo pmset repeat wakeorpoweron MTWRFSU 06:00:00
Run this and as long as your laptop isn't calm shut closed, then it will wake up at six am everyday and crontab will execute the code send a text. The reason why I put crontab at 5:55 is because when I put it at 6am crontab takes too long to load and the laptop just shuts off before it can finish the script.
Conclusion
After all that hard work, I can finally sleep soundly without touching my laptop and get a text telling me what the weather is for that day. I really enjoyed this project and feel more comfortable using crontab, so I will be working on automating more tasks. If I were to expand on this project it would mainly be find a way to encrypt my email and password and put that in the code just because having it plainly in the code is a security concern.
Link to Github
Subscribe to my newsletter
Read articles from Adeel Syed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Adeel Syed
Adeel Syed
Hi! My name is Adeel Syed and I am currently a senior at Westlake High School. I am mainly a self-taught coder, but I have taken some java classes at Westlake. Feel free to contact me at adeelsyed2004@gmail.com!