Your First PHP Script: Hello World!

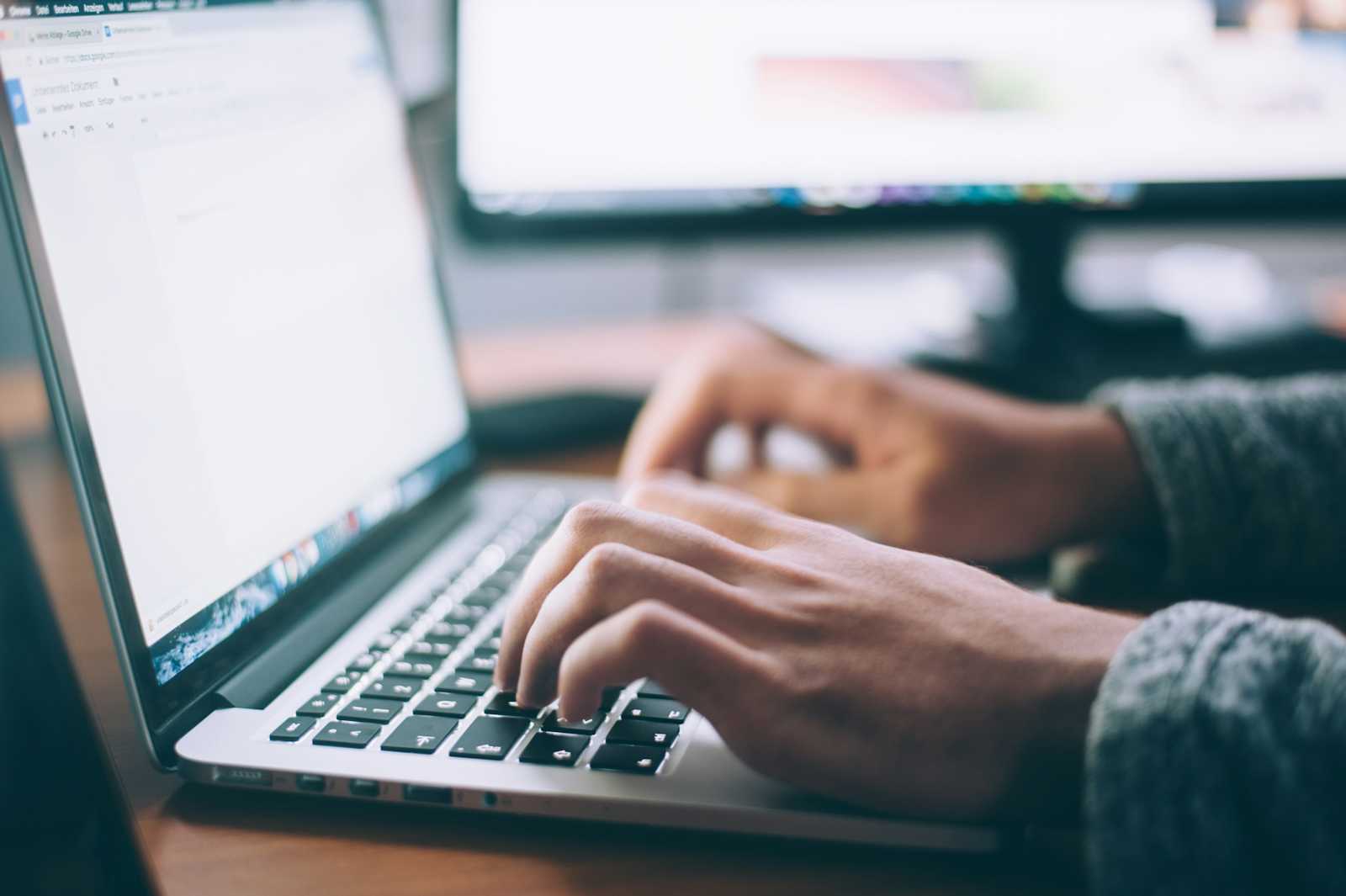
Today, we'll guide you through creating and running your first PHP script. The "Hello World" program is a classic beginner's exercise in many programming languages, and PHP is no exception. Let's dive in!
What is PHP?
PHP (Hypertext Preprocessor) is a popular server-side scripting language designed for web development. It is embedded within HTML and executed on the server, producing dynamic web pages.
Prerequisites
Before we start, make sure you have your PHP development environment set up. If you haven't done this yet, follow our guide from the Installation Blog to install and configure XAMPP.
Writing Your First PHP Script
We'll create a simple PHP file that outputs "Hello World!" to the browser.
1. Create a PHP File:
Open the
htdocs
directory within your XAMPP installation. The default path is:Windows:
C:\xampp\htdocs
macOS:
/Applications/XAMPP/htdocs
Create a new folder named
hello_world
.Inside the
hello_world
folder, create a new file namedindex.php
.
2. Write the PHP Code:
Open
index.php
with your preferred text editor or IDE (like Visual Studio Code, Sublime Text, or Notepad++).Add the following code to
index.php
:
<?php
echo "Hello, World!";
?>
Explanation:
<?php
: This tag starts the PHP code block.echo "Hello, World!";
: This command outputs the string "Hello, World!" to the browser.?>
: This tag ends the PHP code block. It's optional if your PHP script is the only thing in the file.
3. Run Your PHP Script:
Open your web browser and type
http://localhost/hello_world/index.php
in the address bar.You should see the message "Hello, World!" displayed on the page.
Understanding the PHP Syntax
PHP syntax is straightforward and easy to learn. Here's a breakdown of what you've written:
PHP Tags:
<?php
and?>
are used to indicate the start and end of PHP code.Echo Statement:
echo
is a PHP statement used to output text or variables to the browser.Semicolon: Each PHP statement must end with a semicolon (
;
).
Embedding PHP in HTML
One of PHP's strengths is its ability to be embedded within HTML. Let's enhance our script by adding some HTML code.
1. Updateindex.php
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello World</title>
</head>
<body>
<h1><?php echo "Hello, World!"; ?></h1>
</body>
</html>
Explanation:
The HTML structure includes the
DOCTYPE
,html
,head
, andbody
tags.PHP is embedded within the
<h1>
tag, demonstrating how PHP can be used to dynamically generate HTML content.
2. Run the Updated Script:
- Refresh your browser to see the updated page with "Hello, World!" displayed as an
<h1>
heading.
PHP Comments
Comments are essential for documenting your code. PHP supports both single-line and multi-line comments.
1. Single-Line Comment:
// This is a single-line comment
echo "Hello, World!";
2. Multi-Line Comment:
/*
This is a multi-line comment
that spans multiple lines.
*/
echo "Hello, World!";
Comments are ignored by the PHP interpreter and are useful for explaining your code to others (or to yourself).
Conclusion
Congratulations! You've written and run your first PHP script. Today, you learned how to create a PHP file, write basic PHP code, embed PHP in HTML, and use comments. These fundamentals are the building blocks for more complex PHP applications.
Subscribe to my newsletter
Read articles from Saurabh Damale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saurabh Damale
Saurabh Damale
Hello there! ๐ I'm Saurabh Kailas Damale, a passionate Full Stack Developer with a strong background in creating dynamic and responsive web applications. I thrive on turning complex problems into elegant solutions, and I'm always eager to learn new technologies and tools to enhance my development skills.