๐ Mastering the Art of State Management in React: An In-Depth Guide to Recoil
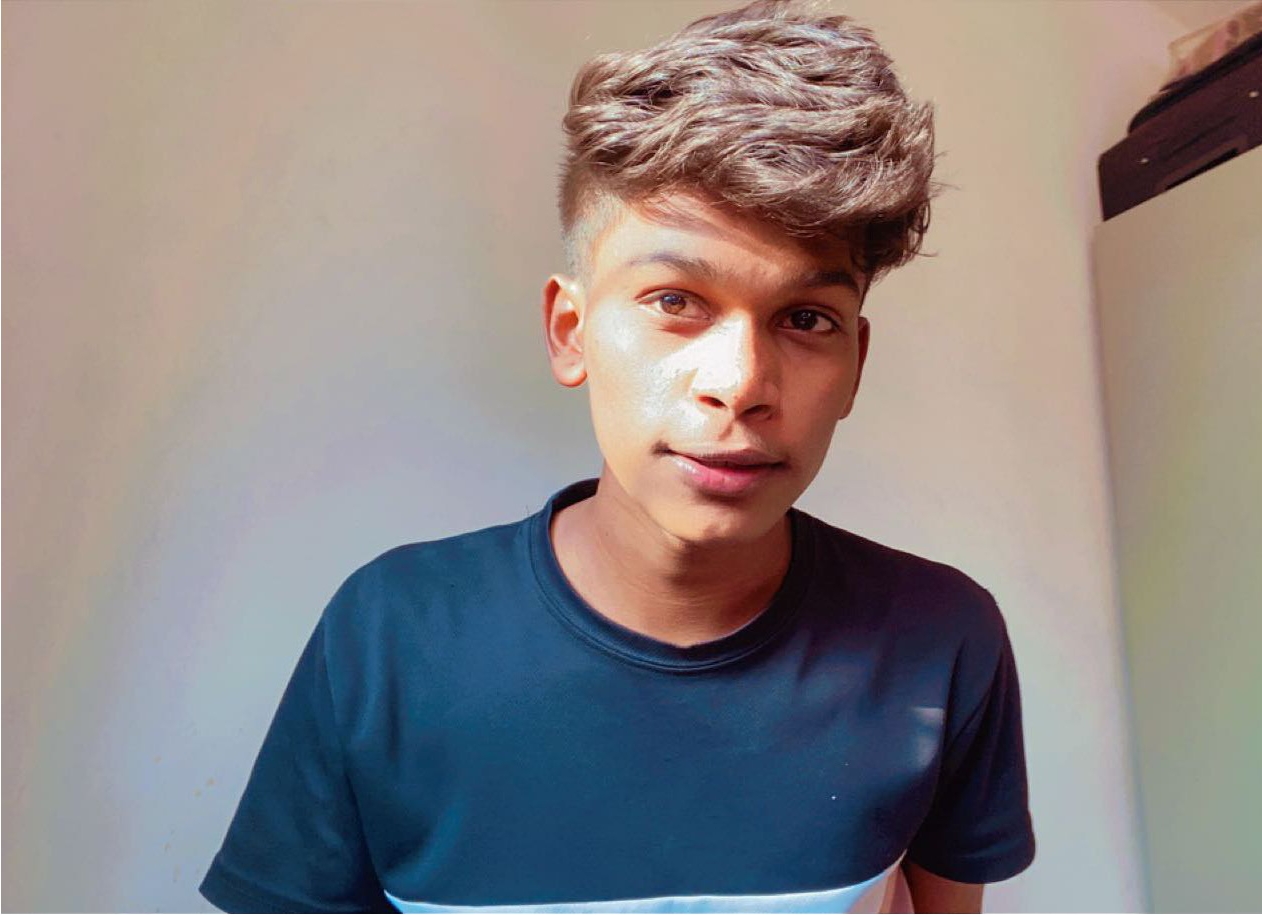
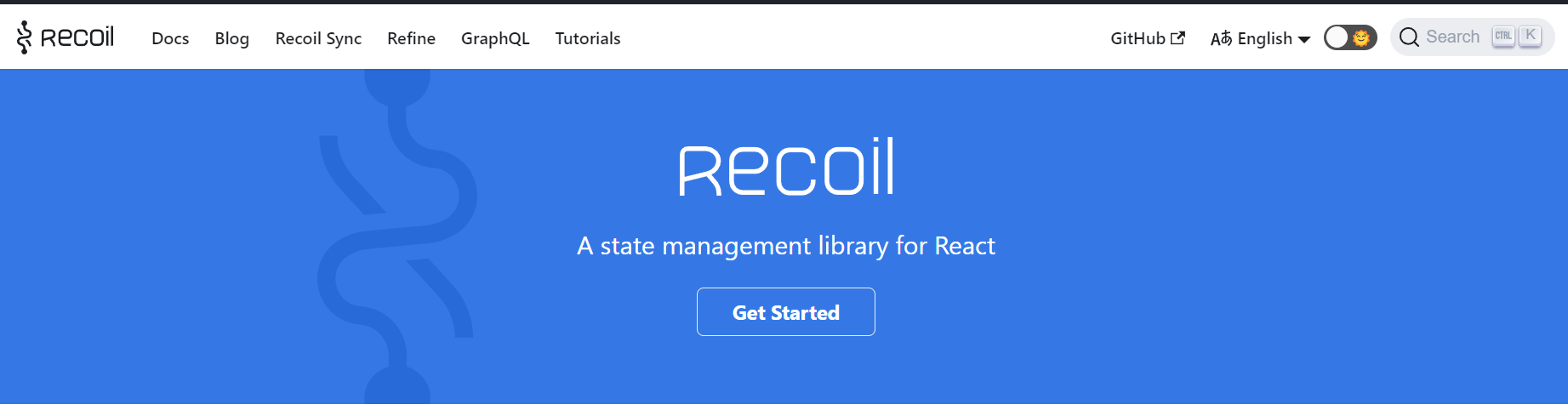
๐ Introduction
Managing state in large React applications can be a daunting task. With various state management libraries like Redux, MobX, and Context API, developers often struggle to choose the right tool for their projects. Enter Recoil โ a state management library from Facebook that promises simplicity, performance, and scalability. In this blog, we'll dive deep into Recoil, explore its features, and see how it can revolutionize your React development workflow. Buckle up and get ready for a ride through the world of efficient state management! ๐โจ
๐ค Why Recoil?
Before we jump into Recoil, let's address the question: why do we need another state management library?
1. Simplicity ๐จ
Recoil aims to provide a simple and intuitive API that makes it easy to manage state without the boilerplate code often associated with other libraries.
2. Performance ๐
Recoil is designed with performance in mind, offering features like asynchronous state updates and efficient re-rendering.
3. Scalability ๐
Whether you're building a small app or a large-scale application, Recoil scales effortlessly, allowing you to manage complex state dependencies with ease.
4. Reactivity ๐
Recoil provides fine-grained reactivity, ensuring that only the components that need to re-render do so, improving overall application performance.
๐ก The Basics of Recoil
Recoil introduces a few key concepts that are essential to understanding how it works:
Atoms โ๏ธ
Atoms are units of state. They can be read from and written to from any component. When an atom's state changes, any component that subscribes to that atom will re-render.
Selectors ๐ฏ
Selectors are derived state. They can compute values based on atoms or other selectors. They are used to encapsulate state logic and can be synchronous or asynchronous.
RecoilRoot ๐ณ
RecoilRoot is a provider component that wraps your application and allows you to use Recoil state throughout your component tree.
๐ ๏ธ Setting Up Recoil in Your React Project
Setting up Recoil in your React project is straightforward. Follow these steps:
1. Install Recoil
npm install recoil
2. Wrap Your App with RecoilRoot
import React from 'react';
import ReactDOM from 'react-dom';
import { RecoilRoot } from 'recoil';
import App from './App';
ReactDOM.render(
<RecoilRoot>
<App />
</RecoilRoot>,
document.getElementById('root')
);
3. Define Your Atoms and Selectors
import { atom, selector } from 'recoil';
export const textState = atom({
key: 'textState', // unique ID (with respect to other atoms/selectors)
default: '', // default value (aka initial value)
});
export const charCountState = selector({
key: 'charCountState', // unique ID (with respect to other atoms/selectors)
get: ({ get }) => {
const text = get(textState);
return text.length;
},
});
4. Use Atoms and Selectors in Your Components
import React from 'react';
import { useRecoilState, useRecoilValue } from 'recoil';
import { textState, charCountState } from './state';
function TextInput() {
const [text, setText] = useRecoilState(textState);
const onChange = (event) => {
setText(event.target.value);
};
return (
<div>
<input type="text" value={text} onChange={onChange} />
<p>Echo: {text}</p>
</div>
);
}
function CharacterCount() {
const count = useRecoilValue(charCountState);
return <p>Character Count: {count}</p>;
}
function App() {
return (
<div>
<TextInput />
<CharacterCount />
</div>
);
}
export default App;
๐ Core Concepts
Asynchronous Selectors โณ
Recoil supports asynchronous selectors, allowing you to fetch data from APIs or perform async operations.
export const asyncData = selector({
key: 'asyncData',
get: async () => {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return data;
},
});
Atom Families ๐จโ๐ฉโ๐งโ๐ฆ
Atom families allow you to create atoms with dynamic keys, useful for managing a collection of similar pieces of state.
import { atomFamily } from 'recoil';
export const itemState = atomFamily({
key: 'itemState',
default: (id) => ({ id, value: '' }),
});
Recoil Persist ๐ฆ
Persisting state across sessions is a common requirement. Libraries like recoil-persist can help with this.
npm install recoil-persist
import { recoilPersist } from 'recoil-persist';
const { persistAtom } = recoilPersist();
export const textState = atom({
key: 'textState',
default: '',
effects_UNSTABLE: [persistAtom],
});
๐ Real-World Use Case
Imagine you're building a todo application. Here's how Recoil can simplify state management:
1. Define Atoms and Selectors
export const todoListState = atom({
key: 'todoListState',
default: [],
});
export const filteredTodoListState = selector({
key: 'filteredTodoListState',
get: ({ get }) => {
const filter = get(todoListFilterState);
const list = get(todoListState);
switch (filter) {
case 'Show Completed':
return list.filter((item) => item.isComplete);
case 'Show Uncompleted':
return list.filter((item) => !item.isComplete);
default:
return list;
}
},
});
2. Create Components
import React from 'react';
import { useRecoilValue, useSetRecoilState } from 'recoil';
import { filteredTodoListState, todoListState } from './state';
function TodoList() {
const todoList = useRecoilValue(filteredTodoListState);
const setTodoList = useSetRecoilState(todoListState);
const addItem = () => {
setTodoList((oldTodoList) => [
...oldTodoList,
{
id: getId(),
text: '',
isComplete: false,
},
]);
};
return (
<div>
{todoList.map((todoItem) => (
<TodoItem key={todoItem.id} item={todoItem} />
))}
<button onClick={addItem}>Add Item</button>
</div>
);
}
function TodoItem({ item }) {
const [todoList, setTodoList] = useRecoilState(todoListState);
const index = todoList.findIndex((listItem) => listItem === item);
const editItemText = ({ target: { value } }) => {
const newList = replaceItemAtIndex(todoList, index, {
...item,
text: value,
});
setTodoList(newList);
};
return (
<div>
<input type="text" value={item.text} onChange={editItemText} />
</div>
);
}
function replaceItemAtIndex(arr, index, newValue) {
return [...arr.slice(0, index), newValue, ...arr.slice(index + 1)];
}
let id = 0;
function getId() {
return id++;
}
๐ Best Practices
1. Keep Atoms Small and Focused ๐ฏ
Avoid creating large atoms that store too much state. Keep them small and focused to ensure optimal performance.
2. Use Selectors for Computations ๐งฎ
Encapsulate state logic within selectors to keep your atoms simple and your components clean.
3. Leverage Atom Families for Dynamic State ๐จโ๐ฉโ๐งโ๐ฆ
Use atom families to manage collections of similar state, reducing redundancy and improving code organization.
4. Persist State Where Necessary ๐ฆ
Use libraries like recoil-persist to persist state across sessions, enhancing user experience.
๐ Conclusion
Switching to Recoil for state management in your React applications can significantly improve your development workflow. With its simplicity, performance, and scalability, Recoil offers a powerful alternative to traditional state management libraries. By embracing Recoil, you'll find yourself writing cleaner, more maintainable code and delivering high-quality applications with ease. So, dive into Recoil, and let it revolutionize the way you manage state in your React projects! ๐โจ
Subscribe to my newsletter
Read articles from Aditya Dhaygude directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
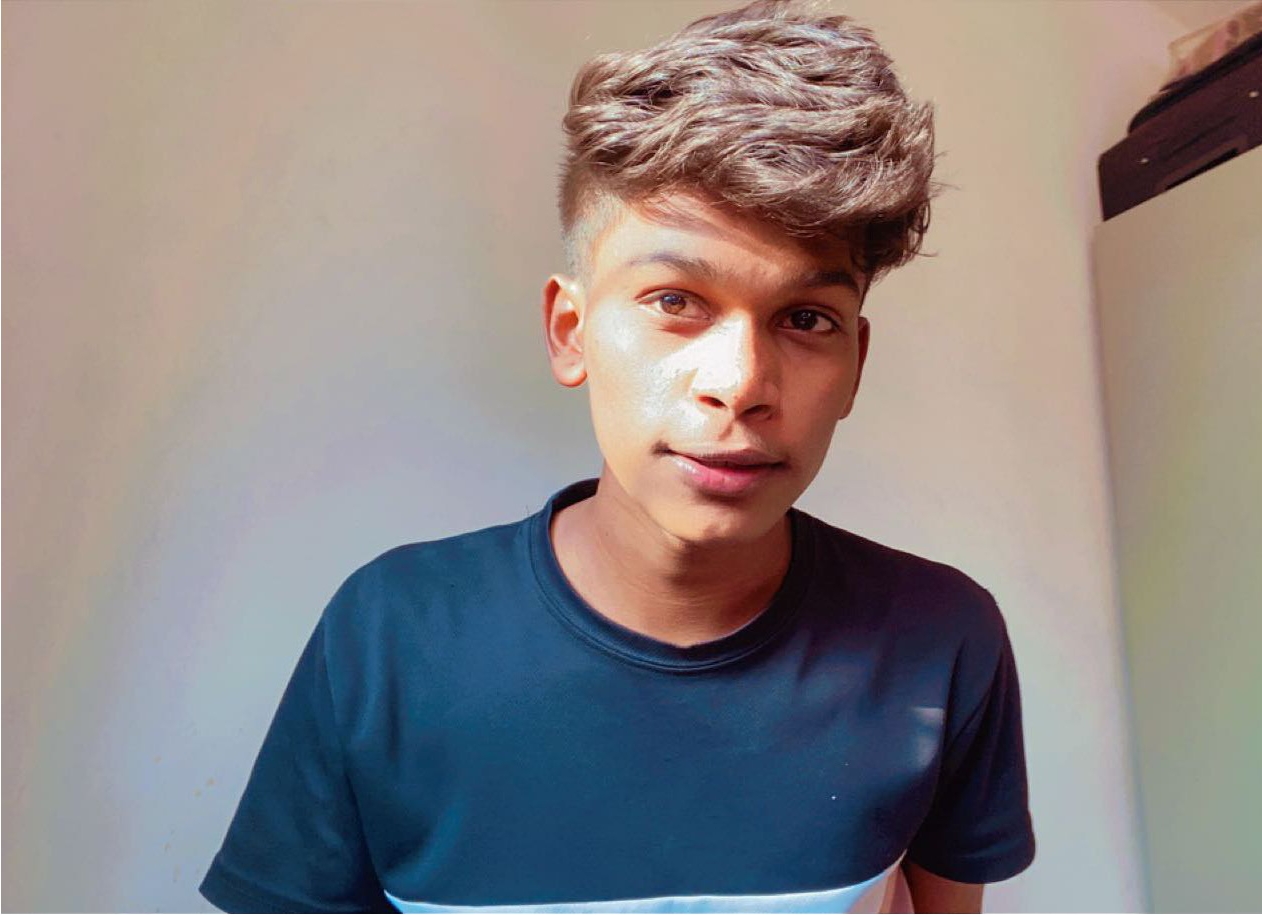
Aditya Dhaygude
Aditya Dhaygude
i Build Stuff