Understanding Char Arrays and Strings
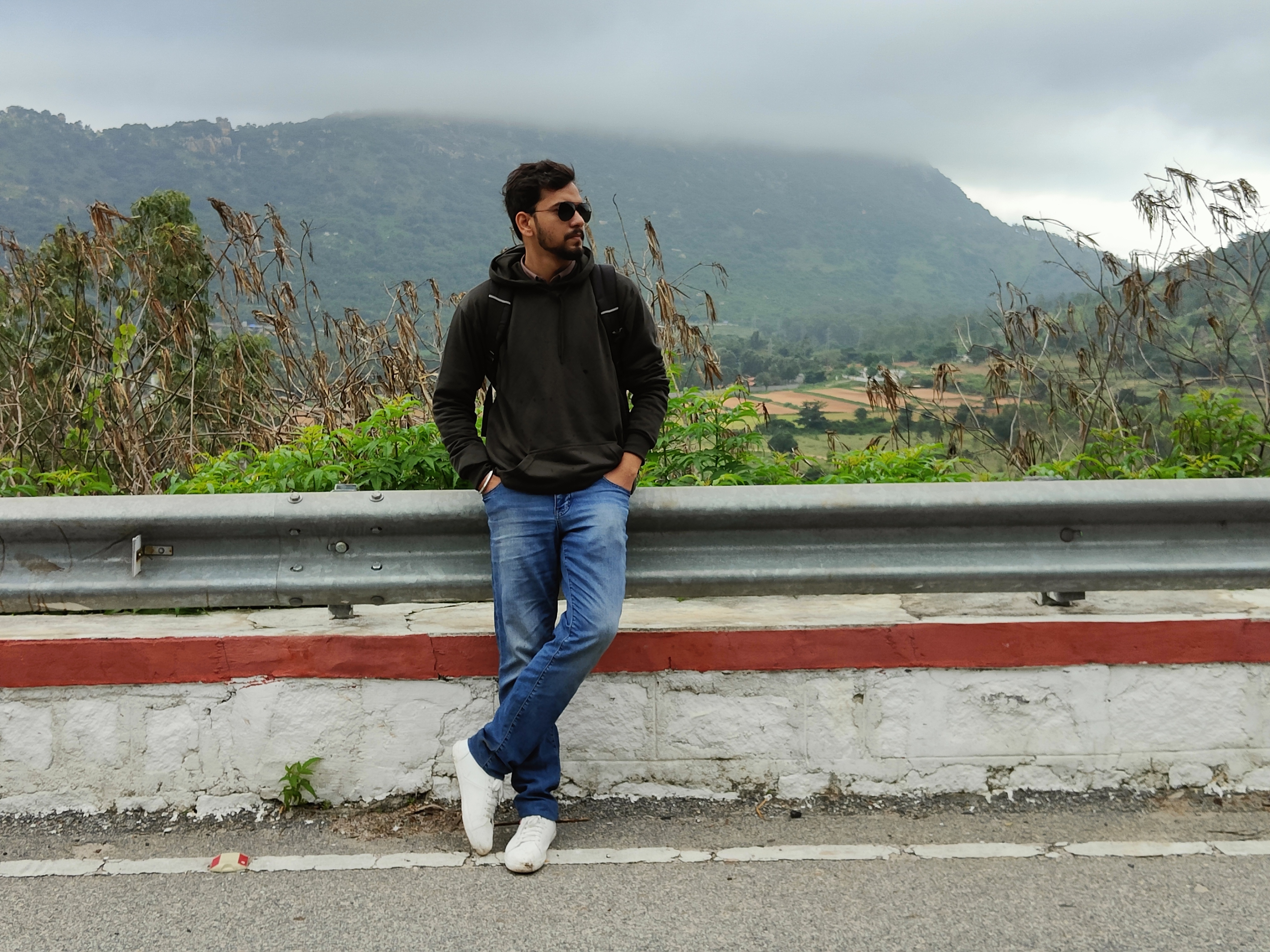

What is char Array[]
?
In C++ a character array (or char array) is a sequence of characters stored in contiguous memory locations. Char arrays are often used to store strings, although C++ also provides the 'std::string' class, which is more convenient and safer to use. Here's a basic overview of how to work with char arrays in C++.
Declaration and Initialization:
Null Terminator: A char array representing a string must end with a null terminator('\0'). This character signals the end of the string.
Size: When declaring a char array with a specific size, make sure the size is large enough to hold the string and the full terminator.
Accessing Elements:
char str[] = "Hello";
char firstChar = str[0]; // 'H'
char secondChar = str[1]; // 'e'
Input & Output:
In C++, 'getline' is a function used to read an entire line of text from an input stream, such as 'cin' (standard input) or a file. It reads characters until it encounters a newline character ('\n') or the end of the input stream.
Some character array questions:
Print All ASCII Value of name:
Output:
For more information on the ASCII table Please visit :
ASCII stands for (American Standard Code for Information Interchange) the value of a character to an 'int'. ASCII are numerical representations of the characters ranging from 0 to 127.
- Reverse the Character Array:
Code Analysis:
A char array of size 100 is given. The code asks the user to give the name of the character array.
getLength() function is used to take the length of the given input. It counts the number of characters in the array excluding the null character. getlin() is used to take input including space.
reverseCharArray() is used to reverse the given array. We need to run the loop till the length of the array. We will use the two-pointer technique to swap the elements. Place two pointers at the first index and last index.
- Check Palindrome:
Code Analysis:
length() function is used to find the length of the given input.
palindrome states the output will same if we read from the first index to the last index or from the last index to the first index.
Here we will use the pointer technique to traverse the input array. If any character does not match then we will return false.
Considerations:
Bounds Checking: C++ does not perform bounds checking on arrays. Accessing out-of-bounds indices can lead to undefined behavior.
Safety: Prefer using 'std::string' for safer and more convenient string manipulation.
By understanding and correctly managing char Arrays, you can effectively handle low-level string operations in C++.
What are strings in C++?
In C++, the 'std::string' class from the C++ standard library provides a more convenient and safer way to work with strings compared to char arrays. Here is an overview of how to use 'std::string' in C++.
Declaration and Initialization:
Common operations:
Concatenation: You can concatenate strings using the '+' operator or the '+=' operator.
std::string str1 = "Hello, "; std::string str2 = "World!"; std::string str3 = str1 + str2; // "Hello, World!" str1 += str2; // str1 is now "Hello, World!"
Accessing Characters: You can access characters using the '[]' operator or the 'at()' operator.
Modifying characters: You can modify characters directly.
length: You can get the length of the string using the 'length()' or 'size()' methods.
std::string str = "Hello"; char ch1 = str[0]; // 'H' char ch2 = str.at(1); // 'e' -----------Modifying---------------- std::string str = "Hello"; str[0] = 'h'; // str is now "hello" ---------length--------------------- std::string str = "Hello"; std::size_t len = str.length(); // 5 std::size_t size = str.size(); // 5
substrings: You can extract substrings using the 'substr()' method.
std::string str = "Hello, World!"; std::string substr = str.substr(7, 5); // "World"
Some Questions on Strings:
Remove All adjacent duplicates from the string:
Code Analysis:
A string is given having adjacent duplicates.
A empty string is required to store the non-duplicate elements.
using for loop traverse the string and check the current element is present or not. If empty string is empty then we will push the element in the answer string. if same element is present remove right most element from the empty string. If element is not same push the element.
Leetcode - 647 Palindromic Substring:
Code Analysis:
Given a string s. Need to find substring which is palindrome.
We need to count the substring. Each character is itself a palindromic substring.
There is a way to solve this type of problems called expand through center. It is same as finding palindrome where we shorten the result, but here we need to expand to right and left of the character. We need to take each character as center and move right and left.
Firstly we need to find odd palindromic substring and then even palindromic substrings.
For odd we need to place i and j on same character and if same we need to move i to left and j to right (here i and j are pointers which point to same index).
For even we need to place i on starting index and j on i+1 index.
Considerations:
Using 'std::string' is generally recommended over char arrays for arrays, ease of use, and functionality. The 'std::string' class handles memory management for you and provides a rich set of methods for string manipulation.
Subscribe to my newsletter
Read articles from Rishabh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
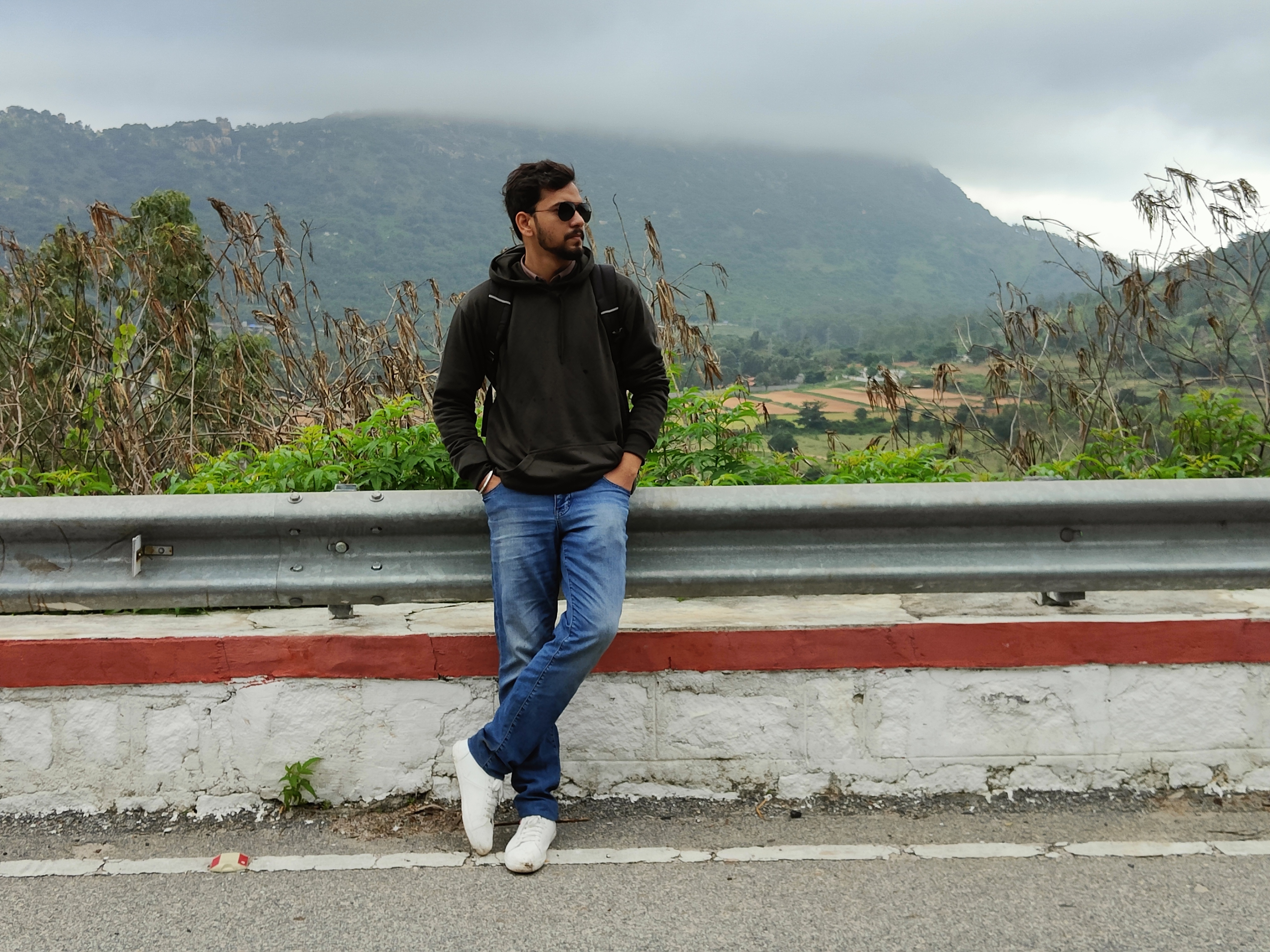