Object-Oriented Programming in Game Development: Key Concepts and Examples
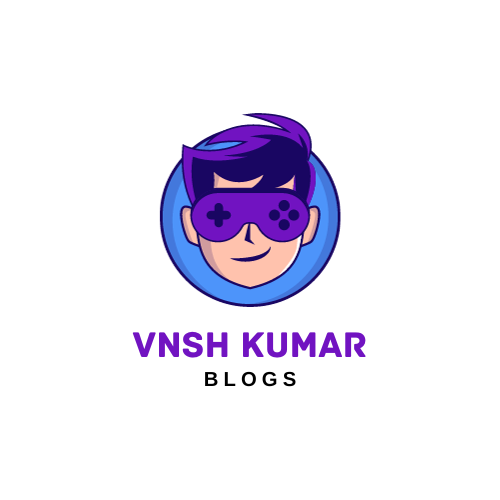
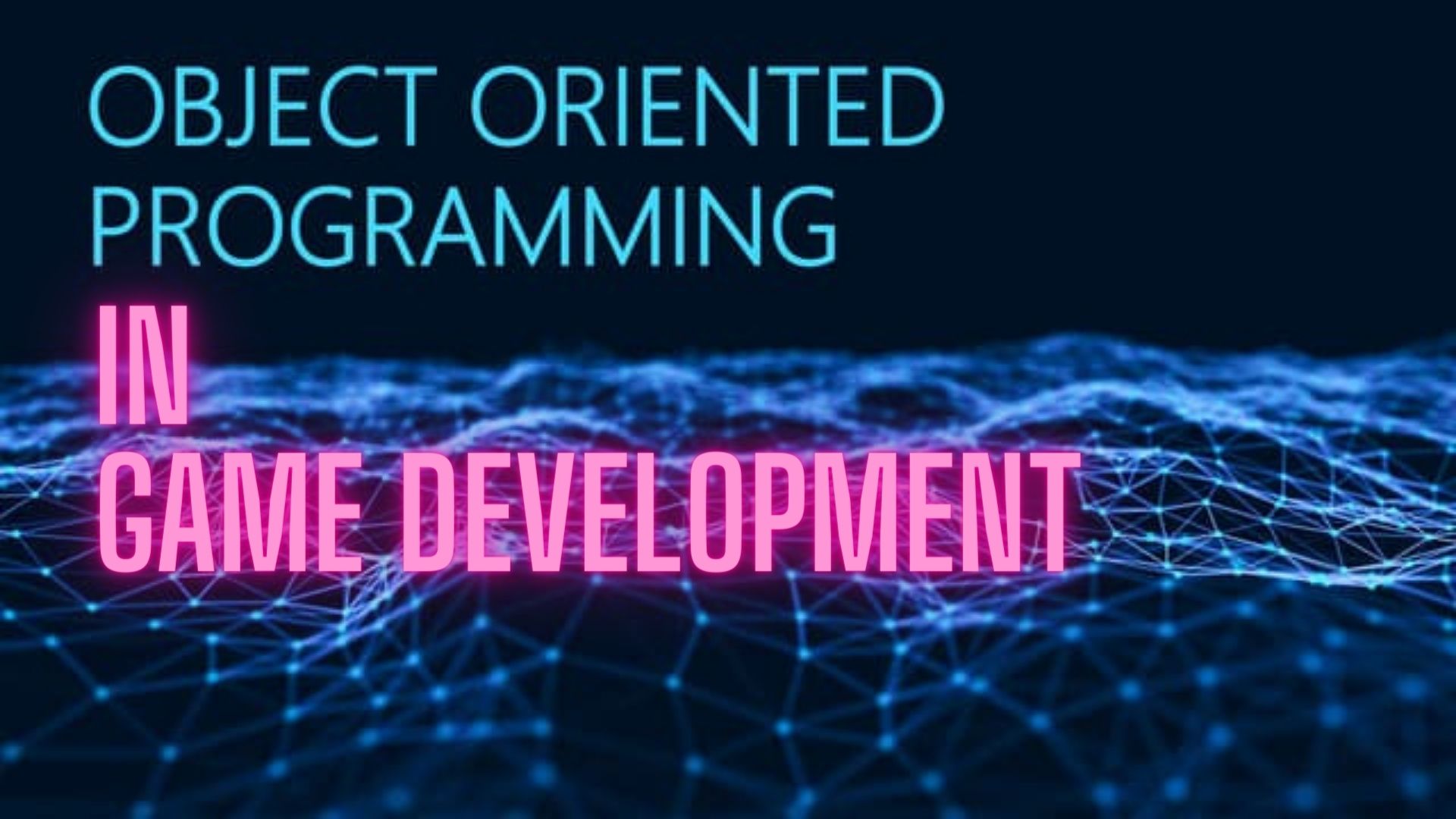
Introduction
Object-Oriented Programming (OOP) is a programming paradigm that uses "objects" to represent data and methods to manipulate that data. It's a powerful approach that's widely used in game development for its ability to manage complex systems and create reusable code. In this blog, we'll delve into the key concepts of OOP and provide detailed examples related to game development.
Table of Contents
What is Object-Oriented Programming?
Key Concepts of OOP
Classes and Objects
Encapsulation
Inheritance
Polymorphism
Abstraction
Practical Examples in Game Development
Creating a Player Class
Implementing Encapsulation with Health and Damage
Using Inheritance for Game Characters
Polymorphism with Abilities and Actions
Abstract Classes for Game Mechanics
Advanced OOP Concepts
Composition over Inheritance
Design Patterns in Game Development
Conclusion
1. What is Object-Oriented Programming?
OOP is a method of programming that organizes software design around data, or objects, rather than functions and logic. An object can be a data field with unique attributes and behavior. This approach helps developers create modular, scalable, and maintainable software.
2. Key Concepts of OOP
Classes and Objects
A class is a blueprint for creating objects (a particular data structure), providing initial values for state (member variables or fields), and implementations of behavior (member functions or methods). An object is an instance of a class.
public class Player
{
public string Name;
public int Health;
public int AttackPower;
public void Attack(Player target)
{
target.Health -= this.AttackPower;
}
}
// Creating objects
Player player1 = new Player();
player1.Name = "Hero";
player1.Health = 100;
player1.AttackPower = 20;
Player player2 = new Player();
player2.Name = "Villain";
player2.Health = 80;
player2.AttackPower = 15;
// Using objects
player1.Attack(player2);
Encapsulation
Encapsulation is the concept of wrapping data and the methods that operate on the data within one unit, e.g., a class in OOP. This mechanism restricts direct access to some of an object's components, which can prevent the accidental modification of data.
public class Player
{
private int health;
public int Health
{
get { return health; }
private set { health = Mathf.Clamp(value, 0, 100); }
}
public void TakeDamage(int damage)
{
Health -= damage;
}
}
Inheritance
Inheritance allows a class (subclass or derived class) to inherit attributes and methods from another class (superclass or base class). This promotes code reuse and establishes a natural hierarchy between classes.
public class Character
{
public string Name;
public int Health;
public virtual void Attack(Character target)
{
// Default attack behavior
}
}
public class Warrior : Character
{
public int Armor;
public override void Attack(Character target)
{
base.Attack(target);
// Additional attack behavior for a Warrior
}
}
public class Mage : Character
{
public int Mana;
public override void Attack(Character target)
{
base.Attack(target);
// Additional attack behavior for a Mage
}
}
Polymorphism
Polymorphism allows methods to be used in different ways based on the object it is acting upon, even though they share the same name. This can be achieved through method overriding and method overloading
public class Character
{
public string Name;
public virtual void Speak()
{
Debug.Log("Character speaks.");
}
}
public class Warrior : Character
{
public override void Speak()
{
Debug.Log("Warrior roars.");
}
}
public class Mage : Character
{
public override void Speak()
{
Debug.Log("Mage chants.");
}
}
public class Game
{
public void MakeCharacterSpeak(Character character)
{
character.Speak();
}
}
Abstraction
Abstraction involves hiding complex implementation details and exposing only the necessary features of an object. This simplifies the interaction with the object and reduces complexity.
public abstract class Weapon
{
public abstract void Use();
}
public class Sword : Weapon
{
public override void Use()
{
Debug.Log("Swinging sword.");
}
}
public class Bow : Weapon
{
public override void Use()
{
Debug.Log("Shooting arrow.");
}
}
3. Practical Examples in Game Development
Creating a Player Class
Let's create a more detailed Player class that incorporates various OOP concepts.
public class Player
{
private string name;
private int health;
private int attackPower;
public Player(string name, int health, int attackPower)
{
this.name = name;
this.health = health;
this.attackPower = attackPower;
}
public string Name => name;
public int Health
{
get { return health; }
private set { health = Mathf.Clamp(value, 0, 100); }
}
public void Attack(Player target)
{
target.TakeDamage(attackPower);
}
public void TakeDamage(int damage)
{
Health -= damage;
}
}
Implementing Encapsulation with Health and Damage
Encapsulation ensures that health cannot be set to an invalid value.
public class Player
{
private int health;
public int Health
{
get { return health; }
private set { health = Mathf.Clamp(value, 0, 100); }
}
public void TakeDamage(int damage)
{
Health -= damage;
if (Health <= 0)
{
Die();
}
}
private void Die()
{
Debug.Log("Player has died.");
}
}
Using Inheritance for Game Characters
We can create different types of characters by extending a base class.
public class Character
{
public string Name { get; private set; }
public int Health { get; private set; }
public Character(string name, int health)
{
Name = name;
Health = health;
}
public virtual void Attack(Character target)
{
Debug.Log($"{Name} attacks {target.Name}");
}
}
public class Warrior : Character
{
public int Armor { get; private set; }
public Warrior(string name, int health, int armor) : base(name, health)
{
Armor = armor;
}
public override void Attack(Character target)
{
base.Attack(target);
Debug.Log($"{Name} deals heavy damage.");
}
}
public class Mage : Character
{
public int Mana { get; private set; }
public Mage(string name, int health, int mana) : base(name, health)
{
Mana = mana;
}
public override void Attack(Character target)
{
base.Attack(target);
Debug.Log($"{Name} casts a spell.");
}
}
Polymorphism with Abilities and Actions
Polymorphism allows us to treat different game objects uniformly.
public abstract class Ability
{
public abstract void Use();
}
public class Fireball : Ability
{
public override void Use()
{
Debug.Log("Casting Fireball.");
}
}
public class Heal : Ability
{
public override void Use()
{
Debug.Log("Casting Heal.");
}
}
public class Character
{
private Ability ability;
public Character(Ability ability)
{
this.ability = ability;
}
public void UseAbility()
{
ability.Use();
}
}
Abstract Classes for Game Mechanics
Abstract classes can define a template for common game mechanics.
public abstract class GameMechanic
{
public abstract void Activate();
public abstract void Deactivate();
}
public class Trap : GameMechanic
{
public override void Activate()
{
Debug.Log("Trap activated.");
}
public override void Deactivate()
{
Debug.Log("Trap deactivated.");
}
}
public class PowerUp : GameMechanic
{
public override void Activate()
{
Debug.Log("PowerUp activated.");
}
public override void Deactivate()
{
Debug.Log("PowerUp deactivated.");
}
}
4. Advanced OOP Concepts
Composition over Inheritance
Composition is often preferred over inheritance as it provides greater flexibility.
public class Engine
{
public void Start()
{
Debug.Log("Engine started.");
}
public void Stop()
{
Debug.Log("Engine stopped.");
}
}
public class Car
{
private Engine engine = new Engine();
public void StartCar()
{
engine.Start();
}
public void StopCar()
{
engine.Stop();
}
}
Design Patterns in Game Development
Design patterns provide solutions to common problems in software design.
Singleton Pattern:
Ensures a class has only one instance and provides a global point of access to it.
public class GameManager
{
private static GameManager instance;
private GameManager() { }
public static GameManager Instance
{
get
{
if (instance == null)
{
instance = new GameManager();
}
return instance;
}
}
}
Observer Pattern:
Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified.
public class Subject
{
private List<Observer> observers = new List<Observer>();
public void Attach(Observer observer)
{
observers.Add(observer);
}
public void Detach(Observer observer)
{
observers.Remove(observer);
}
public void Notify()
{
foreach (Observer observer in observers)
{
observer.Update();
}
}
}
public abstract class Observer
{
public abstract void Update();
}
public class ConcreteObserver : Observer
{
public override void Update()
{
Debug.Log("Observer updated.");
}
}
5.Conclusion
Object-Oriented Programming is a foundational skill for game developers, enabling the creation of complex, scalable, and maintainable game systems. By understanding and applying key OOP concepts such as encapsulation, inheritance, polymorphism, and abstraction, you can significantly enhance your game development projects. Moreover, advanced OOP concepts like composition and design patterns further contribute to writing efficient and reusable code.
Whether you're building a simple RPG or a sophisticated simulator, mastering OOP will help you manage your codebase effectively and bring your game ideas to life. Keep exploring and practicing these concepts, and you'll become a more proficient and versatile game developer.
Happy coding!
Subscribe to my newsletter
Read articles from vnsh kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
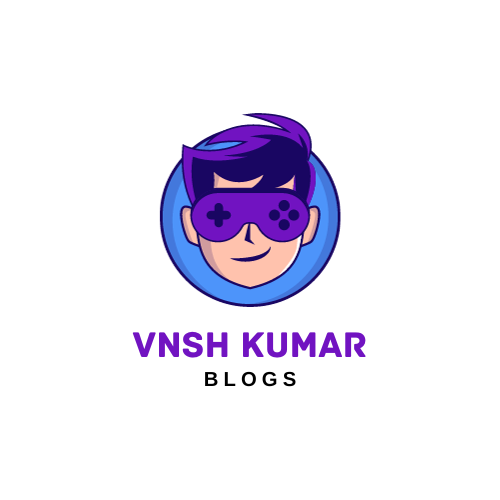
vnsh kumar
vnsh kumar
I am a Game Developer.