FastAPI for Beginners: Building Fast, Modern APIs with Python
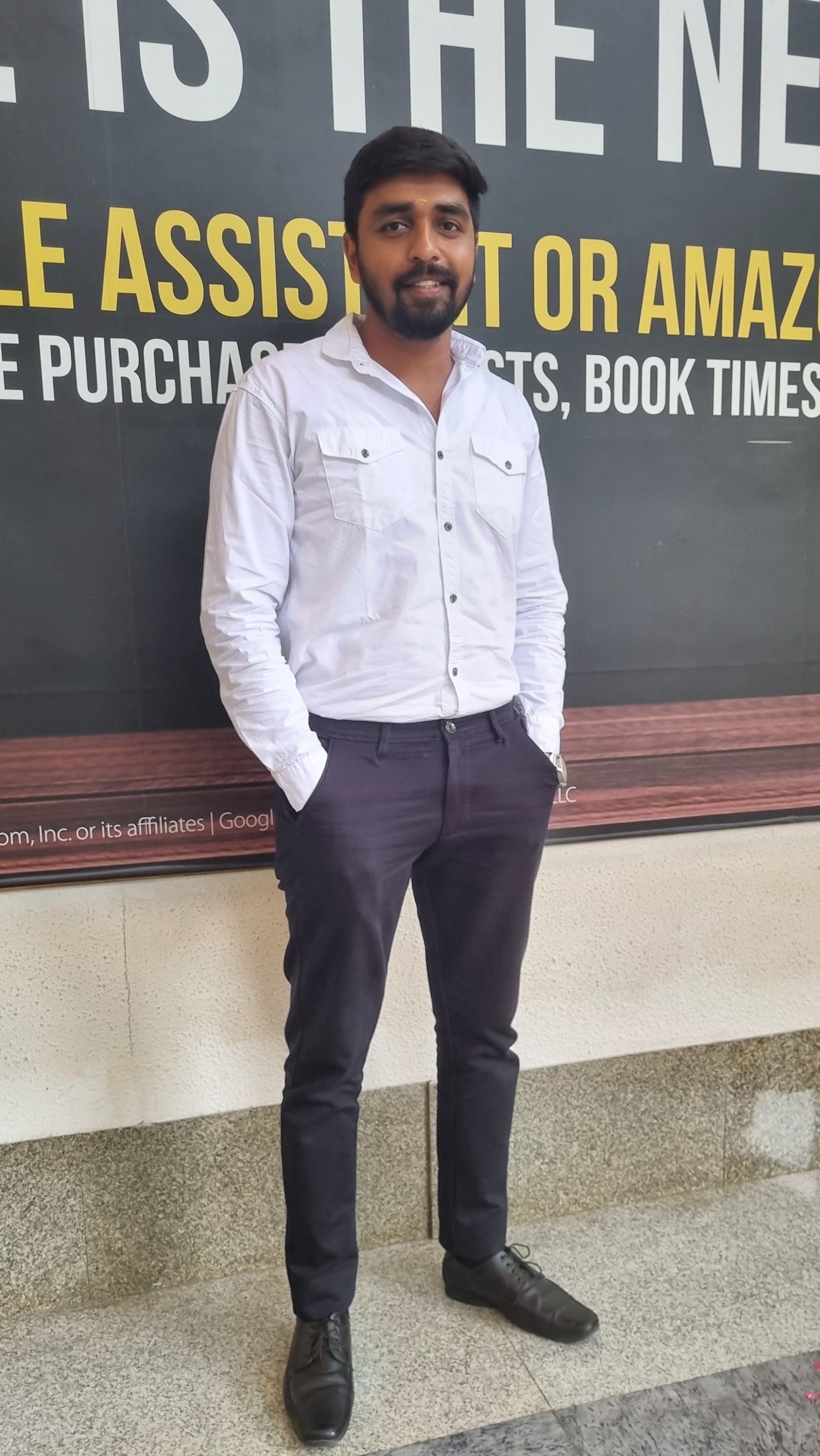
Introduction
FastAPI is a modern, high-performance web framework for building APIs with Python 3.7+ using standard Python type hints. It's designed to be easy to use and to create efficient, scalable web applications.
What is FastAPI?
FastAPI is a web framework that allows you to build APIs quickly and efficiently. It's designed to be developer-friendly, and it's based on Python type hints which makes it highly readable and easy to use.
Why Choose FastAPI?
Key Features of FastAPI
Super Fast: FastAPI is one of the quickest web frameworks for Python, as fast as NodeJS and Go.
Quick to Code: You can build features 2-3 times faster, saving you a lot of development time.
Fewer Bugs: FastAPI helps reduce mistakes made by developers by about 40%.
Easy to Use: It offers great support in code editors, making coding smoother and less prone to errors.
Simple to Learn: FastAPI is designed to be straightforward, so you spend less time reading manuals.
Less Repetition: You write less code, but still get more features, leading to fewer errors.
Ready for Production: It provides reliable code that's ready to be used in real-world applications, with automatic interactive documentation.
Based on Standards: FastAPI follows industry standards for APIs, like OpenAPI and JSON Schema, ensuring it's compatible and reliable.
Pros and Cons
Pros
High Performance: FastAPI is one of the fastest web frameworks available, allowing you to handle many requests per second.
Automatic Documentation: Generates interactive API documentation automatically.
Easy to Use: Based on standard Python type hints, making it highly readable and easy to write.
Cons
Newer Framework: Being relatively new, it might lack some features available in older frameworks.
Learning Curve: While easy to use, understanding async programming and type hints can be challenging for beginners.
Community Size: Smaller community compared to more established frameworks like Django or Flask.
Installing FastAPI
To start using FastAPI, you need to install it and a server called uvicorn
.
pip install fastapi uvicorn
Creating Your First FastAPI Application
Here's a simple example to create a "Hello, World!" application using FastAPI:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def read_root():
return {"message": "Hello, World!"}
To run the application, use the following command:
uvicorn main:app --reload
Navigate to http://127.0.0.1:8000
in your browser to see the result.
Interactive API Documentation
FastAPI automatically generates interactive API documentation, which can be accessed at http://127.0.0.1:8000/docs
.
Best Practices
Structuring Your FastAPI Project
Organize your FastAPI project by separating different components into modules. For example:
/app
/main.py
/models.py
/schemas.py
/routers
/items.py
/users.py
Case Studies and Examples
Real-World Example: Building a Task Manager
Suppose you want to build a simple task manager where users can create and manage their tasks.
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class Task(BaseModel):
title: str
description: str = None
completed: bool = False
tasks = []
@app.post("/tasks/")
def create_task(task: Task):
tasks.append(task)
return task
@app.get("/tasks/")
def read_tasks():
return tasks
Exploring the Swagger UI
When you visit http://127.0.0.1:8000/docs
, you'll see a Swagger UI that lists all the available endpoints in your FastAPI application. You can interact with these endpoints directly from your browser.
POST /tasks/: This endpoint allows you to create a new task. You can fill in the
title
,description
, andcompleted
fields and click the "Execute" button to send the request and see the response.GET /tasks/: This endpoint retrieves all the tasks. You can click "Try it out" and then "Execute" to see the list of tasks.
Resources
Official Documentation: FastAPI Documentation
Tools:
uvicorn
,httpx
for making HTTP requests,pytest
for testing.
Conclusion
FastAPI is a powerful and efficient framework for building APIs in Python. Its ease of use, performance, and automatic documentation make it an excellent choice for both beginners and experienced developers. Give it a try and see how it can improve your development workflow.
Subscribe to my newsletter
Read articles from Kavin (RK) directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
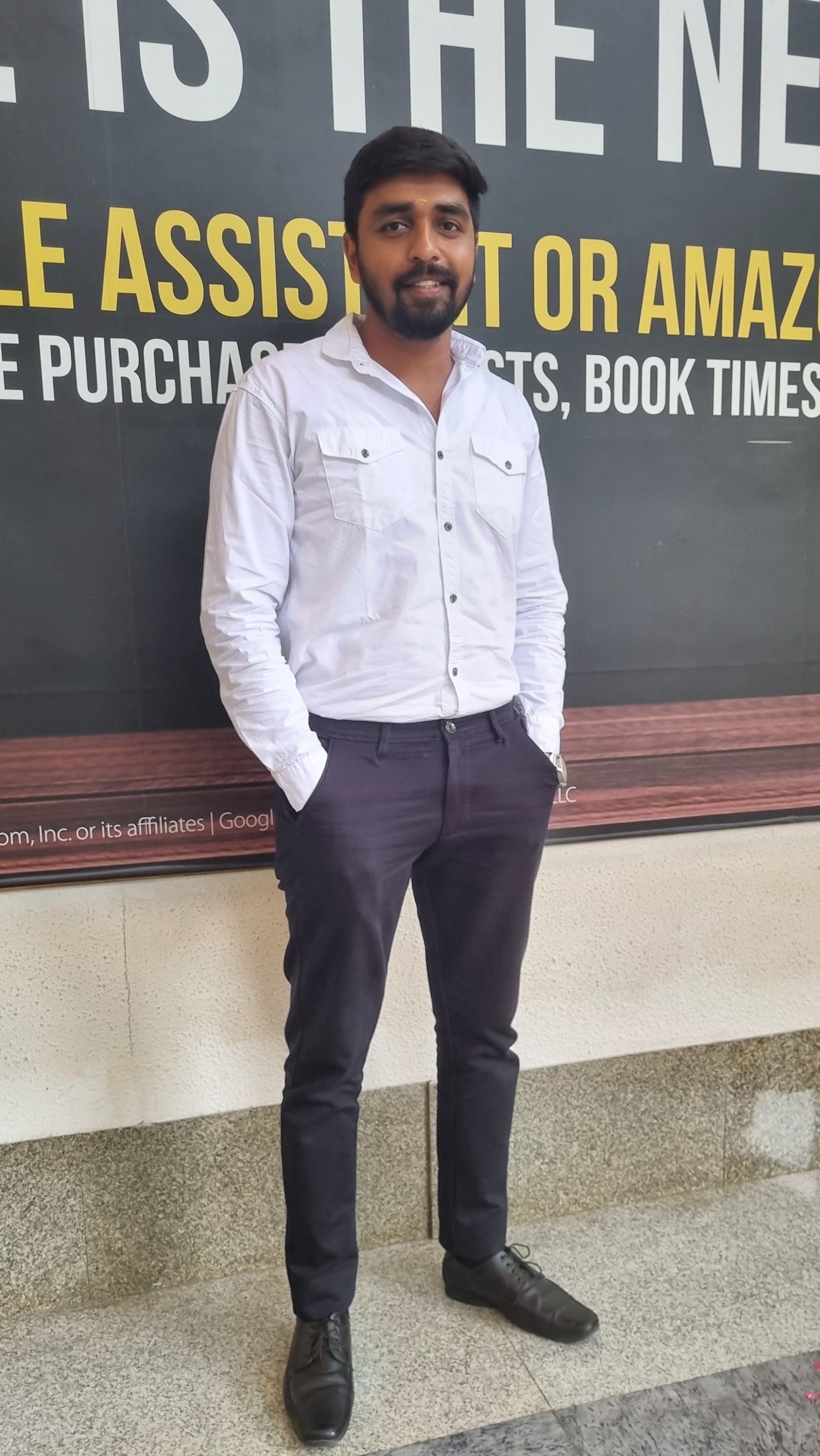