Automating User and Group Creation with a Bash Script
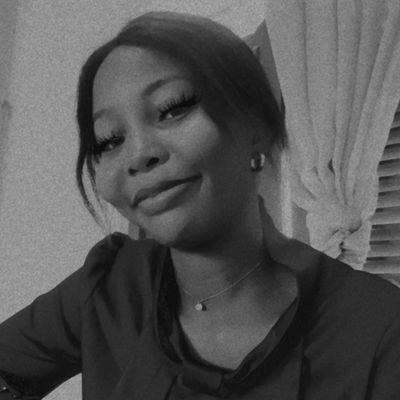
Introduction
This technical article is about the creation of a bash script named create_users.sh designed to automate user and group management on a Linux system. This script is part of the DevOps Stage 1 task for the HNG Internship. It reads a text file (user_lists.txt) with employee usernames and their respective groups, creates the users and groups, sets up home directories with appropriate permissions and ownership, generates random passwords for the users, and logs all actions. The script also securely stores the generated passwords.
Prerequisites
Basic knowledge of bash scripting.
Root access to a Linux system.
Git installed on your system.
A remote Git repository (e.g., GitHub).
Step 1: Creating the Input File
First, you need an input file that lists the users and their associated groups. Create a file named users.txt
with this format:
ronke; sudo,dev,admin
charity; sudo
mosh; designer,admin
Step 2: Writing the Bash Script
Next, you write a bash script named create_
users.sh
to automate the user and group management tasks.
#!/bin/bash
set -x # Enable debugging output
# Check if the script is run as root
if [ "$EUID" -ne 0 ]; then
echo "Please run as root"
exit 1
fi
# Check if input file is provided
if [ -z "$1" ]; then
echo "Usage: $0 <input_file>"
exit 1
fi
# Define the input file and log files
INPUT_FILE="$1"
LOG_FILE="/var/log/user_management.log"
PASSWORD_FILE="/var/secure/user_passwords.txt"
# Ensure /var/secure directory exists
mkdir -p /var/secure
chmod 700 /var/secure
# Clear previous log entries
> "$LOG_FILE"
# Function to generate a random password
generate_password() {
local password_length=12
tr -dc A-Za-z0-9 </dev/urandom | head -c $password_length
}
# Read the input file line by line
while IFS=';' read -r username groups; do
# Remove leading and trailing whitespaces
username=$(echo "$username" | xargs)
groups=$(echo "$groups" | xargs)
# Create user and personal group
if id "$username" &>/dev/null; then
echo "User $username already exists. Skipping creation..." | tee -a "$LOG_FILE"
else
# Create the user with a home directory
useradd -m "$username" -s /bin/bash
if [ $? -eq 0 ]; then
echo "Created user $username" | tee -a "$LOG_FILE"
# Create personal group with the same name as the username
usermod -aG "$username" "$username"
# Set the home directory permissions
chmod 700 /home/"$username"
chown "$username":"$username" /home/"$username"
# Generate a random password
password=$(generate_password)
echo "$username:$password" | chpasswd
# Store the password securely
echo "$username:$password" >> "$PASSWORD_FILE"
chmod 600 "$PASSWORD_FILE"
echo "Password for $username stored securely" | tee -a "$LOG_FILE"
else
echo "Failed to create user $username" | tee -a "$LOG_FILE"
continue
fi
fi
# Create and add groups to the user
IFS=',' read -r -a group_array <<< "$groups"
for group in "${group_array[@]}"; do
group=$(echo "$group" | xargs) # Remove leading and trailing whitespaces
if [ -n "$group" ]; then
if ! getent group "$group" &>/dev/null; then
groupadd "$group"
echo "Created group $group" | tee -a "$LOG_FILE"
fi
if id "$username" &>/dev/null; then
usermod -aG "$group" "$username"
if [ $? -eq 0 ]; then
echo "Added $username to group $group" | tee -a "$LOG_FILE"
else
echo "Failed to add $username to group $group" | tee -a "$LOG_FILE"
fi
else
echo "User $username does not exist to add to group $group" | tee -a "$LOG_FILE"
fi
fi
done
done < "$INPUT_FILE"
echo "User creation process completed." | tee -a "$LOG_FILE"
Step 3: Making the Script Executable
You can make the script executable by running the following:
chmod +x create_users.sh
Step 4: Running the Script
You can only run the script with root permissions:
sudo ./create_users.sh
Step 5: Adding the Script to a Remote Repository
Initialize a Git Repository: git init
Add the Script: git add create_
users.sh
Commit the Changes: git commit -m "commit message"
Add the Remote Repository: git remote add origin <remote_repository_url>
You can then push the Changes: git push origin main
Step 6: Testing the Script
Create a test environment by setting up a virtual machine and ensure you have root access.
Prepare the Input File: The
users.txt
file
ronke; sudo,dev,admin
charity; sudo
mosh; designer,admin
You have to make sure that the create_
users.sh
script is in the same directory as users.txt
- Run the Script:
sudo ./create_users.sh
- Check if the users were created:
getent passwd ronke charity mosh
- Verify the groups each user belongs to:
groups ronke charity mosh
- Confirm the home directories were created with the correct permissions:
ls -ld /home/ronke /home/charity /home/mosh
- Check the log file for actions performed by the script:
cat /var/log/user_management.log
- Ensure passwords are stored securely:
sudo cat /var/secure/user_passwords.txt
Conclusion
This script allows SysOps engineers to efficiently manage employees' usernames and group names, ensuring security and consistency.
To learn more about the HNG program, visit the HNG Internship websites:
Subscribe to my newsletter
Read articles from Ronke Akinyemi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
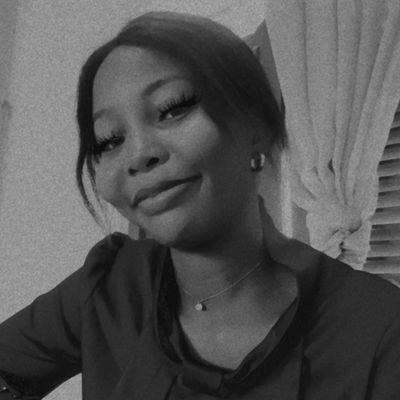
Ronke Akinyemi
Ronke Akinyemi
DevOps Engineer