Extracting Information from a DOCX File Using Python
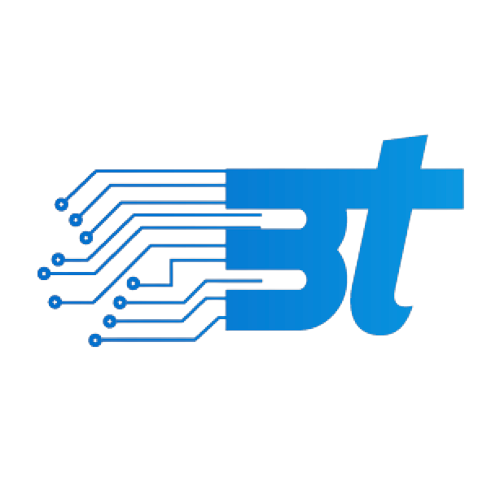
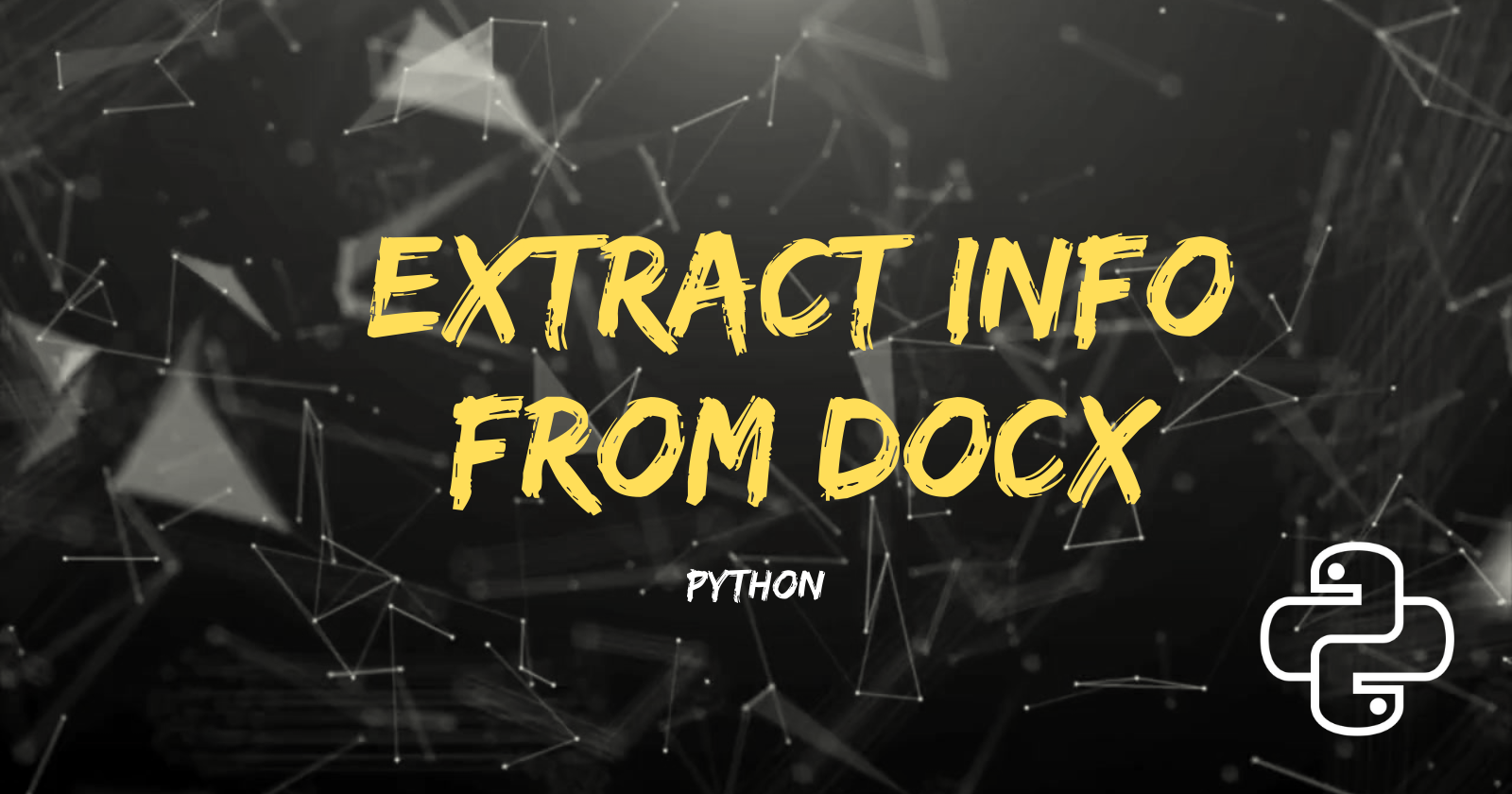
In today's data-driven world, the ability to extract valuable information from documents is crucial. One common task is extracting email addresses and other important details from .docx
files. This tutorial will walk you through how to achieve this using Python.
Prerequisites
Before we start, ensure you have the following installed:
Python 3.x
python-docx
libraryre
(regular expressions) module
You can install the python-docx
library using pip:
pip install python-docx
Step-by-Step Guide
1. Import Necessary Libraries
First, import the necessary libraries:
from docx import Document
import re
2. Load the DOCX File
Next, load the .docx
file:
def load_docx(file_path):
try:
return Document(file_path)
except Exception as e:
print(f"Error loading document: {e}")
return None
doc = load_docx('path/to/your/document.docx')
if not doc:
exit()
3. Extract Text from the DOCX File
We need to extract all the text from the document. The following function iterates through all the paragraphs and tables in the document to extract text:
def extract_text(doc):
full_text = []
for para in doc.paragraphs:
full_text.append(para.text)
for table in doc.tables:
for row in table.rows:
for cell in row.cells:
full_text.append(cell.text)
return '\n'.join(full_text)
document_text = extract_text(doc)
4. Extract Email Addresses
Using regular expressions, we can search for email addresses in the extracted text:
def extract_emails(text):
email_pattern = r'[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}'
emails = re.findall(email_pattern, text)
return emails
emails = extract_emails(document_text)
print("Extracted Emails:", emails)
5. Extract Other Important Information
You can extend the use of regular expressions to extract other types of information, such as phone numbers, dates, or URLs. Here are some examples:
- Phone Numbers:
def extract_phone_numbers(text):
phone_pattern = r'\(?\d{3}\)?[-.\s]?\d{3}[-.\s]?\d{4}'
phone_numbers = re.findall(phone_pattern, text)
return phone_numbers
phone_numbers = extract_phone_numbers(document_text)
print("Extracted Phone Numbers:", phone_numbers)
- Dates:
def extract_dates(text):
date_pattern = r'\b\d{1,2}[/-]\d{1,2}[/-]\d{2,4}\b'
dates = re.findall(date_pattern, text)
return dates
dates = extract_dates(document_text)
print("Extracted Dates:", dates)
- URLs:
def extract_urls(text):
url_pattern = r'http[s]?://(?:[a-zA-Z]|[0-9]|[$-_@.&+]|[!*\\(\\),]|(?:%[0-9a-fA-F][0-9a-fA-F]))+'
urls = re.findall(url_pattern, text)
return urls
urls = extract_urls(document_text)
print("Extracted URLs:", urls)
Handling Exceptions
When dealing with file operations, it's important to handle exceptions to ensure your program doesn't crash unexpectedly. The load_docx
function already includes exception handling for loading the document. You can add similar handling for other parts of your code.
Optimizing the Extraction Process
For large documents, extracting text and running multiple regular expression searches can be time-consuming. Here are some tips to optimize the process:
Read Text in Chunks: Instead of reading the entire document at once, read and process text in chunks to reduce memory usage.
Compile Regular Expressions: Pre-compile your regular expressions to speed up repeated searches.
Here's an optimized version of the email extraction function:
def extract_emails_optimized(text):
email_pattern = re.compile(r'[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}')
return email_pattern.findall(text)
emails = extract_emails_optimized(document_text)
print("Extracted Emails:", emails)
Potential Use Cases
Extracting emails and other information from .docx
files has a wide range of applications:
Data Mining: Extracting contact information for marketing or research purposes.
Document Analysis: Analyzing legal documents, contracts, or reports for specific information.
Automation: Automating the extraction of key details from resumes or applications.
Compliance: Ensuring documents comply with data privacy regulations by identifying and handling sensitive information.
Conclusion
.docx
file using Python. By leveraging the python-docx
library and regular expressions, you can efficiently parse documents and retrieve valuable data. This skill is particularly useful for data analysis, automation, and information retrieval tasks.Feel free to modify and expand upon this script to suit your specific needs. Happy coding!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
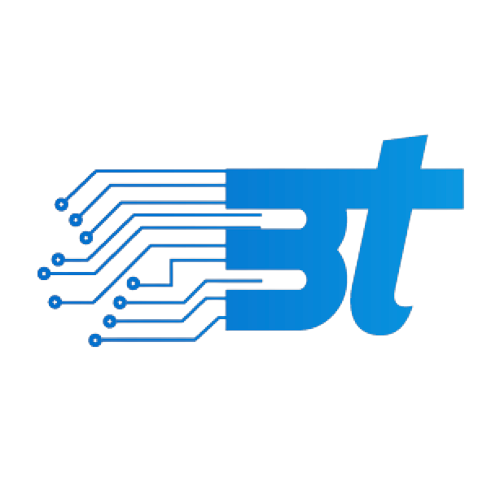
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.