Mastering ReactJS: How the Virtual DOM Efficiently Updates the Real DOM
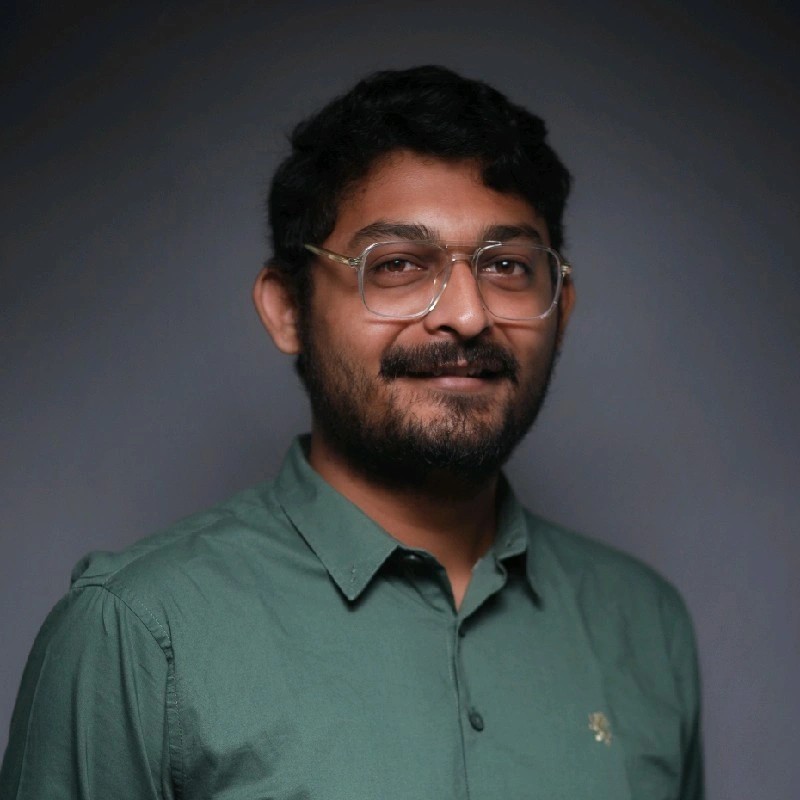
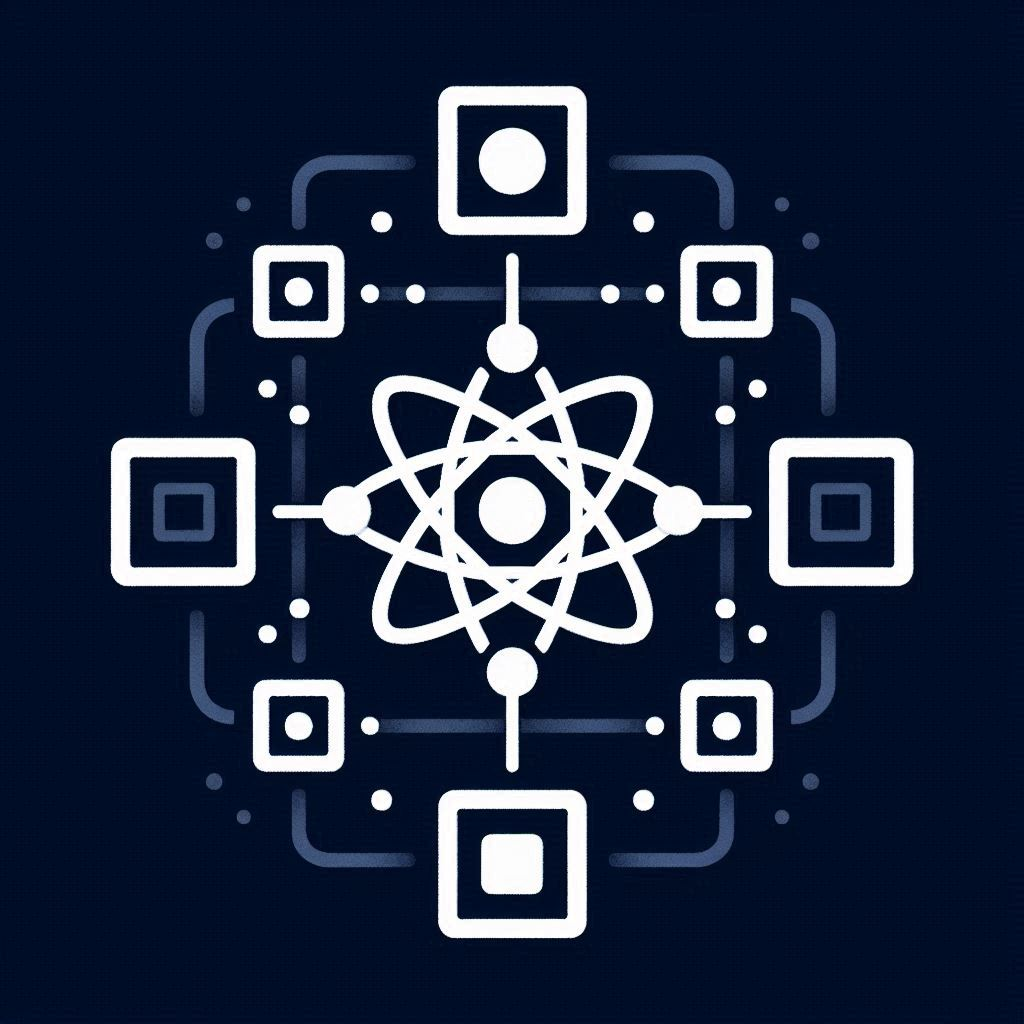
ReactJS has revolutionized front-end development with its efficient and declarative approach to building user interfaces. At the core of React's performance and simplicity is the concept of the Virtual DOM (VDOM). This article delves into the intricacies of the Virtual DOM, how it updates the real DOM, providing a comprehensive understanding of how React works internally.
What is the Virtual DOM?
The Virtual DOM is a lightweight, in-memory representation of the real DOM. Instead of directly manipulating the real DOM, which can be slow and inefficient, React creates a Virtual DOM to keep track of changes. When the state of a component changes, React updates the Virtual DOM first, then compares it with the previous version using a process called "reconciliation." This allows React to determine the minimal set of changes needed to update the real DOM efficiently.
How the Virtual DOM Updates the Real DOM
Initial Rendering:
When a React application is first rendered, React creates a Virtual DOM tree based on the initial component states.
This Virtual DOM tree is then used to generate the real DOM, which is displayed in the browser.
State Changes and Re-rendering:
When a component's state or props change, React triggers a re-render of that component.
A new Virtual DOM tree is created based on the updated state or props.
Diffing Algorithm:
React compares the new Virtual DOM tree with the previous one using a diffing algorithm.
This algorithm identifies the differences (or "diffs") between the two trees.
Reconciliation:
React determines the minimal set of changes required to update the real DOM to match the new Virtual DOM.
This process is called reconciliation and ensures that only the necessary updates are made, improving performance.
Updating the Real DOM:
React updates the real DOM based on the changes identified during reconciliation.
These updates are batched together to minimize the number of changes to the real DOM, further enhancing performance.
Let's dive deep and understand the DOM Updation Process
Element Creation:
React elements are the building blocks of React applications. They are plain JavaScript objects that describe what you want to see on the screen.
For example:
const element = <h1>Hello, world!</h1>;
Rendering:
When a component is rendered, React creates a new Virtual DOM tree representing the UI at that point in time.
ReactDOM.render() is used to render a React element into the real DOM:
ReactDOM.render(element, document.getElementById('root'));
Diffing and Reconciliation:
React's diffing algorithm compares the new Virtual DOM tree with the previous one to determine the minimal set of changes needed to update the real DOM.
React uses a heuristic approach to identify changes in the Virtual DOM efficiently, focusing on changes at the component level and using keys to optimize updates for lists of elements.
Commit Phase:
Once the changes are identified, React commits these changes to the real DOM in a batch to optimize performance.
React batches updates to reduce the number of updates and reflows in the real DOM, which can be expensive operations.
Fiber Architecture:
React's fiber architecture enables efficient reconciliation by breaking the work into units of work, allowing React to pause and resume work as needed. This helps in maintaining a smooth user experience even in complex applications.
Fiber allows React to split rendering work into chunks and spread it out over multiple frames, preventing the UI from becoming unresponsive.
Conclusion
React's Virtual DOM and fiber architecture form a robust foundation for high-performance web apps. The Virtual DOM minimizes the costs of direct DOM manipulation, while the diffing algorithm and reconciliation ensure efficient updates. Fiber architecture breaks rendering work into chunks, enhancing smoothness and responsiveness. Mastering these concepts is key to leveraging React's full potential for creating dynamic, high-performance interfaces.
Subscribe to my newsletter
Read articles from Divyesh Birawat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
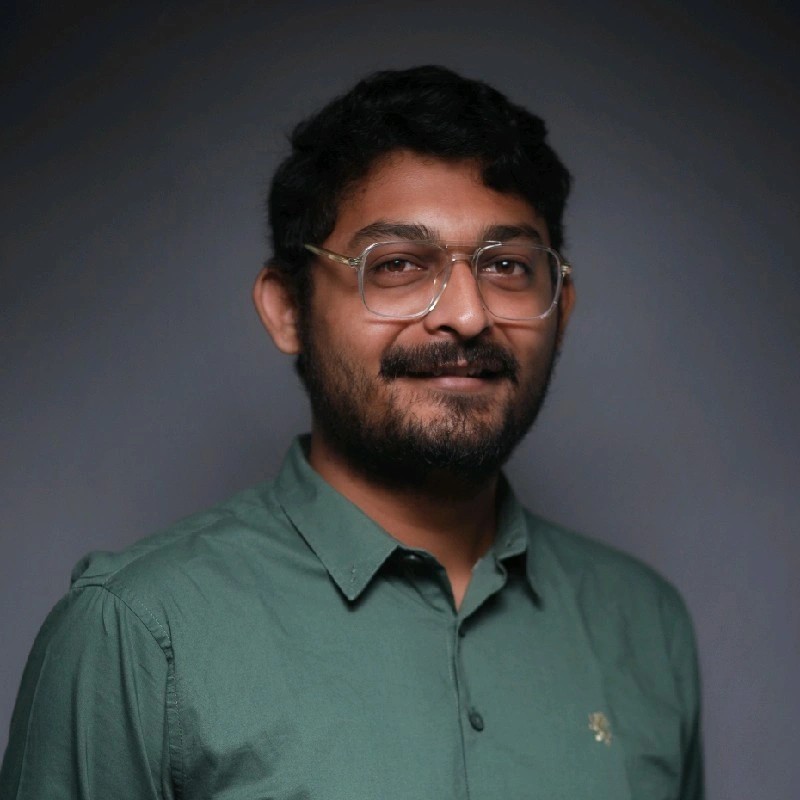
Divyesh Birawat
Divyesh Birawat
π¨βπ» Frontend Advocate | Tech Blogger | Dedicated to creating intuitive user experiences and optimizing web performance with ReactJS and Next.js. Letβs innovate and build exceptional applications together! π