PHP Variables and It's Scope

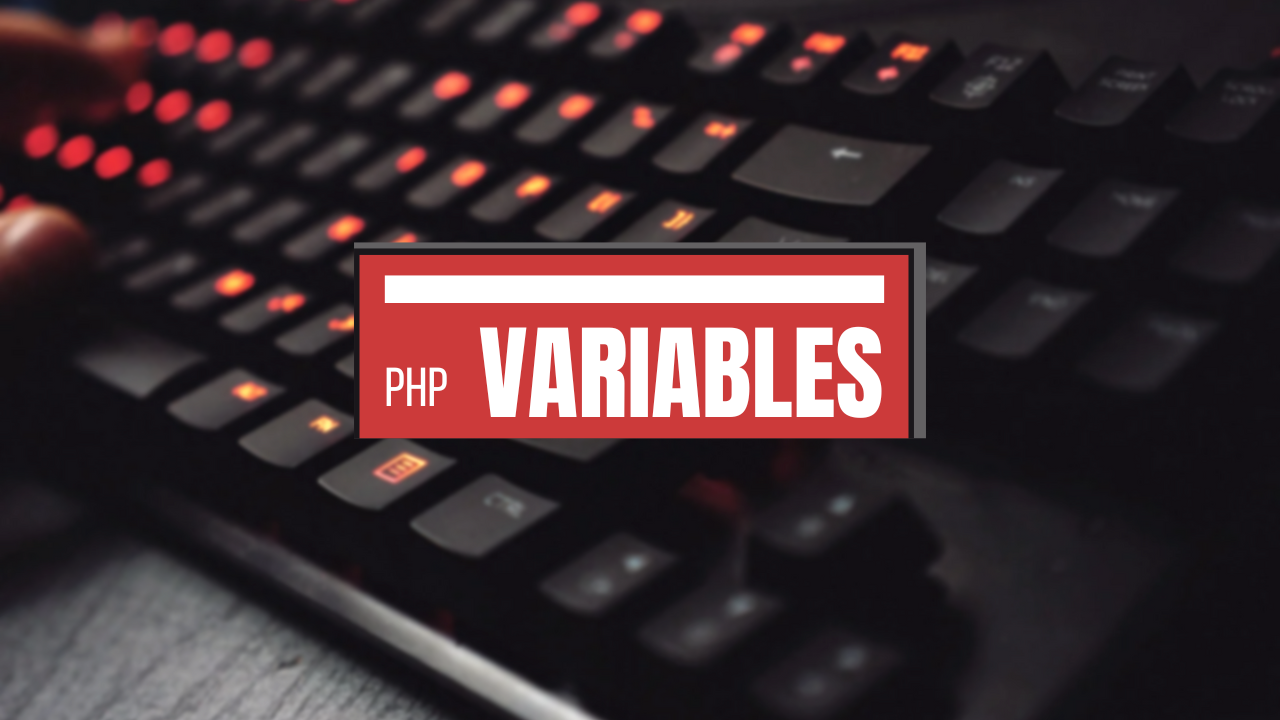
Today, we'll delve into the essentials of PHP variables, including their declaration, initialization, and scope. Understanding how to use variables effectively will allow you to store and manipulate data in your PHP scripts. Let's get started!
PHP Variables
Variables in PHP are used to store data, which can be used and manipulated throughout your script. Variables are declared using the $
sign followed by the variable name.
Variable Naming Rules:
Must start with a letter or underscore (
_
).Followed by any number of letters, numbers, or underscores.
Variable names are case-sensitive (
$name
and$Name
are different).
Do's and Don'ts for PHP Variable Naming Conventions
Do's | Don'ts |
Use meaningful and descriptive names. | Don't use single letters (unless for temporary variables). |
Use camelCase for variable names. | Don't use names starting with a number. |
Start with a letter or underscore. | Don't use special characters (e.g., ! , @ , # ). |
Follow a consistent naming convention. | Don't use reserved PHP keywords. |
Keep names concise but informative. | Don't use overly long variable names. |
Use underscores to separate words for better readability if not using camelCase. | Don't use mixed case or inconsistent casing. |
Use lowercase letters for variable names. | Don't use all capital letters (reserved for constants). |
Declaring and Initializing Variables:
<?php
// Valid Variables
$firstName = "John";
$userAge = 25;
$totalPrice = 99.99;
$isUserLoggedIn = true;
$user_details = array("name" => "John", "age" => 25);
// Invalid Variables (will throw an error)
$1name = "John"; // Starts with a number
$first-name = "John"; // Contains a hyphen
$firstName! = "John"; // Contains a special character
$IF = "value"; // Using a reserved keyword
// Valid but not recommended
$FirstName = "John"; // Inconsistent casing
$UserDetails = array(); // Mixed case
$totalprice = 99.99; // Not using camelCase or underscores
?>
Variable Scope
Variable scope refers to the context in which a variable is defined. PHP has three types of variable scope:
Local Scope
Global Scope
Static Scope
- Local Scope: Variables declared within a function are local to that function. This means you cannot access the variable's value outside of the function. If you try, it will throw an error.
<?php
function myFunction() {
$localVar = "I'm local";
echo $localVar;
}
myFunction(); // Outputs: I'm local
echo $localVar; // Error: Undefined variable $localVar
?>
In the example above, $localVar
is defined within myFunction
and is only accessible inside that function. Attempting to use $localVar
outside of myFunction
results in an error because the variable is not recognized in the global scope.
- Global Scope: Variables declared outside of any function are global. To access a global variable inside a function, you must use the
global
keyword.
<?php
$globalVar = "I'm global";
function myFunction() {
global $globalVar;
echo $globalVar;
}
myFunction(); // Outputs: I'm global
echo $globalVar; // Outputs: I'm global
?>
In this example, $globalVar
is defined in the global scope. Inside myFunction
, we use the global
keyword to access $globalVar
.
- Static Scope: Static variables retain their value between function calls. They are declared with the
static
keyword.
<?php
function myFunction() {
static $count = 0;
$count++;
echo $count;
}
myFunction(); // Outputs: 1
myFunction(); // Outputs: 2
myFunction(); // Outputs: 3
?>
Here, $count
is a static variable. Each time myFunction
is called, $count
retains its previous value and increments by 1.
Best Practices for PHP Variables
1. Use Meaningful Names: Choose descriptive names that clearly indicate the purpose of the variable.
$userAge = 25;
$totalPrice = 99.99;
2. Follow Naming Conventions: Use camelCase for variable names.
$firstName = "John";
$isUserLoggedIn = true;
3. Keep Scope Limited: Limit the scope of variables to where they are needed. This improves readability and reduces the chance of errors.
4. Avoid Using Global Variables: Minimize the use of global variables as they can lead to code that is difficult to debug and maintain.
Conclusion
Today, we explored PHP variables, including their declaration, initialization, and scope. We learned about local, global, and static scopes and discussed best practices for using variables in your PHP code. Understanding these concepts is crucial for writing effective and maintainable PHP scripts.
Subscribe to my newsletter
Read articles from Saurabh Damale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saurabh Damale
Saurabh Damale
Hello there! ๐ I'm Saurabh Kailas Damale, a passionate Full Stack Developer with a strong background in creating dynamic and responsive web applications. I thrive on turning complex problems into elegant solutions, and I'm always eager to learn new technologies and tools to enhance my development skills.