Git Branching and Pushing Guide
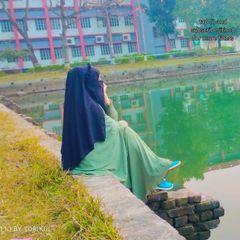
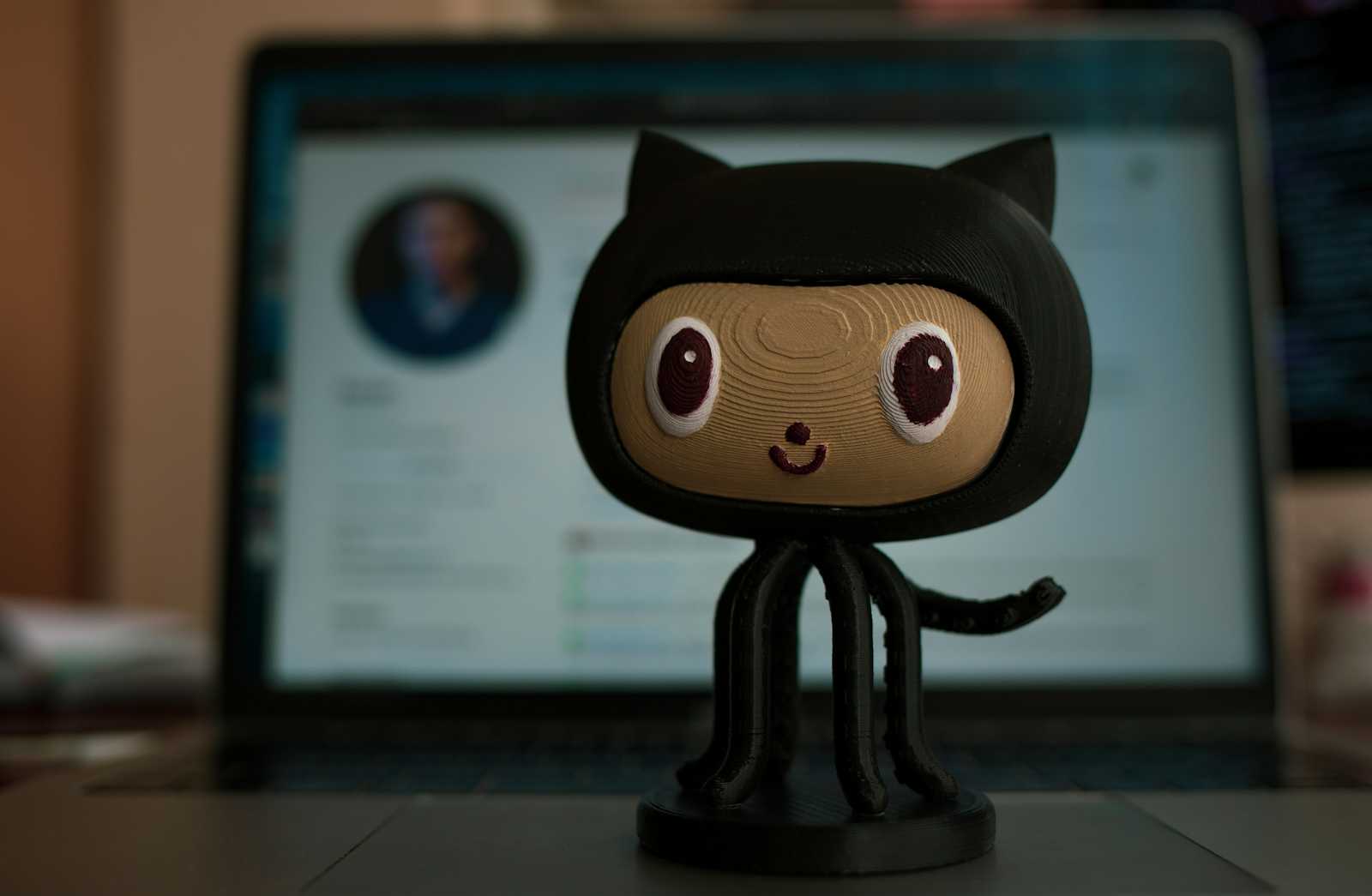
Checking BranchesChecking Branches To see all branches in your repository: ```bash git branch ``` ### Creating a New Branch To create a new branch: ```bash git branch <branch_name> ``` **Example:** ```bash git branch experimental ``` ### Switching Branches To switch to another branch: ```bash git checkout <branch_name> ``` **Example:** ```bash git checkout main ``` ### Deleting a Branch To delete a branch: ```bash git branch -d <branch_name> ``` **Example:** ```bash git branch -d experimental ``` ### Shortcut: Create and Switch to a New Branch To create a new branch and switch to it immediately: ```bash git checkout -b <branch_name> ``` **Example:** ```bash git checkout -b experimental-branch ``` ### Pushing to a Remote Branch To push a branch to the remote repository: ```bash git push origin <branch_name> ``` **Example:** ```bash git push origin experimental-branch ``` ### Detailed Explanation of `git push origin <branch_name>` When you push a branch to a remote repository, you are updating the remote repository with the changes in your local branch. Here’s a step-by-step explanation: 1. **Local Changes**: Make changes and commit them to your local branch. ```bash git add . git commit -m "Your commit message" ``` 2. **Push to Remote**: Push the changes from your local branch to the corresponding branch on the remote repository. ```bash git push origin <branch_name> ``` **Example:** ```bash git push origin experimental-branch ``` #### Explanation of Terms: - **origin**: This is the default name for your remote repository. When you clone a repository, Git automatically names it `origin` unless you specify otherwise. - **<branch_name>**: This is the name of the branch you want to push. ### Branching Workflow Examples #### Example 1: Feature Branch Workflow **Scenario:** You are working on a project and need to add a new feature. You want to develop this feature in isolation and then merge it into the main branch once it's complete. 1. **Clone the Repository** ```bash git clone https://github.com/your-username/your-repo.git cd your-repo ``` 2. **Create a New Branch for the Feature** ```bash git checkout -b feature/new-feature ``` 3. **Work on the Feature** Make your changes and commit them: ```bash git add . git commit -m "Add new feature" ``` 4. **Regularly Sync with the Main Branch** Before merging, ensure your branch is up-to-date: ```bash git checkout main git pull origin main git checkout feature/new-feature git merge main ``` Resolve any conflicts if they arise. 5. **Push the Feature Branch to the Remote Repository** ```bash git push origin feature/new-feature ``` 6. **Create a Pull Request** Go to your repository on GitHub (or your preferred Git hosting service), and create a pull request from `feature/new-feature` to `main`. 7. **Review and Merge the Pull Request** Once the pull request is approved, merge it into the main branch. You can do this via the GitHub interface. 8. **Delete the Feature Branch** After merging, delete the branch locally and remotely: ```bash git branch -d feature/new-feature git push origin --delete feature/new-feature ``` #### Example 2: Hotfix Workflow **Scenario:** A critical bug is found in the production code that needs immediate fixing. 1. **Clone the Repository** ```bash git clone https://github.com/your-username/your-repo.git cd your-repo ``` 2. **Create a Hotfix Branch** ```bash git checkout -b hotfix/critical-bug ``` 3. **Fix the Bug** Make your changes and commit them: ```bash git add . git commit -m "Fix critical bug" ``` 4. **Push the Hotfix Branch to the Remote Repository** ```bash git push origin hotfix/critical-bug ``` 5. **Create a Pull Request** Go to your repository on GitHub and create a pull request from `hotfix/critical-bug` to `main`. 6. **Review and Merge the Pull Request** Once the pull request is approved, merge it into the main branch. 7. **Sync with the Main Branch** After merging, update your local main branch: ```bash git checkout main git pull origin main ``` 8. **Delete the Hotfix Branch** ```bash git branch -d hotfix/critical-bug git push origin --delete hotfix/critical-bug ``` ### Conclusion By following this guide, you can effectively manage branches in your Git repository and ensure smooth collaboration with your team. Whether you are developing new features, fixing critical bugs, or maintaining a stable production environment, Git branching workflows provide a structured approach to version control and code management.
Subscribe to my newsletter
Read articles from Jaynab Binte Azad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
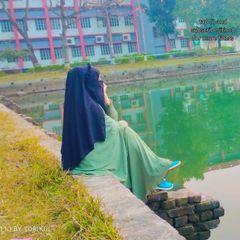
Jaynab Binte Azad
Jaynab Binte Azad
About Me Hi, I'm Jaynab Khatun, a passionate web developer with a keen interest in creating dynamic and engaging web applications. With a strong foundation in HTML, CSS, and JavaScript, I specialize in building scalable solutions using modern technologies like Node.js, React.js, MongoDB, and Next.js. My Journey My journey in web development began with a fascination for how the web works, leading me to dive deep into front-end and back-end technologies. Over the years, I've honed my skills through various projects and continuous learning, always striving to stay updated with the latest trends and best practices in the industry. Skills Front-End Development: HTML, CSS, JavaScript, React.js Back-End Development: Node.js, Express.js Database Management: MongoDB Full-Stack Development: Next.js What I Do I enjoy crafting intuitive and responsive user interfaces, ensuring seamless user experiences. On the back end, I focus on creating robust and efficient server-side applications. My goal is to bridge the gap between design and functionality, delivering products that not only look great but perform exceptionally well. Let's Connect I'm always eager to connect with fellow developers, share knowledge, and collaborate on exciting projects. Feel free to reach out to me here or connect with me on LinkedIn.