Level Up Your JavaScript: Mastering Higher-Order Functions
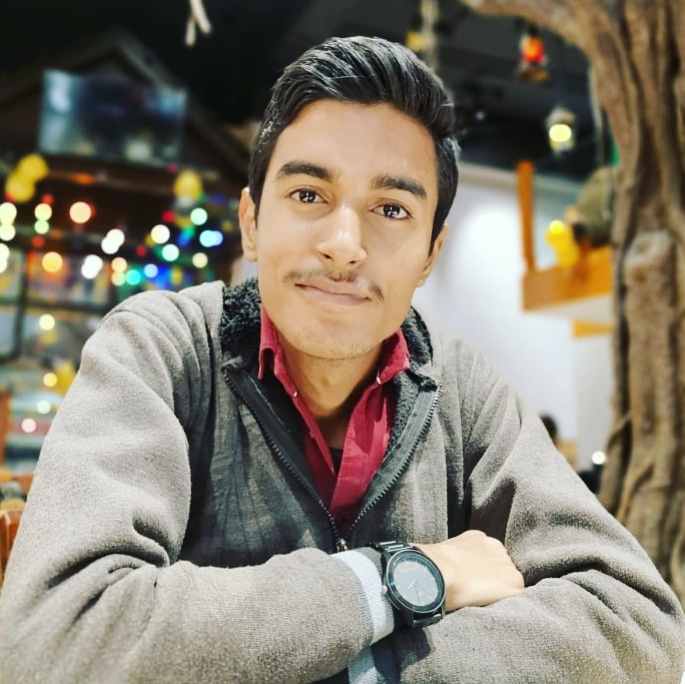
Table of contents
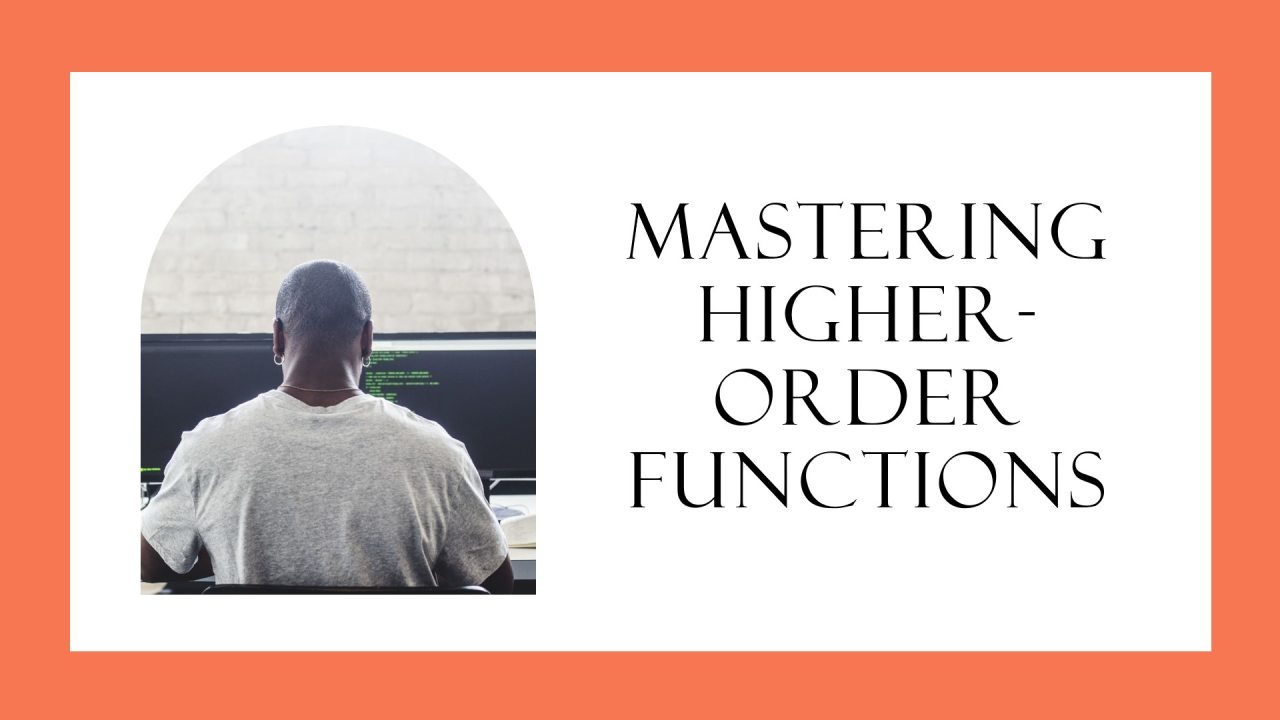
JavaScript is a powerful and versatile language, and higher-order functions are a key feature that unlocks its true potential. But what exactly are higher-order functions, and why should you care?
In essence, higher-order functions are functions that deal with other functions. They can either:
Accept functions as arguments: These functions are often called callbacks, and they allow you to pass specific logic into the higher-order function for execution.
Return a new function: This lets you create reusable functional patterns that can be adapted to different situations.
Here's why higher-order functions are awesome:
Cleaner, More Readable Code: By encapsulating common operations in higher-order functions, you avoid repetitive code blocks and make your code more concise and easier to understand.
Improved Reusability: Higher-order functions promote code reusability. You can create a single function that works with various data sets or functionalities by using callbacks or returning functions.
Functional Programming Style: Higher-order functions are a cornerstone of functional programming, a paradigm that emphasizes immutability and pure functions. This can lead to more predictable and maintainable code.
Now, let's explore some popular higher-order functions in JavaScript:
Unveiling the Powerhouse: Popular Higher-Order Functions
- map()
The map()
function is a workhorse for transforming arrays. It takes a callback function as an argument and applies that function to each element in the original array. The result is a new array containing the transformed elements.
Here's an example of using map()
to double the values in an array:
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map(function(number) {
return number * 2;
});
console.log(doubledNumbers); // Output: [2, 4, 6, 8, 10]
In this example, the callback function function(number) { return number * 2; }
takes a number as input and returns it multiplied by two. The map()
function applies this logic to each element in the numbers
array, creating the doubledNumbers
array.
- filter()
The filter()
function creates a new array containing only elements that pass a test implemented by the provided function.
Here's an example of using filter()
to get only the even numbers from an array:
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(function(number) {
return number % 2 === 0;
});
console.log(evenNumbers); // Output: [2, 4]
The callback function function(number) { return number % 2 === 0; }
checks if a number is even by using the modulo operator (%
). The filter()
function includes only elements that return true
from the callback in the new array.
- reduce()
The reduce()
function applies a function against an accumulator and each element in the array to reduce it to a single value. It's particularly useful for performing calculations or combining elements.
Here's an example of using reduce()
to sum all the elements in an array:
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce(function(accumulator, number) {
return accumulator + number;
}, 0); // Initial value for the accumulator
console.log(sum); // Output: 15
The callback function function(accumulator, number) { return accumulator + number; }
takes two arguments: the accumulator (which holds the running total) and the current element. It adds them together and returns the new accumulator value. The initial value for the accumulator is set to 0
in this example.
These are just a few examples of higher-order functions in JavaScript. By mastering these functions, you'll open up a new world of possibilities in your coding. There are many resources available online to delve deeper into each function and explore more advanced concepts like function composition. So, start incorporating higher-order functions into your projects and experience the power and elegance of functional JavaScript!
Subscribe to my newsletter
Read articles from Abhishek Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
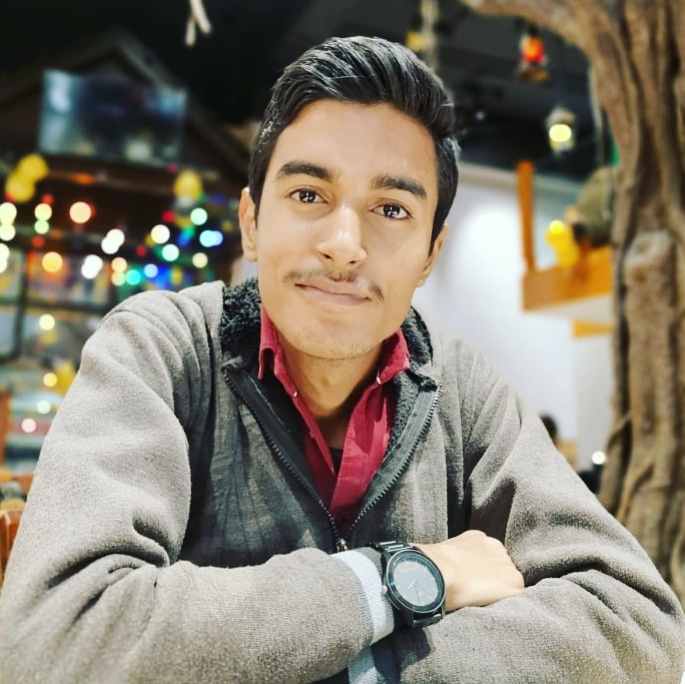
Abhishek Sharma
Abhishek Sharma
Abhishek is a designer, developer, and gaming enthusiast! He love creating things, whether it's building websites, designing interfaces, or conquering virtual worlds. With a passion for technology and its impact on the future, He is curious about how AI can be used to make work better and play even more fun.