🚀 Mastering Spring Data JPA: Implementing @OneToMany Mapping with User and MobilePhone Entities
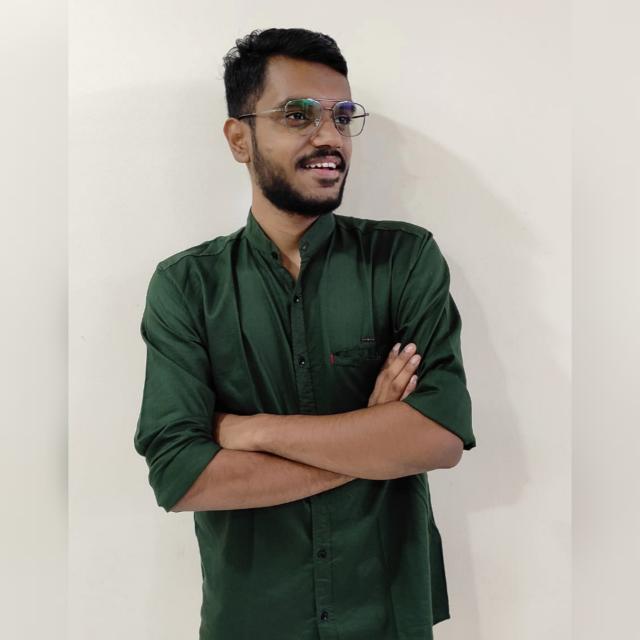
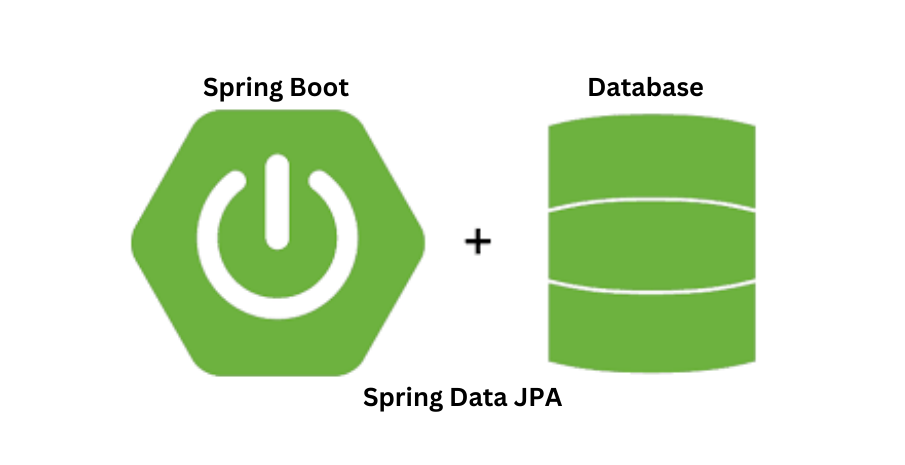
In my previous guide, we explored @OneToOne mapping between User and AadharCard entities. Now, we’ll move on to @OneToMany mapping between User and MobilePhone entities in Spring Data JPA. This guide will show you how to efficiently manage one-to-many relationships in your Spring Boot applications.
Understanding @OneToMany Mapping
@OneToMany mapping with User and MobilePhone means one user can have multiple mobile phones associated with them. For example, a User entity may be associated with several MobilePhone entities, each representing a different mobile phone number or device owned by that user. This relationship allows for efficient management and retrieval of data related to a user's mobile phones within a Spring Boot application.
Prerequisites
Before we dive into the code, make sure you have:
Set up your Spring Boot project with dependencies for Spring Data JPA.
Defined your User and MobilePhone entities with appropriate annotations.
Implementing @OneToMany Mapping
To implement @OneToMany mapping in JPA, follow these steps:
Create User and MobilePhone entities with proper annotations to establish the relationship.
User.java
package com.Spring_Data_Jpa.Entity;
import jakarta.persistence.*;
import lombok.Data;
import java.util.List;
@Entity
@Data
@Table(name = "user")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "id")
private long ID;
@Column(name = "name")
private String name;
@Column(name = "email")
private String email;
@OneToOne(cascade = CascadeType.ALL)
private AadharCard aadharCard;
@OneToMany(mappedBy = "user",cascade = CascadeType.ALL)
private List<MobilePhone> mobilePhones;
}
MobilePhone.java
package com.Spring_Data_Jpa.Entity;
import jakarta.persistence.*;
import lombok.Data;
@Entity
@Data
@Table(name="mobile_phone")
public class MobilePhone {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "id")
private long id;
@Column(name = "mobileNumber")
private long mobileNumber;
@ManyToOne
@JoinColumn(name = "user_id")
private User user;
}
Create a Repository interface for managing entities
MobilePhoneRepository
package com.Spring_Data_Jpa.Repository;
import com.Spring_Data_Jpa.Entity.MobilePhone;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface MobilePhoneRepository extends JpaRepository<MobilePhone,Long > {
}
Create a Controller class for handling requests related to User Entities
package com.Spring_Data_Jpa.Controller;
import com.Spring_Data_Jpa.Entity.AadharCard;
import com.Spring_Data_Jpa.Entity.MobilePhone;
import com.Spring_Data_Jpa.Entity.User;
import com.Spring_Data_Jpa.Repository.UserRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
@RestController
public class UserController {
@Autowired
UserRepository userRepository;
@PostMapping("/addUser")
public String addUser(@RequestBody User user, AadharCard aadharCard) {
if (user.getAadharCard() != null) {
aadharCard = user.getAadharCard();
aadharCard.setUser(user); // Set the user in the AadharCard
}
// Saving Mobile Numbers
if (user.getMobilePhones() != null) {
for (MobilePhone mobilePhone : user.getMobilePhones()) {
mobilePhone.setUser(user); // Set the user in each MobilePhone
}
}
userRepository.save(user);
return "User and AadharCard saved successfully!";
}
}
Testing the @OneToMany Mapping with Postman
Add User: POST /addUser
{
"name": "Mitchell S",
"email": "smitchell@gmail.com",
"aadharCard": {
"number": 619910001617
},
"mobilePhones": [
{ "mobileNumber": 8987286451 },
{ "mobileNumber": 197382611 }
]
}
Conclusion:
We've implemented one-to-many mappings between the User and MobilePhone entities. It's similar to how you might have one AadharCard linked to a single person. However, with mobile phones, it's the opposite: one user can have many mobile phone numbers. Our database keeps track of which phones belong to each user thanks to a special user ID stored in each mobile phone record. This makes it easy to find all the mobile numbers associated with a particular user in your application.
GitHub Access:
You can access the code on GitHub here. Feel free to fork the repository and try it out yourself.
About Me:
Subscribe to my newsletter
Read articles from Sumeet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
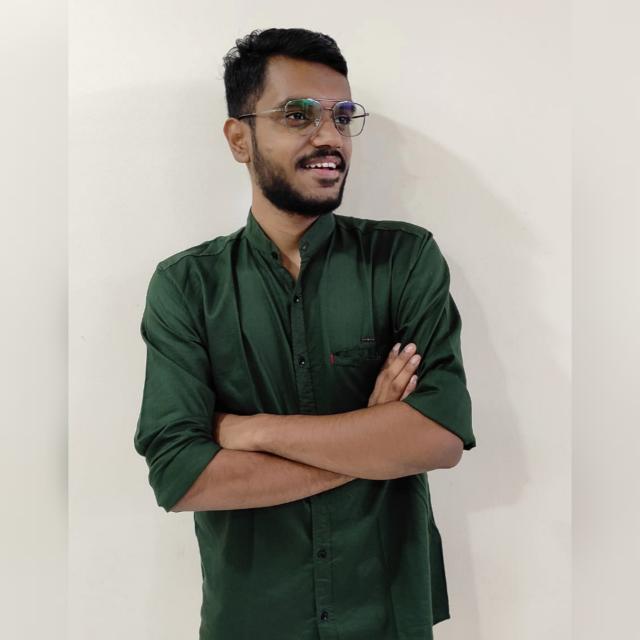