Timing Attacks: What They Are and How to Prevent Them
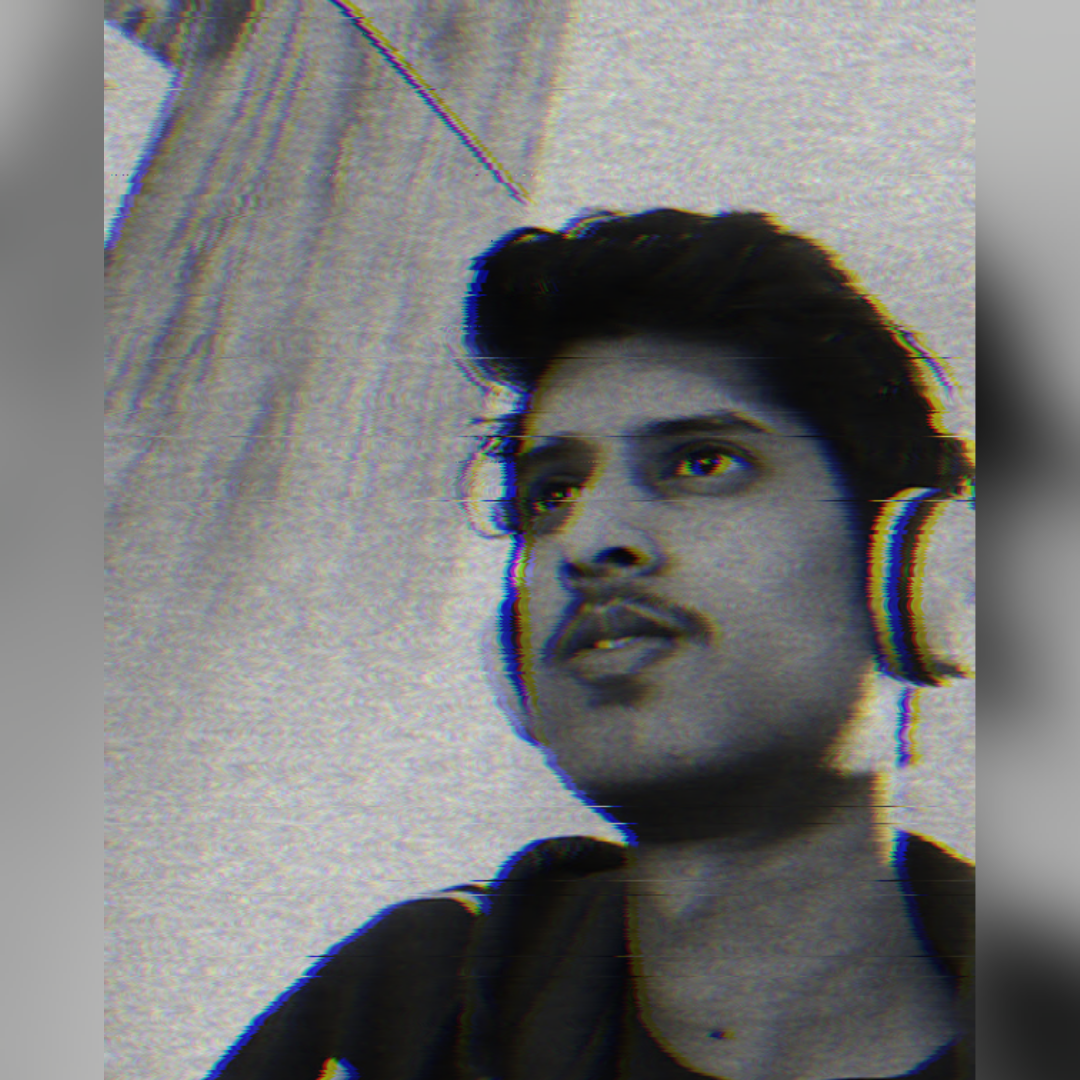
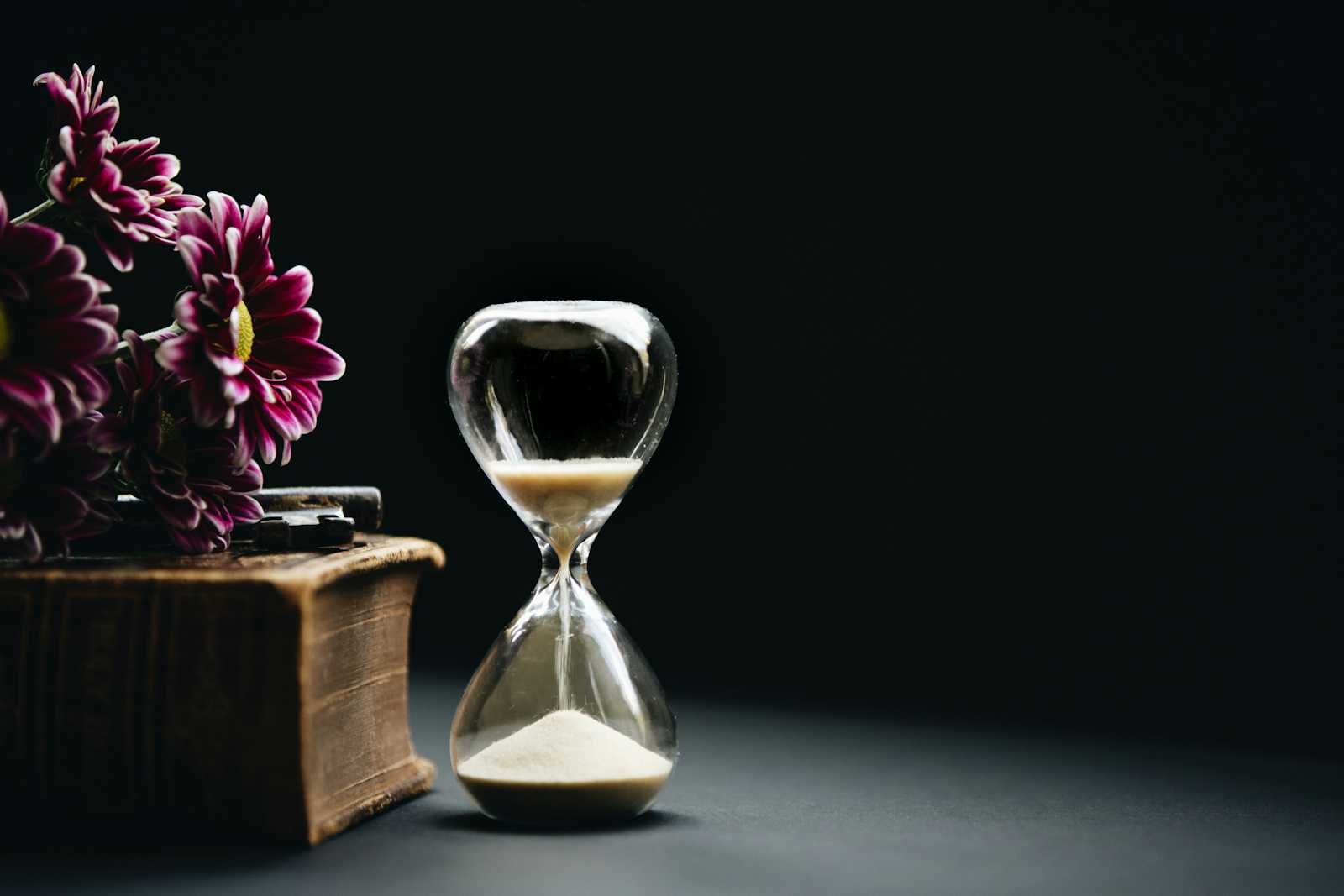
In the realm of cybersecurity, timing attacks represent a sophisticated method used by malicious actors to exploit vulnerabilities in software systems. These attacks capitalize on variations in program execution time to infer sensitive information, such as cryptographic keys or passwords. By carefully measuring how long specific operations take to complete, attackers can deduce crucial details and potentially compromise the security of a system.
How Timing Attacks Work
Timing attacks leverage the fact that the time taken to execute certain code segments can vary depending on the data being processed. For instance, when comparing strings or verifying cryptographic signatures, the comparison process might terminate early upon encountering a mismatch. This discrepancy in execution time can inadvertently leak information about the data being processed.
Vulnerable Code
var actualPassword = "mysecretpass"
func validatePassword(password string) bool {
if password==actualpassword {
return true
}
return false
}
Iteration | User-Provided Password ('x' chars below are non-relevant as comparison never reaches them) | Actual Password | Time Taken (Assuming it takes 1ms to compare one character) | Comment |
1 | mxxxxxxxxxxx | mysecretpass | 2ms | Fails at 2nd char comparison |
2 | myxxxxxxxxxx | mysecretpass | 3ms | Fails at 3rd char comparison |
3 | mysxxxxxxxxx | mysecretpass | 4ms | Fails at 4th char comparison |
4 | mysexxxxxxxx | mysecretpass | 5ms | Fails at 5th char comparison |
5 | mysecxxxxxxx | mysecretpass | 6ms | Fails at 6th char comparison |
6 | mysecrxxxxxx | mysecretpass | 7ms | Fails at 7th char comparison |
7 | mysecrexxxxx | mysecretpass | 8ms | Fails at 8th char comparison |
8 | mysecretxxxx | mysecretpass | 9ms | Fails at 9th char comparison |
9 | mysecretpxxx | mysecretpass | 10ms | Fails at 10th char comparison |
10 | mysecretpaxx | mysecretpass | 11ms | Fails at 11th char comparison |
11 | mysecretpasx | mysecretpass | 12ms | Fails at 12th char comparison |
12 | mysecretpass | mysecretpass | 13ms | Pass |
As seen above, once the first character in the input string matches the actual password, the string comparison takes twice as long, and the attacker now knows the first character of the password. The attacker notices that the time taken to respond increases with each correct character in the User-Provided
password. By analyzing the response times, the attacker can infer the correct password character by character.
Mitigating Timing Attacks with Constant Time Comparisons
One effective mitigation technique against timing attacks is the use of constant time comparisons. The idea behind constant time comparisons is to ensure that the comparison operation always takes the same amount of time, regardless of the data being compared. This prevents attackers from exploiting timing variations to gain information about the compared values.
Implementing Constant Time Comparisons in Golang
In Go (Golang), ensuring constant time comparisons can be achieved by using the crypto/subtle
package, which provides a ConstantTimeCompare
function specifically designed for this purpose. Here’s how you can implement it:
package main
import (
"crypto/subtle"
"fmt"
)
func secureCompare(a, b []byte) bool {
// ConstantTimeCompare returns 1 if the two slices are equal and 0 otherwise
return subtle.ConstantTimeCompare(a, b) == 1
}
func main() {
// Example usage
password := []byte("password123")
userInput := []byte("password123")
if secureCompare(password, userInput) {
fmt.Println("Passwords match!")
} else {
fmt.Println("Passwords do not match!")
}
}
In this example:
subtle.ConstantTimeCompare(a, b)
compares slicesa
andb
in constant time. This internally does the xor comparison on the bits and returns 1(true) if they are the same, else returns 0(false).The function returns
1
if the slices are equal and0
otherwise.This ensures that the comparison operation always takes the same amount of time, irrespective of the data being compared.
Note: There's also a practice to use subtle.ConstantTimeEq
along with subtle.ConstantTimeCompare
to first compare the length of two strings in constant time before comparing their content.
Conclusion
Timing attacks, although rare due to factors like network latency and machine resource variability, remain a significant threat in cybersecurity. By implementing techniques such as constant time comparisons, developers can substantially mitigate these risks. Prioritizing security-conscious coding practices and leveraging language-specific tools, like those provided in Go’s crypto/subtle package, is essential for building robust and resilient software systems. Staying informed about emerging attack vectors and evolving mitigation strategies is crucial in the ongoing battle to protect sensitive data and maintain trust in digital environments.
Subscribe to my newsletter
Read articles from Nihal Wasim directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
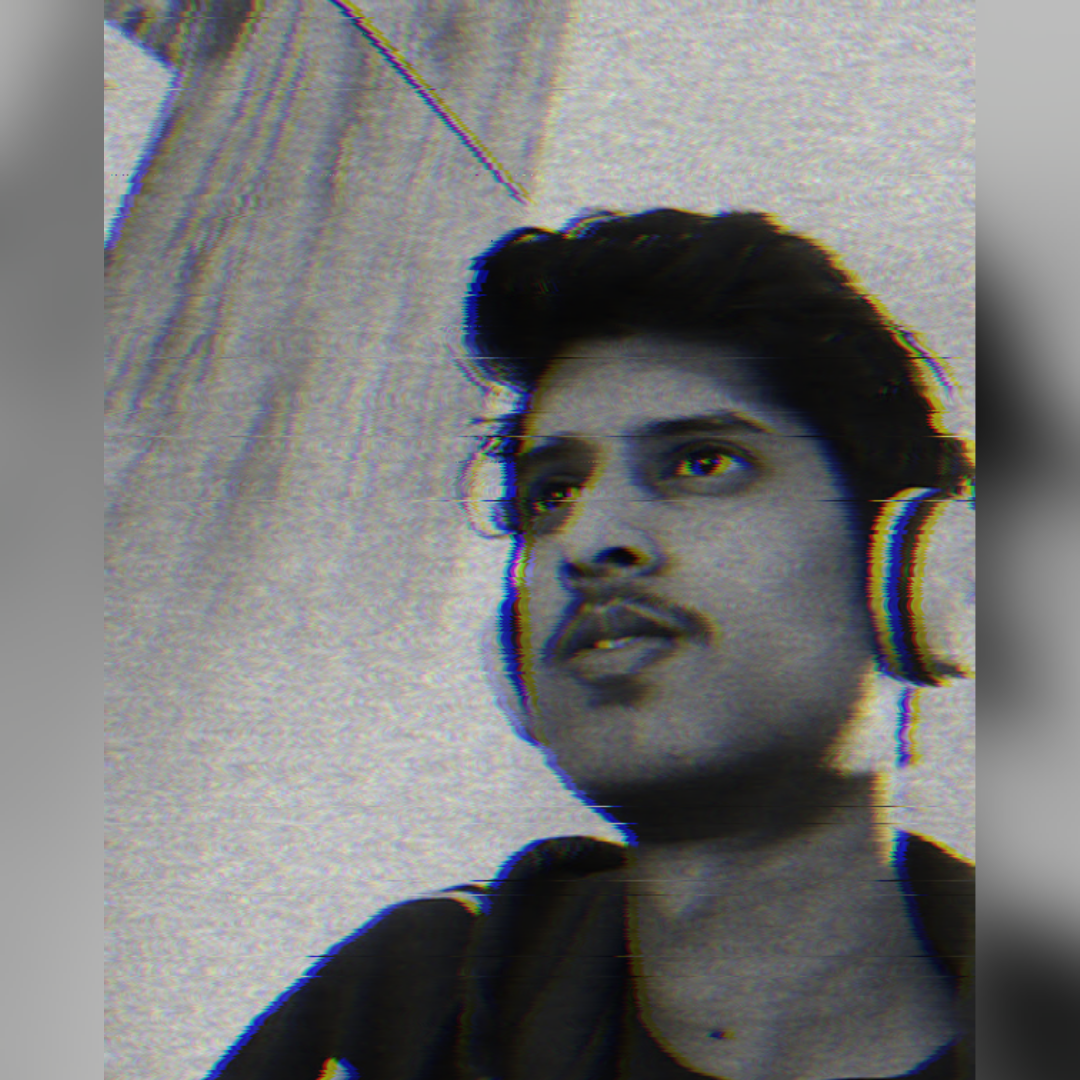