Mastering Error Tracking: Self-Hosting Sentry with Docker and Setting Up Sentry in Flutter
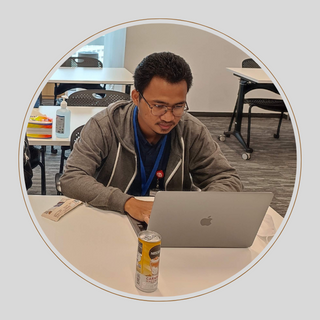
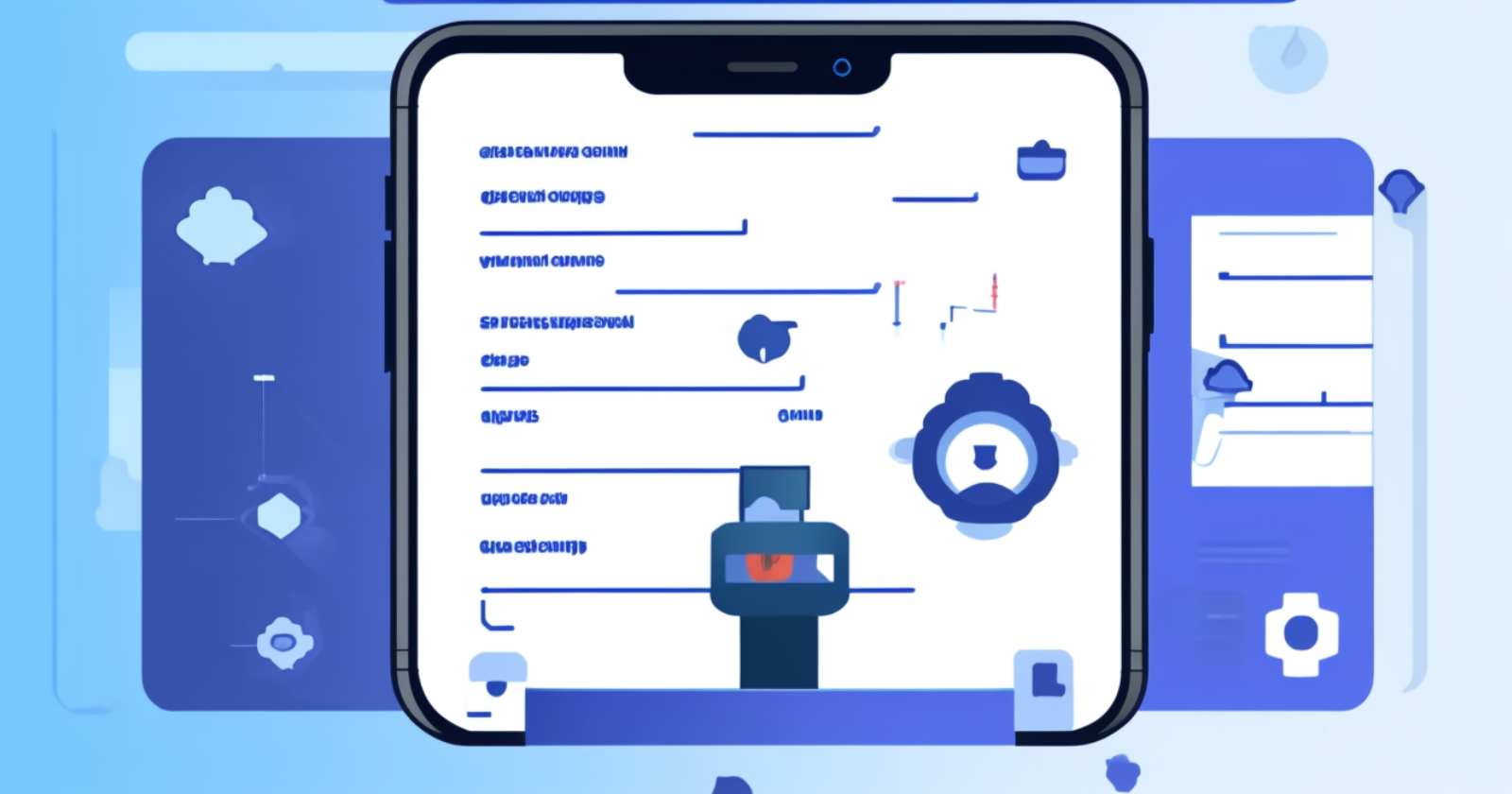
In today's fast-paced development environment, tracking errors effectively is crucial for maintaining high-quality applications. Sentry, an open-source error tracking and monitoring tool, offers powerful capabilities to help developers identify and resolve issues quickly. In this article, we'll explore how to self-host Sentry using Docker and how to set up Sentry in a Flutter application. By the end of this guide, you'll have a comprehensive understanding of leveraging Sentry for robust error tracking.
Self-Hosting Sentry with Docker
Why Self-Host Sentry?
Self-hosting Sentry allows you to maintain full control over your data and customize the setup according to your needs. It can also be more cost-effective for larger teams or projects.
Prerequisites
Docker and Docker Compose installed on your machine
Basic knowledge of Docker and command-line interface
Step-by-Step Guide to Self-Hosting Sentry
Step 1: Clone the Sentry On-Premise Repository
First, clone the official Sentry on-premise repository from GitHub:
git clone https://github.com/getsentry/onpremise.git
cd onpremise
Step 2: Configure Environment Variables
Sentry uses environment variables for configuration. Copy the example configuration file and customize it according to your needs:
cp .env.example .env
Edit the .env
file to configure settings like database credentials, Sentry URL, and email settings.
Step 3: Build and Run Sentry with Docker Compose
Use Docker Compose to build and run the Sentry services:
docker-compose build
docker-compose run --rm web upgrade
docker-compose up -d
The docker-compose build
command builds the Docker images, while docker-compose run --rm web upgrade
initializes the database. Finally, docker-compose up -d
starts the Sentry services in detached mode.
Step 4: Access Sentry
Once the services are running, you can access Sentry by navigating to http://localhost:9000
in your web browser. You will be prompted to create an initial user account and log in.
Setting Up Sentry in Flutter
Why Use Sentry in Flutter?
Integrating Sentry into your Flutter application allows you to capture and track errors, crashes, and performance issues in real-time, providing valuable insights to improve your app's stability and user experience.
Step-by-Step Guide to Setting Up Sentry in Flutter
Step 1: Add Sentry to Your Flutter Project
Add the sentry_flutter
package to your pubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
sentry_flutter: ^6.0.0
Run flutter pub get
to install the package.
Step 2: Initialize Sentry in Your Application
In your main.dart
file, initialize Sentry at the start of your application:
import 'package:flutter/material.dart';
import 'package:sentry_flutter/sentry_flutter.dart';
void main() async {
await SentryFlutter.init(
(options) {
options.dsn = 'YOUR_SENTRY_DSN';
},
appRunner: () => runApp(MyApp()),
);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
);
}
}
Replace 'YOUR_SENTRY_DSN'
with the DSN (Data Source Name) from your Sentry project settings.
Step 3: Capture Errors Manually
You can manually capture errors or exceptions using Sentry's API:
try {
throw Exception('Test exception');
} catch (exception, stackTrace) {
Sentry.captureException(
exception,
stackTrace: stackTrace,
);
}
Step 4: Testing the Integration
Run your Flutter application and trigger an error to verify that it is being captured by Sentry. Check your Sentry dashboard to see the recorded error.
Conclusion
By self-hosting Sentry with Docker, you gain complete control over your error tracking infrastructure, ensuring data privacy and customization. Integrating Sentry into your Flutter application enhances your ability to monitor and resolve errors efficiently, leading to a more stable and reliable app.
This guide has provided you with the necessary steps to set up and use Sentry effectively. Start leveraging Sentry today to improve your application's quality and user experience. Happy coding!
Subscribe to my newsletter
Read articles from Anaz S. Aji directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
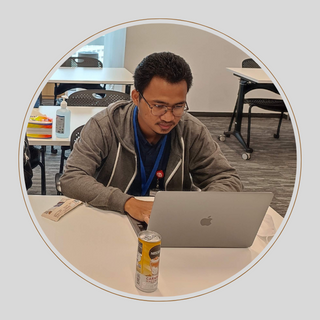
Anaz S. Aji
Anaz S. Aji
I am a dedicated Mobile Developer with a strong passion for exploring the latest advancements in technology and the dynamic world of cryptocurrency. My curiosity and enthusiasm drive me to continuously seek out and experiment with innovative solutions, ensuring that I stay at the forefront of industry trends and developments.