Firebase Realtime Database in ReactJS
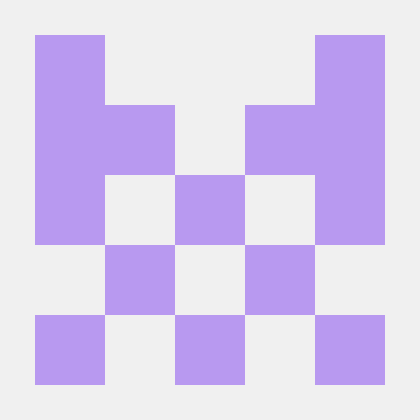
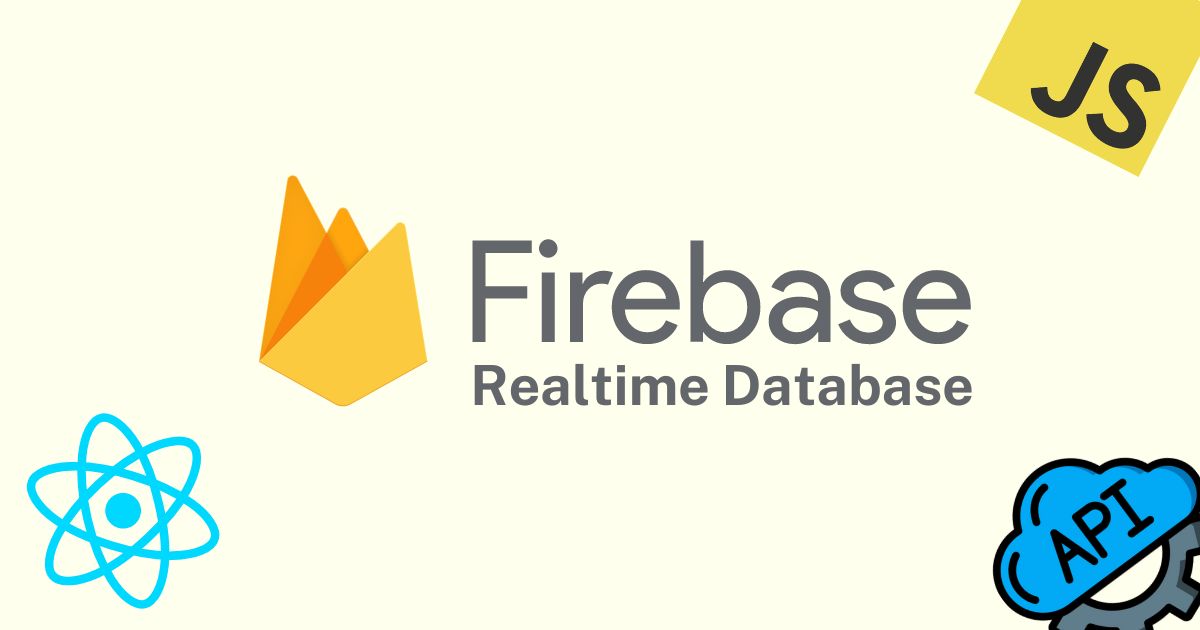
First, follow the initial four steps from the Firebase Authentication in ReactJS guide to create your Firebase project. For detailed instructions, refer to that article. In this article, I won't include every screenshot since the previous guide already covers them comprehensively. I highly recommend reading the Firebase Authentication in ReactJS article at least once.
Step 5: Create you React App using the below command line:
npx create-react-app your-project-name
After the project created, you should clean up the code.
NOTE: Add this script tag to your index.html to avoid red react.js icon in your browser:
<script src="https://unpkg.com/react@18/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@18/umd/react-dom.production.min.js"></script>
Install some of the dependencies like: react-router-dom, dotenv, firebase.
npm install react-router-dom dotenv firebase
Step 6: In your Project Overview and Add a web app to Register your web app.
Step 7: Click on the Build and click on the Realtime Database
Step 8: Click on the Create Database to store data in real time.
There are two steps in setting up a database:
Click on Next button in Realtime Database Location (changing is optional)
Select Start in locked mode in Security Rules. Click on the Enable button.
Change the read and write rules to true and click on Publish button.
Step 9: Go to the Project Settings and scroll down copy the code and paste in firebase.js inside src/components.
Add one more parameter in firbaseConfig called databaseURL. Copy the databaseURL from Realtime Database data:
// Import the functions you need from the SDKs you need
import { initializeApp } from "firebase/app";
// TODO: Add SDKs for Firebase products that you want to use
// https://firebase.google.com/docs/web/setup#available-libraries
// Your web app's Firebase configuration
const firebaseConfig = {
apiKey: process.env.REACT_APP_FIREBASE_API_KEY,
authDomain: process.env.REACT_APP_FIREBASE_AUTH_DOMAIN,
databaseURL: process.env.REACT_APP_FIREBASE_DATABASE_URL,
projectId: process.env.REACT_APP_FIREBASE_PROJECT_ID,
storageBucket: process.env.REACT_APP_FIREBASE_STORAGE_BUCKET,
messagingSenderId: process.env.REACT_APP_FIREBASE_MESSAGING_SENDER_ID,
appId: process.env.REACT_APP_FIREBASE_APP_ID,
measurementId: process.env.REACT_APP_FIREBASE_MEASUREMENT_ID
};
// Initialize Firebase
const app = initializeApp(firebaseConfig);
export default app;
Step 10: Create a .env file in src folder, and past all the key-value pair of firebaseConfig.
REACT_APP_FIREBASE_API_KEY="AIzaSyD**************-yIf68"
REACT_APP_FIREBASE_AUTH_DOMAIN="store-in-db.firebaseapp.com"
REACT_APP_FIREBASE_DATABASE_URL="https://store-in-db-default-rtdb.firebaseio.com/"
REACT_APP_FIREBASE_PROJECT_ID="store-in-db"
REACT_APP_FIREBASE_STORAGE_BUCKET="store-in-db.appspot.com"
REACT_APP_FIREBASE_MESSAGING_SENDER_ID="89********12"
REACT_APP_FIREBASE_APP_ID="1:8********2:web:6231**********33a8c869"
REACT_APP_FIREBASE_MEASUREMENT_ID=""
Note: Make sure you can put .env file inside the .gitignore file, so that .env file ignored during code pushing to GitHub.
Step 11: Create a Write.js file in components folder.
import React, { useState } from 'react'
import app from '../firebase'
import { getDatabase, ref, set, push } from "firebase/database";
function Write() {
const [inputText1, setInputText1] = useState("")
const [inputText2, setInputText2] = useState("")
const saveData = async () => {
const db = getDatabase(app);
const newDocRef = push(ref(db, "nature/fruits"))
set(newDocRef, {
fruitName: inputText1,
fruitDefinetion: inputText2
}).then(() => {
alert("Data saved successfully")
}).catch((error) => {
alert("error: ", error.message);
})
}
return (
<div>
<h1>WRITE/HOMEPAGE</h1>
<input type='text' value={title}
onChange={(e) => setTitle(e.target.value)} />
<input type='text' value={description}
onChange={(e) => setDescription(e.target.value)} /> <br />
<button onClick={saveData}>SAVE</button>
</div>
)
}
export default Write
Step 12: Change the codes in App.js file
import './App.css';
import { BrowserRouter as Router, Routes, Route } from 'react-router-dom';
import Write from './components/Write';
function App() {
return (
<div>
<Router>
<Routes>
<Route path='/' element={ <Write /> } />
<Route path='/write' element={ <Write /> } />
</Routes>
</Router>
</div>
);
}
export default App;
Congratulations, you are now able to store your data in Firebase Realtime Database. Test it is working properly or showing error. If it show an error then re-read the article and check where you did wrong.
Step 13: To read the data from Firebase Realtime Database. You should create a Read.js file in src/components.
import React, { useState } from 'react'
import app from '../firebase'
import { getDatabase, ref, get } from "firebase/database";
function Read() {
const [fruitArray, setFruitArray] = useState([]);
const fetchData = async () => {
const db = getDatabase(app);
const dbRef = ref(db, "nature/fruits");
const snapshot = await get(dbRef);
if(snapshot.exists()) {
setFruitArray(Object.values(snapshot.val()));
} else {
alert("error");
}
}
return (
<div>
<h1>READ</h1>
<button onClick={fetchData}> Display Data </button>
<ul>
{fruitArray.map( (item, index) => (
<li key={index}>
{item.title}: {item.description}
</li>
))}
</ul>
</div>
)
}
export default Read
Congratulations, you are now able to read your data from Firebase Realtime Database.
Step 14: To read and update the firebase data, we need to write this code:
import React, {useState} from 'react';
import app from "../firebase";
import { getDatabase, ref, get, remove } from "firebase/database";
function UpdateRead() {
let [fruitArray, setFruitArray] = useState([]);
const fetchData = async () => {
const db = getDatabase(app);
const dbRef = ref(db, "nature/fruits");
const snapshot = await get(dbRef);
if(snapshot.exists()) {
const myData = snapshot.val();
const temporaryArray = Object.keys(myData).map( myFireId => {
return {
...myData[myFireId],
fruitId: myFireId
}
} )
setFruitArray(temporaryArray);
} else {
alert("error");
}
}
const deleteFruit = async (fruitIdParam) => {
const db = getDatabase(app);
const dbRef = ref(db, "nature/fruits/"+fruitIdParam);
await remove(dbRef);
window.location.reload();
}
return (
<div>
<h1>UPDATE READ</h1>
<button onClick={fetchData}> Display Data </button>
<ul>
{fruitArray.map( (item, index) => (
<>
<li key={index}>
{item.fruitName}: {item.fruitDefinetion} : {item.fruitId}
<button className='button1' onClick={ () => navigate(`/updatewrite/${item.fruitId}`)}>UPDATE</button>
<button className='button1' onClick={ () => deleteFruit(item.fruitId)}>DELETE</button>
</li>
</>
) )}
</ul>
</div>
)
}
export default UpdateRead
Step 15: To update the firebase data.
import React, {useState} from 'react';
import app from "../firebase";
import { getDatabase, ref, get, remove } from "firebase/database";
function UpdateRead() {
let [fruitArray, setFruitArray] = useState([]);
const fetchData = async () => {
const db = getDatabase(app);
const dbRef = ref(db, "nature/fruits");
const snapshot = await get(dbRef);
if(snapshot.exists()) {
const myData = snapshot.val();
const temporaryArray = Object.keys(myData).map( myFireId => {
return {
...myData[myFireId],
fruitId: myFireId
}
} )
setFruitArray(temporaryArray);
} else {
alert("error");
}
}
const deleteFruit = async (fruitIdParam) => {
const db = getDatabase(app);
const dbRef = ref(db, "nature/fruits/"+fruitIdParam);
await remove(dbRef);
window.location.reload();
}
return (
<div>
<h1>UPDATE READ</h1>
<button onClick={fetchData}> Display Data </button>
<ul>
{fruitArray.map( (item, index) => (
<>
<li key={index}>
{item.fruitName}: {item.fruitDefinetion} : {item.fruitId}
<button className='button1' onClick={ () => navigate(`/updatewrite/${item.fruitId}`)}>UPDATE</button>
<button className='button1' onClick={ () => deleteFruit(item.fruitId)}>DELETE</button>
</li>
</>
) )}
</ul>
</div>
)
}
export default UpdateRead
Step 16: Update the App.js code to import remaining routes.
import './App.css';
import { BrowserRouter as Router, Routes, Route } from 'react-router-dom';
import Write from './components/Write';
import Read from './components/Read';
import UpdateRead from './components/UpdateRead';
import UpdateWrite from './components/UpdateWrite';
function App() {
return (
<div>
<Router>
<Routes>
<Route path='/' element={ <Write /> } />
<Route path='/write' element={ <Write /> } />
<Route path='/read' element={ <Read /> } />
<Route path='/updateread' element={ <UpdateRead /> } />
<Route path='/updatewrite/:firebaseId' element={ <UpdateWrite /> } />
</Routes>
</Router>
</div>
);
}
export default App;
Bravo! You were learned a CRUD operation in Firebase Realtime Database using ReactJS.
Credit goes to Abdul Hakim at Soft.Tomatoes
Subscribe to my newsletter
Read articles from Mohd Ahsan Raza Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
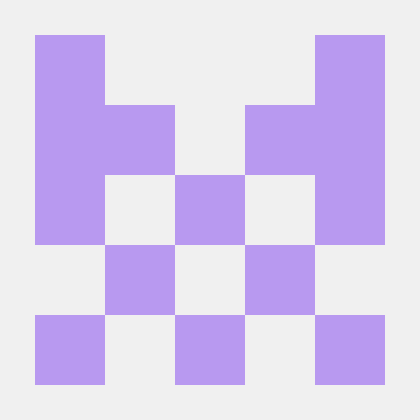
Mohd Ahsan Raza Khan
Mohd Ahsan Raza Khan
๐ Hi, I'm Mohd Ahsan Raza Khan! I know you might be thinking my name is quite long, right? Don't worry, you can call me MARK. I'm a software developer with a passion for building web applications and sharing knowledge through writing. Currently, I work as a Web Developer at Kepler Voice, where I specialize in full-stack development. I love exploring new technologies and frameworks, and I'm particularly interested in JavaScript, ReactJS, NodeJS, ExpressJS, and MongoDB. In my free time, I enjoy learning about new technologies and writing tutorials to help others learn and grow in their coding journey. I'm always excited to connect with fellow developers, so feel free to reach out to me on LinkedIn. Let's build something amazing together!