How to Create a File Organizer Project Using NodeJs

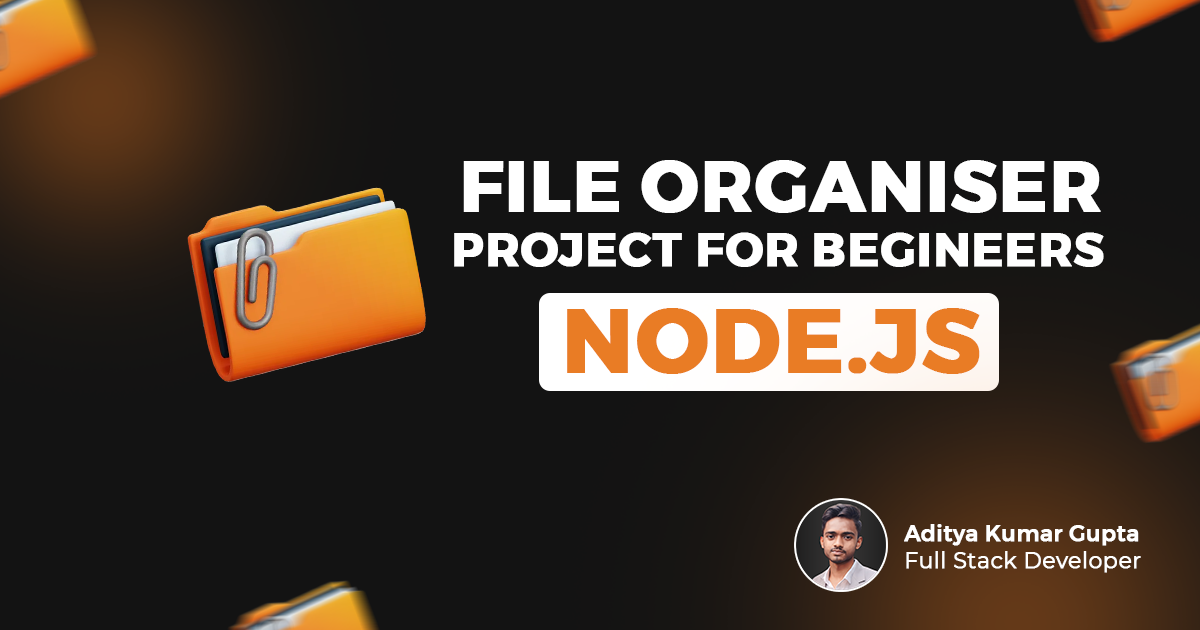
Github Repository Link
Do you struggle with organizing files? If so, you should check out this project! Today, I’m excited to share a file organizer project with you that helps manage your files by their specific extensions. This file organizer is made using Node.js and comes with a Command Line Interface.
To use this File Organizer, just set it up on your local system. Then, place all the files you want to organize in a folder.
After that, simply run the program. The File Organizer will take care of the rest by creating folders based on file extensions and moving each file to its corresponding folder.
Project Overview
This is a simple yet powerful file organizer project built with Node.js. It is a Command Line Interface (CLI) application that leverages the Path Module, FS Module, and FS Sync Module to perform its tasks efficiently.
The primary objective of this project is to help users manage their files by organizing each one into a folder named after its extension. This means that all PNG files will be moved to a folder named "PNG," JPEG files will be placed in a "JPEG" folder, and so on.
This method of organization makes it incredibly easy to locate specific types of files without having to sift through a cluttered directory. Whether you have a mix of documents, images, or other file types, this tool will sort them neatly, saving you time and effort in the long run.
Setting Up the Project in Your System
In this guide, we'll walk you through the steps to set up a project that organizes your files by their extensions using Node.js.
By following these instructions, you can easily manage and sort your mixed file types, saving you time and effort.
Step 1. Click the above FileOrganiser Github Repository Link.
Step 2. Then copy the code button.
Step 3. Then Copy the Github Repository Link.
Step 4. Open the terminal and paste the command given below.
git clone https://github.com/adityaguptareal/FileOrganiser.git
Step 5. Now open that folder
Step 6. Go to the Unorganised folder and paste all your cluttered files there.
Step 7. Now back to the folder and run the FileOrganiser.js file using vscode or terminal.
Step 8. After a few seconds, all your files will be sorted into folders based on their extensions.
How to Create a File Organizer in Node.js
Creating a File Organizer in Node.js is a practical project that helps manage and sort files based on their extensions. This project leverages Node.js's powerful modules to automate the organization of files, making it easier to locate specific types of files without manually sifting through a cluttered directory.
The File Organizer is a Command Line Interface (CLI) application that uses the Path Module, FS Module, and FS Sync Module to perform its tasks efficiently.
The primary objective is to move files into folders named after their extensions. For example, all PNG files will be moved to a "PNG" folder, JPEG files to a "JPEG" folder, and so on.
By following the steps outlined in this guide, you can set up the File Organizer on your local system, place all the files you want to organize in a designated folder and run the program.
The File Organizer will then create folders based on file extensions and move each file to its corresponding folder, saving you time and effort in managing your files.
Code Breakdown
Project Requirement
Nodejs
CodeEditor
FS Module
FS sync Module
Path Module.
Step 1. Import the important Module like Path, Fs, Fs/promises
import fs from "fs/promises"
import path from "path"
import fsn from "fs"
Step 2. Make a Variable that has a path to that folder
const folder="C:\\Users\\codea\\Documents\\Web Development\\File organiser\\Unorganised Files"
Note: Make sure to use extra "\" in the file path
Step 3. Create a File and use readdir( )
from the fs/promise module and add await so that it reads that directory and then moves to the next part of the code.
let files=await fs.readdir(folder)
Step 4. Now we will run a loop for each file that exists in that directory which we passed in the folder variable. Then we will make variables with that store file name, file path, file extension, target folder etc.
for (const file of files) {
let fileName=file
//uses path module with extaname function to get the file extension
let fileExtension=path.extname(file).split(".")[1]
let oldFilePath=path.join(folder,fileName)
//making a new path for the file extesnion use join method from the path module
let targetFolder=path.join(folder,fileExtension)
//checking if the file already exist or not
if(fsn.existsSync(targetFolder)){
// moving gile to new folder
fs.rename(oldFilePath,path.join(folder,fileExtension,fileName))
console.log('File Moved SuccesFully');
}
else{
//making directory then moving it to the new folder
fs.mkdir(targetFolder)
fs.rename(oldFilePath,path.join(folder,fileExtension,fileName))
}
console.log('Thanks For Using it');
}
Conclusion
In conclusion, creating a File Organizer using Node.js is a practical and efficient way to manage and sort files based on their extensions. By leveraging Node.js's powerful modules such as the Path Module, FS Module, and FS Sync Module, this project automates the organization of files, making it easier to locate specific types of files without manually sifting through a cluttered directory.
By following the steps outlined in this guide, you can set up the File Organizer on your local system, place all the files you want to organize in a designated folder and run the program.
The File Organizer will then create folders based on file extensions and move each file to its corresponding folder, saving you time and effort in managing your files. This project not only enhances productivity but also ensures a more organized and efficient file management system.
Project Link
Subscribe to my newsletter
Read articles from Aditya Kumar Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Aditya Kumar Gupta
Aditya Kumar Gupta
Hi there! I'm Aditya, a passionate Full-Stack Developer driven by a love for turning concepts into captivating digital experiences. With a blend of creativity and technical expertise, I specialize in crafting user-friendly websites and applications that leave a lasting impression. Let's connect and bring your digital vision to life!