Cracking the Code: Understanding Merge Sort Algorithm in Simple Steps
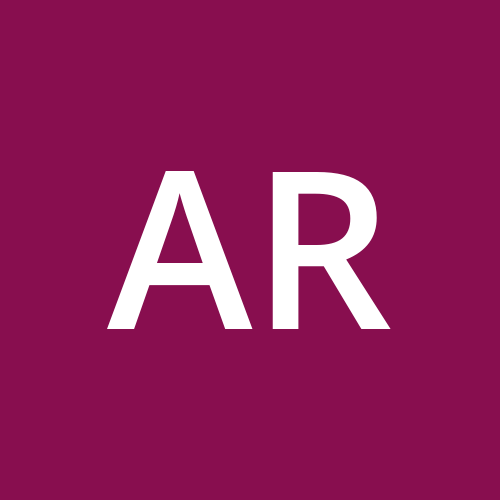
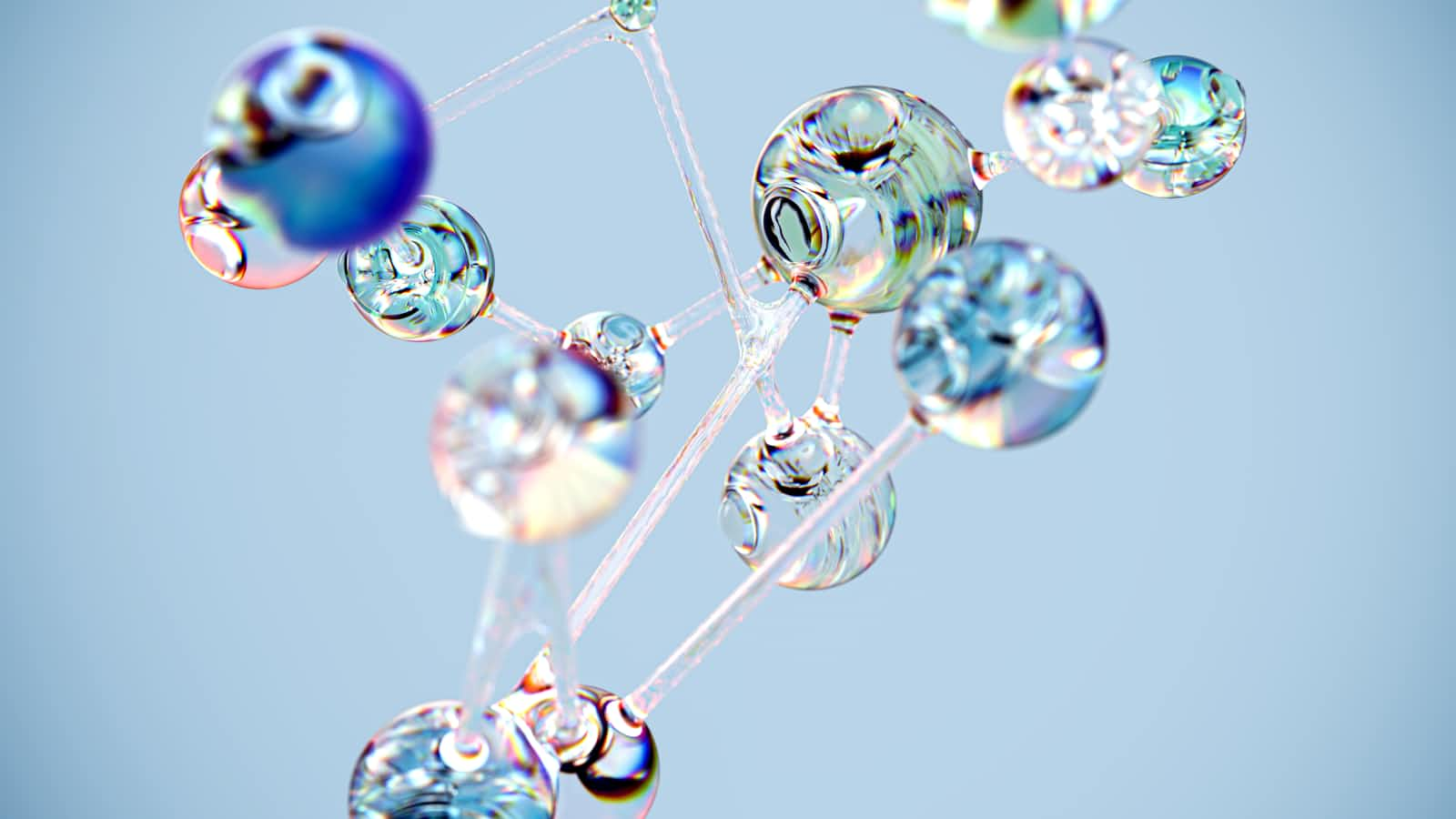
Introduction
Hey there, fellow coders! Have you ever tried to sort your toys or cards in order? Sorting is something we do all the time, and computers need to do it too. One of the coolest ways computers sort things is called Merge Sort. Today, we’re going to break down this awesome algorithm in a way that’s super easy to understand. Ready to dive into the world of coding magic? Let’s go!
What is Merge Sort?
Imagine you have a big pile of cards that you want to sort. Merge Sort is a special trick that helps you do it quickly by breaking the pile into smaller piles, sorting them, and then putting them back together. Here’s how it works in a nutshell:
Divide: Split the pile of cards into two smaller piles.
Conquer: Keep splitting those piles until you have lots of tiny piles with just one card each (since one card is always sorted!).
Merge: Start putting the piles back together in the right order until you have one big, sorted pile.
How Does Merge Sort Work?
Let’s walk through an example with pictures to make it easy:
Start with a Pile:
- Imagine you have a pile of cards: [5, 3, 8, 6, 2, 7, 4, 1].
Divide the Pile:
- Split it into two smaller piles: [5, 3, 8, 6] and [2, 7, 4, 1].
Divide Again:
- Split each smaller pile: [5, 3] and [8, 6], and [2, 7] and [4, 1].
Keep Dividing:
- Until you have single cards: [5], [3], [8], [6], [2], [7], [4], [1].
Merge:
Now, start putting them back together in order:
Merge [5] and [3] into [3, 5].
Merge [8] and [6] into [6, 8].
Merge [2] and [7] into [2, 7].
Merge [4] and [1] into [1, 4].
Keep Merging:
Merge the sorted piles again:
Merge [3, 5] and [6, 8] into [3, 5, 6, 8].
Merge [2, 7] and [1, 4] into [1, 2, 4, 7].
Final Merge:
- Merge the last two sorted piles into one big sorted pile: [1, 2, 3, 4, 5, 6, 7, 8].
And there you have it! Your cards are now sorted using Merge Sort!
Why is Merge Sort Cool?
Efficiency: Merge Sort is super-fast, especially for big piles of data. It’s like having a magic sorting wand!
Divide and Conquer: It breaks problems into smaller pieces, making them easier to solve.
Consistency: It always sorts in the same amount of time, even if the data is jumbled up.
Merge Sort in Code
Here’s how you can write Merge Sort. Don’t worry if you don’t understand everything yet. Just see how we translate the card sorting trick into a language that computers understand:
JavaScript implementation:
const array = [5, 3, 8, 6, 2, 7, 4, 1];
function mergeSort(array) {
// Base case: arrays with fewer than 2 elements are already sorted
if (array.length < 2) {
return array;
}
// Split the array into two halves
const middle = Math.floor(array.length / 2); // Find middle point of the array
const leftArray = array.slice(0, middle); // Divide array from left to mid - left array
const rightArray = array.slice(middle); // Divide array from mid to right - right array
// Recursively sort both halves
const sortedLeft = mergeSort(leftArray); // Recursively sort the left half of the array until each subarray has only one element
const sortedRight = mergeSort(rightArray); // Recursively sort the right half of the array until each subarray has only one element
// Merge the sorted halves
return merge(sortedLeft, sortedRight); // Use helper function to sort and merge subarrays
}
function merge(left, right) {
const result = [];
let leftIndex = 0;
let rightIndex = 0;
// Compare elements from both arrays and merge them in sorted order
while (leftIndex < left.length && rightIndex < right.length) { // As long as left and right index do not exceed subarray length
if (left[leftIndex] < right[rightIndex]) {
result.push(left[leftIndex]);
leftIndex++; // Move left array index to the right to check the next value
} else {
result.push(right[rightIndex]);
rightIndex++; // Move right array index to the right to check the next value
}
}
// Concatenate the remaining elements (if any)
return result.concat(left.slice(leftIndex)).concat(right.slice(rightIndex)); // First concatenate the right array to the left and then concatenate it to the result array
}
Python implementation:
array = [5, 3, 8, 6, 2, 7, 4, 1]
def merge_sort(array):
# Base case: arrays with fewer than 2 elements are already sorted
if len(array) < 2:
return array
# Split the array into two halves
middle = len(array) // 2
left_array = array[:middle]
right_array = array[middle:]
# Recursively sort both halves
sorted_left = merge_sort(left_array) # Recursively sort the left half of the array until each subarray has only one element
sorted_right = merge_sort(right_array) # Recursively sort the right half of the array until each subarray has only one element
# Merge the sorted halves
return merge(sorted_left, sorted_right)
def merge(left, right):
result = []
left_index = 0
right_index = 0
# Compare elements from both arrays and merge them in sorted order
while left_index < len(left) and right_index < len(right):
if left[left_index] < right[right_index]:
result.append(left[left_index])
left_index += 1
else:
result.append(right[right_index])
right_index += 1
# Concatenate the remaining elements (if any)
result.extend(left[left_index:])
result.extend(right[right_index:])
return result
More About Algorithms
If you enjoyed learning about Binary Search, you might also find these articles interesting:
Cracking the Code: Understanding Quick Sort Algorithm in Simple Steps
Bubble Sort Demystified: A Beginner's Guide to Sorting Algorithms
These posts will help you further explore different sorting algorithms and improve your coding skills!
Conclusion
Merge Sort is like a magic trick for sorting that makes big problems smaller and easier to handle. By breaking things down and putting them back together in order, you can sort anything quickly and efficiently. So next time you have a big pile of toys or cards, think of Merge Sort and how you can use its magic to get everything in order! Keep exploring and practicing, and soon you’ll be a master of sorting algorithms and more!
If you found this post helpful, please give it a like and share it with others who might benefit from it. Happy coding!
Subscribe to my newsletter
Read articles from Artur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
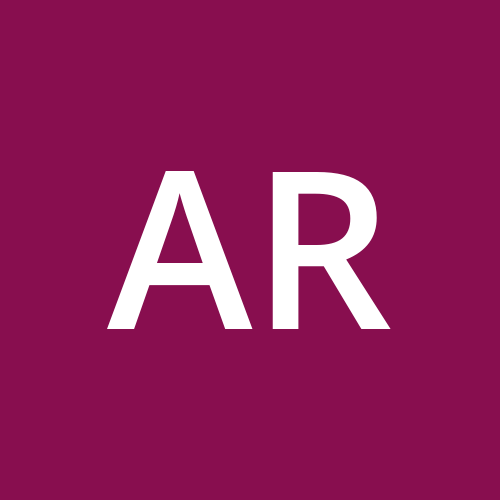