Guide to Reentrancy Vulnerabilities in Solidity : Attack #02
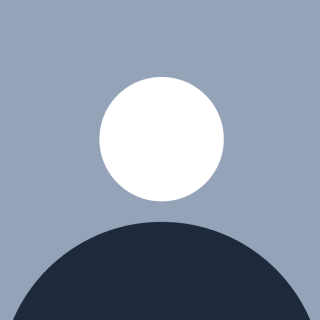
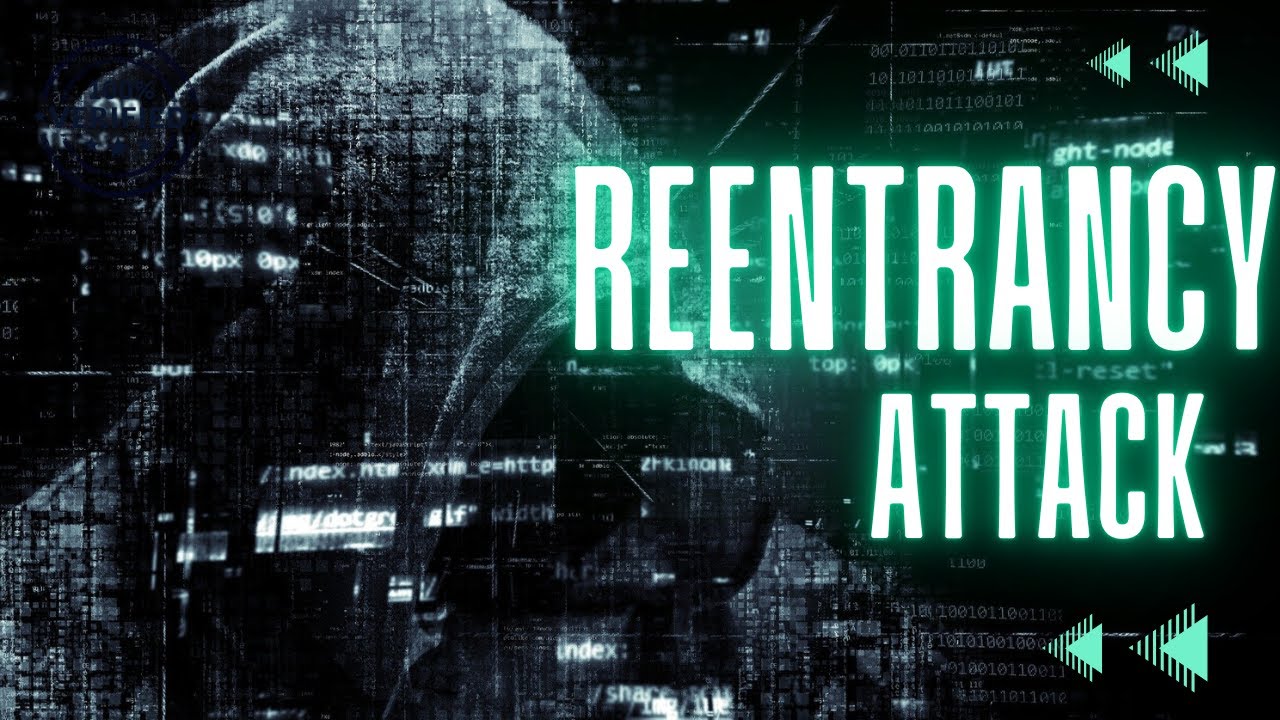
Introduction:
A reentrancy attack is a type of vulnerability that can occur in a smart contract, where an attacker exploits the contract by repeatedly calling a function before the initial execution of that function is complete and the state of the contract variable is updated. This allows the attacker to drain funds from the contract and potentially cause significant financial loss to the organization. The attacker can manipulate the contract's state in such a way that it bypasses the intended logic, enabling them to withdraw more funds than they should be able to. Proper safeguards and coding practices are essential to prevent such vulnerabilities and protect the integrity of the smart contract.
How does this happen?
Reentrancy vulnerabilities happen when a contract calls an external contract and continues executing before the external contract finishes. This lets an attacker call the contract repeatedly and manipulate its state.
Example of a Vulnerable Contract
Let's consider a simple example of a vulnerable smart contract to illustrate how a reentrancy attack can occur. Below is a basic Solidity contract that allows users to deposit and withdraw Ether.
pragma solidity ^0.8.0;
contract VulnerableContract {
mapping(address => uint) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint _amount) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
(bool success, ) = msg.sender.call{value: _amount}("");
require(success, "Transfer failed");
balances[msg.sender] -= _amount;
}
}
In this contract, users can deposit Ether into their account and withdraw it later. However, the withdraw
function is vulnerable to a reentrancy attack. Here's how an attacker can exploit this vulnerability:
The attacker first deposits some Ether into the contract.
The attacker then creates a malicious contract that calls the
withdraw
function of the vulnerable contract.In the malicious contract, the fallback function is designed to call the
withdraw
function of the vulnerable contract again before the initialwithdraw
call completes.This reentrant call allows the attacker to withdraw more Ether than they originally deposited, as the balance is only updated after the Ether transfer is completed.
Here is an example of a malicious contract that exploits the vulnerability:
pragma solidity ^0.8.0;
import "./VulnerableContract.sol";
contract Attacker {
VulnerableContract public vulnerableContract;
constructor(address _vulnerableContractAddress) {
vulnerableContract = VulnerableContract(_vulnerableContractAddress);
}
function attack() public payable {
vulnerableContract.deposit{value: msg.value}();
vulnerableContract.withdraw(msg.value);
}
fallback() external payable {
if (address(vulnerableContract).balance >= msg.value) {
vulnerableContract.withdraw(msg.value);
}
}
}
In this example, the attacker deposits Ether into the vulnerable contract and then calls the withdraw
function. The fallback function in the Attacker
contract repeatedly calls the withdraw
function, draining the Ether from the vulnerable contract.
To prevent such vulnerabilities, it is crucial to follow best practices, such as updating the state variables before making external calls or using the Checks-Effects-Interactions pattern. Here is a revised version of the withdraw
function that mitigates the reentrancy attack:
function withdraw(uint _amount) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
balances[msg.sender] -= _amount;
(bool success, ) = msg.sender.call{value: _amount}("");
require(success, "Transfer failed");
}
By updating the balance before making the external call, we ensure that the contract's state is correctly updated, preventing the attacker from exploiting the reentrancy vulnerability.
Use of OpenZeppelin's ReentrancyGuard to Prevent Such Attacks:
To further enhance the security of your smart contracts and prevent reentrancy attacks, you can use OpenZeppelin's ReentrancyGuard
contract. This contract provides a simple yet effective way to prevent reentrant calls to a function.
First, you need to import the ReentrancyGuard
contract from the OpenZeppelin library:
import "@openzeppelin/contracts/security/ReentrancyGuard.sol";
Next, make your contract inherit from ReentrancyGuard
:
contract SecureContract is ReentrancyGuard {
mapping(address => uint) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint _amount) public nonReentrant {
require(balances[msg.sender] >= _amount, "Insufficient balance");
balances[msg.sender] -= _amount;
(bool success, ) = msg.sender.call{value: _amount}("");
require(success, "Transfer failed");
}
}
In this example, the nonReentrant
modifier is applied to the withdraw
function. This modifier ensures that the function cannot be called again until the first call is completed, effectively preventing reentrancy attacks.
By using ReentrancyGuard
, you add an extra layer of protection to your contract, making it more secure against potential exploits. This approach, combined with best practices like updating state variables before making external calls, helps ensure the robustness and reliability of your smart contracts.
Conclusion
Implementing security measures in smart contracts is essential to safeguard against various types of attacks, including reentrancy attacks. In this guide, we demonstrated how to use the ReentrancyGuard
contract from the OpenZeppelin library to add a layer of protection to your smart contracts. By inheriting from ReentrancyGuard
and applying the nonReentrant
modifier to vulnerable functions, such as the withdraw
function in our example, you can effectively prevent reentrancy attacks.
The ReentrancyGuard
contract works by preventing a function from being called again until its initial execution is complete. This is critical in scenarios where malicious actors may try to exploit reentrancy vulnerabilities to drain funds or manipulate contract behavior.
Moreover, it is important to follow best practices, such as updating state variables before making external calls, to further strengthen the security of your smart contracts. Thoroughly testing your contracts and conducting security audits can also help identify and mitigate potential vulnerabilities.
By incorporating these security techniques, you can build more robust and reliable smart contracts, ensuring the safety of your users' funds and data. Always stay updated with the latest security recommendations and continuously improve your contracts to address emerging threats.:
Subscribe to my newsletter
Read articles from Ashish Kumar Sahoo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Ashish Kumar Sahoo
Ashish Kumar Sahoo
I am a passionate Web3.js Ambassador and smart contract developer with over 2 years of hands-on experience building decentralized applications (DApps) and crafting secure smart contracts using Solidity. My expertise spans across Ethereum-compatible protocols, Uniswap V3, and Chainlink integrations, where I have developed solutions with a focus on performance, scalability, and security. With a strong background in frontend (React.js, Next.js) and backend (Node.js) development, I seamlessly bridge the gap between user interfaces and blockchain ecosystems, leveraging libraries like ethers.js and web3.js to create intuitive and functional experiences. My technical journey also includes building innovative blockchain projects such as Fluid Pass and Delta-X Token, which solve real-world problems using crypto streaming, cross-chain interoperability, and decentralized finance (DeFi) mechanisms. Currently, I am honing my skills in advanced blockchain tools like Foundry and exploring Rust to expand my smart contract expertise into platforms like CosmWasm. With a keen interest in decentralized finance and a vision to enhance blockchain security, I am committed to contributing to the evolution of Web3 technology through secure code, rigorous audits, and innovative projects.