Best practices for improving SEO in a NextJs application built using app router
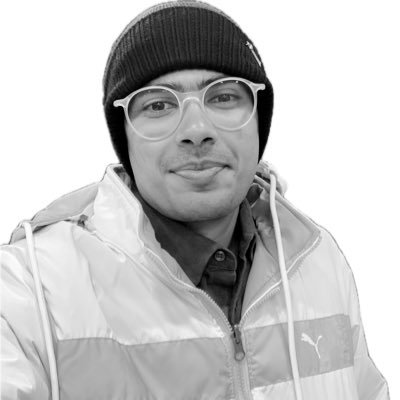
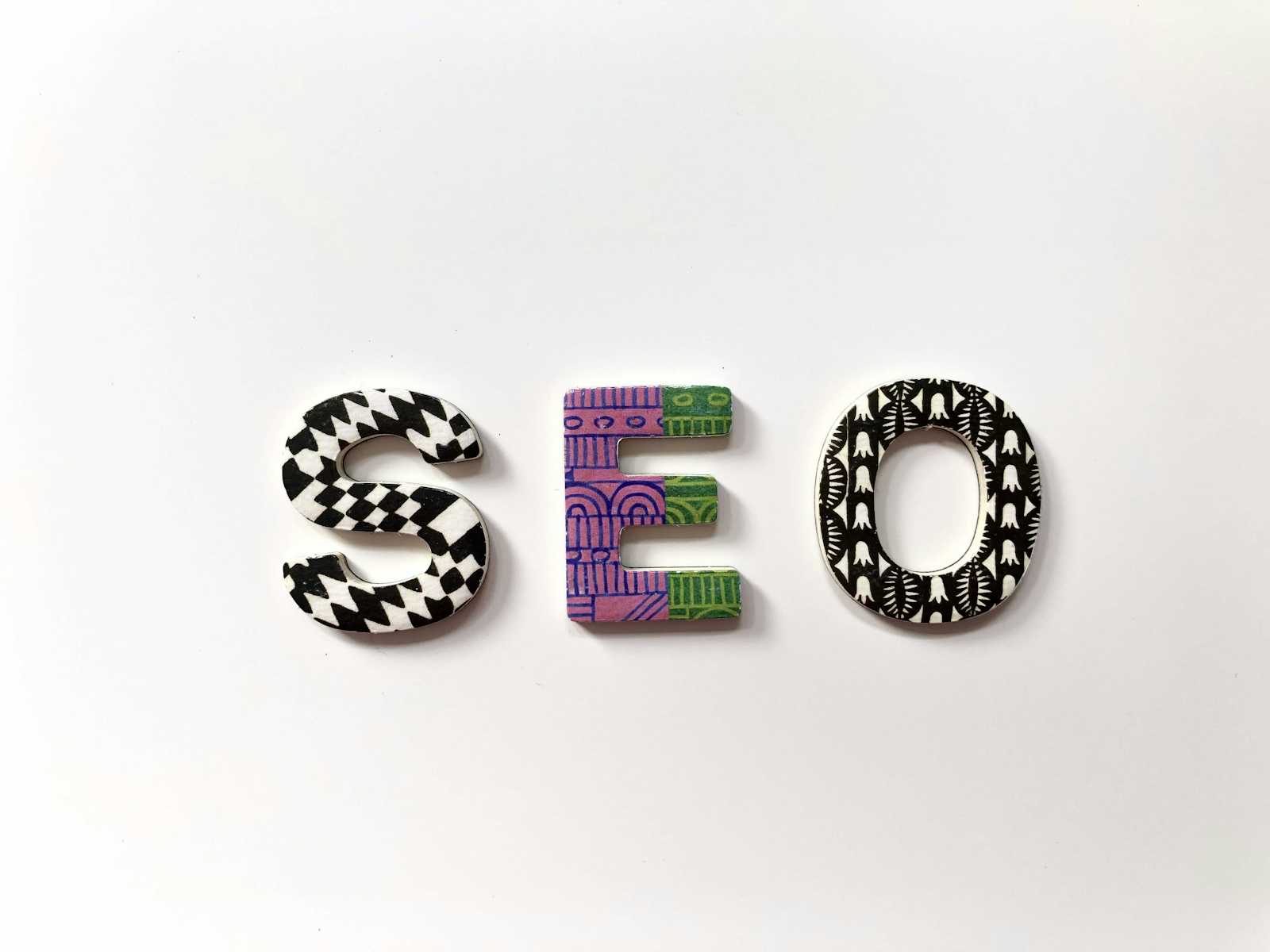
Some best practices within App Router are contributing to letting search engines crawl, index, and rank your site effectively. The following are a number of key strategies for improving SEO in your Next.js application:
1. Server Side Rendering and Static Site Generation
SSR: Dynamically generated pages—so it considers the request—it provides, therefore, in no time, content crawlable for search engines. SSG: Targeting those pages that are not dynamic—Static Site Generation—not frequently updated, fast load times, content already available to the crawlers in advance.
2. Meta Tags and Open Graph Tags
Title Tags: Another title tag and its description is supposed to be on every page.
Meta Descriptions: A short and relevant meta description of the pages.
Open Graph Tags: The Open Graph tags are used to add richer structure to your pages when shared on social media.
import Head from 'next/head';
const MyComponent = () => (
<Head>
<title>My Page Title</title>
<meta name="description" content="My page description" />
<meta property="og:title" content="My Page Title" />
<meta property="og:description" content="My page description" />
<meta property="og:type" content="website" />
</Head>
);
3. Canonical Tags
Adding canonical tags avoids duplicate content and pins that version of the page as preferred for search engines.
import Head from 'next/head';
const MyComponent = () => (
<Head>
<link rel="canonical" href="https://www.example.com/my-page" />
</Head>
);
4. Structured Data
Use schema.org structured data to describe what kind of content is coming from your pages. Information regarding structured data should be added in JSON-LD format.
import Head from 'next/head';
const MyComponent = () => (
<Head>
<script
type="application/ld+json"
dangerouslySetInnerHTML={{
__html: JSON.stringify({
"@context": "https://schema.org",
"@type": "WebPage",
"name": "My Page Title",
"description": "My page description",
}),
}}
/>
</Head>
);
5. Performance Optimization
Image Optimization: Use
next/image
to solve all the image loading issues it can.Code Splitting: Apply dynamic imports to help trim down the initial load time to a minimum.
Caching: Proper caching using HTTP headers.
6. URL Structure and Routing
This will contain clean and readable URLs targeting the keywords for better SERP position. Proper settings for dynamic routes and dynamic paths for SEO-friendly URLs in Next.js.
import { useRouter } from 'next/router';
const Post = () => {
const router = useRouter();
const { id } = router.query;
return <p>Post: {id}</p>;
};
7. Internal Linking
Logically and clearly structuring inner linking makes some layers of hierarchy or other relations smoothly explained to the search engines.
8. XML Sitemaps
Generate an XML sitemap and send it over to the search engines for them to more easily discover the pages and index those that are necessary.
import { SitemapStream, streamToPromise } from 'sitemap';
import { Readable } from 'stream';
const sitemap = async (req, res) => {
const links = [{ url: '/', changefreq: 'daily', priority: 0.3 }];
const stream = new SitemapStream({ hostname: `https://example.com` });
res.writeHead(200, { 'Content-Type': 'application/xml' });
const xmlString = await streamToPromise(Readable.from(links).pipe(stream)).then((data) =>
data.toString()
);
res.end(xmlString);
};
export default sitemap;
9. Mobile-Friendliness
Make it responsive by following responsive design best practices. This is because Google gives mobile-friendly websites a priority in its search results.
10. Monitoring and Analytics
Track how your site is performing, and where there is room for improvement from Google Analytics and Google Search Console.
These best practices, when used within your project, will greatly enhance SEO in a Next.js application and make your application discoverable to search engines.
I have personally used these strategies in building my AI Interviewing app project, Recroo AI, and they have been providing great results so far.
Subscribe to my newsletter
Read articles from Vivek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
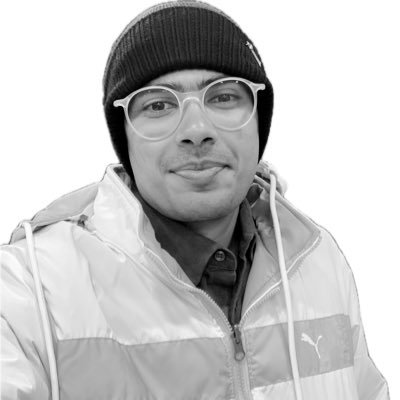
Vivek
Vivek
Curious Full Stack Developer wanting to try hands on ⌨️ new technologies and frameworks. More leaning towards React these days - Next, Blitz, Remix 👨🏻💻